Improving Website Performance with Lazy Loading in JavaScript
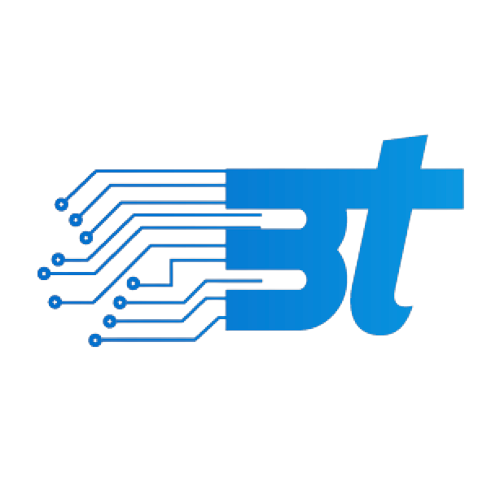
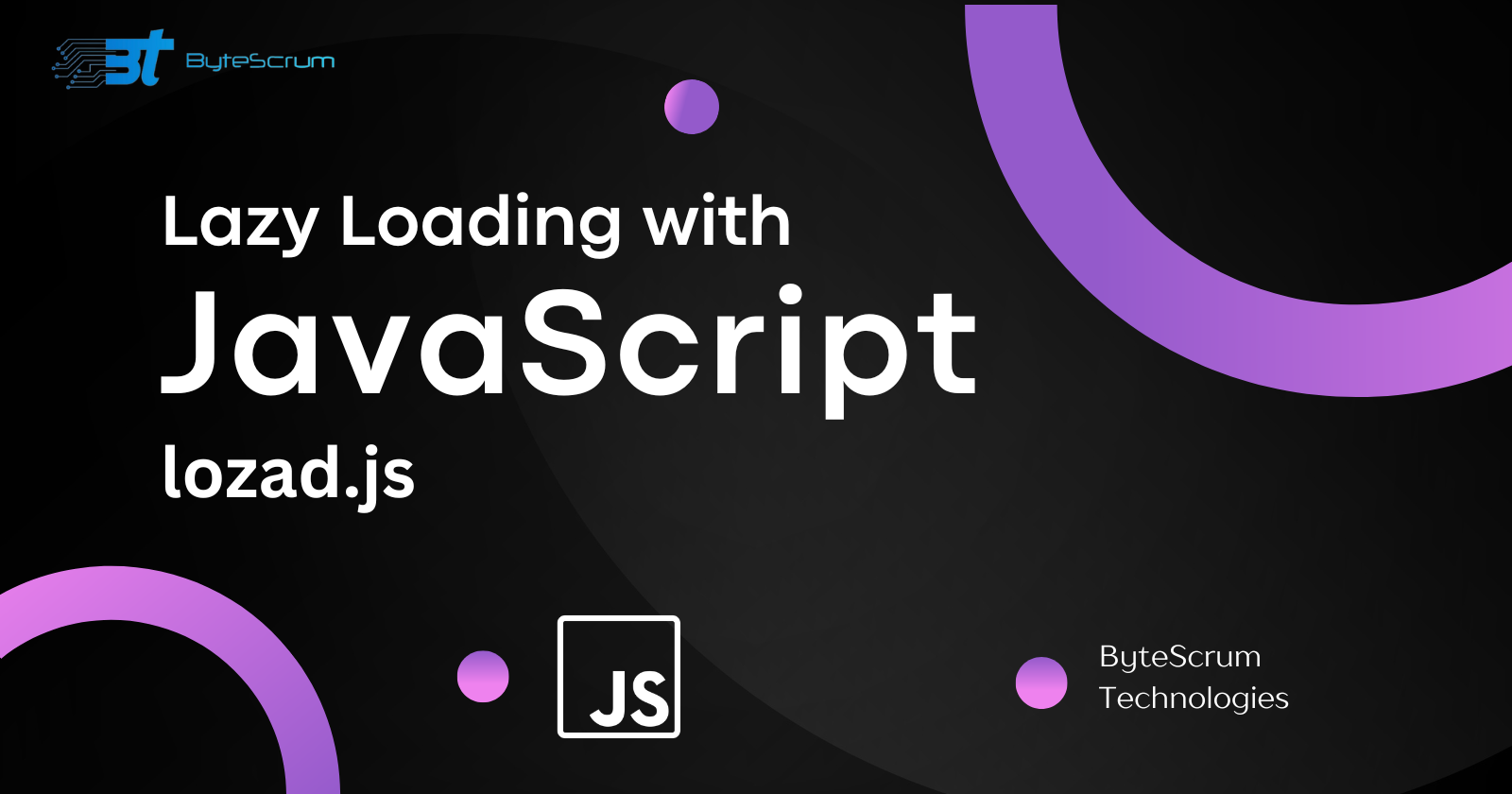
Introduction
Lazy loading is a technique used to defer the loading of non-essential resources at the time the page is initially loaded. This can significantly improve website performance by reducing the initial load time and only loading resources as they are needed. In this guide, we'll explore how to implement lazy loading for images and other resources to improve website speed and user experience.
Benefits of Lazy Loading
Faster Initial Load Time: By deferring the loading of non-essential resources, lazy loading allows the critical content of your website to load quickly, improving the overall user experience.
Reduced Data Transfer: Lazy loading reduces the amount of data transferred over the network, especially for users on slow or limited data connections, leading to faster loading times and reduced data costs.
Improved Page Performance: By loading resources only when they are needed, lazy loading can reduce the strain on the browser and improve the responsiveness of your web pages.
Check some of the best ways to implement the lazy load in javascript below.
Step 1: Identify Resources to Lazy Load
The first step is to identify which resources on your website can benefit from lazy loading. Typically, images, videos, and iframes are good candidates for lazy loading since they often constitute the largest portion of a webpage's size.
Step 2: Install a Lazy Loading Library
There are several JavaScript libraries available that make implementing lazy loading easy. One popular choice is lozad.js
. You can install it via npm:
npm install lozad
Step 3: Initialize the Lazy Loading Library
Once you have installed the library, you need to initialize it in your JavaScript file. Here's an example of how you can do this:
import lozad from 'lozad';
const observer = lozad('.lozad', {
loaded: function(el) {
el.classList.add('fade');
}
});
observer.observe();
In this example, '.lozad'
is the selector for elements you want to lazy load. The loaded
callback is optional and allows you to add a class (e.g., 'fade'
) to the element once it has been loaded.
Step 4: Add the Lazy Loading Class to Elements
Finally, you need to add the lozad
class to the elements you want to lazy load. For example, for images:
<img class="lozad" data-src="path/to/image.jpg" alt="Description">
The data-src
attribute contains the path to the image, which will be loaded when the element comes into view.
Step 5: Testing and Optimization
Once you have implemented lazy loading, test your website to ensure that resources are loading correctly and that there are no performance issues. You may also want to consider optimizing your images and other resources to further improve loading times.
Conclusion
Thank you for reading the blog, please subscribe for more!!
Subscribe to my newsletter
Read articles from ByteScrum Technologies directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
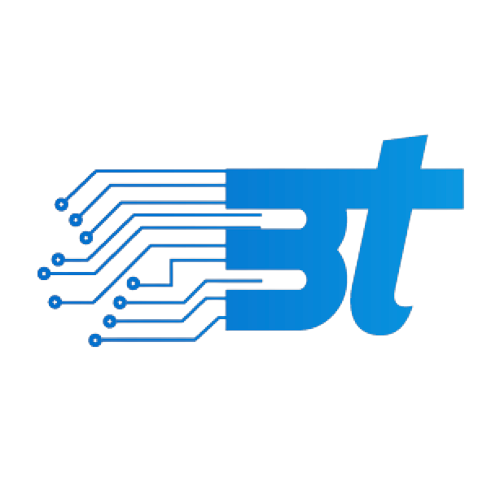
ByteScrum Technologies
ByteScrum Technologies
Our company comprises seasoned professionals, each an expert in their field. Customer satisfaction is our top priority, exceeding clients' needs. We ensure competitive pricing and quality in web and mobile development without compromise.