Spring JPA Cardinality
Table of contents
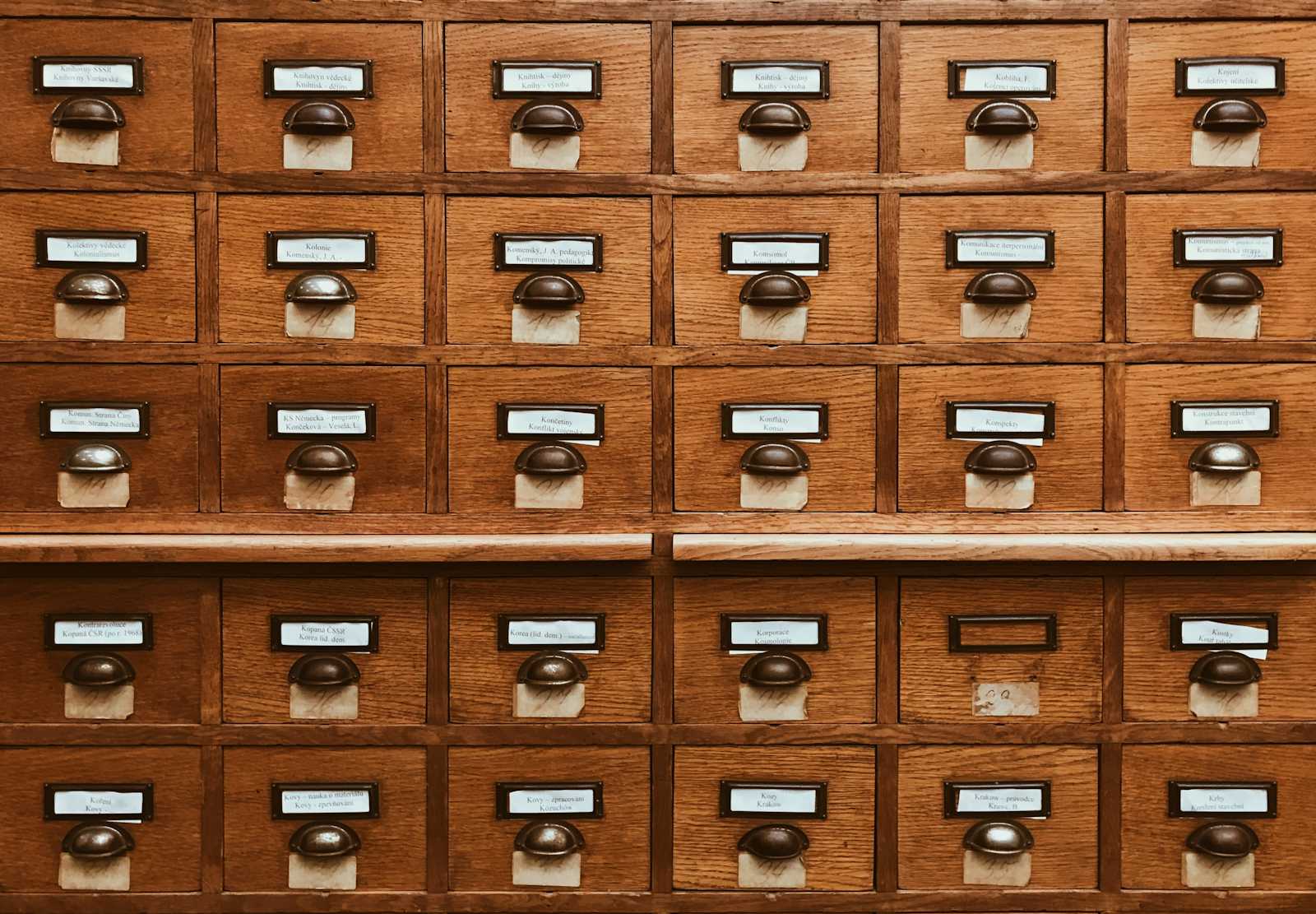
Spring JPA Cardinality refers to the relationship between two entities regarding how many instances of one entity can be associated with a single instance of another entity. Here's a breakdown with code examples:
- One-to-One (OneToOne): One entity instance can be associated with at most one instance of another entity, and vice versa.
@Entity
public class User {
@Id
private Long id;
@OneToOne(mappedBy = "user")
private Account account;
// Getters and Setters
}
@Entity
public class Account {
@Id
private Long id;
@OneToOne
@JoinColumn(name = "user_id") // Optional for defining foreign key column
private User user;
// Getters and Setters
}
One-to-Many (OneToMany): One entity instance can be associated with many instances of another entity, but a single instance of the other entity can only be associated with one instance of the first entity.
@Entity public class Instructor { @Id private Long id; @OneToMany(mappedBy = "instructor") private List<Course> courses; // Getters and Setters } @Entity public class Course { @Id private Long id; @ManyToOne @JoinColumn(name = "instructor_id") // Optional for defining foreign key column private Instructor instructor; // Getters and Setters }
- Many-to-One (ManyToOne): Similar to One-to-Many, but reversed. Many entity instances can be associated with one instance of another entity.
@Entity
public class Order {
@Id
private Long id;
@ManyToOne
@JoinColumn(name = "customer_id") // Optional for defining foreign key column
private Customer customer;
// Getters and Setters
}
@Entity
public class Customer {
@Id
private Long id;
@OneToMany(mappedBy = "customer")
private List<Order> orders;
// Getters and Setters
}
Many-to-Many (ManyToMany): Many entity instances can be associated with many instances of another entity. This usually involves a separate join table to manage the relationships.
@Entity public class Student { @Id private Long id; @ManyToMany(mappedBy = "students") private List<Course> courses; // Getters and Setters } @Entity public class Course { @Id private Long id; @ManyToMany @JoinTable(name = "student_course", joinColumns = @JoinColumn(name = "course_id"), inverseJoinColumns = @JoinColumn(name = "student_id")) // Define join table details private List<Student> students; // Getters and Setters }
Subscribe to my newsletter
Read articles from Abdulla Al Saimun directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
Abdulla Al Saimun
Abdulla Al Saimun
Hello, I'm Abdulla Al Saimun, a dedicated Java Spring Boot Developer with two years of hands-on experience in crafting scalable and efficient software solutions. Specializing in microservices architecture, I seamlessly integrate React Material UI for captivating front-end experiences, while leveraging Oracle SQL for robust and optimized database management. Passionate about problem-solving in the dynamic realm of software development, I thrive on translating ideas into impactful applications. My journey is fueled by the commitment to excellence, continuous learning, and a profound love for overcoming challenges in the ever-evolving landscape of technology