Web Scraping with Python Beautiful Soup
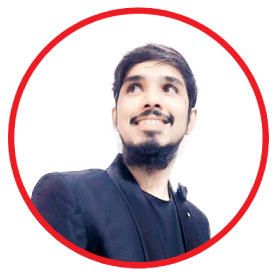
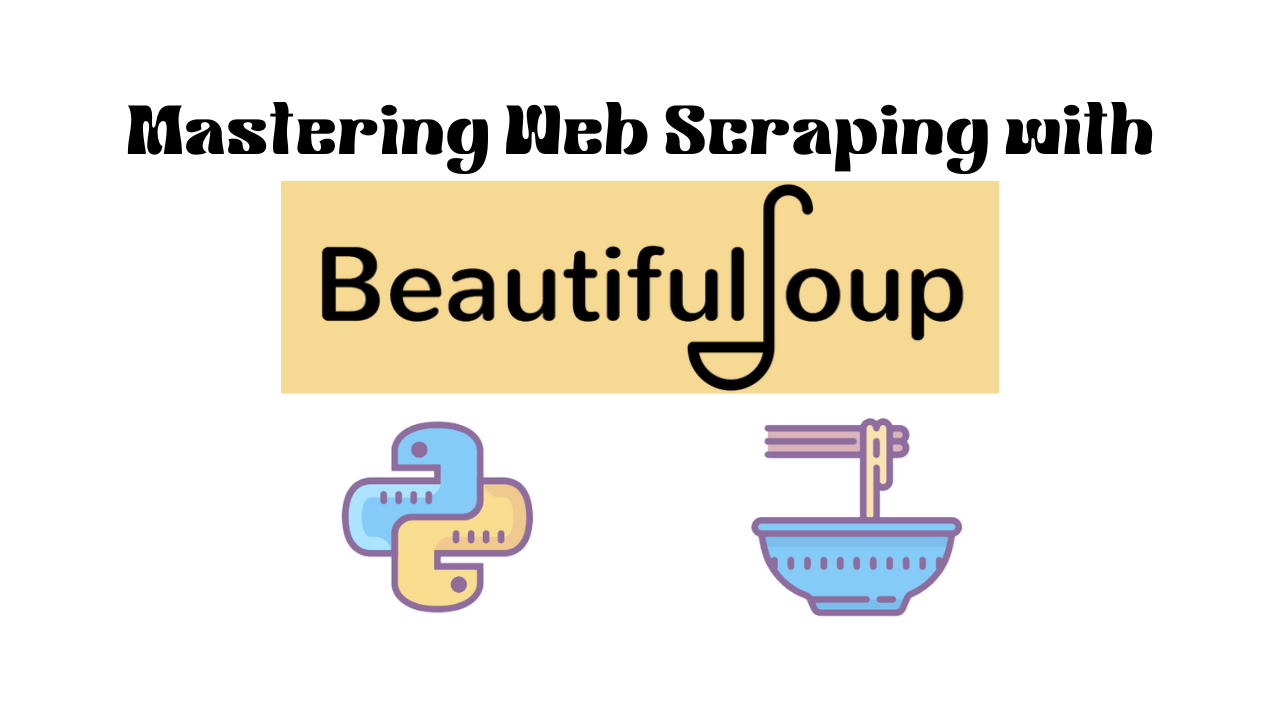
Beautiful Soup is a Python library designed for web scraping HTML and XML files. It offers a convenient way to extract data from web pages by parsing the HTML/XML markup and navigating the document's structure.
Here's why Beautiful Soup is useful for web scraping:
Easy to Use: Simplifies parsing HTML/XML documents for data extraction.
Robust Parsing: Handles poorly formatted markup gracefully.
Powerful Navigation: Offers methods for efficient traversal and searching.
Flexible Extraction: Extracts various data types like text, links, images, etc.
Integration: Easily integrates with other Python libraries like Requests and Pandas.
Community Support: Benefits from a large and active user community with ample resources.
Installation of Beautiful Soup:
Use pip:
pip install beautifulsoup4
To get started with basic usage:
Import the library:
from bs4 import BeautifulSoup
Parse HTML/XML:
soup = BeautifulSoup(html_doc, 'html.parser')
Navigate and extract data:
# Find elements by tag name soup.find('tag') # Find elements by class soup.find_all(class_='class_name') # Find elements by id soup.find(id='element_id') # Access element attributes element['attribute'] # Get text content element.get_text()
Beautiful Soup parses HTML and XML documents by:
Building a Parse Tree: It constructs a parse tree representation of the document, capturing the hierarchical structure of elements.
Parsing Algorithm: Beautiful Soup uses a parsing algorithm to analyze the markup and create the parse tree, identifying tags, attributes, and their relationships.
Flexible Parser: It employs different parsing strategies depending on the underlying parser library chosen (e.g., 'html.parser', 'lxml', 'html5lib'), each with its advantages in speed, flexibility, and compatibility.
Handling Encoding: Beautiful Soup handles different character encodings, ensuring proper interpretation of text content within the document.
Methods for navigating and search
find(tag, attributes): Finds the first element matching the specified tag and attributes.
soup.find('div', class_='content')
find_all(tag, attributes): Finds all elements matching the specified tag and attributes.
soup.find_all('a', href=True)
select(css_selector): Finds elements using CSS selectors.
soup.select('.title')
parent: Navigates to the parent element.
element.parent
contents: Accesses direct children of an element as a list.
element.contents
next_sibling, previous_sibling: Navigates to the next or previous sibling element.
element.next_sibling
descendants: Iterates over all descendants of an element.
for child in element.descendants: print(child)
Handling Encodings and Malformed Markup
- Yes, Beautiful Soup can handle different character encodings and gracefully parse poorly formatted HTML/XML, ensuring accurate interpretation of text content within the document.
Extracting Specific Data
- Utilize Beautiful Soup's methods like find, find_all, and select to target specific elements based on tags, attributes, or CSS selectors. Then extract data by accessing element attributes or text content.
Best Practices for Web Scraping
- Follow best practices such as respecting website's robots.txt, using appropriate user-agent headers, avoiding aggressive scraping, and adhering to website's terms of service to ensure ethical and efficient scraping with Beautiful Soup.
Limitations and Challenges
- Some limitations include the inability to execute JavaScript, reliance on the structure of the HTML/XML document, and occasional difficulties with dynamic content. Challenges may arise from website changes, anti-scraping measures, or rate limiting.
Official Documentation: beautifulsoup4 · PyPI
Subscribe to my newsletter
Read articles from Ahmed Reza directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
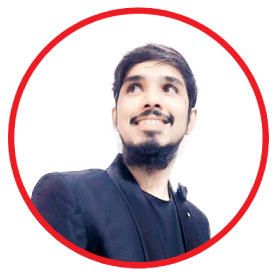