Setting Up Virtual Environments in Python
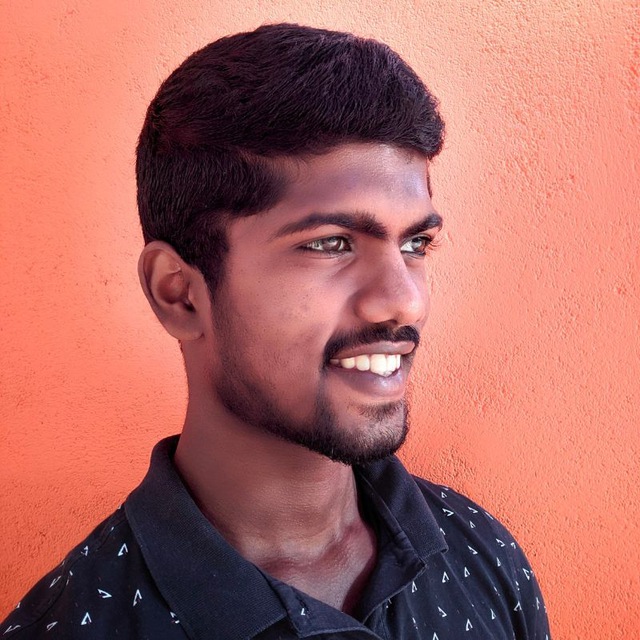

Introduction
In the world of Python development, managing dependencies and keeping your project environments organized can be a challenge, especially when you're working on multiple projects with different package requirements. This is where virtual environments come into play.
What is virtual environment in python?
A virtual environment is a self-contained directory that isolates an installation of Python and its dependencies from other projects on your system.
Think of a virtual environment as a separate workspace or sandbox for each of your Python projects. By creating a virtual environment, you can ensure that the packages and libraries you install for one project don't interfere with those of another project. This not only helps in avoiding conflicts but also makes it easier to manage and maintain your projects' dependencies.
Why virtual environment?
Using virtual environments is considered a best practice in Python development, and it's recommended to create a new virtual environment for each project you work on. This way, you can easily maintain different versions of packages for different projects without running into compatibility issues.
Whether you're a seasoned Python developer or just starting out, understanding and using virtual environments will help you streamline your development process, maintain a clean and organized codebase, and save you from potential headaches caused by conflicting dependencies.
Let's dive in and learn how to set up and utilize virtual environments effectively!
Creating a Virtual Environment
Python 3 comes with the venv
module, which provides a convenient way to create virtual environments without the need for a separate package like virtualenv
.
To create a new virtual environment, follow these steps:
Open your terminal or command prompt and navigate to the directory where you want to create the virtual environment.
Run the following command:
python -m venv /path/to/new/virtual/environment
Replace
/path/to/new/virtual/environment
with the desired location and name for your virtual environment. For example, if you want to create a virtual environment named.venv
in the current directory, the command would be:python -m venv .venv
This will create a new directory
.venv
containing the isolated Python environment.โNote that in case of Unix/macOS usepython3
instead ofpython
.After running the command, you should see a new directory created at the specified location, containing files and directories required for the virtual environment.
The structure of the virtual environment directory will look something like this:
.venv/ Include/ Lib/ Scripts/ pyvenv.cfg
The
Scripts
orbin
(in Unix/macOS) directory contains the executable files for activating the virtual environment and running Python scripts within it. TheLib
directory is where the Python packages and libraries will be installed.
Activating the Virtual Environment
After creating a new virtual environment using the python -m venv
command, the next step is to activate it. An activated virtual environment ensures that any Python packages you install or any scripts you run are isolated within that environment, preventing conflicts with packages installed globally or in other virtual environments.
To activate the virtual environment, follow these steps:
On Windows:
Navigate to the directory where you created the virtual environment using the command prompt or PowerShell.
Run the following command:
\path\to\env\Scripts\activate
Replace
\path\to\env
with the actual path to your virtual environment. For example, if your virtual environment is located in current directory with the name.venv
, the command would be:.venv\Scripts\activate
On Unix or macOS:
Navigate to the directory where you created the virtual environment using the terminal.
Run the following command:
source /path/to/env/bin/activate
Again, replace
/path/to/env
with the actual path to your virtual environment. For example, if your virtual environment is located inprojects/.venv
, the command would be:source projects/.venv/bin/activate
After running the appropriate command, you should see your command prompt prefixed with the name of the activated virtual environment, indicating that you're working within that isolated environment.
(.venv) $
Now, any Python packages you install using pip
or any scripts you run will be confined to this virtual environment, without affecting your system's global Python installation or other virtual environments you may have set up.
Working with the Virtual Environment
After creating and activating a virtual environment, you can start running Python scripts and commands within its isolated environment. This ensures that any packages or dependencies you install or use are confined to the virtual environment, preventing conflicts with other projects or your system's global Python installation.
Here are some common tasks you might perform while working with an activated virtual environment:
Running Python Scripts: To run a Python script within the virtual environment, simply execute the script as you normally would, using the
python
command. For example:python script.py
The script will run using the Python interpreter and packages installed within the virtual environment.
Running Python Interactive Shell: You can start the Python interactive shell (also known as the REPL) within the virtual environment by running the
python
command without any arguments:python
This will open the Python shell, allowing you to execute Python commands and test code interactively using the packages installed in the virtual environment.
Running Jupyter Notebook: If you use Jupyter Notebook for your Python development, you can start the notebook server from within the virtual environment:
jupyter notebook
This will ensure that the Jupyter Notebook kernel runs with the packages installed in the virtual environment.
Installing Packages: While working within the virtual environment, you can install packages using
pip
as needed :pip install new_package
The new package and its dependencies will be installed within the virtual environment. Any packages you install within the activated virtual environment will be isolated from other environments and your system's global Python installation. This means that you can safely install and use different versions of packages for different projects without worrying about conflicts or compatibility issues.
Deactivating the Virtual Environment: When you're done working with the virtual environment, you can deactivate it to return to your system's default Python environment:
deactivate
After deactivating the virtual environment, you'll see that your command prompt or terminal is no longer prefixed with the virtual environment's name.
This practice promotes a clean and organized development workflow, making it easier to manage and maintain your projects over time.
Best Practices
While virtual environments provide a powerful way to manage dependencies and keep your Python projects organized, there are some best practices you should follow to get the most out of them:
Create a New Virtual Environment for Each Project: It's recommended to create a separate virtual environment for each project you work on. This helps isolate dependencies and prevent conflicts between different projects that may require different package versions or have conflicting requirements.
Use a Version Control System: Always use a version control system (like Git) to track changes in your project's code and dependencies. This allows you to easily revert to a previous state if needed and collaborate with others effectively.
Keep Requirements Files Up-to-Date: Whenever you install or update packages within a virtual environment, make sure to update the corresponding
requirements.txt
file. This ensures that you can easily recreate the same environment on another machine or share it with your team.Use a Virtual Environment Management Tool: While the built-in
venv
module is great for creating and managing virtual environments, you might find it more convenient to use a dedicated tool likepipenv
orpoetry
. These tools provide additional features and a more streamlined workflow for managing virtual environments and dependencies.
Additional Tools
Here are some popular tools that can help you manage virtual environments and dependencies more effectively:
virtualenv:
virtualenv
is a popular third-party tool that supports both older and newer Python versions. Withvirtualenv
, you can create a sandboxed environment for your Python projects.pipenv:
pipenv
is a popular tool that combines the functionality ofpip
andvirtualenv
. It automatically creates and manages virtual environments for your projects and provides a more user-friendly interface for installing and managing packages.poetry:
poetry
is a modern package management tool for Python that focuses on simplicity and reproducibility. It handles virtual environments, dependency resolution, and package publishing in a seamless and efficient manner.conda:
conda
is a package and environment management system that comes with Anaconda, a popular Python distribution for scientific computing and data science. It provides a convenient way to create and manage virtual environments, as well as install packages from different channels.tox:
tox
is a tool primarily used for automated testing in Python projects, but it also provides a convenient way to create and manage virtual environments for different Python versions and environments (e.g., development, production).
These tools offer additional features and conveniences over the standard venv
module, but they all serve the same fundamental purpose: helping you manage dependencies and virtual environments for your Python projects more effectively.
By following these best practices and exploring additional tools, you can ensure that your Python development workflow remains organized, reproducible, and free from dependency conflicts.
Potential pitfalls and Gotchas
There are a few potential pitfalls and gotchas to be aware of when working with virtual environments in Python. Here are some common ones:
Path Issues: When you activate a virtual environment, the Python executable and scripts within that environment are added to your system's PATH. However, if you have multiple virtual environments with the same package installed, the order of the PATH can determine which version of the package is used. This can lead to unexpected behavior or conflicts. To avoid this, always be aware of the currently activated virtual environment and its PATH.
Virtualenv in Virtualenv: It's generally not recommended to create a virtual environment within another virtual environment. This can lead to complex dependency issues and make it harder to manage and reason about your environments. Instead, create separate virtual environments side-by-side if needed.
Forgetting to Activate the Virtual Environment: It's easy to forget to activate the virtual environment, especially if you're working on multiple projects simultaneously. This can lead to packages being installed in the wrong environment or conflicts with your system's global Python installation. Always double-check that you're in the correct virtual environment before installing or running packages.
Removing or Deleting Virtual Environments: Be careful when removing or deleting virtual environments, as this action is irreversible. If you accidentally delete a virtual environment, you'll need to recreate it and reinstall all the dependencies. It's a good practice to back up your virtual environments or use version control to track changes.
Namespace Packages: Namespace packages, which are packages that span multiple directories or distributions, can sometimes cause issues when used within virtual environments. If you encounter problems with namespace packages, you may need to investigate and address them on a case-by-case basis.
Virtual Environment Bloat: Over time, as you install and uninstall packages in a virtual environment, it can become bloated with cached files and unused dependencies. This can increase the size of the virtual environment unnecessarily. Periodically, you may want to clean up or recreate your virtual environments to keep them lean and efficient.
While these potential pitfalls can cause some hiccups, being aware of them and following best practices can help you avoid or mitigate most issues when working with virtual environments in Python.
Conclusion
In the world of Python development, managing dependencies and maintaining a clean and organized codebase can be a daunting task, especially when working on multiple projects with varying package requirements. This is where virtual environments come into play, providing a powerful solution to isolate project dependencies and prevent conflicts.
Throughout this blog post, we've covered the essential steps for setting up and utilizing virtual environments in Python:
Creating a new virtual environment for your project.
Activating the virtual environment.
Working with the virtual environment, including running Python scripts, Jupyter Notebooks, installing packages and deactivating virtual environment.
Following best practices, such as creating a new virtual environment for each project and using version control systems
Exploring additional tools like
virtualenv
,pipenv
,poetry
,conda
, andtox
for more advanced virtual environment management
By embracing virtual environments, you can ensure that your projects remain organized, maintainable, and free from package conflicts. Each project can have its own isolated environment with the necessary dependencies, without interfering with other projects or your system's global Python installation.
Furthermore, using requirements files and following best practices like version control and consistent environment management across your team, you can promote reproducibility and make it easier to collaborate on projects or share your work with others.
So, what are you waiting for? Embrace the power of virtual environments and take your Python development to new heights of organization and efficiency!
โIf you found this article helpful, why not spread the knowledge? Share it with fellow developers, and letโs create a community of Python enthusiasts! ๐๐โ
Subscribe to my newsletter
Read articles from Prasanna Kumar Shetty directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
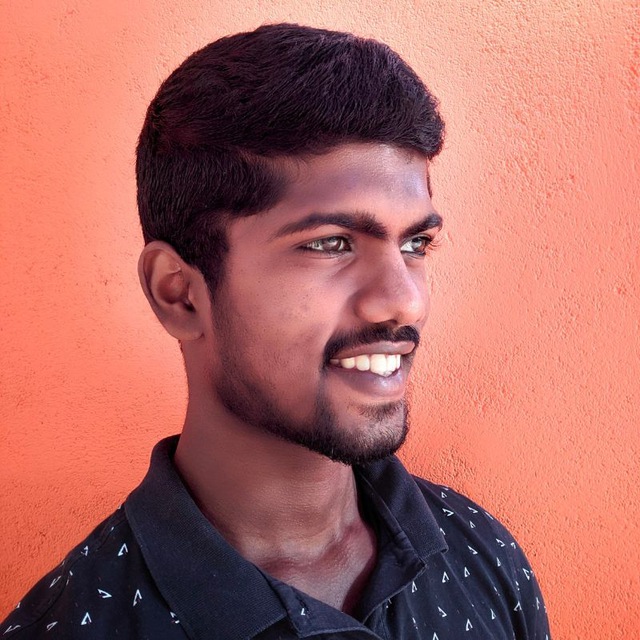