Full Stack Web Application using Next JS
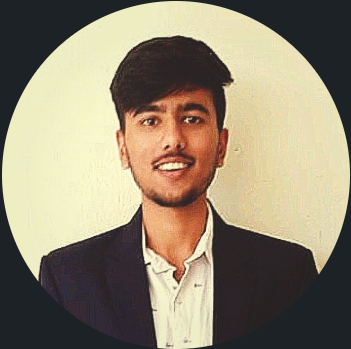
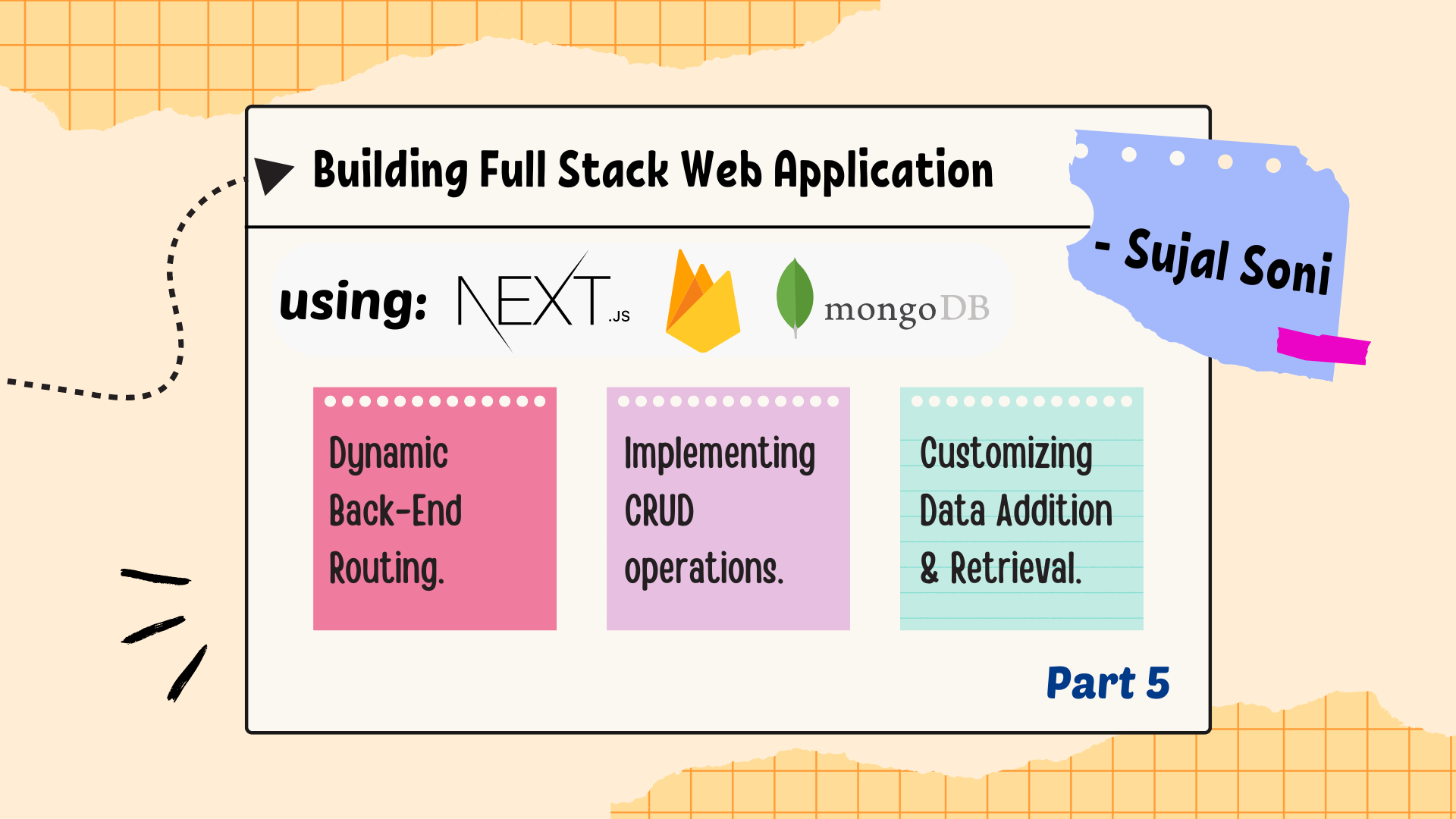
Here's the 5th blog in this ongoing series, continuing our journey of building a Full Stack Web Application using Next JS.
Till now, I have uncovered the secrets of implementing backend with Next JS and performing User Authentication & Token Generation. Also In the previous blogs , I have provided the steps to setup Next JS project from scratch.
Coming up Next โฉ:
Setting up User Data Routes and User Data Model.
Setting up Frontend Pages for displaying essential data.
Explanation of CRUD operations being performed during the process.
Before starting up, let me introduce myself ๐ฆ๐ผ
I'm Sujal Soni, a dedicated Full Stack Web Developer proficient in technologies like Node.js, Express.js, React.js, and MongoDB. With a passion for problem-solving and attention to detail, I've successfully completed various projects. Eager to contribute to innovative projects and collaborate with talented teams to drive impactful solutions.
You can connect with me on Linkedin as well as on Twitter ๐จ๐ผโ๐ป
If you are following this series from the start, then I'm sure that you have made and understood important bits of next.js project setup.
Now we shall continue with the further setup and coding โฌ
File Setup:
- Model file:
Create a new file named
userData.js
in yourmodels
directory.This will be used to define the schema for your user data using Mongoose.
- Route files:
Create a new folder inside the
api
folder as "userData".Inside
userData
folder, add a route file as "route.js" and another folder namedid
.Inside the
id
folder add a file as "route.js"
You might be wondering why 2 route files are arranged in this order inside userData
folder ๐ค
Here is a simple diagram to explain the same :
Route file (route.js
) marked as 1*does not require any * id (data ID) in order to proceed with the request. It would contain the POST and GET request functions.
Route file (route.js
) marked as 2*necessarily requires id* (data ID) in order to proceed with the request. It would contain the GET, PUT (update) and DELETE request functions.
๐กA similar pattern can also be seen in the frontend file arrangement where some pages require "id" to access them. Following section covers one such page arranged as "dashboard/editData/[id]/page.js
".
- Frontend files:
Inside "dashboard" folder, add a
page.js
file. This would typically act as a Home page for Dashboard.Add 2 folders inside the Dashboard as
addData
andeditData
.Further add
page.js
files inside both the folders respectively.You can access these pages using the following URLs:
Dashboard Home:
localhost:3000/dashboard
Add Data:
localhost:3000/dashboard/addData
Edit Data:
localhost:3000/dashboard/editData/{dataID}
Refer to the directory structure below for reference:
src/app/dashboard โโโ page.js โโโ addData/ โ โโโ page.js โโโ editData/[id] โโโ page.js
Code Reference:
You can either put your own creativity inside the files or you can simply refer to my GitHub Repository for adding the code:
https://github.com/Sujal-2820/Full-Stack-Web-Application
Understanding the Code:
models/userData.js:
It defines the structure of the UserData model using Mongoose's Schema.
Fields to focus on:
title: A required string field representing the title of the data.
category: A required string field representing the category of the data.
description: A required string field representing the description of the data.
imageURL: A string field representing the URL of an image associated with the data. It has a default value of null because we currently will not be including the image along with data. This will be covered in the next blog.
date: A date field representing the creation date of the data. It has a default value of the current date and time, and it's marked as immutable to prevent changes to this field once the data is created.
userID: This field is used to associate the userID with the UserData It references the ObjectId of the user model.
/api/userData/route.js:
This file handles POST and GET requests for user data.
POST request function:
Creates new user data entry based on provided JSON body.
Verifies authorization token from cookies.
Extracts user ID from decoded token.
Saves new user data to the database.
Responds with success message and status code 201 if successful, otherwise error message and status code 500.
GET request function:
Retrieves user data entries associated with the authenticated user.
Verifies authorization token from cookies.
Extracts user ID and username from decoded token.
Queries the database for user data based on user ID.
Responds with username and user data if successful, otherwise error message and status code 500.
/api/userData/[id]/route.js:
This file handles GET, PUT, and DELETE requests for specific user data by ID.
GET request:
Receives the Data ID from Frontend.
Retrieves user data entry by ID from the database.
Responds with the user data if found, otherwise returns a 404 error.
PUT request:
Updates user data entry by ID with the provided JSON body.
Verifies authorization token from cookies.
Checks if the token value is present.
Finds the userData document by ID and updates it with the new data.
Responds with a success message if the update is successful, otherwise returns an error message.
DELETE request:
Deletes user data entry by ID from the database.
Verifies authorization token from cookies.
Checks if the token value is present.
Finds the userData document by ID and deletes it.
Responds with a success message if the deletion is successful, otherwise returns an error message.
/src/dashboard/page.js:
This file defines the main page for the dashboard, responsible for rendering the user interface of user dashboard.
It defines functions for handling sorting, fetching user data, deleting user data, truncating title and description, and formatting date.
In the useEffect hook, it calls the fetchData function when the component mounts to fetch user data from the route file.
Image Snippet of Dashboard:
How to send Data ID from dashboard page to editData page or any page showing a particular Data?
Focus upon the diagram representation showing flow of data from dashboard/page.js
to dashboard/editData/[id]/page.js
The above diagram clearly depicts how "dataID" flows from one component to another in order to correctly fetch the data you desire to get.
Well Done ๐, you have successfully implemented CRUD operations inside the Next JS project using routes and model.
In the further parts of this series "Building Full-Stack Web Application using Next.js" I would be covering the following:
Image Upload using Firebase ๐ฅ
Deploying the project globally to make it shareable ๐
In case of any doubt, you can reach out to me via LinkedIn ๐จโ๐ป
You can also connect with me on various other platforms like Twitter, GitHub
If you liked this Blog, make sure to give it a ๐ and do Follow me on this platform to get notified for my next blogs in this series.
Happy Coding!! ๐จโ๐ป
Subscribe to my newsletter
Read articles from Sujal Soni directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
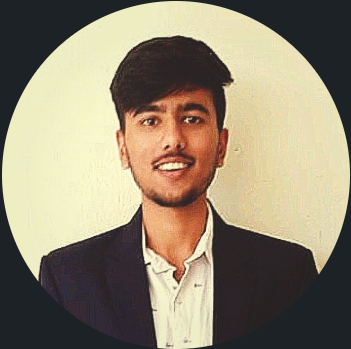
Sujal Soni
Sujal Soni
A passionate Web Developer who seeks to learn new Technologies every day