Let's make something by make
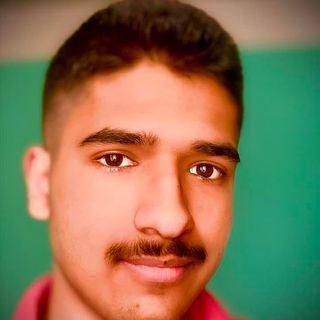
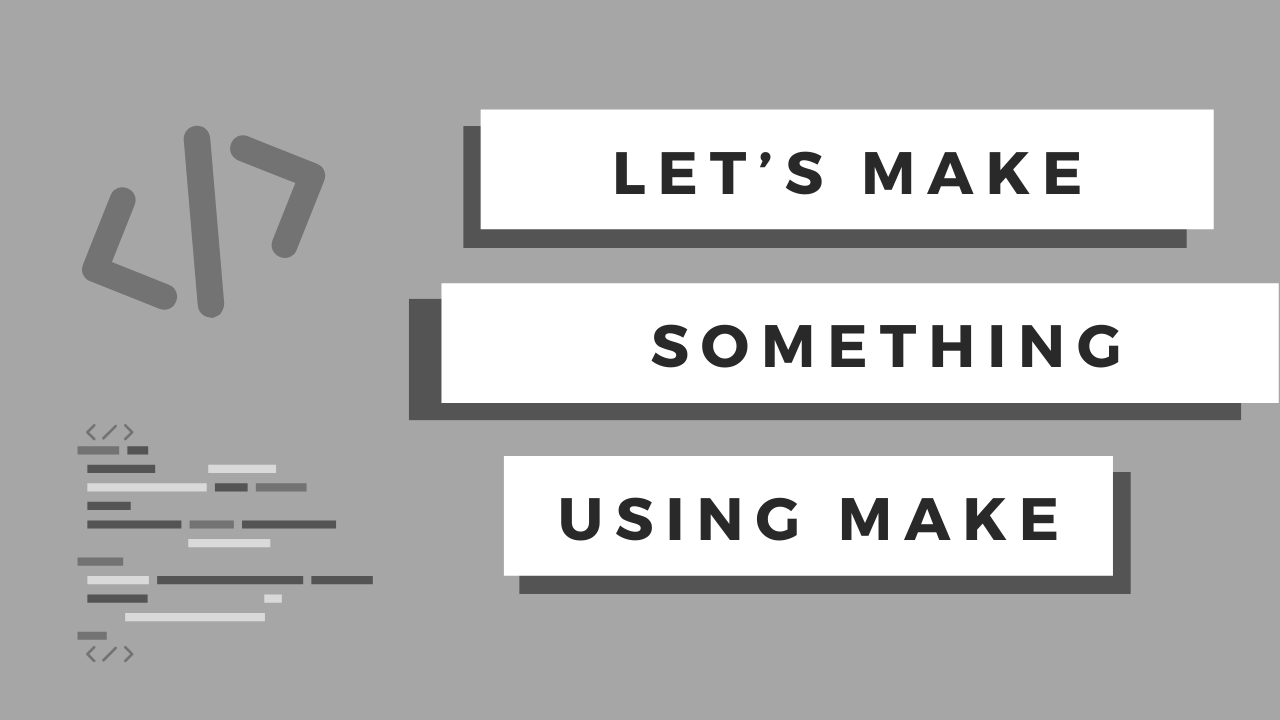
Hey there, fellow developer! Let's dive into the wonderful world of make
and how it can save us from the headaches of manual compilation. If you've ever found yourself drowning in a sea of source files, make
is here to throw you a lifebuoy.
What's the Deal with make
?
Alright, so make
is like your personal assistant for building software. It's a nifty tool commonly used in Unix-like systems that automates the build process based on rules defined in a Makefile
. Think of it as your code-building genie—rub the Makefile
lamp, and voila! Your project is magically compiled.
Getting to Know make
Lingo
Before we jump into coding, let's understand a few key terms:
Targets: These are the end products we want
make
to create—like our shiny executable program.Dependencies: Files that our targets depend on. If a dependency changes,
make
knows it's time to rebuild.Rules: Instructions that tell
make
how to create targets using commands.
The Marvels of make
Here's why make
is the superhero of build automation:
1. Efficiency Booster
make
is like a ninja—it only rebuilds what's necessary. If you tweak one source file, make
intelligently rebuilds only the affected parts, saving you precious time and preventing unnecessary recompilation.
2. Dependency Management Wizardry
With make
, managing dependencies is a breeze. If you update a header file, make
knows exactly which source files need to be recompiled. It's like having a mind-reading assistant who knows what you need before you do!
3. Cross-Platform Compatibility
Need to build your project on different systems? No problemo! make
plays nice with Unix, Linux, macOS, and even Windows (thanks to tools like MinGW). It's the Swiss Army knife of build tools.
Example Project Structure
Consider a project directory with the following files:
project/
│
├── main.c
├── utils.c
├── utils.h
├── math.c
├── math.h
└── Makefile
Visualizing Dependencies with a Graph
Let's map out the dependencies of our project with a cool graph. Imagine a web of interconnected files where changes in one file can trigger a cascade of rebuilds. Behold, the mighty dependency graph of make
:
+---------+
| main.o |
+----+----+
|
+------+------+
| |
v v
+----------+ +----------+
| utils.o | | math.o |
+-----+----+ +-----+----+
| |
v v
+----------+ +----------+
| utils.h | | math.h |
+----------+ +----------+
In this graph:
main.o
depends onmain.c
,utils.o
, andmath.o
.utils.o
depends onutils.c
andutils.h
.math.o
depends onmath.c
andmath.h
.
Crafting the Makefile
Masterpiece
Let's write a Makefile
that will do the heavy lifting for us:
# Define compiler and flags
CC = gcc
CFLAGS = -Wall -g
# Define our target executable
TARGET = myprogram
# List of all source files
SRCS = main.c utils.c math.c
# Generate a list of corresponding object files
OBJS = $(SRCS:.c=.o)
# Default target
all: $(TARGET)
# Rule to link all object files into the executable
$(TARGET): $(OBJS)
$(CC) $(CFLAGS) $^ -o $@
# Rule to compile each source file into its own object file
%.o: %.c
$(CC) $(CFLAGS) -c $< -o $@
# Clean rule to remove generated files
clean:
rm -f $(TARGET) $(OBJS)
Let's Get Silly with make
Now, the fun part—using make
to compile our project!
To Compile: Open your terminal, navigate to the directory with your
Makefile
, and type:make
Watch in amazement as
make
compiles each source file into its own object file and then magically links them together intomyprogram
. ✨To Clean Up: Feeling the need to tidy up? No worries! Just run:
make clean
This will sweep away the generated executable and object files like a digital broomstick.
Another massive makefile examples
Here's another comprehensive example of a Makefile
for a C project that consists of multiple directories, header files, and dependencies. This Makefile
will demonstrate how to organize and build a more complex project using make
.
Project Structure:
project/
│
├── src/
│ ├── main.c
│ ├── utils.c
│ ├── math/
│ │ ├── math.c
│ │ └── math.h
│ └── lib/
│ ├── lib.c
│ └── lib.h
│
├── include/
│ ├── utils.h
│ └── common.h
│
├── bin/
│
└── Makefile
Makefile
Example:
# Compiler and flags
CC = gcc
CFLAGS = -Wall -Wextra -Iinclude
# Directories
SRCDIR = src
INCDIR = include
BINDIR = bin
LIBDIR = $(SRCDIR)/lib
MATHDIR = $(SRCDIR)/math
# Source files
SRCS = $(wildcard $(SRCDIR)/*.c) \
$(wildcard $(LIBDIR)/*.c) \
$(wildcard $(MATHDIR)/*.c)
# Object files
OBJS = $(SRCS:%.c=%.o)
OBJDIR = $(BINDIR)/obj
OBJS_WITH_DIR = $(addprefix $(OBJDIR)/, $(notdir $(OBJS)))
# Executable name
TARGET = $(BINDIR)/myprogram
# Default target
all: directories $(TARGET)
# Create directories
directories:
@mkdir -p $(BINDIR) $(OBJDIR)
# Rule to link object files into executable
$(TARGET): $(OBJS_WITH_DIR)
$(CC) $(CFLAGS) $^ -o $@
# Rule to compile each source file into object files
$(OBJDIR)/%.o: $(SRCDIR)/%.c
$(CC) $(CFLAGS) -c $< -o $@
$(OBJDIR)/%.o: $(LIBDIR)/%.c
$(CC) $(CFLAGS) -c $< -o $@
$(OBJDIR)/%.o: $(MATHDIR)/%.c
$(CC) $(CFLAGS) -c $< -o $@
# Clean rule to remove generated files
clean:
rm -rf $(BINDIR)
# PHONY targets
.PHONY: all clean
Explanation:
CC
: Compiler command (gcc
).CFLAGS
: Compiler flags (-Wall -Wextra -Iinclude
includes theinclude
directory for header files).SRCDIR
: Directory containing source files.INCDIR
: Directory containing header files.BINDIR
: Directory to store the compiled binary.LIBDIR
: Directory containing library source files.MATHDIR
: Directory containing math-related source files.SRCS
: List of all source files using wildcard expansion.OBJS
: List of corresponding object files derived from source files.OBJDIR
: Directory to store object files.OBJS_WITH_DIR
: List of object files with full path.TARGET
: Executable name with path.
Makefile
Features:
all
: Default target that depends on$(TARGET)
. It ensures directories are created before building the target.directories
: Rule to create necessary directories (bin
andbin/obj
).$(TARGET)
: Rule to link object files into the executable.$(OBJDIR)/%.o
: Rule to compile each source file into object files and store them in the object directory.clean
: Rule to remove thebin
directory (cleaning up generated files).
Usage:
To Compile: Run
make
in the directory containingMakefile
:make
This will compile all source files into object files and then link them into
bin/myprogram
.To Clean Up: To remove the generated
bin
directory and all its contents, run:make clean
Notes:
The
$(wildcard ...)
function is used to automatically discover source files within specified directories.Dependency directories (
bin
andbin/obj
) are created using thedirectories
rule.Object files are stored in
bin/obj
to keep the project directory clean.Customize
CFLAGS
,SRCDIR
,INCDIR
, etc., based on your project's structure and requirements.
This Makefile
example demonstrates a more complex project setup with multiple directories and source files. Feel free to modify and expand upon it to suit your specific project needs!
Further Reading and References
Expand your make
mastery with these helpful resources:
GNU
make
Manual: The official manual covering all aspects ofmake
.Tutorialspoint -
make
Tutorial: A beginner-friendly tutorial onmake
andMakefile
.Dr. Heckendorns
make
Primer: Dr Heckendorns's work onmake
primer.
Conclusion
And there you have it—make
to the rescue! With make
, you can conquer complex build processes and spend more time coding and less time pulling your hair out over manual compilation.
So go ahead, give make
a spin, and let it work its magic on your projects. Your future self will thank you for automating the mundane tasks, leaving you more time for the important stuff—like contemplating the meaning of life or perfecting your rubber duck debugging skills.
Happy coding and may your Makefile
always be error-free!
Subscribe to my newsletter
Read articles from Dristanta Silwal directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
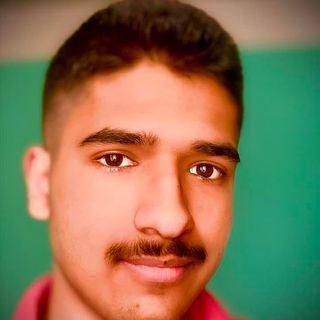
Dristanta Silwal
Dristanta Silwal
Bio: 🖥️ Computer Science Student | ✍️ Passionate Blogger 💡 Exploring the Intersection of Tech and Creativity 🎓 Currently pursuing a degree in Computer Science, I am a curious and driven student with a deep passion for all things technology. I am constantly seeking new avenues to expand my knowledge and skills in this ever-evolving field. 📚 As an aspiring computer professional, I am immersed in the world of programming languages, algorithms, and software development. However, my true excitement lies in blending my technical expertise with my creative spirit. ✏️ I have recently embarked on an exciting journey as a blogger, where I channel my love for writing to explore the fascinating world of technology, digital trends, and innovative ideas. Through my blog, I aim to share valuable insights, tutorials, and thought-provoking content that inspires others to embrace the wonders of the digital age. 🌐 When I'm not busy coding or crafting blog posts, you can find me tinkering with gadgets, experimenting with new software, or exploring the latest tech innovations. I'm always on the lookout for fresh perspectives and innovative ideas to incorporate into my work. 🤝 Let's connect and explore the limitless possibilities of the tech realm together! Feel free to reach out if you have any questions, collaboration opportunities, or just want to geek out over the latest trends. Let's shape the future of technology one blog post at a time! #ComputerScienceStudent #BloggingEnthusiast #TechGeek #CodeAndCreativity