Speed Up Your Code with Memoization
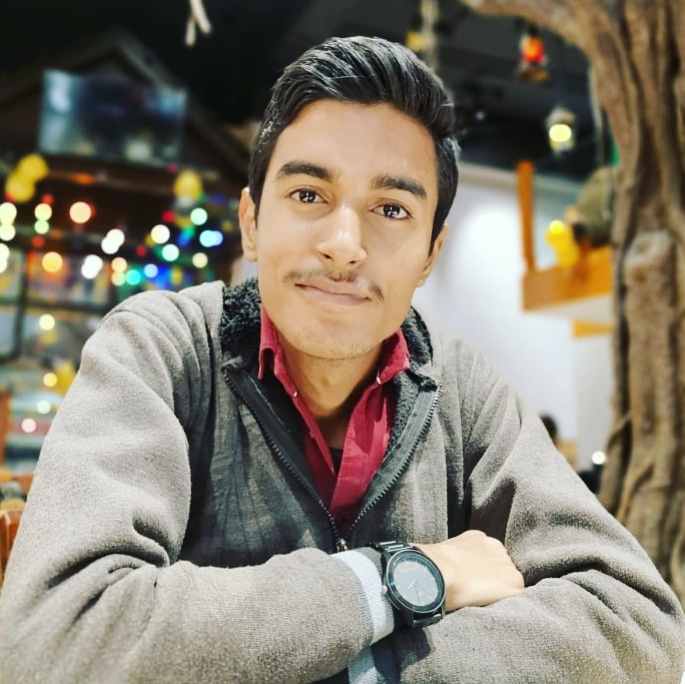
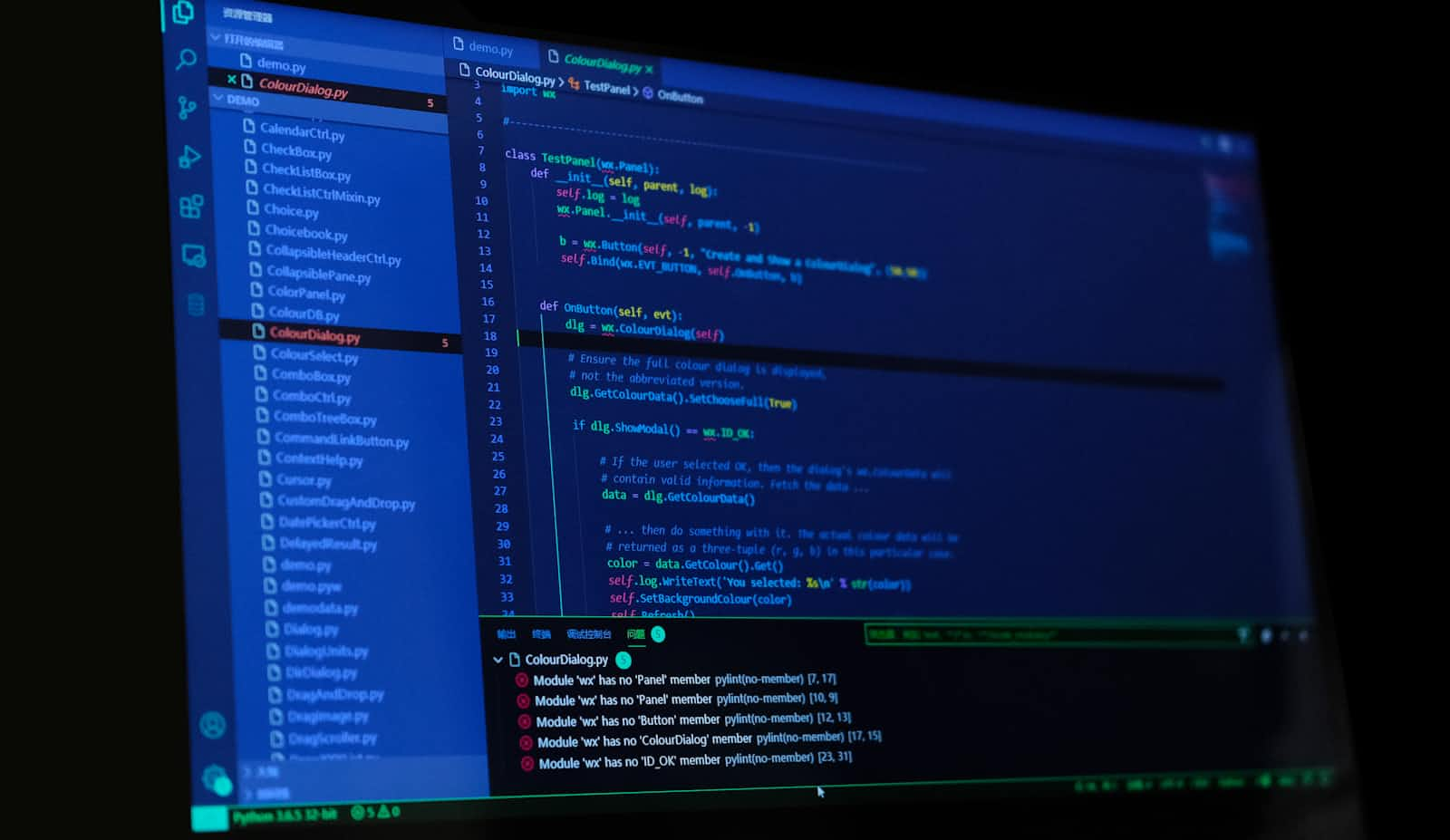
As programmers, we strive for efficiency. We write clean, well-structured code, but there's always room for optimization. One powerful technique to consider is memoization.
Memoization is an optimization technique that involves storing the results of expensive function calls and reusing them for subsequent calls with the same arguments. This can significantly improve the performance of your code, especially for functions that perform complex calculations or repetitive tasks.
Here's how memoization works
Wrap your function: Create a wrapper function around the original function you want to optimize.
Store results in a cache: Inside the wrapper function, use a dictionary or other data structure to store the previously computed results based on the function's arguments.
Check the cache first: Before performing the original function's logic, check the cache for the arguments. If a match is found, simply return the cached result.
Calculate and store: If there's no match in the cache, execute the original function and store the result for future calls with the same arguments.
Benefits of Memoization
Improved Performance: By eliminating redundant calculations, memoization can dramatically speed up your code, especially for functions involving complex computations or recursive calls.
Reduced Redundancy: Memoization prevents unnecessary calculations, making your code more efficient and resource-friendly.
Simplified Debugging: A well-designed cache can aid in debugging by providing a record of function calls and their results.
Example: Fibonacci Sequence
Let's consider a classic example - the Fibonacci sequence. This mathematical sequence defines each number as the sum of the two preceding ones. Calculating large Fibonacci numbers can be computationally expensive. Here is how we write it normally using python:
def fibonacci_recursive(n):
"""
This is a recursive function to calculate the nth Fibonacci number.
"""
if n <= 1:
return n
else:
return fibonacci_recursive(n-1) + fibonacci_recursive(n-2)
# This function can become very slow for larger values of n
Here's a memoized version of the Fibonacci function:
def fibonacci_memo(n, memo={}):
"""
This function implements memoization to optimize the Fibonacci sequence calculation.
"""
if n in memo:
return memo[n]
if n <= 1:
return n
else:
result = fibonacci_memo(n-1, memo) + fibonacci_memo(n-2, memo)
memo[n] = result
return result
# This version is significantly faster for larger n
Subscribe to my newsletter
Read articles from Abhishek Sharma directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
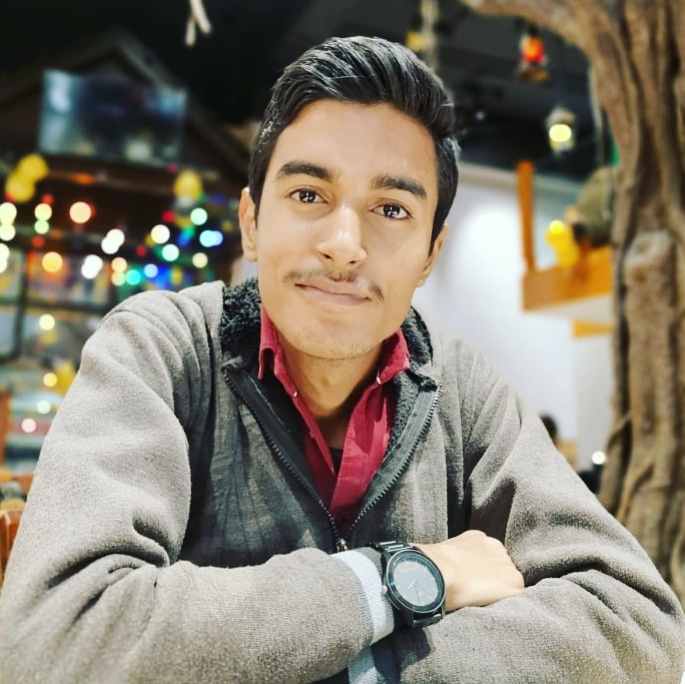
Abhishek Sharma
Abhishek Sharma
Abhishek is a designer, developer, and gaming enthusiast! He love creating things, whether it's building websites, designing interfaces, or conquering virtual worlds. With a passion for technology and its impact on the future, He is curious about how AI can be used to make work better and play even more fun.