A Developer’s Intro to Huddle01 SDK and Ideas to Build.
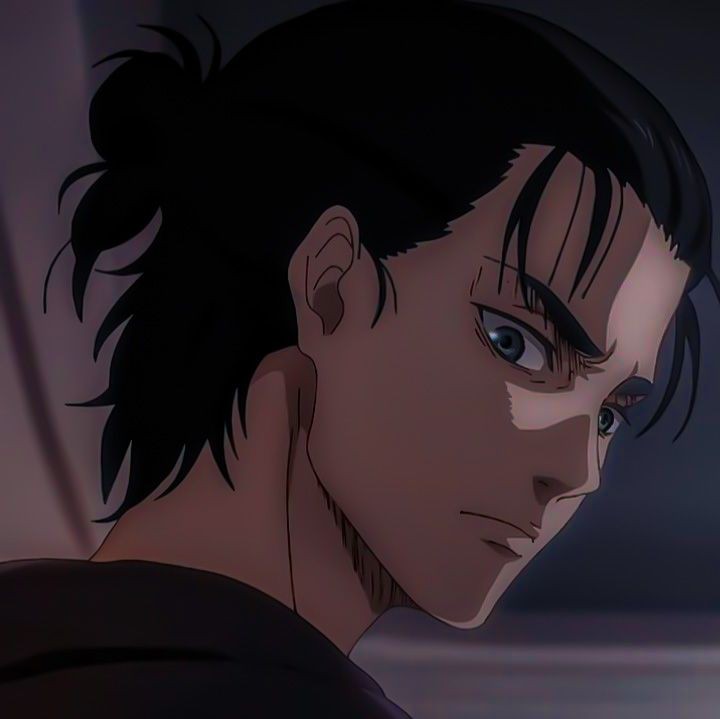
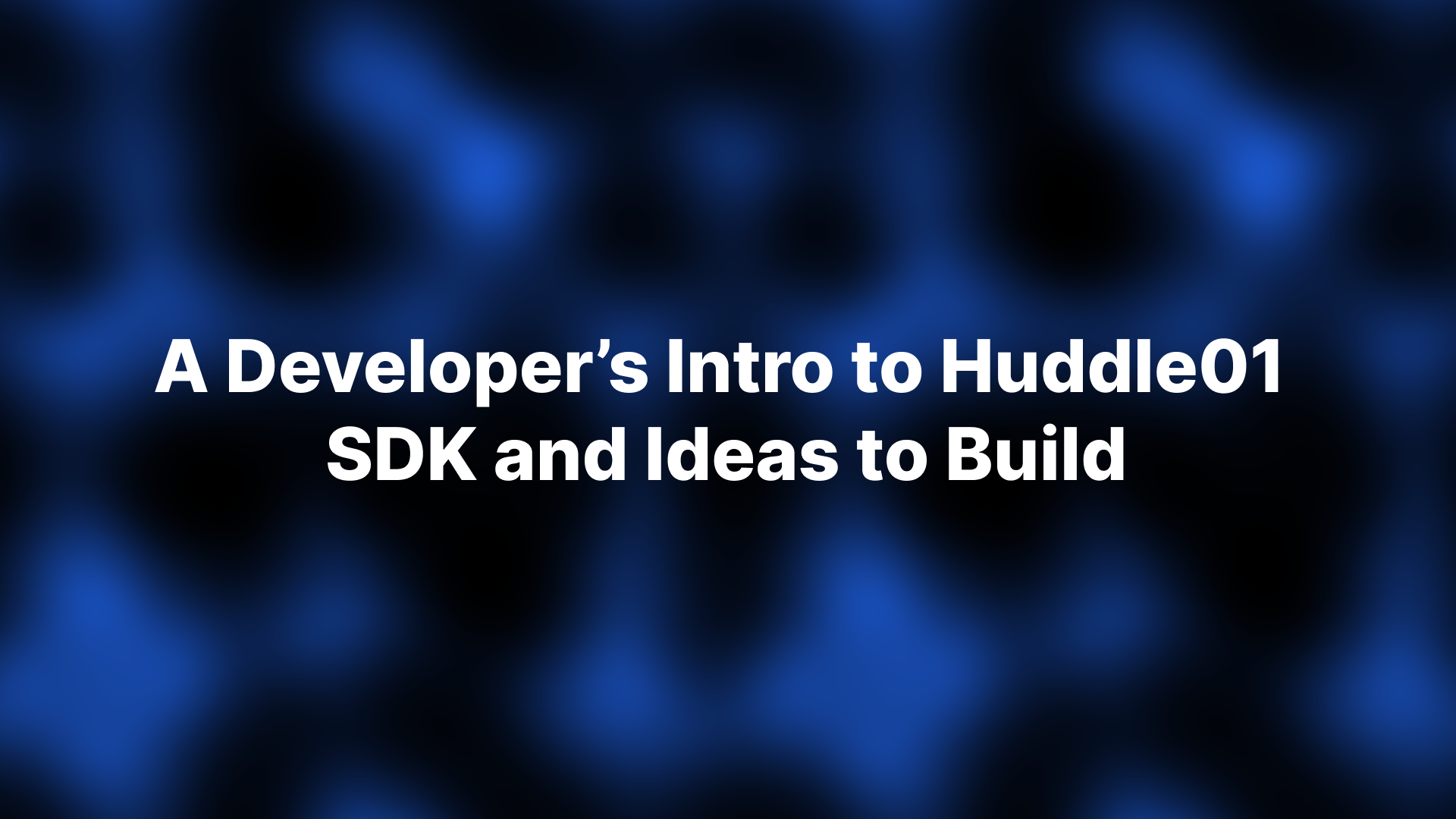
Huddle01
Huddle is a DePIN(Decentralised Physical Infrastructure Network) who are bringing privacy, security and speed to WebRTC using web3 infrastructure and the dRTC network. Huddle provides this functionalities using three main components.
The SDK(s).
The Huddle01 Platform.
The Huddle01 dRTC Network.
But wait a minute! WTF is a DePIN ?
As the name suggests DePIN(s) are a network of physical infrastructure hardware devices which are connected to each other in a decentralised manner using blockchain technologies which provide a transparent, efficient and user centric access to resources.
Exploring Huddle01
Now, we have understood what are DePIN(s) and how they benefit the blockchain community and the users. Let’s discuss about dRTC and how it works.
dRTC and it’s advantages
dRTC stands for decentralised Real-Time Communication.
One of many problems with most of the today’s infra is that it is generally owned by one entity for eg. AWS( owned by Amazon), GCP (owned by Google) etc. Here are some more problems with this infra:
Vulnerable : Due to the centralised structure of these servers, they are susceptible to hacks which puts user’s data and privacy in danger.
Latency : Due to these server being in a special location, it introduces latency problems or lag, which impacts the communication quality.
Profit Driven Model : these platforms prioritise profit, which sometimes might not align with user interests, resulting in intrusive ads or data selling.
Here dRTC comes to the rescue by offering following solutions.
Security : Data encryption and Blockchain technology ensures secure communication and user privacy.
More Scalable : The network can handle a large number of users without losing performance. Huddle01 dRTC network can be 95% cheaper than AWS.
User Control : Users have more control over their data and communication experience.
Huddle01 has built a dRTC protocol that forms the foundation for their video conferencing app and communication infrastructure. All the data streams go through community powered nodes and provides end to end encryption. The node operators earn $HUDL for providing the infra to ensure secure, private and fast communication experience to users.
Below is a picture of Balaji Srinivasan at FIL Bangalore 2023 organised by Huddle.
Deep Diving into Huddle01 SDK (v2)
Huddle has migrated their SDK’s from v1 to v2. for information about previous versions go here : huddle v1 docs
Huddle01 provides you with SDK(s) and API’s to create high quality and secure communication experiences for both mobile and web applications. Huddle01 provides two ways to integrate the huddle infra to your app which are through SDKs or iFrame package.
The fastest and easiest way to integrate huddle into your website is by using the iframe package which integrates the whole huddle web app into your website and you are ready to communicate with your employees or clients in real time. The steps are as follows:
To integrate Huddle01 iframe into a NextJS web app :
pnpm create next-app@latest
cd project_name
pnpm add @huddle01/iframe # adding the iframe package
In the page.tsx
file , delete the contents between the <main> .... </main>
tag and replace it with
import { HuddleIframe } from "@huddle01/iframe";
...
<HuddleIframe roomUrl="https://iframe.huddle01.com/YOUR_ROOM_ID" className="w-full aspect-video" />
...
pnpm dev
Go to localhost:3000 to see the web app running.
You are almost ready to go now. Now using different methods provided by Huddle you can customise it to you needs from changing colour scheme, background etc to live streaming the meeting to a platform.
You can also find an official demo here : https://customize-iframe-example.vercel.app/
Now let's build a real app ourselves. We'll be building a react based web app using NextJS and will be including Huddle's ReactJS SDK. Let's get started.
Adding Huddle's react SDK to your app.
Initialise the project.
bun create next-app@latest #you can also use npm,pnpm or yarn
Go to https://docs.huddle01.com/docs/api-keys and get your API Key and Project ID and save it in
.env
file.# .env NEXT_PUBLIC_PROJECT_ID="YOUR_PROJECT_ID" API_KEY="YOUR_API_KEY"
Installing the Huddle01 React SDK in your app.
bun add @huddle01/react @huddle01/server-sdk
Creating an instance of
HuddleClient
and Wrapping your app with<HuddleProvider> </HuddleProvider>
// layout.tsx "use client"; import { HuddleClient, HuddleProvider } from "@huddle01/react"; ... const huddleClient = new HuddleClient({ projectId: process.env.NEXT_PUBLIC_PROJECT_ID!, }); export default function RootLayout({ children, }: Readonly<{ children: React.ReactNode; }>) { return ( <html lang="en"> <HuddleProvider client={huddleClient}> <body className={inter.className}>{children}</body> </HuddleProvider> </html> ); }
To join a room we need a roomId which we can create by calling the Create Room API . This code calls the API with your
API KEY
included in theheader
and returns theroomId
as a response result.// utils/createRoom.ts "use server"; export const createRoom = async () => { const response = await fetch("https://api.huddle01.com/api/v1/create-room", { method: "POST", body: JSON.stringify({ title: "Huddle01 Room", }), headers: { "Content-type": "application/json", "x-api-key": process.env.API_KEY!, }, cache: "no-cache", }); const data = await response.json(); const roomId = data.data.roomId; return roomId; };
After the
roomId
generation, we need anaccessToken
to join a room. Create aroute.ts
file intoken
folder. Using@huddle01/server-sdk ,
it generates and returns atoken
with the status code as a response.// token/route.ts import { AccessToken, Role } from "@huddle01/server-sdk/auth"; export const dynamic = "force-dynamic"; export async function GET(request: Request) { const { searchParams } = new URL(request.url); const roomId = searchParams.get("roomId"); if (!roomId) { return new Response("Missing roomId", { status: 400 }); } const accessToken = new AccessToken({ apiKey: process.env.API_KEY!, roomId: roomId as string, role: Role.HOST, permissions: { admin: true, canConsume: true, canProduce: true, canProduceSources: { cam: true, mic: true, screen: true, }, canRecvData: true, canSendData: true, canUpdateMetadata: true, } }); const token = await accessToken.toJwt(); return new Response(token, { status: 200 }); }
To facilitate joining and leaving the room we can use the
useRoom
hook in the SDK which different methods such asjoinRoom
,leaveRoom
etc. Its recommended to sendroomId
over[url]/roomId
. for NextJS you can make a folder named[roomId]
and a file in it namedpage.tsx
and paste the following code .import { useRoom } from '@huddle01/react/hooks'; const App = () => { const { joinRoom, leaveRoom } = useRoom({ onJoin: () => { console.log('Joined the room'); }, onLeave: () => { console.log('Left the room'); }, }); return ( <div> <button onClick={() => { joinRoom({ roomId: 'YOUR_ROOM_ID', token: 'YOUR_ACCESS_TOKEN' }); }}> Join Room </button> <button onClick={leaveRoom}> Leave Room </button> </div> ); };
for sending media across the participant you can use
useLocalVideo
,useLocalAudio
anduseLocalScreenShare
hook.import { useLocalVideo, useLocalAudio, useLocalScreenShare } from '@huddle01/react/hooks'; const App = () => { const { stream, enableVideo, disableVideo, isVideoOn } = useLocalVideo(); const { stream, enableAudio, disableAudio, isAudioOn } = useLocalAudio(); const { startScreenShare, stopScreenShare, shareStream } = useLocalScreenShare(); return ( <div> {/* Webcam */} <button onClick={() => { isVideoOn ? disableVideo() : enableVideo() }}> Fetch and Produce Video Stream </button> {/* Mic */} <button onClick={() => { isAudioOn ? disableAudio() : enableAudio(); }}> Fetch and Produce Audio Stream </button> {/* Screen Share */} <button onClick={() => { shareStream ? stopScreenShare() : startScreenShare(); }}> Fetch and Produce Screen Share Stream </button> </div> ); };
And for receiving the audio, video and screen capture streams you can do the following
"use client"; import { usePeerIds, useRemoteVideo, useRemoteAudio, useRemoteScreenShare, } from "@huddle01/react/hooks"; import { Audio, Video } from "@huddle01/react/components"; import { FC } from "react"; import { Role } from "@huddle01/server-sdk/auth"; interface RemotePeerProps { peerId: string; } const RemotePeer: FC<RemotePeerProps> = ({ peerId }) => { const { stream: videoStream } = useRemoteVideo({ peerId }); const { stream: audioStream } = useRemoteAudio({ peerId }); const { videoStream: screenVideoStream, audioStream: screenAudioStream } = useRemoteScreenShare({ peerId }); return ( <div> {videoStream && <Video stream={videoStream} />} {audioStream && <Audio stream={audioStream} />} {screenVideoStream && <Video stream={screenVideoStream} />} {screenAudioStream && <Audio stream={screenAudioStream} />} </div> ); }; const ShowPeers = () => { const { peerIds } = usePeerIds({ roles: [Role.HOST, Role.CO_HOST] }); // Get Hosts And Cohost's peerIds console.log("show peers called"); console.log(peerIds); return ( <div> {peerIds.map((peerId) => { return <RemotePeer peerId={peerId} key={peerId} />; })} </div> ); }; export default ShowPeers;
You're now all set.
Leaving useRoom
aside , Huddle01 also provides more powerful hooks such as useRoomControls
, usePeerIds
, useDevices
which let's you customise and tailor it to your needs perfectly. Apart from ReactJS SDK, Huddle also have React Native SDK and Flutter SDK for mobile applications and Server SDK for performing protected admin actions on server side.
Apart from SDK's Huddle01 offers different powerful API's such as Token Gated Create Room API, which allows you to create a room where access is restricted based on the type and value of a token. Other API's include Get Key Metrics API
, Live Meetings API
, Get Recordings API
and much more.
You can learn all about it here : https://docs.huddle01.com/docs
Now if I come to think what can people build with this, I have three ideas right now :
Generally, if you are building a product that involves video and audio communication, using Huddle01 can be a very good option coz it provides the webRTC infra at 95% cheaper cost than AWS, more securely and fast.
Private Virtual Events Platform : Using Huddle01 SDK's, we can make a decentralised platform where the creators can hold events with their loyal audience and the audience get rewarded through NFT's, the users can pay the creator using cryptocurrencies. The most important thing is everything here will be secure, not owned by a central authority, and transparent.
Decentralised health Platform : Using huddle as the fundamental infra, we can make a health platform where the patients can held private, secure, end to end encrypted meetings with their doctors. all the medical records remain on chain safe and secure.
Decentralised Live Streaming : A platform leveraging huddle01 SDK to power the infra of the platform which will provide low latency, high quality live streams at much cheaper cost than the traditional centralised servers. and the user will have control over their personal data and content rights. we can also implement decentralised content moderation systems for safety.
A lot of apps are already using Huddle01, check'em out here : https://docs.huddle01.com/docs/showcase
To know more about Huddle01 , here are some resources
Huddle01 Youtube Channel : https://www.youtube.com/@huddle0174
Huddle01 Hashnode Blog : Huddle01
Huddle01's twitter/x : https://twitter.com/huddle01com
me : shivam.ing
Subscribe to my newsletter
Read articles from shivam directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
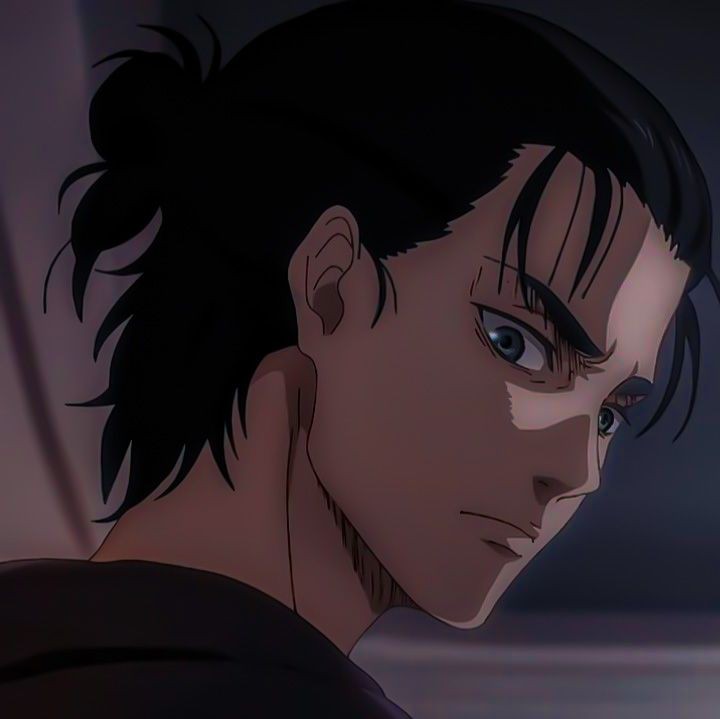
shivam
shivam
dev etc etc.