How to 'Git' ?

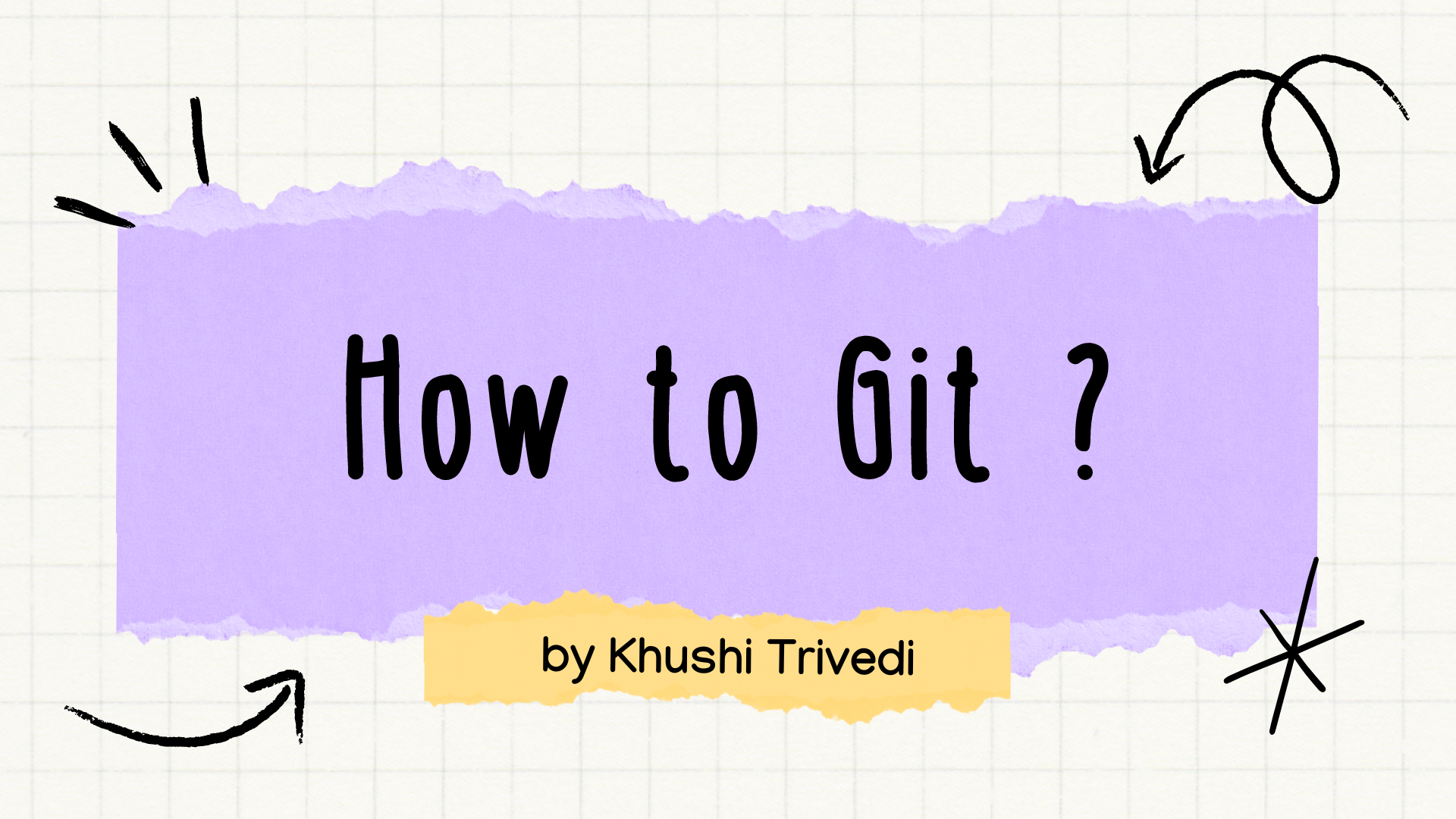
This blog is specifically for the total newbies, who are either confused about the version control system, or have been a total beginner in tech.
NOTE: GIT and GITHUB are two different things! (Git isn't a part / subset of GitHub, or vice versa)
What is Git?
Linus Torvalds, the developer who created Linux OS, created Git in 2008 with the intent to create his very own distributed-version-control-system that work quite fast & efficient. He wished to keep it free and Open-Source for all the users globally.
Let's explore more about Git in further headings.
Installation
Git : works as a software which is to be downloaded on Windows/ Mac/ Linux. https://git-scm.com/
💻 Video Tutorial to install Git for WINDOWS: https://www.youtube.com/embed/nqCY3njHg2U?start=258&end=549
💻Video Tutorial to install Git for MaC : https://www.youtube.com/embed/nqCY3njHg2U?start=607&end=813
GitHub: could be optional to download. We've got it's website, desktop app as well as a mobile app to operate GitHub. It could be operated from any Browser or Operating System. https://github.com/
Why do we use Git?
Big organizations like Microsoft, Apple, & Google, etc. have hundreds of employees working on the same project. Then how do they get to know 'who did which task?' (detailed explanation here)
Git is a distributed version-control system. That means it is used for tracking changes in the source code in software development.
It allows multiple developers to work on the same project and track every change, together. Git is called 'distributed' because it gives it's users the flexibility to work independently, without relying upon a central server.
Here's a list of things Git can help us with. We can call them, FEATURES OF GIT
Similar to this, we use Git for tracking all the changes from the first line of code, to the last one.
Why is Git called version-control system?
Consider you are creating a Mobile App with a team of 4 members. Now, you decided to add a new feature i.e. a ChatBot to your app.
You and your team added it, but somehow it's not working- and you finally decide that you want to 'UNDO' your changes.
So, Git helps you to maintain versions of your code. And you can easily move to any version at any point of time (previous or next). Without Git, it becomes very tough to manually maintain every version, and move back to it!
Understanding Stages within Git
As mentioned, you were working with your friends in a team of 4, and building a mobile app. Hence, in the technical terminology you are at the WORKING DIRECTORY.
Now, once your code is ready, you would like to "SAVE" your changes. In software development we have a different way to 'save' or 'save as'.
Saving changes in Git is a TWO-STEP process, similar as a 2-factor authentication of the Gmail account login.
If you'd like to save your changes, you will have to give the git add .
command on the Git window (first step). After giving this command, your project will shift to STAGGING AREA.
Second step will to type git commit -m "Name of the changes/work done"
command on the Git window. The message inside could be anything general that you've worked upon. For example "Added a chatbot" or "Updated the nav bar" etc. After this, your code files will successfully be called a RESPOSITORY now.
Understanding by an Example
You might have worked on Microsoft Office Word. Right? And made a document by typing from the first line. If yes, then it'll be really easy for you to understand the working of Git with an example.
Well, whenever you typed the word document, you always save your changes. Similarly, for any update on code, you get to save it as soon as you're done adding it the the code. Git adds those changes, and doesn't overwrite over the last file, thereby updating a version of your code file.
Now, in Microsoft Office Word, whenever we clicked on the 'Save' button or typed 'Ctrl+S' it take us to a pop-up window. It's the second step to select the location & entering the file name.
Similarly for Git, simply replace:
'Ctrl+S' -->
git add .
'Entering File Name' -->
git commit -m "Name of the changes/work done"
How do we operate the Git window?
Good question! Because the git window interface actually looks like this:
But nothing to worry, as we've got a couple of commands to operate Git :
git clone
, git add .
, git merge [branch]
, git commit -m "[]"
, git status
,
git fetch
, git checkout
, git push origin main
, git pull
....etc.
And all you need is to understand these commands without actually memorizing!
You can find the entire list of Git Commands from here: https://education.github.com/git-cheat-sheet-education.pdf
Explaining the Git Commands
In this section, we will look for git terminology and it's commands- including their use case, and examples.
git setup commands
In this, we basically tell the Git program (installed on our local machine, i.e. laptop/PC), the name of person who's making changes in the code.
First we tell the name, and then the email by:
git config --global user.name "FirstName LastName"
(press enter)
git config --global user.email "Your Email"
(press enter)
How to know if the Git command is working?
If your git command works successfully, it will straightaway take you to the next line. It only displays any text in case of an error. Watch this video snippet for understanding in detail :https://www.youtube.com/embed/nqCY3njHg2U?start=2451&end=2686
git init
With git init
, we tell Git to initiate/start tracking the changes to the code files within it's directory. We use this command usually before making a new repository.
(However, simply running git init
doesn't automatically start tracking all files. You need to explicitly add files to the staging area using git add
to start tracking them.)
git clone
We use git clone [url]
command in order to download an online repository to our local computer. For this, we need the URL link to that repository. It could be present on GitHub, GitLab, Bitbucket, or similar platforms.
Fun Fact: by "clone" we basically mean to "download" when we use Git.
git clone "https://github.com/trivedi-khushi/Portfolio-Website-demo-for-gdsc-upv.git"
git status
As the name suggests, git status
tells you the status of your Git repository. The output possibility of this command could be :
which files of your repo have been MODIFIED ,
files that are STAGED (i.e. added to the next commit) ,
& if any of those files are UNTRACKED (i.e. not yet added to Git's version control).
git add
As we've seen before, this is the first step to 'save' changes in Git terminology. Well, git add [File_Name]
is exactly like 'Ctrl+S' in MS Word, besides you need to mention the file name inside the Git command.
NOTE: if you need to save all the files, then you must enter git add .
instead of file name. The dot in this command stands for 'all the files' that belongs to the repository.
For Example: if the file name is '" hashnode.txt " then the git add command will be:
git add hashnode.txt
git commit
This command is the second step while we save the code changes to the repository. git commit -m "Your Message"
could be understood as a final confirmation of saving your changes, and you do this by giving a name to your changes. Just for example, "added the search bar", or :
git commit -m "Updated the CSS animation"
When you enter the git commit
command, it means you've successfully added your changes & input to the repository.
Take it similar like you're adding a puzzle piece while solving it with your friends. Yeah, that's how you add your contribution to the overall code, like adding a piece.
git branch
Before knowing what this command actually does, we must understand the answer to ' what is a branch in git '. Well, branching helps developers to experiment a new feature on the software/project that is being built, by making a copy and not risking the original /source code. This is because we don't want to ruin the main code of the project, so we make a copy of it within the same repository. If the feature works on the copy, we merge it to the original branch of the code using 'pull requests'.
Read this blog for more understanding: How is Branching (git branch) different from Forking on GitHub?
Didn't understand? Let's look into an example to understand git branch
Consider you made some a Soup for family dinner and it's less in some spices. Now, instead of adding any flavors /spices directly to the main soup, we try mixing seasonings, peppers, salt, and other spices to a bowl of soup, instead of the main soup pot. After it has been finalized which spices to be added, and which not - you will finally add the same proportion of selected flavors to the main soup pot.
Similarly, we try to experiment, fix a bug, add a new feature, or bring a new color theme to our existing project. But, instead of risking the whole project for a little new edit, it's safe & better to get a copy of it to test it before adding it to the main & final copy of the code.
Git Branch commands
There are mainly 4 branch commands that is used & mentioned in the cheatseet.
git branch
: used to see the list of all the existing branches of the repository.git branch [new-branch-name]
: it creates a new branch on the selected repo.git checkout
: it's used to checkout for the current branch you are in.git checkout [branch-name]
: it helps you to switch from the current branch to another existing branch of the repository.
for any existing branch named "updates" we can enter:
NOTE: There will always be one branch made automatically called "main". It is the default branch in any repository. Changes made in the 'main branch' will be shown in the final overall output.
git merge
There is a command existing to merge the changes of one branch into another. We can enter the command : git merge [branch-name]
in order to add the changes of [branch-name]
into the branch that you're already working on.
NOTE: We can use this command to combine any branch with the main branch as well.
In that case, it'll be git merge main
: (source- GeeksforGeeks.org)
git pull
The Git Pull command is nothing but updating your local repo with the one that is existing at GitHub/ GitLab's platform.
After entering git pull
the files in your local computer's repository will be updated with the files present in that URL link from where you initially cloned it in your system.
It will make the local repository as same as the remote repository.
git push
'Push' in Git terminology could be understood as 'Upload'. This command is often written in a 4-word form like:
Here, git push origin main
means that we are uploading the changes made to the 'main' branch of the repository. The last word must be the branch name. Note: the git push command is only applicable for the existing branches of the repo.
For instance, if we wanted to upload the code changes to an existing branch called "cloud-updates", then we get to enter the command as:
git push origin cloud-updates
git fetch
This git command is used just for 'fetching' and downloading the information about the 'branched' from the original remote repository. git fetch
Conclusion ❤️
I hope the very concept of a "distributed version-control-system" has been inclined well with the mentioned examples, and snippets.
In the next write-up we will specifically look up to understanding how is Git (version-control) is different from the Open-Source development platforms like GitHub, GitLab, etc. ‼️
In case of any queries, do reach out to Khushi Trivedi 's socials mentioned. ❤️❤️
If this blogpost has been effective enough, do check out my other blogs and video contents ✨
Subscribe to my newsletter
Read articles from Khushi Trivedi directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by

Khushi Trivedi
Khushi Trivedi
Currently working as a Developer Relations Engineer @SuprSend.com A community person surrounded by techies who build with me. Front-end developer now also trying my hands on UX Designing. I'm also an Open Source Technical Writer, and would love to collaborate on Hashnode ❤️