How to easily create React animations using Framer Motion.
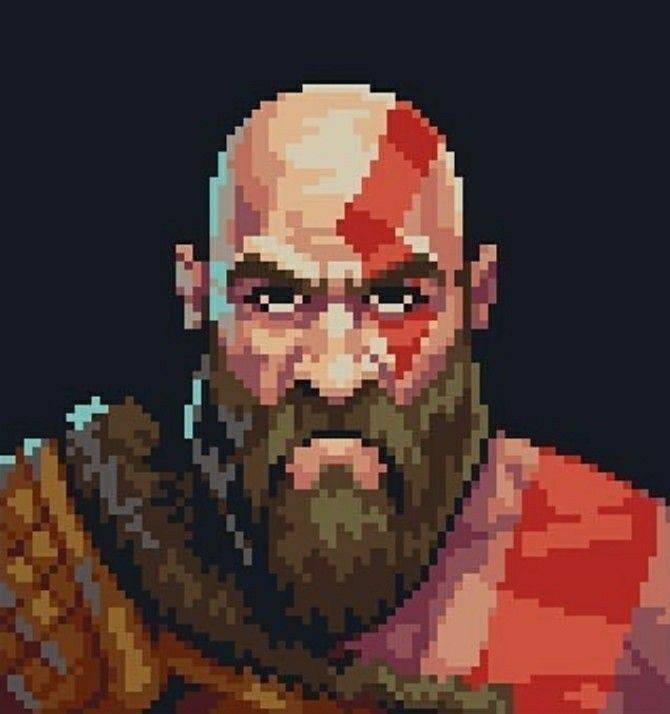
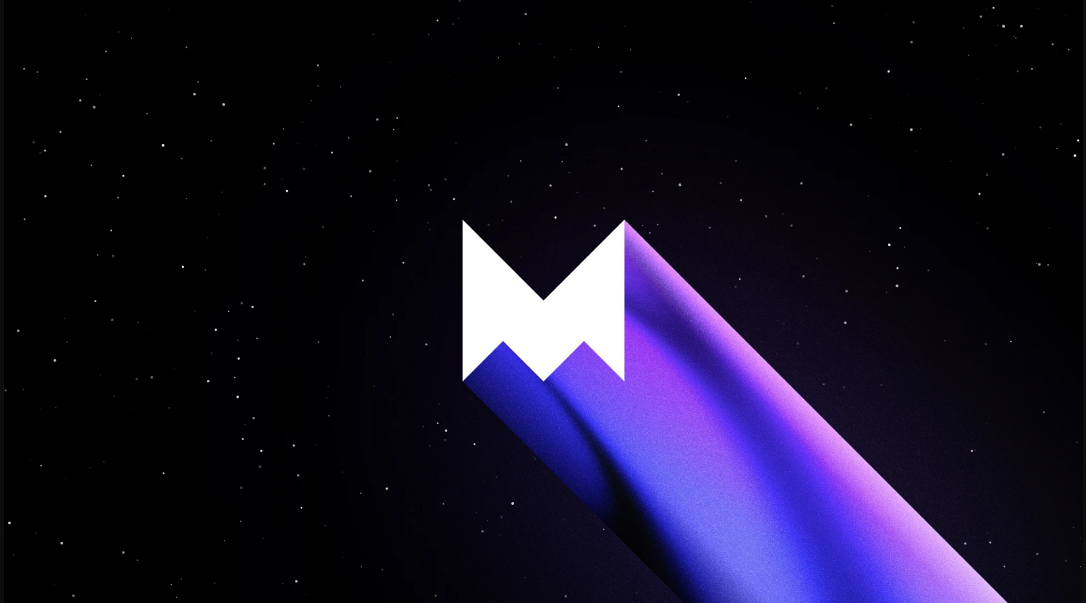
Recently, while updating my portfolio, I incorporated Framer Motion for animations, finding it incredibly intuitive and powerful. Inspired by this experience, I decided to share insights on how to leverage Framer Motion for React animations in this blog post. (Here's my portfolio if you wish to see it)
What is Framer Motion?
Framer Motion is a popular animation library for React that enables developers to create smooth, fluid animations with minimal effort. It provides a declarative API and intuitive syntax, making it easy to add animations to your React components.
Getting Started
Before we dive into creating animations, let's set up a React project with Framer Motion. You can start by creating a new React app using Create React App or any other preferred method. Once your project is set up, install Framer Motion using npm or yarn:
npm install framer-motion
# or
yarn add framer-motion
Creating Basic Animations
With Framer Motion installed, you can start animating your React components. Let's create a simple animation for a div element. First, import the necessary components from Framer Motion:
import { motion } from 'framer-motion';
Now, you can use the <motion.div>
component to wrap the element you want to animate. For example, to animate a div with a scale effect:
const MyComponent = () => {
return (
<motion.div
initial={{ scale: 0 }}
animate={{ scale: 1 }}
transition={{ duration: 0.5 }}
>
Hello, Framer Motion!
</motion.div>
);
};
In this example, the div will start with a scale of 0 and animate to a scale of 1 over a duration of 0.5 seconds when the component mounts.
Adding Interactivity
Framer Motion allows you to easily add interactivity to your animations. You can respond to user interactions such as clicks, hovers, and drags. Let's create a draggable element using Framer Motion:
const DraggableBox = () => {
return (
<motion.div
drag
dragConstraints={{ left: 0, right: 0, top: 0, bottom: 0 }}
>
Drag me around!
</motion.div>
);
};
In this example, the <motion.div>
is draggable within the specified constraints.
Complex Animations
Framer Motion also supports complex animations with multiple properties and keyframes. You can create staggered animations, animate between different layouts, and much more. Here's an example of a staggered animation:
const StaggeredAnimation = () => {
return (
<motion.div
initial={{ opacity: 0, x: -100 }}
animate={{ opacity: 1, x: 0 }}
transition={{ duration: 0.5, delay: 0.2 }}
>
Animated Text
</motion.div>
);
};
In this example, the text will fade in and move from left to right with a slight delay.
Conclusion
Framer Motion simplifies the process of creating React animations, allowing you to add fluid and engaging motion to your web applications with ease. Whether you're building simple transitions or complex interactions, Framer Motion provides the tools you need to bring your designs to life.
Subscribe to my newsletter
Read articles from YashMandi directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
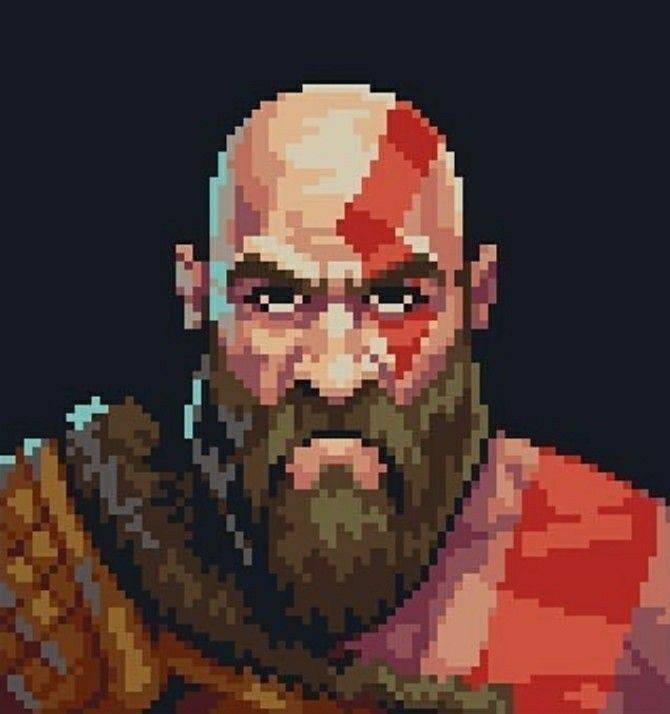
YashMandi
YashMandi
ms grad. software engineer intern. java. springboot. currently working on mern stack. freelancer