Master JavaScript: A Comprehensive Guide from Beginner to Advanced Levels
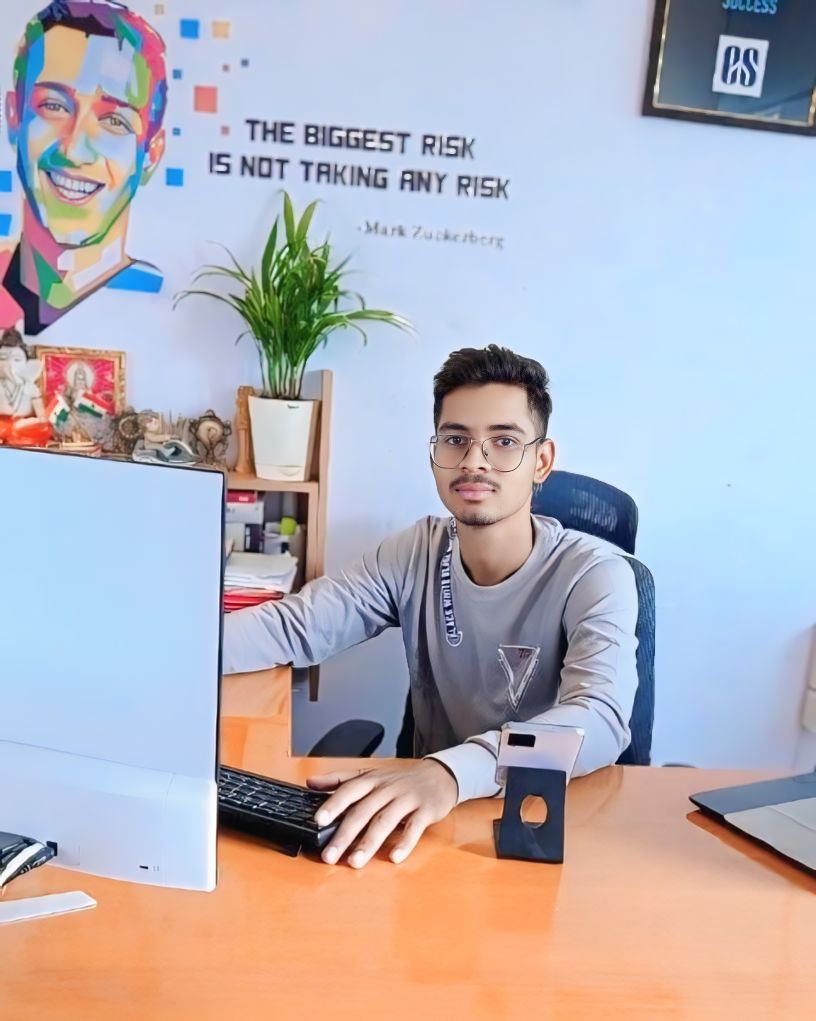
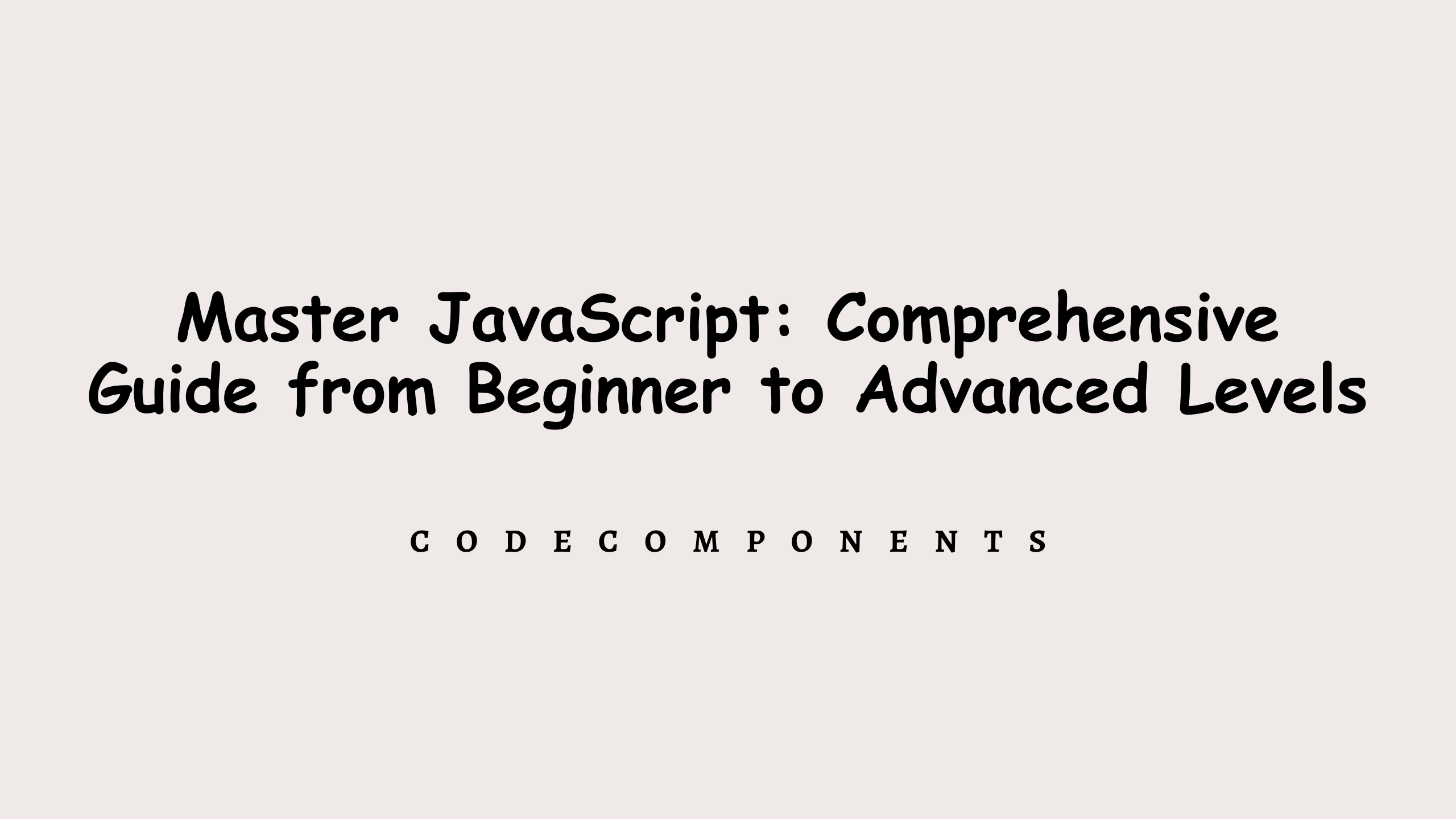
Topics Covered:
Basic points
Data Types
Conversion
Operations
Data Type Details
Memory Management
String in JavaScript
Numbe in JavaScript
Create array
Some Method of Array
slice and splice
Flat
Aske is an array Or convert in to array
OBJECTS
Create array
Literal
Singleton or Consteracter
Object Destructuring
Basic of Functions
Parameters And Arguments
Scope
OBJECTS
Literal
Use Symbol key inside Object
Object freeze
Singleton or Consteracter
Execution Context And Call Stack
Condition
Switch Case
Truethy and Falsethy
Cheak empty or not
Nullish Coalescing Operator
Terniary operator
Break and Continue Keyword
For loop
While loop
Do while loop
for of loop
For in loop
Map loop
ForEach loop
Filter
Map
Chaning
Reduce loop
Diagram of HTML Work in Windows
Windows Basic
Color Changer Project
Your Body Mass Project
Digital Clock Project
Guess The Number Project
Event Basic
Async Work Diagram
Async Code
Bg Color Change Advance Project
Keword Tracker Project
XMLHttpRequest
console
promice
Fetch
Intro about OOP
Object literal
Constructor function
Property and method
ABOUT
this
KEYWORDPrototype
ABOUT
new
KEYWORDMake Own Method
Prototype INHERITANCE
Call Stack Behavior function in side function
Call Stack
Class
Inheritance
StsticProp
Bind
MathPI
GaterSater
Closers
Basic Points
Curly brace operator:
{}
Square brackets:
[]
JavaScript is a prototype language.
Difference Between Null, Undefined & Symbol: Three distinct data types
Null(Object) value : let val = ""; absence of value.
Undefined(Undefined) value : let val; not initialized any value.
Symbol : unique; Each (symbol) value is guaranteed to be unique.
Conversions
Use to convert one data type to another data type.
Convert to Number: Number()
Convert to Boolean: Boolean()
Convert to String: String()
Notes (Conversion Number):
"33" => 33
"33abc" => NaN
null => 0
Undefined => NaN
true => 1
false => 0
"Mritunjay" => NaN
Operations
(+,-,<,>,*,/,%,==,!=,===,>=,<=)
"str"+2=>str2
1+"str"=>1str
1+"2"=>12 (type of string)
2+2+"2"=>42 (type of string)
"2"+2=>22 (type of string)
"2"+2+2=>222 (type of string)
2+4*5/9 => Wrong Way Use this way ((2+4)*5)/9
if true = 1
+true =>1
-true =>-1
+false =>0
-false =>-0
+"" => 0
+"mk" => NaN
num1 = num2 = num3 = 2+9
game = 100
game++, game-- postfix operator
++game, --game prefix operator
Data Type Details
Two types of data type javascript.
Primitive data type
number, string, boolean, bigint, null, undefined, symbol
Non-Primitive data type
Object{}, Array(), Functions()
Primitive DataType (Call by value)
In primitive data types, whenever you declare a variable, you get a copy of its value, not a reference to that value. Any changes you make to the variable happen in that copy.
Example:-
let myYoutubeName = 'MritunjayKumar' let anotherChannel = myYoutubeName anotherChannel = "YoutubeChannel" console.log([myYoutubeName, anotherChannel]) return:-[ 'MritunjayKumar', 'YoutubeChannel' ]
const id = Symbol("123") const anotherid = Symbol("123") console.log([id, anotherid, typeof id, typeof anotherid, id === anotherid]); return :- [ Symbol(123), Symbol(123), 'symbol','symbol', false ] //id and anotherid not same because symbol() datatype is convert the space and unique .symbol() is converted any value into a unique value. const bitNumber = 21654654516581454516484515454n console.log(typeof bitNumber); return:-BigInt
Non-Primitive/Reference (call by reference)
In non-primitive data types, whenever you declare a variable, you get its reference. This means you don't get a copy of the value itself; instead, you get a direct link to the original value. Any changes you make to the variable directly affect the original value.
Example:-
const heroArr = ['Github', "gitbas", "visualstudio"] const heroObj = { name: 'Github', age: 22, isLoggedIn: true} const heroFun = function () {console.log("My Fun")} console.log([typeof heroArr, typeof heroObj, typeof heroFun]) return:- ['object', 'object', 'function']
Memory Management
Two types of memory:-
Stack memory.
Heap memory.
Primitive Datatypes use Stack memory.
Primitive data types directly contain values. They are
small in size and can be stored on the stack memory
. Stack memory is a portion of the RAM where the CPU stores primitive data types, method calls, and local variables.The difference between the primitive data type and the stack memory is
SUBTITLE
. Primitive data types are stored directly on the stack memory. When you create a variable and assign a value to it, the value is stored in the memory allocated for that variable.For example, in JS, if you declare a number variable, const num = 10; The value 10 is stored in the stack memory as a 32-bit integer.
Example Memory work
:
let myYoutubeName2 = 'MritunjayKumar'
let anotherChannel2 = myYoutubeName2
anotherChannel2 = "YoutubeChannel"
console.log([myYoutubeName2, anotherChannel2])
return:-[ 'MritunjayKumar', 'YoutubeChannel' ]
Non-Primitive Datatypes use Heap memory.
Non-primitive data types are stored in heap memory.
Heap memory is a
large and flexible area of memory used for dynamic memory allocation
. When a new object is created in JS, it is allocated memory in the heap memory.For example, when you create an integer array of length 10, you are creating an object that consists of an integer array reference, an integer count, and 10 integer values. Each integer is 32 bits in size, so the entire array object is approximately 400 bits (32 bits x 10 integers) in size.
Example (Memory Work):
let userOne = {
email: 'user@google.com',
upi: 'user@yblu',
}
console.log([userOne, userTwo])
return :-
[{ email: 'mritunjay@gmail.com', upi: 'user@yblu' },
{ email: 'mritunjay@gmail.com', upi: 'user@yblu' }]
Strings in JavaScript
Declare string 2 way
First way.
const gameName = "Mritunjay Kumar";
Second way.
const gameName = new String("Mritunjay Kumar"); //'new' use to create object. Also declear new string. console.log(gameName) console.log(gameName[0]) return :- [String: 'Mritunjay Kumar'] M
If you try to run this line
new String('Mritunjay Kumar')
in the browser console, you will get all the methods and properties of the string.Some property for string:
const gameName = "Mritunjay Kumar"; gameName.length; //find length of string. gameName.toUpperCase(); //convert to upper case gameName.charAt(2); //find second index character gameName.indexOf("t"); //find character index const newString = " Mritunjay "; newString = Mritunjay newString.trim() = Mritunjay const url = "https://mritunjay://com%20domain%20kumar"; console.log(url.replace("%20", "_")) // return:- https://mritunjay://com_domain%20kumar url.includes("sundar") = false url.includes("mritunjay") = true url.split("/") = [ 'https:', '', 'mritunjay:', '', 'com%20domain%20kumar' ]
Numbe in JavaScript
Declear number 2 way
First way
const score = 400;
Second Way
const balance = new Number(100);
If you try to run this line new Number(100)
in the browser console, you will get all the methods and properties of numbers.
Some Methods of Numbers
balance = 1;
balance.toString().length; //return :- 3
balance.toFixed(2); //return :- 100.00
MATHS Library
If you try to run this line console.log(Math)
in the browser console, you will see all the functions of Math.
Math.abs(-4) //return:- 4
//abs() function change - to + not + to -
Math.round(4.3) = 4
// round() function is used for remove the decimal part from a number
Math.ceil(4.3) = 5
//top value select 4 sa thoda bhe jayada hoga to top vlalue choose
Math.floor(4.9) = 4
//lower value select 5 sa thoda bhe kam hoga to lower vlalue choose
Math.min(4, 8, 1, 0, 9) = 0
//lower value select
Math.random()
//bitween 0 to 1 (ex:-0.85984596585296, 0.85854585452842,0.7896541241 and etc 0.sumthing)
Math.random() * 10 = 5.98989898
//any random number under 10 degit
Example of Math:
const min = 10;
const max = 20;
Math.floor(Math.random() * (max - min + 1)) + min
// random number bitween 10 to 20.
// (max - min + 1): Calculates the range of values. In your //example, with min as 10 and max as 20, the range is 11.
// Math.random() * (max - min + 1): Scales the random number// to fit within the range (0, 11).
// (max - min +1) => 11;
// (Math.random() * (max - min +1)) => 0 to 11
// (Math.random() * (max - min +1))+min => 10 to 21
Array
Create array
In Method parentheses are used like arr.pop()
. Here, pop
is the method, and ()
represents parentheses.
let array1 = ["mritunjay", true, 452522];
let array2 = [1, "mk", false];
arr = [...array1, ...array2]; //Spreade operater Use to murj two or more array
Return: -["mritunjay", true, 452522, 1, "mk", false];
arr[3]; //Get Particuler value by index id from array
Return: -1;
arr.push("Rahul"); //Add element on last in array
Return: -["mritunjay", true, 452522, 1, "mk", false, "Rahul"];
arr.pop(); //Remove last element from last and return removal element
Return: -Rahul;
arr;
Return: -["mritunjay", true, 452522, 1, "mk", false];
arr.unshift(9); //Add element in start
console.log(arr);
Return: -[9, "mritunjay", true, 452522, 1, "mk", false];
arr.shift(9); //Remove start element from array
console.log(arr);
Return: -["mritunjay", true, 452522, 1, "mk", false];
arr.includes(9); //If element present then true another wise false
Return: -false;
arr.indexOf("mritunjay"); //Get element index (if element not present then return -1)
Return: -1;
arr.join(); //Convert to string ( And typeof also return string)
Return: -mritunjay, true, 452522, 1, "mk", false;
Slice and splice
arr = [4, 5, 6, "mritunjay", true];
Slice (extract a section of an array)
Syntax : arr.slice(startIndex, endIndex)
console.log("A", arr);
Return: -A[(4, 5, 6, "mritunjay", true)];
myn1 = arr.slice(1, 3);
console.log(myn1);
Return: -[5, 6];
console.log("B", arr);
Return: -B[(4, 5, 6, "mritunjay", true)];
Splice (remove elements from an original array)
Syntax : arr.splice(startIndex, deleteCount)
console.log("C", arr);
Return: -C[(4, 5, 6, "mritunjay", true)];
myn2 = arr.splice(1, 3);
console.log(myn2);
Return: -[5, 6, "mritunjay"];
console.log("D", arr);
Return: -D[(4, true)];
Some methode IMP
const arr1 = ["Mritunjay", 9835678727, true];
const arr2 = ["Kumar", 0, false];
arr1.push(arr2); //Insert arr2 inside arr1 original Existing array.
Return: -["Mritunjay", 9835678727, true, ["Kumar", 0, false]];
arr1[3][0]; //Get elements
Return: -Kumar;
arr1.concat(arr2); // add two array and return new array(Not disturb Existing array)
Return: -["Mritunjay", 9835678727, true, "Kumar", 0, false];
flat (use to deStructure the array)
const another_array = [1, 2, 3, [4, 5, 6], 7, [8, 9, [10, 11]]];
arr.flat();
Return: -[1, 2, 3, 4, 5, 6, 7, [8, 9, [10, 11]]];
another_array.flat(Infinity);
Return: -[1, 2, 3, 4, 5, 6, 7, 8, 9, 10, 11];
another_array.flat(1);
Return: -[1, 2, 3, 4, 5, 6, 7, 8, 9, [10, 11]];
another_array.flat(2);
Return: -[1, 2, 3, 4, 5, 6, 7, 8, 9, 10, 11];
Aske is an array or convert in to array
IMP
Array.isArray("Mritunjay Kumar"); //Cheak is array or not
Return: -false;
Array.from("Mritunjay Kumar"); //Convert to array
Return: -[
"M",
"r",
"i",
"t",
"u",
"n",
"j",
"a",
"y",
" ",
"K",
"u",
"m",
"a",
"r",
];
console.log(Array.from({ name: "mritunjay" }));
// convert in to array empty array return :- {}
console.log(Array.from({ name: "mritunjay" }));
// convert in to array anothe way if convert object ot array then use key or value
let score1 = 100;
let score2 = 200;
let score3 = 300;
let score4 = 400;
Array.of(score1, score2, score3, score4);
Return: -[100, 200, 300, 400];
OBJECTS
new Object();
All methods are used in the console.Objects can be declared in two ways: through
Literals
orConstructors
.The difference between
Literals
&Constructors
is Singleton. Non-singleton{}
, singletonnew Object()
. Singleton is created only by Constructors.
Literal Wise
Using literal notation is a straightforward way to create an object
let car = { name: "GT", maker: "BMW", engine: "1998cc", };
Constructor Wise
Constructor
Constructors are functions
are used with thenew
keyword to create instances (occurrence of something) of objects.function Vehicle(name, maker, engine) { this.name = name; this.maker = maker; this.engine = engine; }; let car = new Vehicle("GT", "BMW", "1998cc");
Singleton Object
const TinderObject = new Object();
This is Singleton Object.
Using Object.create()
Object.create({ isStudying: false, printIntroduction: function () { console.log(`My name is ${this.name}. Am I studying?: ${this.isStudying}`); }, });
Using es6 classes
class Vehicle { constructor(name, maker, engine) { this.name = name; this.maker = maker; this.engine = engine; } } let car1 = new Vehicle("GT", "BMW", "1998cc");
Literal
In programming, an object literal is a notation for creating an object using a set of key-value pairs.
const JsUser = {};
Create an empty Object And Non-Singleton Object
const JsUsers = { //Create Object with Key and value
name: "Mritunjay",
age: 18,
"full name": "Mritunjay Kumar", //Alwase Object treat all Key as a String.
location: "Jaipur",
email: "mritunjay@gmail.com",
isLoggedIn: false,
lastLoginDays: ["Monday", "Saturday"],
fullName: function() {
return this.firstName + ' ' + this.lastName;
}
}
//JsUsers is an object literal with properties firstName, lastName, age, and method is fullName.
JsUsers.email; //Get data from Object
JsUsers."full name"; //or Use JsUsers.full name; //Both Give Error
JsUsers["full name"]; //Use Another way Becouse JsUsers.full name not posible, thats way we use JsUsers[""full name""]
Get value from Object
const user = {
name: "Mritunjay",
symbolKey: "User123", //not Work This Way symbolKey Kay is a type of string.
age: 18,
};
- `user["age"];` return :- 18 //Get value from object.
- `user.age` return :- 18
- `user["name"];` return :- Mritunjay
- `user.name` return :- Mritunjay
- `user["symbolKey"]` return :- User123
- `user.symbolKey` return :- User123
- `user[symbolKey];` return :- undefined //Not that wat to get value
- `user[age];` return :- undefined
- `user[name];` return :- undefined
use Symbol key inside Object Using [Key]
const symbolKey = Symbol("ID");
const user1 = {
name: "Mritunjay",
symbolKey: "Kumar",
[symbolKey]: "User123", //Work This Way symbolKey Kay is a type of Symbol.
age: 18,
};
user1[symbolKey];
Access Symbol returns: User123user1["symbolKey"];
returns: Kumar
Object freeze
No one can change the value of in object.
user1; //Return :- { name: 'Mritunjay', symbolKey: 'User123', age: 18, [Symbol(ID)]: 'User123'}
user1.email = "Kumar@gmail.com"; //Insert Date in Object
user1; // Return :-{ name: 'Mritunjay', symbolKey: 'User123',age: 18, email: 'Kumar@gmail.com', [Symbol(ID)]: 'User123'}
Object.freeze(user1); // Use to freeze the object (No One can change the value of in object.)
Object.isFrozen(user1); // Cheak Object if freez or not return :- true
user1.email = "Mritunjay@gmail.com";
user1; //Return :- { name: 'Mritunjay', symbolKey: 'User123', age: 18, email: 'Kumar@gmail.com', [Symbol(ID)]: 'User123'}
const UnFreezonObject = Object.assign({}, user1); // UsFreez the Object.
UnFreezonObject.greeting = function () {
console.log("Hello Function...");
};
UnFreezonObject.greetingTwo = function () {
console.log(`Hello Function... ${this.name}`); // this is use to get same object data (it's refrence of same object).
};
console.log(UnFreezonObject.greeting); // return :- [Function (anonymous)]
console.log(UnFreezonObject.greeting()); //return :- Hello Function...
console.log(UnFreezonObject.greetingTwo()); //return :- Hello Function... Mritunjay
Singleton or Consteracter
Singleton or none Singleton
const Object1 = new Object(); // This is Singleton Object const Object2 = {}; // This is Non Singleton Object
Some Property
const TinderObject = {}; TinderObject.id = "123abc"; TinderObject.name = "Mritunjay"; TinderObject.isLoggedIn = false; Object.keys(TinderObject); //return:- [ 'id', 'name', 'isLoggedIn' ] Object.values(TinderObject); //return:- [ '123abc', 'Mritunjay', false ] Object.entries(TinderObject); //return:- [[ 'id', '123abc' ],[ 'name', 'Mritunjay' ],[ 'isLoggedIn', false ]] TinderObject.hasOwnProperty("name"); //hasOwnProperty() this property cheak is key avelable or not. return:- true const regularUser = { email: "mk@mk.com", fullName: { userfullname: { firstName: "Mritunjay", lastName: "Kumar", }, }, }; regularUser.fullName.userfullname; // return :-{ firstName: 'Mritunjay', lastName: 'Kumar' } regularUser.fullName.userfullname.firstName; // return :-Mritunjay const Obj1 = { 1: "a", 2: "b" }; const Obj2 = { 3: "c", 4: "b", 5: "e" }; const obj3 = { Obj1, Obj2 }; obj3; // return :- {Obj1: { '1': 'a', '2': 'b' },Obj2: { '3': 'c', '4': 'b', '5': 'e' }} const obj4 = Object.assign(Obj1, Obj2); const obj5 = Object.assign({}, Obj1, Obj2); // Correct Way const obj6 = { ...Obj1, ...Obj2 }; // Correct Way obj4; // return :- { '1': 'a', '2': 'b', '3': 'c', '4': 'b', '5': 'e' } obj5; // return :- { '1': 'a', '2': 'b', '3': 'c', '4': 'b', '5': 'e' } obj6; // return :- { '1': 'a', '2': 'b', '3': 'c', '4': 'b', '5': 'e' }
Come Data From Data Base (Handle)
const DataBaseUser = [ { id: 1, email: "mk@gmail.com" }, { id: 2, email: "mk1@gmail.com" }, { id: 3, email: "mk2@gmail.com" }, { id: 4, email: "mk3@gmail.com" }, { id: 5, email: "mk4@gmail.com" }, ]; console.log(DataBaseUser[1].email);
Object Destructuring
let person = { name: "John Doe", age: 30, cityName: "New York", }; const { cityName: city, age, name } = person; // 2th Way (Correct Way) (cityName: city, Change the variable city = cityName)
Execution Context And Call Stack
Condition
<,>,<=,>=,==,=== (cheak type),!=, !(negative cheak), &&, ||
if (true) console.log("if");
Wron Way to use, Use simple.
Switch Case
const month = 3;
switch (month) {
case 1:
console.log("Jan");
break;
case 2:
console.log("Feb");
break;
case 3:
console.log("Mar");
break;
default:
console.log("Invalid Month");
break;
}
break :
By defoult in switch when key match with case then whole case code run . If you not use 'break;' then next whole case code run without matching case.
Truethy and Falsethy
falsethy Values :
flase, 0, -0(false), 0n (BigIn?t), null, undefined, NaNTruethy Values :
'false', "0", " ", [], {}, function(){},
Cheak empty or not
Cheak is not any value in side variable
const userEmail = ""; if (userEmail) { console.log("Got User Name"); } else { console.log("Do Not Got User Eamil"); // this return }
Cheak Array is empty or not
const Cheakarr = [];
if (Cheakarr.length > 0) {
console.log("Cheakarr is not empty");
} else if (Cheakarr.length === 0) {
console.log("Cheakarr is empty");
}
Cheak Object is empty or not
const CheakObj = {};
if (Object.keys(CheakObj).length > 0) {
console.log("CheakObj is not empty");
} else if (Object.keys(CheakObj).length === 0) {
console.log("CheakObj is empty");
}
Nullish Coalescing Operator (??)
It returns the first operand if it is not Null or Undefined; otherwise, it returns the second operand.
??:-Useful when we need to set a default value for a variable that could be null or undefined (for example, when data from a database is null or undefined, it returns the default value).
syntex:- Real value ?? defoult value (If real value is null or undefined then run default value.)
let userActive = false;
let defaultActive = true;
let isActive = userActive ?? defaultActive;
console.log(isActive); // Output: true
let user = null;
let defaultUser = "Anonymous";
let username = user ?? defaultUser;
console.log(username); // Output: Anonymous
let vall;
vall = 5 ?? 10;
console.log(vall); //return 5
console.log(null ?? 15); // Return: 15
console.log(undefined ?? 25); // Return: 25
console.log(50 ?? 25); // Return: 50
Terniary operator
(same as if elase)
syntex:- condition ? true : false
console.log(5 > 2 ? 5 : 2); // Return: 5 console.log(true ? 5 : 2); // Return: 5 console.log(false ? 5 : 2); // Return: 2
Break and Continue Keyword
continue;
- it will continue the inner loopbreak;
- it will stop the outer loop
For loop
Syntex:-
for (let index = 0; index < 10; index++) {
console.log(index);
}
Insert value > Cheak condition > Run Boloc of code {} > index++
for (let i = 0; i < 10; i++) { console.log(`Outer value ${i}:`); for (let j = 0; j < 10; j++) { console.log(`Inner value ${j}`); } }
for (let i = 0; i < 10; i++) { if (i == 5) { console.log("5 is detected"); break; // it will stop the loop } console.log(`${i} is best`); }
While loop
Syntex:-
let arr = ["mk", "sk", "pk"]; let j = 0; while (j < arr.length) { console.log(arr[j]); j = j + 1; }
Do while loop
1st time it runs whenever the condition is true or false, then it checks true or false.
let d = 0;
do {
console.log(`do while loop :- ${d}`);
d = d + 1;
} while (d <= 10);
For of loop
Syntex:-
let data = ["mk", "sk", "pk"];
for (let value of data) {
console.log(value);
} //return :- mk sk pk
Destructuring the data and returning them one by one inside the value.
You can use any type of data such as arrays, objects, arrays of objects, and so on.
let obj = [{ 1: "mk" }, { 2: "sk" }, { 3: "pk" }];
for (let value of obj) {
console.log(value);
} //return :-{ '1': 'mk' }{ '2': 'sk' }{ '3': 'pk' }
let val = "Mritunjay";
for (const i of val) {
console.log(i);
} //return :- M r i t u n j a y
For in loop
Iterate to get each index from the data one by one.
Syntex:-
for (let key in obj) { console.log(key); } //return :- 0 1 2 for (let key in arr) { console.log(key); } //return :- 0 1 2 for (const i in val) { console.log(i); } //return :- 0 1 2 3 4 5 6 7 8
Map loop
Not storing duplicate values means that if you set a duplicate value, it will only return the first occurrence of the duplicate key with its value.
Its take key and value.
Syntex Create map
const map1 = new Map();
.Set Key and value
map1.set("IN", "India");
.Get value by key
map.get("IN")
;get both key value
for (const key of map) {
console.log(key);
}
//Or DeStructring the map
for (const [key, value] of map) {
console.log(`${key} and ${value},`);
}
Remember that iterating using [key, value] is only applicable with maps.
Remember Some points.
const myObj = { name: "Mritunjay", age: 25, city: "Mumbai", }; //Not use like that with object for (const [key, value] of myObj) { console.log(`${key} and ${value}`); } // return :- error myObj not iterable // Use by this with object for (const key in myObj) { console.log(key); } // return :- name age city
Get both key and value
for (const key in myObj) { console.log(`${key} shortcut if for ${myObj[key]} ,`); } // return :- name shortcut if for Mritunjay , age shortcut if for 25 , city shortcut if for Mumbai ,
Use array
const inArr = ["mk", "pl", "ki", "ok"]; for (const key in inArr) { console.log(`${key} value :- ${inArr[key]}, `); } //return :-0 value :- mk 1, value :- pl, 2 value :- ki, 3 value :- ok,
Use for in in map
for (const key in map) { console.log(key); } // return :- empty
Because in a map, you can't access data using an index. You can only retrieve data using the associated key.
ForEach loop
Take 3 Argument.
Callbackfun means a function that will be used inside without any named function.
const coding = ["JS", "Ruby", "Python", "HTML", "C", "C++", "cpp"]; coding.forEach(function (val) { console.log(val); }); //Return:- JS Ruby Python HTML C C++ cpp // Or coding.forEach((item) => { console.log(item); }); //Return:- JS Ruby Python HTML C C++ cpp //or function PrintName(params) { console.log(params); } coding.forEach(PrintName); //Return:- JS Ruby Python HTML C C++ cpp
Argument take
coding.forEach((item, index, arr) => { console.log(item, index, arr); }); Return:- JS 0 [ 'JS', 'Ruby', 'Python', 'HTML', 'C', '++', 'cpp'] Ruby 1 [ 'JS', 'Ruby', 'Python', 'HTML', 'C', '++', 'cpp'] Python 2 [ 'JS', 'Ruby', 'Python', 'HTML', 'C','C++', 'cpp'] HTML 3 [ 'JS', 'Ruby', 'Python', 'HTML', 'C', '++', 'cpp'] C 4 [ 'JS', 'Ruby', 'Python', 'HTML', 'C', '++', 'cpp'] C++ 5 [ 'JS', 'Ruby', 'Python', 'HTML', 'C', '++', 'cpp'] cpp 6 [ 'JS', 'Ruby', 'Python', 'HTML', 'C', '++', 'cpp'] const myCoding = [ { languageName: "JavaScript", languageFileName: "JS", }, { languageName: "java", languageFileName: "java", }, { languageName: "python", languageFileName: "py", }, ]; myCoding.forEach((item) => { //item return the one by one object from array. console.log(item.languageName); //object ma sa lainga languageName. return:- JavaScript java python });
const coding = [1, 2, 3, 4, 5, 6, 7]; const value = coding.forEach((item) => { console.log(item); }); console.log(value); //Print :- 1 2 3 4 5 6 7 undefiner const value1 = coding.forEach((item) => { console.log(item); return item; }); console.log(value1); //return :- 1 2 3 4 5 6 7 undefiner
How can we store the data from a foreach loop in a variable for further use?
use filter()
Filter
const coding = [1, 2, 3, 4, 5, 6, 7];
const nuwNum = coding.filter((num) => num > 4);
console.log(nuwNum); //return:- [ 5, 6, 7 ]
const nuwNum1 = coding.filter((num) => {
num > 4;
});
console.log(nuwNum1); //return:- []
When you use Scope
{}
, it's mandatory to use thereturn
keyword.const nuwNum2 = coding.filter((num) => { return num > 4; }); console.log(nuwNum2); // return:- [ 5, 6, 7 ]
const myCoding = [ { languageName: "JavaScript", languageFileName: "JS", }, { languageName: "java", languageFileName: "java", }, { languageName: "python", languageFileName: "py", }, { languageName: "java", languageFileName: "java", }, ]; const filterdata = myCoding.filter((cod) => cod.languageName === "java"); console.log(filterdata); return:-[ { languageName: "java", languageFileName: "java" }, { languageName: "java", languageFileName: "java" },]; const filterdata1 = myCoding.filter((cod) => { cod.languageName === "java"; }); console.log(filterdata1); return:-[] const filterdata2 = myCoding.filter((cod) => { return cod.languageName === "java"; }); console.log(filterdata2); return:-[ { languageName: 'java', languageFileName: 'java' }, { languageName: 'java', languageFileName: 'java' }] const filterdata3 = myCoding.filter((cod) => { return cod.languageName === "python" && cod.languageFileName === "py"; }); console.log(filterdata3); return:-[ { languageName: 'python',languageFileName: 'py' } ]
Map
const myCoding = [1, 2, 3, 4, 5, 6, 7];
const newNums = myCoding.map((num) => num + 10);
console.log(newNums);
return:- [ 11, 12, 13, 14, 15, 16, 17]
Chaning
const newNums1 = myCoding
.map((num) => num + 10)
.map((num) => num - 1)
.filter((num) => num >= 15);
console.log(newNums1);
return:- [ 15, 16 ]
Reduce loop
Use in add totla card amount
Higher-order function
Applying a callback function
Result only single value
Takes two main parameters
accumulates
¤t value
.
const myCoding1 = [1, 2, 3];
const myTotal = myCoding1.reduce((acc, curentVal) => {
//accumilater take second parparameters of reduce.By defoult it's take 0.
console.log(`accumilater Value: ${acc} & current Value: ${curentVal}`);
return acc + curentVal;
}, 0); //pass value in acc (accumilater).
console.log(myTotal);
return:-
accumilater Value: 0 & current Value: 1
accumilater Value: 1 & current Value: 2
accumilater Value: 3 & current Value: 3
6
//OR you add second parameters is 100 the return
const myCoding = [1, 2, 3];
const myTotal = myCoding1.reduce((acc, curentVal) => {
//accumilater take second parparameters of reduce.By defoult it's take 0.
console.log(`accumilater Value: ${acc} & current Value: ${curentVal}`);
return acc + curentVal;
}, 100); //pass value in acc (accumilater).
console.log(myTotal);
return:-
accumilater Value: 100 & current Value: 1
accumilater Value: 101 & current Value: 2
accumilater Value: 103 & current Value: 3
106
One more example
const shoppingBag = [ { itemName: "Js Course", price: 499, }, { itemName: "Py Course", price: 999, }, { itemName: "DataScience Course", price: 1299, }, { itemName: "mobile dav Course", price: 5999, }, ]; //add all amount of shopping bag:- const BagAmount = shoppingBag.reduce((acc, item) => acc + item.price, 0); console.log(BagAmount);
Diagram of HTML Work in Windows
Windows Basic
console.log(document)
return every things for windows.console.log(document.baseURL)
then return URL of page.console.log(
document.link
)
then it returns all the links used in this document. Or, it returns the links in the HTML collection like an array of links.<!DOCTYPE html> <html lang="en"> <body> <h2 id="title" class="Header">DOM learning on Mritunjay kumar.</h2> <h2>Lorem ipsum dolor sit amet consectetur.</h2> <input type="number" /> <p>Lorem ipsum dolor sit amet.</p> <ul> <li class="list-item">One</li> <li class="list-item">Two</li> <li class="list-item">Three</li> <li class="list-item">Four</li> </ul> <script></script> </body> </html>
Getting value by ID
const get1 = document.getElementById("title");
console.log(get1);
return :- <h2 id="title">DOM learning on Mritunjay kumar.</h2>
console.log(get1.id);
return :- title
console.log(get1.class);
return :- undefined
//class is not use in Javascript use className
console.log(get1.className);
return :- Header
console.log(get1.getAttribute);
return:- ƒ getAttribute() { [native code] }
//it's a function
console.log(get1.getAttribute("id"));
return:- title
console.log(get1.getAttribute("class"));
return:- Header
Set value by ID
get1.setAttribute("class", "test");
In class attribute set to the class name is 'test' .
setAttribute will overwrite the value of the old attribute when set.
get1.setAttribute("class", "Header test");
IIt's set old value and new value.Set Style
get1.style.backgroundColor = "green"; get1.style.padding = "10px"; get1.style.borderRadius = "15px";
Get content
console.log(get1.textContent);
return :- DOM learning on Mritunjay kumar.
console.log(get1.innerHTML);
return :- DOM learning on Mritunjay kumar.
console.log(get1.innerText);
return :- DOM learning on Mritunjay kumar.
Different between textContent and innerText
textContent is not effected by HTML tag like
<b>,<i>
. etc.innerText is effected by HTML tag like
<b>,<i>
. etc.let h2 is
<h2 id="title" class="Header">DOM learning on <span style="display:none;">Mritunjay kumar.</span></h2>
.get1.innerText
=return :- DOM learning on
.get1.textContent
=return :- DOM learning on Mritunjay kumar
.get1.innerHTML
=return :- DOM learning on <span style="display:none;">Mritunjay kumar.</span>
.
Get Element by className
const get2 = document.getElementsByClassName("Header");
console.log(get2);
Get Element by querySelecter
const get3 = document.querySelector("#title");
const get3 = document.querySelector(".Header");
const get3 = document.querySelector("h2"); //Only return 1th element's. :- <h2 id="title" class="Header">DOM learning on Mritunjay kumar.</h2>
const get3 = document.querySelector('input[type="number"]');//its an css selecter. return:-<input type="number">
const get3 = document.querySelector('p:first-child'); //its an css selecter. return:-<input type="number">
Advance Selecter querySelector And querySelectorAll
const ulget = document.querySelector("ul");
return whole ul
const liget = ulget.querySelector("li");
return 1th li elements.
liget.style.color = "green";
liget.innerText = "5";
const ulgetAll = document.querySelectorAll("li");
return:-`NodeList(3) [li, li, li]` when you go inside prototype of nodeList.`its not array not work array prototype work only NodeList prototype`.
ulgetAll[0].style.color = "red";
//work
ulgetAll.forEach((item) => {
console.log(item.innerText);
return one by one all li text
item.style.background = "yellow";
item.style.margin = "10px";
});
Get element by className
console.log(document.getElementsByClassName(""));
return:- HTMLCollection [] anothe selecter ma null daga.
const getByClass = document.getElementsByClassName("list-item");
console.log(getByClass);
Return :- HTMLCollection(4) [li.list-item, li.list-item, li.list-item, li.list-item]
//How to select and work on all list
// then convert HTMLCollection [] into Array:-
let arr = Array.from(getByClass);
console.log(arr);
return:- (4) [li.list-item, li.list-item, li.list-item, li.list-item]
arr.forEach((l) => {
console.log(l);
return all element
l.style.color = "black";
});
Color Changer
<!DOCTYPE html>
<html lang="en">
<body>
<h1>Color Changer:</h1>
<div class="buttons">
<button class="#000000">#000000</button>
<button class="#FFFFFF">#FFFFFF</button>
<button class="#FFA384">#FFA384</button>
<button class="#FF0000">#FF0000</button>
</div>
</body>
</html>
const buttons = document.querySelectorAll("button");
const body = document.querySelector("body");
buttons.forEach((but) => {
but.addEventListener("click", (e) => {
// body.style.background = but.className;
//or
if (e.target.className === but.className) {
body.style.background = but.className;
}
});
});
Your Body Mass
<!DOCTYPE html>
<html lang="en">
<body>
<h1>Calculate Your Body Mass (BMI)</h1>
<form>
Height(cm): <input type="text" id="height" /><br /><br />
Weight(kg): <input type="text" id="weight" /><br /><br />
<button>Calcilate</button>
</form>
<div></div>
</body>
</html>
const form = document.querySelector("form");
//ya do vale ka yah is taraha sa select na kera andar ma ker form ka.
// const height = document.getElementById("height");
// const Weight = document.getElementById("weight");
form.addEventListener("submit", (e) => {
e.preventDefault();
/* e.preventDefault(); use to stope defoult option a form when submit its data get or post to data base thats way its refresh. to stop this to refresh use this.*/
const height = parseInt(document.getElementById("height").value);
const Weight = parseInt(document.getElementById("weight").value);
document.querySelector("div").innerHTML = `BMI is : ${
Weight / ((height * height) / 10000).toFixed(2)
}`;
});
Digital Clock
<!DOCTYPE html>
<html lang="en">
<body>
<h2>Your local time</h2>
<p id="time">hh:mm:ss</p>
</body>
</html>
const time = document.getElementById("time");
setInterval(() => {
//Most IMP for Intriview
const localTime = new Date();
time.innerHTML = localTime.toLocaleTimeString(undefined, {
hour: "2-digit",
minute: "2-digit",
second: "2-digit",
hour12: true,
});
console.log(localTime.toLocaleTimeString(undefined, { hour12: true }));
}, 1000);
Guess The Number
<!DOCTYPE html>
<html lang="en">
<body>
<h2>Guess the number bitween 1 to 100.</h2>
<form>
<input
type="text"
name="GuessNumber"
inputmode="numeric"
placeholder="gased number"
/>
<button>Submit</button>
</form>
<p><strong>Preview Guess</strong>:<strong class="Preview"></strong></p>
<p>
<strong>Guess Remaining</strong>:<strong class="Remaining">10</strong>
</p>
<button id="refresh">Refresh</button>
</body>
</html>
let GuessAray = [];
const randomNumber = Math.floor(Math.random() * 100);
const form = document.querySelector("form");
const storeForm = form.innerHTML;
const refresh = document.querySelector("#refresh");
const Preview = document.querySelector(".Preview");
const Remaining = document.querySelector(".Remaining");
refresh.addEventListener("click", (e) => {
GuessAray = [];
Preview.innerHTML = " ";
Remaining.innerHTML = "10";
form.innerHTML = storeForm;
});
form.addEventListener("submit", (e) => {
e.preventDefault();
let input = document.querySelector("input");
input = Number(input.value);
if (input > 100 || input < 1) {
alert("Please number enter bitween 1 to 100!");
} else {
Preview.innerHTML += `${input}, `;
Remaining.innerHTML = 10 - (GuessAray.length + 1);
GuessAray.push(input);
if (randomNumber === input) {
form.innerHTML = `<h2>Congratulation! You guessed it right, You win!<h2>`;
} else if (randomNumber < input) {
alert("Your guess is too high! Try again.");
} else if (randomNumber > input) {
alert("Your guess is too low! Try again.");
}
}
if (GuessAray.length > 9) {
form.innerHTML = `<h2>You complit the game!<h2>`;
}
});
Event Basic
<body>
<h2>Amazing Image</h2>
<ul id="images">
<li>
<img
width="100px"
height="150px"
id="book"
src="data:image/jpeg;base64,/9j/4AAQSkZJRgABAQAAAQABAAD/"
/>
</li>
<li>
<img
width="200px"
height="150px"
id="birds"
src="data:image/jpeg;base64,/9j/4AAQSkZJRgABAQAAAQABAAD/"
/>
</li>
<li>
<img
width="200px"
height="150px"
id="child"
src="data:image/jpeg;base64,/9j/4AAQSkZJRgABAQAAAQABAAD/"
/>
</li>
<li>
<img
width="200px"
height="150px"
id="goodNight"
src="https://encrypted-tbn0.gstatic.com/"
/>
</li>
<li>
<img
width="200px"
height="150px"
id="child"
src="https://encrypted-tbn0.gstatic.com/"
/>
</li>
<li>
<img
width="200px"
height="150px"
id="dog"
src="https://encrypted-tbn0.gstatic.com/"
/>
</li>
</ul>
<br />
<a href="https://google.com">Google.com</a>
</body>
addEventListener
JavaScript runs sequentially from top to bottom.
Avoid using functions directly inside any tag; it's not a good practice in pure JavaScript. Instead, place the function in the JavaScript code towards the bottom.
1th Approch
// Ya approch kam feature data. document.getElementById("child").onclick = function () { alert("Owl Click..."); }; //Ya function feature kam data hy
2nd Approch
This approach is correct, the best approach.
eventListener is give very usefulle feature.
cheak in property in console
var img = document.getElementById("goodNight");
img.addEventListener(
"click",
() => {
alert("Good Night Click");
},
false
);
//use 3 parameter, false is default parameter.
3rd Approch
attachEvent() & jQuery -on
ya dono old age ma use kerta tha.
event (e) property
This is the most important.
Check in property in console "Everything is a property of (e)".
This is a pointer event and an object.
Master all properties.
document.getElementById("dog").addEventListener(
"click",
(e) => {
console.log(e);
},
false
);
Basic events.
type, timestamp, preventDefault, target, toElement,srcElements, currentTarget, clientX, clientY, pageX, pageY, screenX, screenY, offsetX, offsetY which, metaKey,ctrlKey, shiftKey, altKey, keyCode
.
Event Proprgation
When we click on a child element, the parent element also gets clicked automatically. This is known as Event Propagation.
parent
->child
->grandchild
->great-grandchild
Nested child click happens when a child's parent is clicked, but the work is done from top to bottom in event propagation.
2 types of event Proprgation:-
Event Bubling,
Event Capturing
Capturing means from top to bottom.
Bubbling means from bottom to up.
In HTML there are two types of Events.
Traditional Events : mouseover ,mouseout etc..
Pointer Events : click ,touchstart etc...
To stop this Event Propgation we can use stopPropagation().
By defoult events false hotha hy jo ke event bubling hy.
Exmaple: When i click on ul element then automaticaly click next on li, Becouse li inside the ul.
let ul = document.getElementById("images"); let li = document.getElementById("birds");
Contition A
when 3rd keyWork false or empty or byDefoult.
ul.addEventListener( "click", (e) => { console.log("click on ul."); }, false ); li.addEventListener( "click", (e) => { console.log("click on li."); }, false );
when click on birds then console return:-
click on li.
click on ul.
when click on images then console return:-
- click on ul.
Use Bubling (bottom to top).
Contition B
when 3rd keyWork true
ul.addEventListener( "click", (e) => { console.log("click on ul."); }, true ); li.addEventListener( "click", (e) => { console.log("click on li."); }, true );
when click on birds then console return:-
click on ul.
click on li.
when click on images then console return:-
- click on ul.
Use Capturing (top to bottom).
Contition C
Stopt the event Bubbling and event Capturing
ul.addEventListener("click", (e) => { console.log("click on ul."); }); li.addEventListener("click", (e) => { console.log("click on li."); e.stopPropagation(); });
when click on birds then console return:-
- click on li.
when click on images then console return:-
- click on ul.
byDefoult (bottom to top).
preventDefoult()
Use to stop the byDefoult task of the tag funcanality.
const atag = document.querySelector("a"); atag.addEventListener("click", function (event) { event.preventDefault(); //this will stop the redirection of page after clicking the link. console.log("Google Click"); });
Project to remove the image when click
ul.addEventListener("click", (e) => { console.log(e.target); //return kis element pa click kia hy ap. // console.log(e.target.parentNode) let removeIt = e.target.parentNode; // removeIt.remove(); //or // removeIt.parentNode.removeChild(removeIt); /*In dono ma agar image pa click ho rha hy to image he gayab hoga lakin agar image ka bagae ul ka andar click hoga to pura ka pura body ka andar wala he gayab ho jayaga*/ //Solution (Add cheaks.):- console.log(e.target.tagName); //return IMG if (e.target.tagName === "IMG") { console.log(e.target.id); removeIt.remove(); } });
Async Work Diagram
Async Code
<!DOCTYPE html> <html lang="en"> <body> <h1>Java script course.</h1> <button id="stop">Stope</button> <button id="start">start</button> </body> </html>
setTimeout will run the code once after a specific time.
setTimeout(function () { console.log("Print after 2 second..."); }, 2000); // 2 second bad exicute hoga code const ChangeH1 = () => { const h1 = document.querySelector("h1"); h1.innerText = "Best java Script Course."; }; const stopSetTimeout = setTimeout(ChangeH1, 3000);
Stope
setTimeOut
usingclearTimeout(refrence of setTimeout)
.clearTimeout(stopSetTimeout);
or
document.querySelector("#stop").addEventListener("click", () => { clearTimeout(stopSetTimeout); });
setInterval
in the inner code runs in a loop, executing the code repeatedly after a given time. Take break and executed repeatedly in the loop function.setInterval(() => { console.log("Printing in loop after 3 second."); }, 3000); const Interval = function (str) { console.log(str, Date.now()); }; let intervalref; document.querySelector("#stop").addEventListener("click", () => { clearInterval(intervalref); }); document.querySelector("#start").addEventListener("click", () => { intervalref = setInterval(Interval, 1000, "Mritunjay"); });
Bg Color Change Advance Project
<!DOCTYPE html>
<html lang="en">
<body>
<h1>Change the background colour after 1 second continuesly.</h1>
<button id="start">Start</button>
<button id="stop">Stop</button>
</body>
</html>
const startBtn = document.getElementById("start");
const stopBtn = document.getElementById("stop");
let body = document.querySelector("body");
const generateRandomColor = () => {
const colorCode = "0123456789ABCDEF";
let color = "#";
for (let index = 0; index <= 5; index++) {
color += colorCode[Math.floor(Math.random() * 16)];
}
return color;
};
let interval;
startBtn.addEventListener("click", () => {
if (!interval) {
//cheak if null Better code to write
interval = setInterval(() => {
body.style.backgroundColor = generateRandomColor();
}, 1000); // Change every 1 sec
}
});
stopBtn.addEventListener("click", () => {
clearInterval(interval);
interval = null; //Profoconal way Agar kam nhi hy to hata do
});
Keword Tracker Project
<!DOCTYPE html>
<html lang="en">
<body>
<h1>Press the key and watch the magic.</h1>
<br />
<h1 id="keyboard"></h1>
</body>
</html>
// Get a reference to the body element.
const body = document.querySelector("body");
let strong = document.querySelector("#keyboard");
// Add a click event listener to the body element.
body.addEventListener("keypress", (e) => {
// Log the event object to the console when a click occurs.
strong.innerHTML += `${e.key} - ${e.code}<br/>`;
});
All are the part of
asyncronous setTimeout
,setInterval
or etc all the part of asyncronous.Asynchronous JavaScript is not a default part..
J**avascript ka engeion nhi hy asyncronous** but JavaScript ka complite run time asyncronous hy becouse all api sath ma ban ka aa rahi hy like setTimeout, setInterval or etc. Lakin Bydefoult javascript engein asyncronous nhi hy.
XMLHttpRequest
XMLHttpRequest: readyState property
Value | State | Description |
0 | UNSENT | Client has been created. open() not called yet. |
1 | OPENED | open() has been called. |
2 | HEADERS_RECEIVED | send() has been called, and headers and status are available. |
3 | LOADING | Downloading; responseText holds partial data. |
4 | DONE | The operation is complete. |
XMLHttpRequest()
It's a class.new XMLHttpRequest()
convert to object using new.xhr.onreadystatechange
=>Use to track the state her bar.This function asks us what to do. It is asynchronous.let body = document.querySelector("body"); const reqURL = "https://api.github.com/users/MritunjayKumar07"; const xhr = new XMLHttpRequest(); console.log("Set the xhr state:", xhr.readyState); xhr.open("GET", reqURL); xhr.onreadystatechange = () => { console.log(xhr.readyState); if (xhr.readyState === 4) { console.log("Request is completed!"); const data1 = xhr.response; console.log(data1); //or you use const data2 = JSON.parse(xhr.responseText); body.innerHTML += ` <div style="border: 2px solid #000000; width: 270px; border-radius: 10px;"> <div style="padding: 10px 10px"> <img width="250px" height="250px" style="border-radius: 10px" src=${data2.avatar_url} alt="image" /> <div> <h2>${data2.name}</h2> <strong><span>Location:</span> ${data2.location}</strong> <p>${data2.bio}</p> <div>Followers:${data2.followers} <br />Following:${data2.following}</div> <a style=" background: greenyellow; font-size: 18px; font-weight: 900; display: flex; width: 100%; text-align: center; justify-content: center; margin-top: 20px; padding-top: 10px; padding-bottom: 10px; border-radius: 15px; text-decoration: none; color:"#ffffff"; " href=${data2.html_url} target="_blank" >View profile</a > </div> </div> </div> `; } }; console.log("Open status:", xhr.readyState); xhr.send(); // use to confirm the open //or const xhr = new XMLHttpRequest(); console.log("UNSENT", xhr.readyState); // readyState will be 0 xhr.open("GET", "/api", true); console.log("OPENED", xhr.readyState); // readyState will be 1 xhr.onprogress = () => { console.log("LOADING", xhr.readyState); // readyState will be 3 }; xhr.onload = () => { console.log("DONE", xhr.readyState); // readyState will be 4 }; xhr.send(null);
console
Console javascript ka part nhi hy ya dev tool hy or deBug tool browser ka part hy.
Console javascript ka run time data hy. V8 engeon ma console ata hy.
V8 engein made in c or c++ link of git repo :- https://github.com/v8/v8Javascript wrape with C++
Promice
Imagine you have a task that takes some time to finish, like fetching data from the internet. The Promise is an Object like a guarantee or a commitment that the task will be completed in the future. It can either be successful (completed) or unsuccessful (failed). So, the Promise represents the result of that task (the value you get) once it's done, and it lets you handle the outcome, whether it's a success or a failure, in a structured way in your code.
A Promise is in one of these
Pending: initial state, neither fulfilled nor rejected.
Fulfilled: meaning that the operation was completed successfully.
Rejected: meaning that the operation failed.
Promice is a object (IMP)
Promise has two ways of handling it.
.that() .catch() .finally()
async await
Promice
Make Promise or create promise. And promise takes a callback function. Promise will return two things: either the promise is resolved or rejected.
Promice
const promiceOne = new Promise((resolve, reject) => { //Do an async task //DB call, criptography, network setTimeout(() => { console.log("Async task is complite"); resolve(); // Use to connect to then(); }, 3000); }); //Promice is created. //b> then() automatic connected hy resolve ka sath promiceOne.then(() => { console.log("Promic consume"); });
Promice
new Promise((resolve, reject) => { setTimeout(() => { console.log("Async second task is complite"); resolve(); // Use to connect to then(); }, 5000); }).then(() => { console.log("Asyn second resolve..."); });
Promice
const PromicThree = new Promise((resolve, reject) => { setTimeout(() => { console.log("Async third task is complite"); resolve({ username: "Mritunjay", email: "email@example.com" }); //send resolve data if promice successfull. }, 6000); }); PromicThree.then((res) => { console.log(res); });
Promice
const PromicFour = new Promise((resolve, reject) => { setTimeout(() => { console.log("Async four task is complite"); let error = true; if (!error) { resolve({ username: "Mritunjay", password: "123" }); } else { reject("Error in authentication"); } }, 7000); }); PromicFour.then((res) => { console.log(res); }).catch((err) => { console.log(err); });
Promice Channing Function
const PromicFive = new Promise((resolve, reject) => { setTimeout(() => { console.log("Async five task is complite"); let error = false; if (!error) { resolve({ username: "Mritunjay", password: "123" }); } else { reject("Error in authentication"); } }, 8000); }); PromicFive.then((res) => { console.log(res); return res.username; }) .then((name) => { //Channing console.log(`Hello ${name}`); }) .catch((err) => { console.log(err); });
Promice Channing Function
const PromicSix = new Promise((resolve, reject) => { setTimeout(() => { console.log("Async Six task is complite"); let error = false; if (!error) { resolve({ username: "Mritunjay", password: "123" }); } else { reject("Error in authentication"); } }, 9000); }); PromicSix.then((res) => { console.log(res); }) .catch((err) => { console.log(err); }) .finally(() => { //finally is run alwase ither promice complit or not. console.log("The promic is either resolve or rejected."); });
Promice
const PromicSeven = new Promise((resolve, reject) => { setTimeout(() => { console.log("Async seven task is complite"); let error = true; if (!error) { resolve({ username: "Javascript", password: "123" }); } else { reject("Error in authentication"); } }, 10000); }); //Use asyn awai same as a .then or .catch //async wait kerta hy kam hona ka agar kam ho jata hy to he aga badhata hy kam ma. warna wahi pa error da data hy. ConsumePromicSeven = async () => { //async ma error nhi ayage. const response = await PromicSeven; console.log(response); }; ConsumePromicSeven(); //GIve error
Promice
const PromicEight = new Promise((resolve, reject) => { setTimeout(() => { console.log("Async eight task is complite"); let error = false; if (!error) { resolve({ username: "C++", password: "123" }); } else { reject("Error in authentication"); } }, 10000); }); //Use asyn awai same as a .then or .catch //async wait kerta hy kam hona ka agar kam ho jata hy to he aga badhata hy kam ma. warna wahi pa error da data hy. ConsumePromicEight = async () => { //async ma error nhi ayage. const response = await PromicEight; console.log(response); }; ConsumePromicEight();
Promice
const PromicNine = new Promise((resolve, reject) => { setTimeout(() => { console.log("Async nine task is complite"); let error = false; if (!error) { resolve({ username: "Python", password: "123" }); } else { reject("Error in authentication"); } }, 10000); }); //Use asyn awai same as a .then or .catch //async wait kerta hy kam hona ka agar kam ho jata hy to he aga badhata hy kam ma. warna wahi pa error da data hy. ConsumePromicNine = async () => { //async ma error nhi ayage. try { const response = await PromicNine; console.log(response); } catch (error) { console.log(error); } }; ConsumePromicNine();
Promice
getAllUses = async () => { //fetch ak object hy network url hy time lagaga that way use await. // https://api.github.com/users try { const res = await fetch("https://api.github.com/users"); console.log(res); const data = await res.json(); //To convert the json it's take time that way hear also use await. console.log(data); } catch (error) { console.log(error); } }; // getAllUses();
Promice
Error occurs catch when it fails to fetch the network URL. .
But another status code error will come in the response then it's come from API.
fetch("https://api.github.com/users")
.then((res) => {
console.log(res);
})
.catch((err) => {
console.log(err);
});
- One problom : Why api call and error first and othe which are top of the api call are call last after call api. Becouse We learn in lase events async code 'Promice Queue/Micro task Queue' that way it's run first .Iska Priority jayda rahata hy ya Task Queue apacely fetch ka lia banta hy.
Fetch
Intro about OOP
Javascript and classes
- Javascript is prototype-based language.
OOP
Object Oriented Programming (OOP) is a programming paradigm(paeadime) that uses "objects".
Object
collection of properties and method.
Ex : toLowercase, Promice, Data and etc.
why use OOP
Reusability - if we have common functionality in multiple objects then instead of writing the same code again and again we can create one object which contains this functionlity and other objects can use it by calling this object. This saves time and effort.
Maintainability - If there are 50 forms in an application and all these forms need to display date in dd/mm/yyyy format then instead of changing code for each form we just change the behavior of Date object.
Extensibility - We can add new features to existing objects without modifying them.
Modularity - It helps us to group related functionalities together under single entity i.e., A class represents a module or unit of software.
Modularity - It helps us to group related functionalities together under single entity called class and make our program more modular.
Part of OOP
Object literal
Constructor function
Prototypes
Classes
Instances (new, this)
4 Pillars
Encapsulation
Inheritance
Polymorphism
Abstraction
Object literal
const user = {
name: "John Doe",
age: 32,
email: "john.doe@example.com",
getUserDetails: function () {
console.log("this is use for current context:-", this.name);
},
};
console.log(user.name);
console.log(user.getUserDetails());
console.log(this);
return :- {}
this keyword is Object.
{} No current context. Empty because in Node.js, this does not have any value. But in the browser, inside this, the current context is window.
Constructor function
Use
new
to make Constructor function.new
key word use to create new context.const promiseOne = new Promise();
const date = new Date();
Function also like that.
function User(username, loginCount, isLoggedIn) { //Also key or variable define like that. this.username = username; //`this.username` is a variavle this.loginCount = loginCount; this.isLoggedIn = isLoggedIn; this.generate = function () { console.log(Math.floor(Math.random() * 10)); }; return this; //`this` return the current context. } const userOne = User("Mritunjay", 12, true); const userTwo = User("Amit", 10, false); console.log(userOne); console.log(userTwo.isLoggedIn);
In that way pass parimeter is overWrite the data of
User
Solution: Constructor function.
new: Use
new
to create an empty object. The function call happens because of thenew
keyword. Due to the new keyword, whatever data is there, it packs it inside itself.
Property and method
Inharit 2 way
Prototype Inheritance (proto)
const User = { name: "John Doe", age: 25, }; const Admin = { role: "Admin", level: 999, __proto__: User, //1th Way (Inharit from inside) }; const Support = { role: "Support", level: 777, };
Support.__proto__ = User;
2nd Way (Inharit from OutSide)console.log(Admin.name); return :- John Doe console.log(Support.name); return :- John Doe console.log(User.role); return :- undefine
Using Modern syntax (Behinde the seen wahi chal raha hy
__proto__
)- Object.setPrototypeOf(Admin, User); //Admin ko User ka axcess dia.
Replace trim().length to Own method
let anotherUser = "Mritunjay ";
String.prototype.trimLength = function () {
console.log("Return this", this);
Return:- Return this [String: 'Mritunjay ']
// console.log(this.trim().length);
//or
return this.trim().length;
};
console.log("Normal length", anotherUser.length);
Return:26
console.log("Using Trim Length", anotherUser.trimLength());
Return:-Using Trim Length 9
console.log("Using Trim Length", "Mritunj ".trimLength());
Return:-Using Trim Length 7
Call Stack Behavior function in side function
Call Stack
function CheakUserName(userName) {
this.userName = userName;
}
function CreateUser(userName, email, password) {
CheakUserName(userName);
//CheakUserName(userName); It should have automatically been set to this.userName = userName; because this task was outsourced, so it should have happened.
this.email = email;
this.password = password;
}
//Here, we are creating a new object and storing all the values in it.
const chai = new CreateUser("Mritunjay", "mritunj@gmail.com", "123");
console.log(chai);
return:- CreateUser { email: 'mritunj@gmail.com', password: '123' }
Ya problom hy
solution:-
function CheakUserName1(userName) { this.userName = userName; console.log("Called 1"); }
function CreateUser1(userName, email, password) { CheakUserName1(userName); /*1st point: Here, we are not calling the function, we are just passing a reference to it. 2nd point: Because CheakUserName1() is globally executed, it executes when the global execution starts. This means that when the global execution starts, the CheakUserName1 function is executed and removed from the call stack. In execution, whatever is executing will be removed once it's done executing. So, to hold a reference, there are various ways like .bind, .call, and so on. 3rd point: Functions behave in two ways: as objects and as functions.*/ CheakUserName1.call(this, userName); // We are passing our this. Then, CheakUserName1 won't use its own this, it will use my this. It means we are shifting our current execution to CheakUserName1. //This implies that we are transferring our current execution to CheakUserName1. this.email = email; this.password = password; } const chai1 = new CreateUser1("Mritunjay", "mritunj@gmail.com", "123"); console.log(chai1); return:- CreateUser { email: 'mritunj@gmail.com', password: '123' }
Class
class User {
constructor(username, email, password) {
//Bassic Constructrer same as `new` keyword
this.username = username;
this.email = email;
this.password = password;
}
//In classes, functions are used like this
encriptPassword() {
// this is `metthod`
return `${this.password}abc`;
}
changeUsername() {
return `${this.username.toUpperCase()}`;
}
}
Creating object and place all value
const chai = new User("Mritunjay", "mk@gmail.com", "123");
//apply own custome method.
console.log(chai.encriptPassword());
Return :- 123abc
console.log(chai.changeUsername());
Return :- MRITUNJAY
//Top code Behind the code How thay work
function User1(username, email, password) {
this.username = username;
this.email = email;
this.password = password;
}
User1.prototype.encriptPassword = function () {
return `${this.password}abc`;
};
User1.prototype.changeUsername = function () {
return `${this.username.toUpperCase()}`;
};
const tea = new User1("MritunjayKumar", "mkr@gmail.com", "1234");
console.log(tea.encriptPassword());
Return :- 1234abc
console.log(tea.changeUsername());
Return :- MRITUNJAYKUMAR
Inheritance
class User {
constructor(username) {
this.username = username;
}
logMe() {
console.log(`User Name is ${this.username}`);
}
}
//`Extends` is providing all the functionalities of the "User" to the "Teacher".
class Teacher extends User {
constructor(username, email, password) {
super(username);
/*The super keyword is used to call the constructor of the parent class,
which is User, and within the constructor, this keyword is automatically added by default.*/
this.email = email;
this.password = password;
}
addCourse() {
console.log(`A new course was addes by ${this.username}`);
}
}
// const chai = Teacher("John Doe", "john@gmail.com","123456"); //Return :- TypeError: Class constructor Teacher cannot be invoked without 'new'
const chai = new Teacher("John Doe", "john@gmail.com", "123456");
chai.addCourse();
Return :- A new course was addes by John Doe
chai.logMe(); //Axcess
Return :- User Name is John Doe
const tea = new User("tea");
// tea.addCourse() //Not axcess
Return:- TypeError: tea.addCourse is not a function
tea.logMe();
Return:- User Name is tea
//Cheak equal or not:-
console.log(tea === chai);
Return false
console.log(tea === Teacher);
Return false
//For cheak correct way
console.log(chai instanceof User);
Return true
console.log(chai instanceof Teacher);
Return true
StsticProp
class User {
constructor(username) {
this.username = username;
}
logMe() {
console.log(`UserName is: ${this.username}!`);
}
creteId() {
return `123`;
}
}
const hitesh = new User("Mritunjay");
console.log(hitesh.creteId()); // 123
hitesh.logMe(); // UserName is: Mritunjay!
class User1 {
constructor(username) {
this.username = username;
}
logMe() {
console.log(`UserName is: ${this.username}!`);
}
//static use for stope the axcess of this method.
static creteId() {
return `123`;
}
}
const mritunjay = new User1("Mritunjay");
hitesh.logMe(); // UserName is: Mritunjay!
console.log(mritunjay.creteId()); // TypeError: mritunjay.creteId is not a function
class Teacher extends User1 {
constructor(username, email) {
super(username);
this.email = email;
}
}
const tech = new Teacher("Mritunjay", "Mk@gmail.com");
tech.logMe(); // UserName is: Mritunjay!
console.log(tech.creteId()); // TypeError: tech.creteId is not a function
Bind
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8" />
<meta name="viewport" content="width=device-width, initial-scale=1.0" />
<title>React</title>
</head>
<body>
<button>Botton Click</button>
</body>
<script>
class Ract {
constructor() {
this.library = "React";
this.server = "https://localhost:3000";
//requirement
document
.querySelector("button")
.addEventListener("click", this.handleClick);
}
handleClick() {
console.log("Button clicked!"); //Button clicked!
console.log(this.server); //Undefine
console.log(this); //<button>Botton Click</button>
}
}
const App = new Ract();
//Use bind to give axcess of constructor to handleClick
class Ract1 {
constructor() {
this.library = "React";
this.server = "https://localhost:3000";
//requirement
document
.querySelector("button")
.addEventListener("click", this.handleClick.bind(this));
//bind(this) is to send bind the all thing and send.
}
handleClick() {
console.log("Button clicked 1!"); //Button clicked!
console.log(this.server); //https://localhost:3000/
console.log(this); //Ract1 {library: 'React', server: 'https://localhost:3000'} library:"React" server : "https://localhost:3000" [[Prototype]] : Object
}
}
const App1 = new Ract1();
</script>
</html>
MathPI
Math.PI ka 3.1415 sumthing hy kya hum change ker sakata hy is ko. And its constant in JS.
console.log(Math.PI); //3.141592653589793 Math.PI = 5; console.log(Math.PI); //3.141592653589793
But we can not change the value of Math.E because it's a read-only
getOwnPropertyDescriptor
using that to get thePI
value fromMath
module
const descripter = Object.getOwnPropertyDescriptor(Math, "PI");
console.log(descripter);
Return: {
value: 3.141592653589793,
writable: false,
enumerable: false,
configurable: false,
};
Two way to create Module
1th way:-
const myMethod = Object.creter(null).
create()
thia is factory function, By default, it is null in a factory function.
Older Way
const chai = { //Normal Object name: "Masala chai", price: 50, isAvelabe: true, }; console.log(chai); Return: { name: 'Masala chai', price: 50, isAvelabe: true }
Lets gae to the point's
console.log(Object.getOwnPropertyDescriptor(chai, "name")); eturn: -{ value: "Masala chai", writable: true, enumerable: true, configurable: true, };
Make unchangable
Object.defineProperty(chai, "name", { writable: false, enumerable: false, //The reason it doesn't show name: "Masala chai", in the return of getOwnPropertyDescriptor when iterated is that... configurable: true, });
console.log(Object.getOwnPropertyDescriptor(chai, "name")); return: { value: 'Masala chai', writable: false, enumerable: false, configurable: true }
Itrate the chai and giv the all value
for (let [key, value] of chai) { console.log(`${key} : ${value}`); } return : TypeError: chai is not iterable //There for use that way for (let [key, value] of Object.entries(chai)) { console.log(`${key} : ${value}`); } return : price : 50 isAvelabe : true
What is code burst
const chai2 = { name: "Masala chai2", price: 150, isAvelabe: true, order: function () { console.log("This code fatna"); }, }; for (let [key, value] of Object.entries(chai2)) { console.log(`${key} : ${value}`); } return: Return:- name : Masala chai2 price : 150 isAvelabe : true order : function () { console.log("This code fatna"); }
GaterSater
Getter and Setter Using new metthod
class User { constructor(email, password) { this.email = email; this.password = password; } /*- Getters and setters are present in every class by default.. - Whatever properties are defined automatically get a default getter and setter method with the same name. For example, the property "email" gets a method named "email()", and the property "password" gets a method named "password()". - We can change its value.*/ /*IMP := In simple terms, "getters" (get) and "setters" (set) are used to control how values are retrieved and assigned. When someone tries to retrieve a value (using a getter), you can customize what value is returned. When someone tries to set a value in the constructor, you can edit and customize the value before setting it.*/ //If we don't want to provide someone with our password, we can use getters and setters for them. /*Get used to it: when anyone retrieves a value from outside the class, they should do it my way. */ /*If you use only get not use set then come error:- TypeError: Cannot set property password of #<User> which has only a getter*/ // get password() {//Any one want to get the value // return this.password.toUpperCase(); // } // set password(value) {//Any one want to set the value // this.password = value; // } /*When you use set like that then come error :- RangeError: Maximum call stack size exceeded. - The error occurs because the call stack is becoming full due to repeated executions. It's running repeatedly because the constructor is also setting and getting values in the getter and setter methods, and you're also getting and setting values in the getter and setter methods using get password(){} and set password(){}.*/ // set use to set the value in constructer //Solution:- get password() { return this._password.toUpperCase(); //We have changed the password to _password (created a new property), We did this to avoid an error from occurring.:- RangeError: Maximum call stack size exceeded } set password(value) { this._password = value; //We changed the password and created a new property called _password. We did this to prevent an error from occurring. :- RangeError: Maximum call stack size exceeded } //Behind the code constructer set ker raha valu only in emal, password set nhi ker raha hy Q ke hum password ko over right ker dia hy. } const mritunjay = new User("mritunjay@gmail.com", "123456abcde"); console.log(mritunjay.password); //123456ABCDE
Getter and Setter Using function base
Pahala class nhi tha to function use kerta tha.
function UserFun(email, password) { this._email = email; this._password = password; /*And the object itself also enables accessing getter and setter properties, with the help of defineProperty function, which is also an object.*/ //`defineProperty` doesn't have its own 'this' here, it gets passed from here. Which property to work with, then the Object. Object.defineProperty(this, "email", { get: function () { return this._email.toUpperCase(); }, set: function (value) { this._email = value; }, }); } var user = new UserFun("mritunjay@gmail.com", "12456"); console.log(user.email); //MRITUNJAY@GMAIL.COM
Getter and Setter Using Object base
UserObj = { _email: "mritunjay@gmail.com", _password: "1234567890", get email() { return this._email.toUpperCase(); }, set email(value) { this._email = value; }, };
create` this is factory function ya Array and Object ma use kerta hy.
Ya bolta hy ke kiska bases pa hum function create kera. by defoult null hota hy. create(null).
const tea = Object.create(UserObj); console.log(tea.email);
dono same hy _email or email.
_
undersore ya batata hy ke hum private property define ker raha hy jo normal use ka use ma nhi ayaga.geter amd setter ko ya fark nhi padata hy ke kon sa property private hy usa sirf name chaia.
Getter and setter ak method hy jo property ka upar lag raha hy.
get email() { //A getter method has been applied to any property, name, whether it's private or anything else. return this._email.toUpperCase(); //Then changed the value of the property. },
UserObj = {
email: "mritun@gmail.com",
_email: "mritunjay@gmail.com",
_password: "1234567890",
get email() {
return this.email.toUpperCase();
},
set email(value){
this.email = value;
}
};
//Comer erro:- RangeError: Maximum call stack size exceeded
UserObj = {
email: "mritun@gmail.com",
_email: "mritunjay@gmail.com",
_password: "1234567890",
get email() {
return this._email.toUpperCase();
},
set email(value){
this._email = value;
}
};
Return :- MRITUNJAY@GMAIL.COM
Closers
A closure is a function that has access to its own scope, the outer function’s scope.
<!DOCTYPE html> <html lang="en"> <head> <meta charset="UTF-8" /> <meta name="viewport" content="width=device-width, initial-scale=1.0" /> <title>Closer</title> </head> <body> <h1>Closers</h1> <span >closure gives you <strong>access to an outer function's scope</strong> from an inner function. In JavaScript, closures are created every time a function is created, at function creation time. </span> <br /> <br /> <br /> <br /> <button id="orange">Orange</button> <button id="green">Green</button> <!-- Note: Higher order experience is like setTimeout, setInterval, and so on. --> </body> <script> //Lexical (Before understanding "closures," you first need to understand "lexical."):- function Outer(params) { let myName = "Mritunjay"; } // console.log(myName); //Return error:- 01_Closers.html:59 Uncaught ReferenceError: myName is not defined function OuterFun() { let name = "Mozilla"; function InnerFun() { console.log(name); // use variable declared in the parent function } InnerFun(); } OuterFun(); //"Lexical scope" means that the parent scope shares its memory with the child scope. //Closers:- function makeFunc() { const name = "Mritunjay Kumar"; function displayName() { console.log(name); } return displayName; //Hear we return function refrence. Or return execution. /*More importantly, we are passing the entire memory.*/ //In intriview speak only lexical. // We are providing the entire Lexical scope return } //We are providing a reference of entire outer and inner function in myFun. const myFunc = makeFunc(); // makeFunc() We are not execute here, we are only giving refrence now. //If we execute the function, it will run and then finish. (execute and remove from call stack) myFunc(); //We execute it here. console.log(myFunc.name); // return :- displayName //A "closers" function returns a function that is inside another function. </script> <script> //#01:- document.getElementById("orange").onclick = () => { document.body.style.background = `orange`; }; document.getElementById("green").onclick = () => { document.body.style.background = `green`; }; //This is not good ways if there are 50 buttons //#02:- const clickHandler = (color) => { document.body.style.background = color; }; /*document.getElementById("orange").onclick = clickHandler; Here, we are passing a reference to the function. It means that we are providing the definition of the function.*/ // document.getElementById("orange").onclick = clickHandler("orange"); // document.getElementById("green").onclick = clickHandler("green"); //#03:- //Lexical and Closer real-world problom solution. const clickHandler2 = (color) => { return () => { document.body.style.background = color; }; //clickHandler2 return another function unNamed function. }; document.getElementById("orange").onclick = clickHandler2("orange"); document.getElementById("green").onclick = clickHandler2("green"); </script> </html>
Subscribe to my newsletter
Read articles from Mritunjay Kumar directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
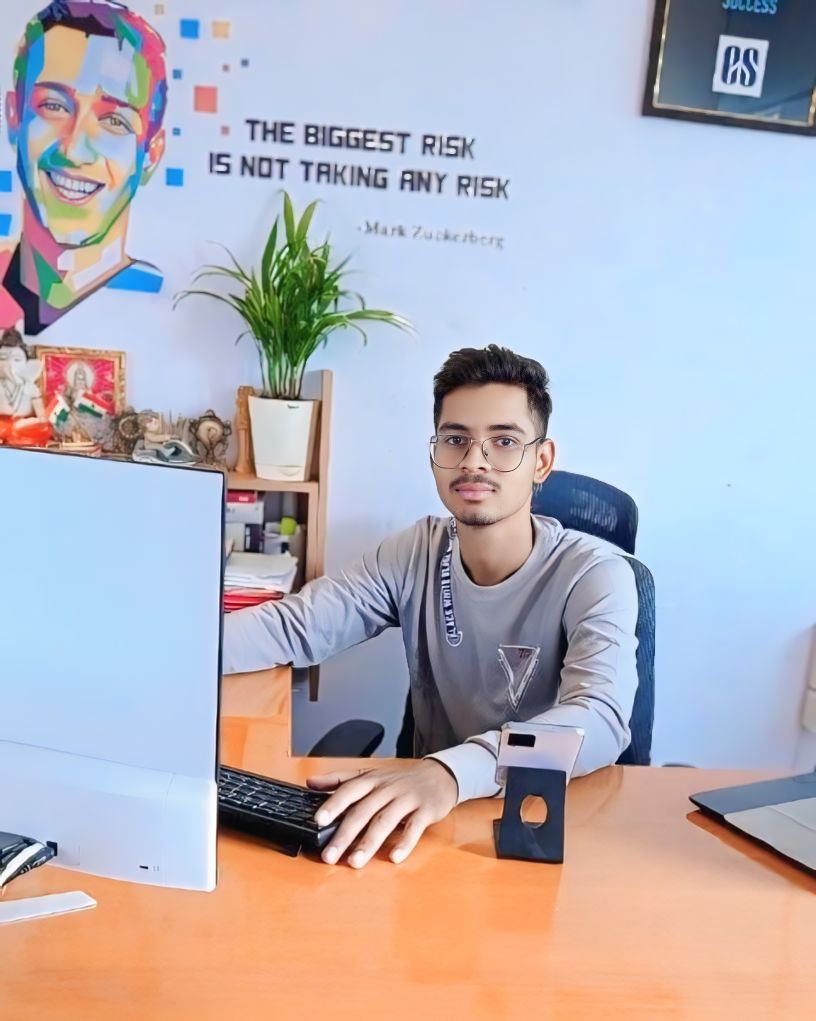