Deploying a Node.js App to AWS Lambda: A Step-by-Step Guide
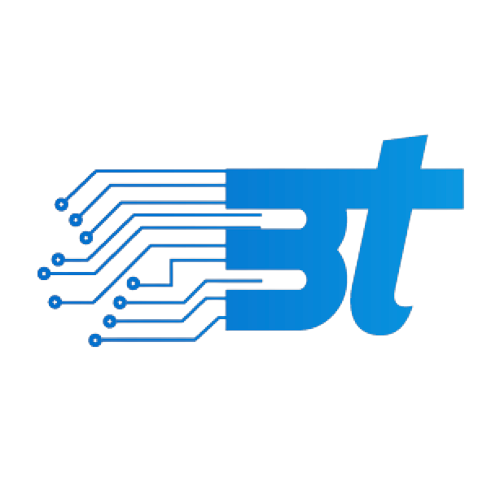
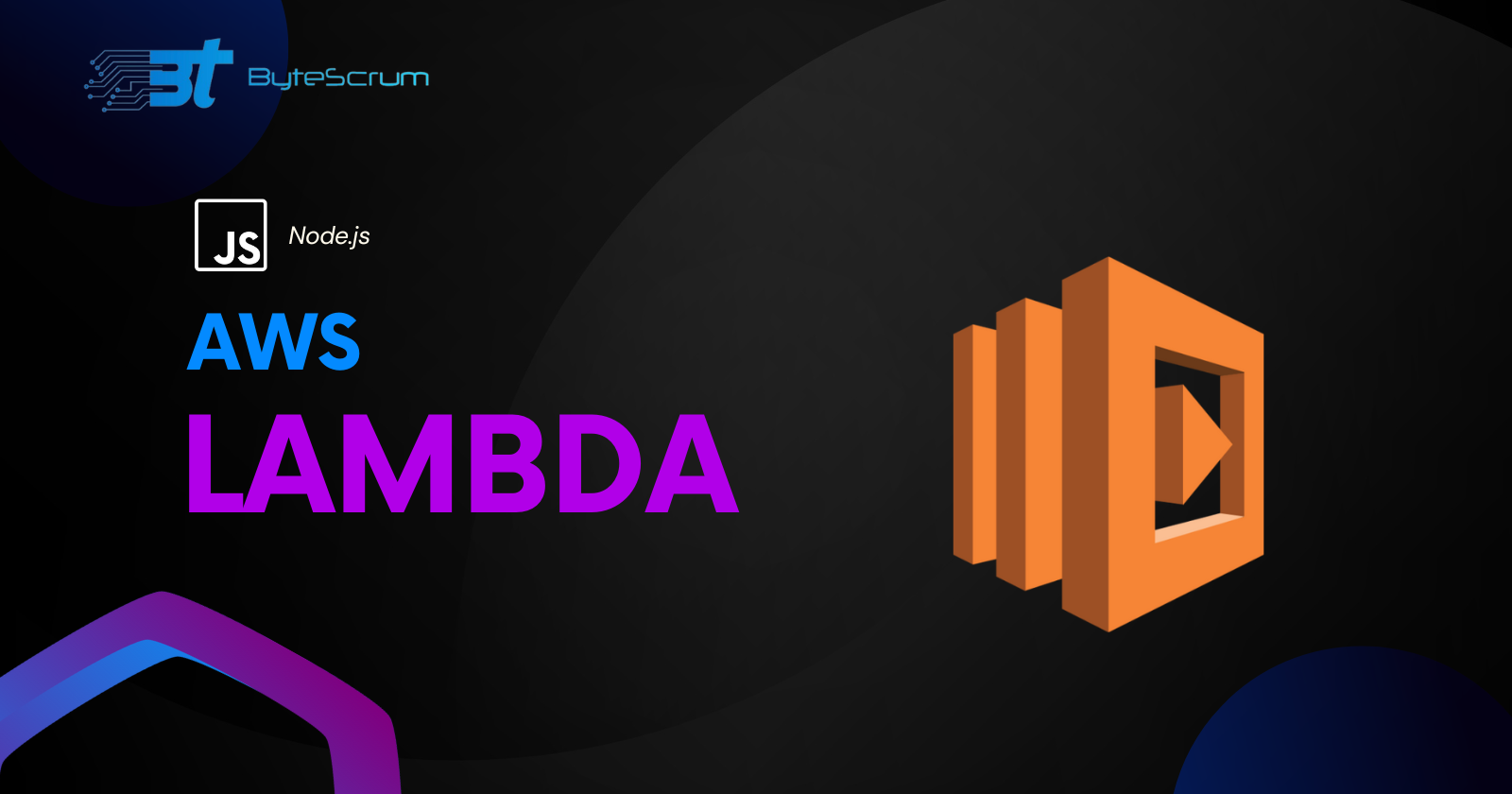
Introduction
AWS Lambda provides a serverless computing platform that allows you to run code without provisioning or managing servers. Deploying a Node.js application to AWS Lambda can bring scalability and cost efficiency to your project. In this guide, we will walk through deploying a Node.js app to AWS Lambda, from setting up your AWS environment to deploying and testing your application.
1. Setting Up Your AWS Environment
Create an AWS Account: If you don't already have an AWS account, sign up for one at AWS Management Console.
Install AWS CLI: Install the AWS Command Line Interface (CLI) on your local machine by following the instructions for your operating system here.
Configure AWS CLI: Run
AWS configure
in your terminal and enter your AWS Access Key ID, Secret Access Key, default region, and default output format.
2. Creating a Node.js Application
Initialize a Node.js Project: Create a new directory for your project and run
npm init
to initialise a new Node.js project. Follow the prompts to set up your project.Install Dependencies: Install any dependencies your application requires using
npm install
.
Write Your Application Code: Write your Node.js application code. For example, a simple Express.js server:
const express = require('express');
const app = express();
app.get('/', (req, res) => {
res.send('Hello, AWS Lambda!');
});
app.listen(3000, () => {
console.log('Server running on port 3000');
});
3. Packaging Your Application
Create a Deployment Package: Create a ZIP file containing your application code and any dependencies. Exclude development dependencies and files not required for execution.
Example Package Command:
zip -r my-node-app.zip index.js node_modules
4. Deploying Your Application to AWS Lambda
Create an IAM Role: In the AWS Management Console, navigate to IAM and create a new IAM role for your Lambda function. Attach the
AWSLambdaBasicExecutionRole
policy to this role.Deploy Your Function: Use the AWS CLI to deploy your function to AWS Lambda. Replace
<function-name>
with a unique name for your function.
aws lambda create-function --function-name <function-name> --zip-file fileb://my-node-app.zip --handler index.handler --runtime nodejs14.x --role arn:aws:iam::<your-account-id>:role/<your-role-name>
- Test Your Function: Use the AWS Lambda console to test your function. You can also invoke your function using the AWS CLI.
5. Updating Your Function
Update Your Application Code: Make changes to your application code as needed.
Update Your Deployment Package: Create a new ZIP file containing your updated code.
Update Your Function: Use the AWS CLI to update your function with the new deployment package.
aws lambda update-function-code --function-name <function-name> --zip-file fileb://my-node-app.zip
Conclusion
References
Share Your Thoughts
Have you deployed a Node.js application to AWS Lambda? Share your experiences and tips in the comments below!
Subscribe to my newsletter
Read articles from ByteScrum Technologies directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
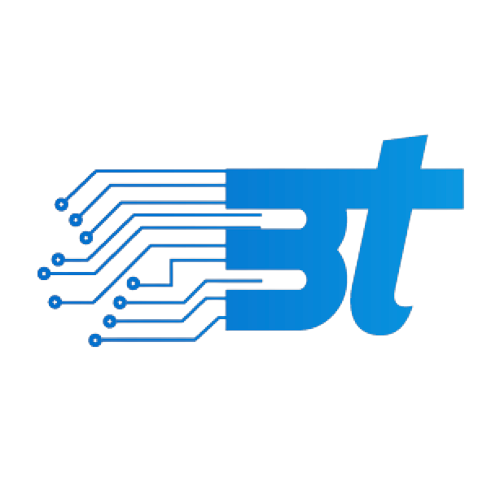
ByteScrum Technologies
ByteScrum Technologies
Our company comprises seasoned professionals, each an expert in their field. Customer satisfaction is our top priority, exceeding clients' needs. We ensure competitive pricing and quality in web and mobile development without compromise.