Day 63 - Terraform Variables

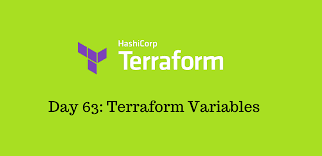
In Terraform, variables are used to pass values from external sources into Terraform configuration. They provide a way to configure the infrastructure without hardcoding values directly into the configuration. Variables in Terraform are quite important, as you need to hold values of names of instance, configs etc.
We can create a variable.tf file which will hold all the variables.
variable "filename" {
default = "/home/ubuntu/terraform/terraform-variable/var-demo.txt"
}
variable "content" {
default = "This is terraform variable demo file"
}
Task-01
Create a local file using Terraform.
Create a variable.tf file
The filename variable has a default value of /home/ubuntu/terraform/terraform-variable/var-demo.txt, which is the path and filename where the local file will be created.
The content variable has a default value of "This is terraform variable demo file", which is the text that will be written to the local file.
Create a main.tf file
Terraform code block creates a local_file resource named "devops" that writes a file to disk with the filename and content specified by the filename and content variables.
Initializes a new or existing terraform working directory
Generates an execution plan that shows what actions terraform will take to reach the desired state specified in the configuration file
When you run terraform apply, Terraform will create a file called var-demo.txt in the local directory with the content specified in the content variable.
Check file is created in specified folder using ls command.
Data Types in Terraform
Map
variable "file_contents" {
type = map
default = {
"statement1" = "this is cool"
"statement2" = "this is cooler"
}
}
Task-02
Use terraform to demonstrate usage of List, Set and Object datatypes
Put proper screenshots of the outputs
Map:
A map is a collection of values where each value is identified by a string label
variable "content_map" {
type = map
default = {
"content1" = "this is cool content1"
"content2" = "this is cooler content2"
}
}
In the example, a map variable named content_map is defined with a default value of two key-value pairs. The keys are strings and the values are also strings.
This variable can be used in your Terraform configuration to reference the values associated with each key defined in the map. For example, you might use it to configure a resource that requires content,
resource "local_file" "devops" {
filename = "/home/ubuntu/terraform/terraform-variable/type-map/demo.txt"
content = var.content_map["content1"]
}
In this example, we use the content_map variable to set the content parameter of local_file resource. We reference the value associated with the key "content1" using the dot notation and var.content_map["content1"] syntax.
terraform plan
terraform apply
Check demo.txt file content
List:
A list is an ordered collection of values of the same type. To define a list variable, use the list type and specify the type of elements in the list. Here's an example:
variable "file_list"{
type = list
default = ["/home/ubuntu/terraform/terraform-variable/type-map/file1.txt","/home/ubuntu/terraform/terraform-variable/type-map/file2.txt"]
}
In this example, we define a variable named "file_list" as a list of strings with a default value which contain files path.
This variable can be used in your Terraform configuration to reference the file paths defined in the list.
resource "local_file" "devops" {
filename = var.file_list[0]
content = var.content_map["content1"]
}
In this example, we use the file_list variable to set the filename parameter of an local_file resource. We reference the first element of the list using the square bracket notation and var.file_list[0] syntax.
Terraform plan
Terraform apply
Check 2 files created with different content
Object:
An object is a structural type that can contain different types of values, unlike map, list. It is a collection of named attributes that each have their own type.
In the example, an object variable named aws_ec2_object is defined with a default value of a set of properties for an EC2 instance.
This variable can be used in your Terraform configuration to reference the individual properties of the EC2 instance defined in the object.
variable "aws_ec2_object" {
type = object({
name = string
instances = number
keys = list(string)
ami = string
})
default = {
name = "test-ec2-instance"
instances = 4
keys = ["key1.pem","key2.pem"]
ami = "ubuntu-bfde24"
}
}
This output can be used to display the value of the ami property in the Terraform output after applying the configuration.
output "aws-ec2-instances"{
value = var.aws_ec2_object.ami
}
terraform plan
terraform apply
you can see output of aws-ec2-instance value.
Set:
A set is an unordered collection of unique values of the same type. To define a set variable, use the set type and specify the type of elements in the set. Here's an example:
variable "security_groups" {
type = set
default = ["sg-12345678", "sg-87654321"]
}
In this example, we define a variable named "security_groups" as a set of strings with a default value.
Use terraform refresh
To refresh the state by your configuration file, reloads the variables
terraform refresh is a Terraform command that retrieves the current state of the resources defined in your configuration and updates the Terraform state file to match that state.
By running terraform refresh, you can update the state file to reflect the current state of your resources, so that Terraform has an accurate view of what needs to be changed. This command does not make any changes to your resources, but simply updates the state file to match the current state of your resources.
Thank you for reading!
Subscribe to my newsletter
Read articles from Nikhil Yadav directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by

Nikhil Yadav
Nikhil Yadav
I am a highly motivated and enthusiastic individual completed B.Tech from Savitribai Phule University, Pune . With a strong interest in DevOps and Cloud technologies, I am eager to kick-start my career in this domain. Although I do not have much professional experience, I possess a willingness to learn, excellent problem-solving skills, and a passion for technology. I am committed to contributing my best to any team I work with.