NetSuite SFTP Module: Download and Upload Files to SFTP server in SuiteScript 2.1
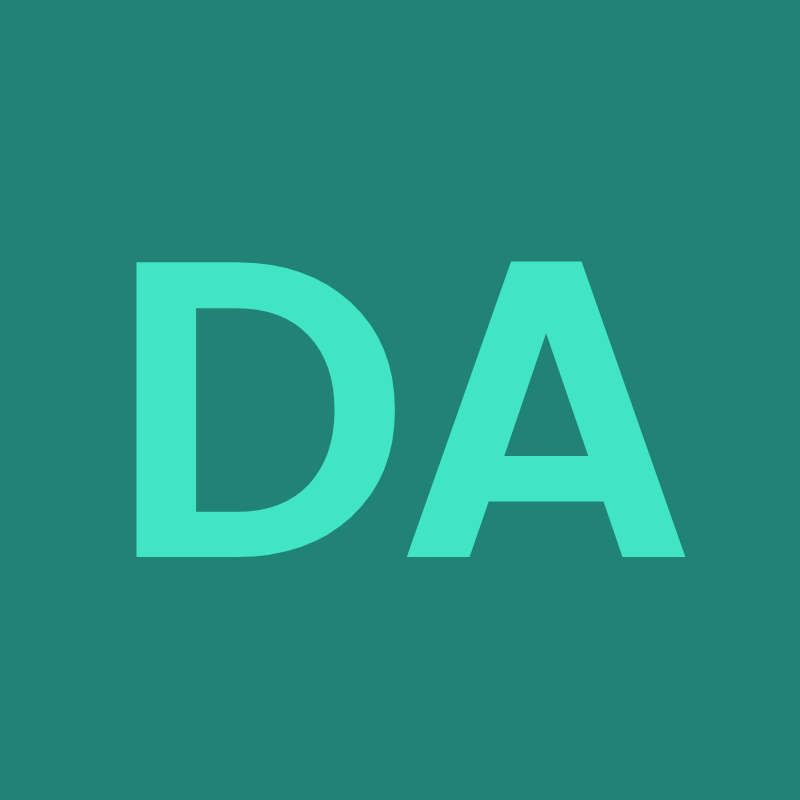
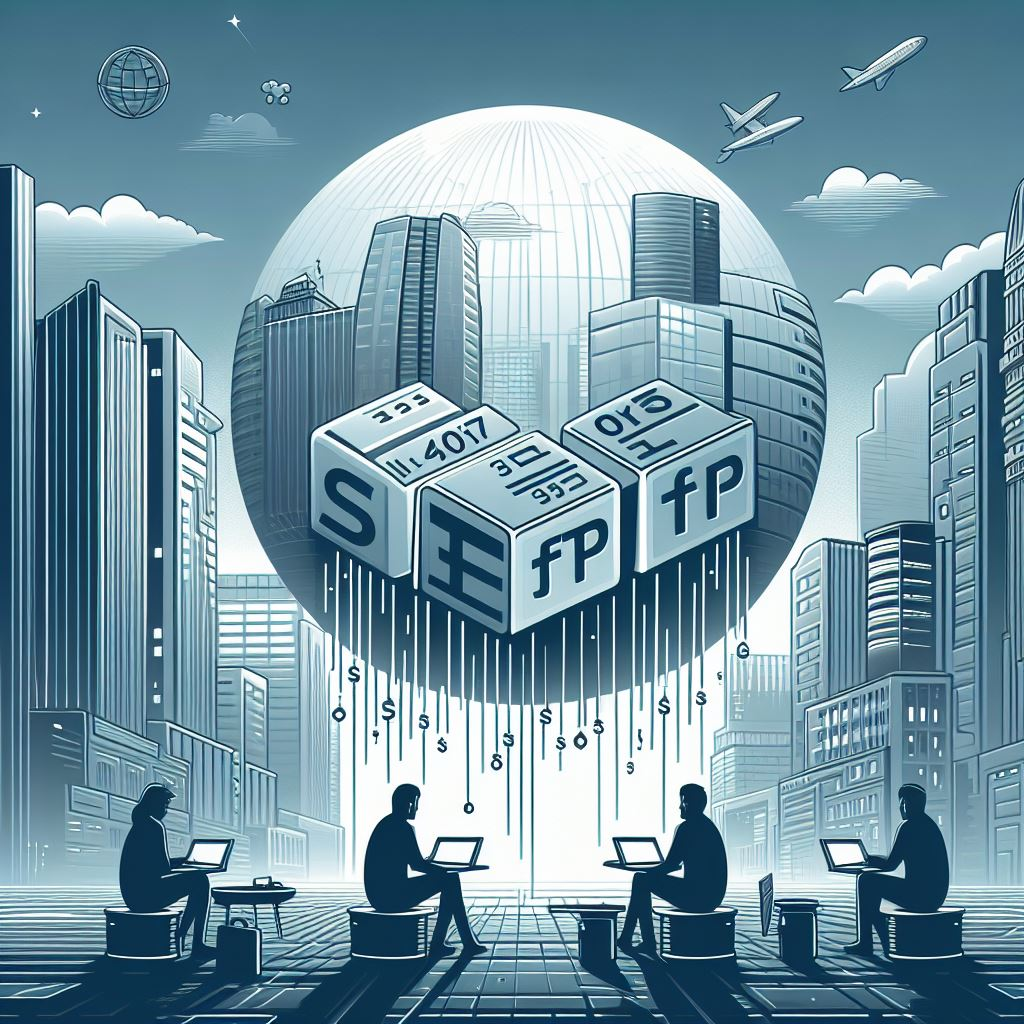
In this post, we will upload a sample text file to SFTP server, then download the same file from the server and log it into execution logs.
For SFTP connection, first we need password GUID which can be created suing a suitelet form and credentials fields.
const credField = form.addCredentialField({
id: 'password',
label: 'Password',
restrictToDomains: ['ftp.domain.com'], // FTP/SFTP server domain
restrictToCurrentUser: false,
restrictToScriptIds: 'customscript_my_script' // Script using SFTP Module
});
You can find the full suitelet at this link
https://yourcompanyID.app.netsuite.com/app/help/helpcenter.nl?fid=article_0110064929.html
Replace yourcompanyID with your NetSuite account ID.
restrictToDomains is the FTP or SFTP server URL.
restrictToScriptIds is the script ID of your current deployed script from where you are performing SFTP operations.
After you have Password GUID, we need to create a host key.
Use following command to generate host key on a linux server
ssh-keyscan -t rsa -p 22 ftp.domain.com
After you are done with all the above steps now we can create a scheduled script to upload and download a file from SFTP server. Please note that you can use you FTP server as SFTP just change the port number to 22, but this should be supported by your server.
Full source code of the working scheduled script.
/**
* @NApiVersion 2.1
* @NScriptType ScheduledScript
*/
define(["N/sftp", "N/file"], /**
* @param{sftp} sftp
*/ (sftp, file) => {
const passwordGUID = "195e8110afa84b84b3d24a08e2402b4e";
const hostKey =
"AAAAB3NzaC1yc2EAAAADAQABAAABAQDtya9/VvSaYpk3kNfGsfJEeDlvowFjFz7FB4xooHCM8hro0tUDlYVbjjSr5f5FQh0dz+FJl1fwxUO9foHlf1CLh9712fpURFQ0VTZirhI88Bo2WiUe4ACX/Na45rk+Jo3lAIfh9vL7oeCm8h5/ipXKTmpO57J9vDR36Rh5xBpyhqql7dilL4nJcCpllu45tAZRIMHVjAQNDJ+cnQeVIK7VhZ4q64g8JXdCKWH/xeHe9E8d0m7V3P6qSNwTu8+xCnVjq3cIRLZMUPBqQ17ecggB4Iz4dyPsQWv+QfKOFyC6uL3PiN0trw/vmGjbmS9a411cua8pnEzLgmRs/j2q8IeX";
const userName = "contezsg";
const port = 22;
const sftpURL = "ftp.domain.com";
const netsuiteUploadFolderId = 296; // Upload files from this folder to SFTP Server
const netSuiteDownloadFolderId = 297; // Download files from SFTP server to this folder
const SFTPDirectory = "netsuite";
const SFTPUploaddirectory = "upload";
/**
* Defines the Scheduled script trigger point.
* @param {Object} scriptContext
* @param {string} scriptContext.type - Script execution context. Use values from the scriptContext.InvocationType enum.
* @since 2015.2
*/
const upload = (connection, fileId, SFTPUploaddirectory) => {
try {
connection.upload({
directory: SFTPUploaddirectory,
file: file.load({ id: fileId }),
replaceExisting: true,
});
} catch (e) {
if (e.name === "FTP_NO_SUCH_FILE_OR_DIRECTORY") {
connection.makeDirectory({
path: "upload", // netsuite/upload
});
connection.upload({
directory: SFTPUploaddirectory,
file: file.load({ id: fileId }),
replaceExisting: true,
});
}
}
};
/**
* Defines the Scheduled script trigger point.
* @param {Object} scriptContext
* @param {string} scriptContext.type - Script execution context. Use values from the scriptContext.InvocationType enum.
* @since 2015.2
*/
const download = (connection, fileId, SFTPDownloadDirectory) => {
let filelist = connection.list({
path: SFTPDownloadDirectory,
});
for (let i = 0; i < filelist.length; i++) {
let fileName = filelist[i].name;
if (fileName != "." && fileName != "..") {
log.debug("file name to download", fileName);
let downloadedFile = connection
.download({
filename: SFTPDownloadDirectory + "/" + fileName,
})
.getContents();
log.debug("file contents", downloadedFile);
}
}
};
const createTestFile = () => {
var fileObj = file.create({
name: "test.txt",
fileType: file.Type.PLAINTEXT,
contents: "Hello World\nHello World, This is a test file",
});
fileObj.folder = netsuiteUploadFolderId;
var fileId = fileObj.save();
return fileId;
};
const createSFTPconnection = () => {
const connectionObj = sftp.createConnection({
// establish connection to the FTP server
username: userName,
passwordGuid: passwordGUID,
url: sftpURL,
directory: SFTPDirectory,
hostKey: hostKey,
});
return connectionObj;
};
/**
* Defines the Scheduled script trigger point.
* @param {Object} scriptContext
* @param {string} scriptContext.type - Script execution context. Use values from the scriptContext.InvocationType enum.
* @since 2015.2
*/
const execute = (scriptContext) => {
let connectionObj = createSFTPconnection(); // create connection to SFTP server
let fileId = createTestFile(); // create a test file
upload(connectionObj, fileId, SFTPUploaddirectory);
download(connectionObj, fileId, SFTPUploaddirectory);
};
return { execute };
});
Execute is the main function, where we are calling upload and download functions.
Here is the script log
Subscribe to my newsletter
Read articles from Anurag Kumar directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
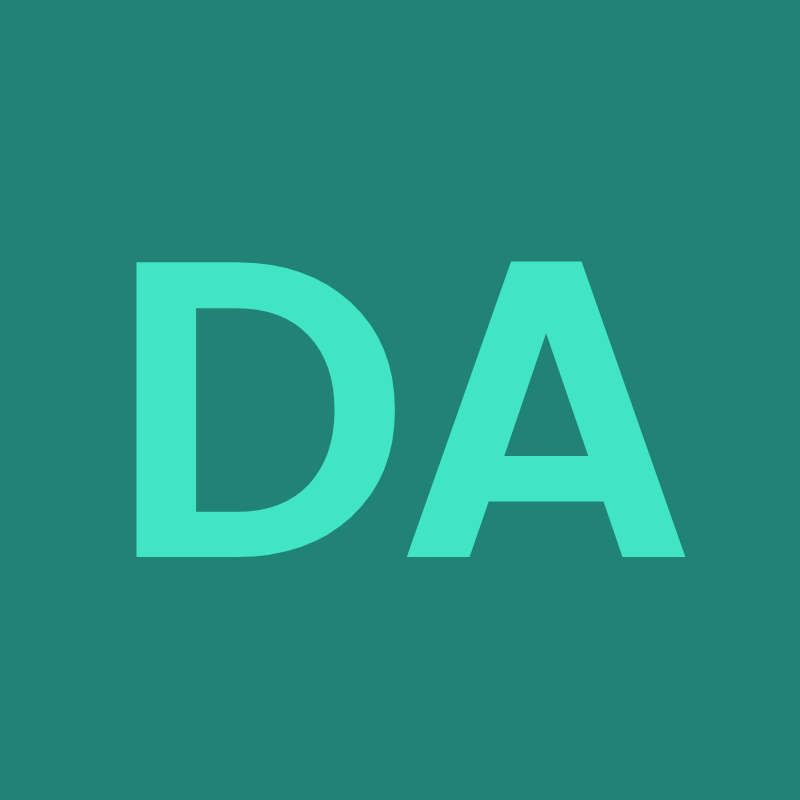
Anurag Kumar
Anurag Kumar
I am a NetSuite Certified SuiteCloud Developer with over 8 years of work experience.