Topic: 10 Understanding the Widgets In Flutter

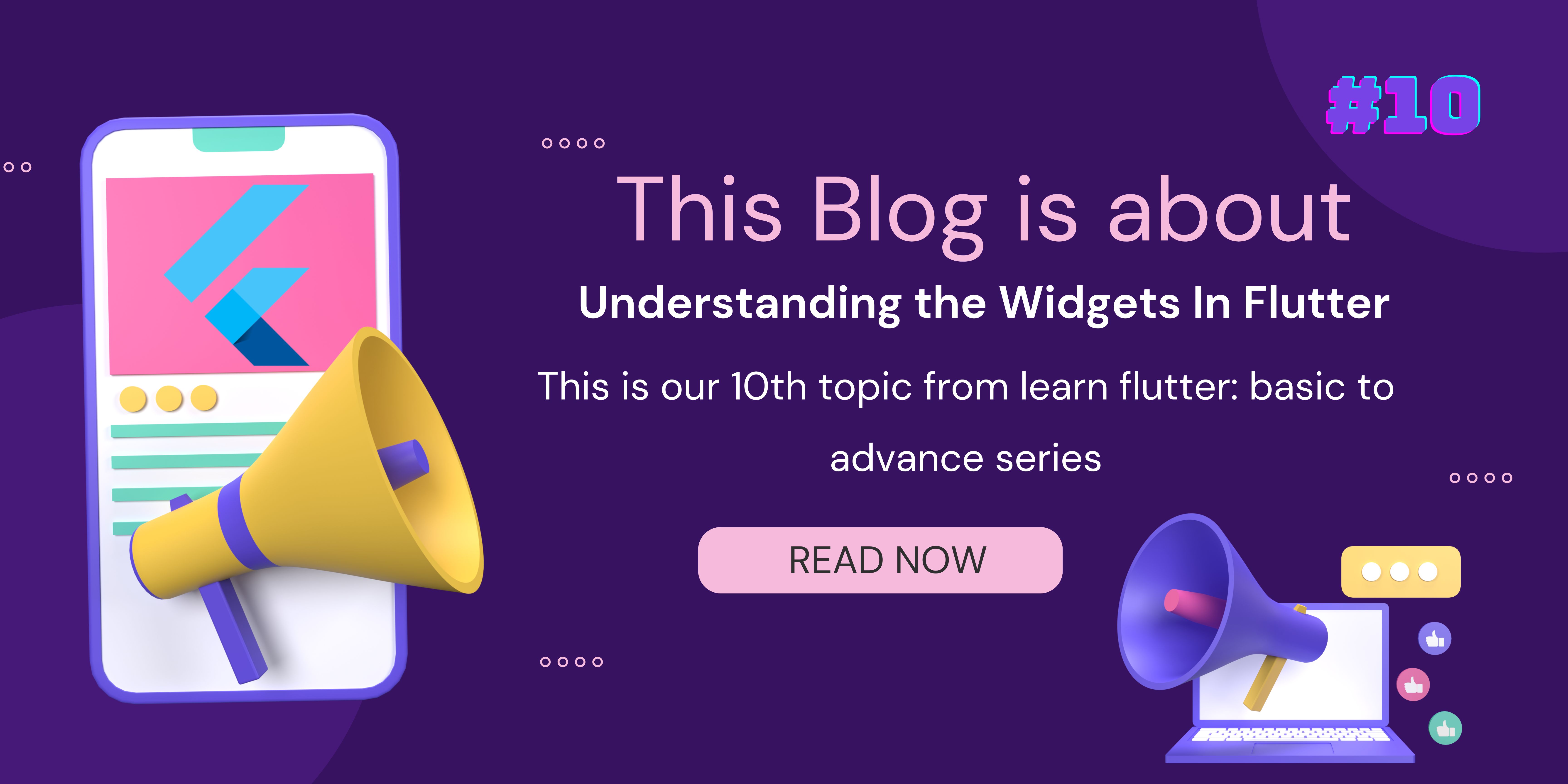
Hello devs, In this blog post, we took a deep dive into exploring the wide range of widgets that Flutter offers. These widgets are like magic tools that help us create beautiful and user-friendly interfaces for our apps. By getting to know each widget's unique features and uses, we can use them smartly to create captivating UI designs that enhance how users experience our apps.
Container:
- A versatile widget for containing other widgets with customizable styling.
Container(
color: Colors.blue,
padding: EdgeInsets.all(16),
margin: EdgeInsets.symmetric(vertical: 20),
alignment: Alignment.center,
child: Text('Hello, Flutter!', style: TextStyle(color: Colors.white)),
)
Row:
- Arrange its children horizontally.
Row(
mainAxisAlignment: MainAxisAlignment.spaceEvenly,
children: [
Icon(Icons.star),
Icon(Icons.star),
Icon(Icons.star),
],
)
Column:
- Arrange its children vertically.
Column(
mainAxisAlignment: MainAxisAlignment.spaceEvenly,
children: [
Text('Option 1'),
Text('Option 2'),
Text('Option 3'),
],
)
Stack:
- Stacks its children on top of each other.
Stack(
children: [
Container(color: Colors.red),
Container(color: Colors.green),
Container(color: Colors.blue),
],
)
ListView:
- Displays a scrollable list of children.
ListView(
children: [
ListTile(title: Text('Item 1')),
ListTile(title: Text('Item 2')),
ListTile(title: Text('Item 3')),
],
)
GridView:
- Displays a scrollable grid of children.
GridView.count(
crossAxisCount: 2,
children: [
Container(color: Colors.red),
Container(color: Colors.green),
Container(color: Colors.blue),
],
)
Flexible:
- Expands to fill available space within its parent.
Row(
children: [
Flexible(child: Container(color: Colors.red)),
Flexible(child: Container(color: Colors.green)),
],
)
Expanded:
- Expands to fill available space along the main axis of its parent.
Row(
children: [
Expanded(child: Container(color: Colors.red)),
Expanded(child: Container(color: Colors.green)),
],
)
Text:
- Displays styled text.
Text('Hello, Flutter!', style: TextStyle(fontSize: 20, fontWeight: FontWeight.bold))
TextField:
- Accepts user input as text.
TextField(
decoration: InputDecoration(
labelText: 'Enter your name',
border: OutlineInputBorder(),
),
)
Checkbox:
- A checkable box for binary choices.
dartCopy codeCheckbox(
value: isChecked,
onChanged: (newValue) {
setState(() {
isChecked = newValue;
});
},
)
RadioButton:
- Allows the user to select one option from a group.
Column(
children: [
Radio(value: 1, groupValue: radioValue, onChanged: (value) => setState(() => radioValue = value)),
Radio(value: 2, groupValue: radioValue, onChanged: (value) => setState(() => radioValue = value)),
Radio(value: 3, groupValue: radioValue, onChanged: (value) => setState(() => radioValue = value)),
],
)
Switch:
- A toggleable switch widget.
Switch(
value: isSwitched,
onChanged: (newValue) {
setState(() {
isSwitched = newValue;
});
},
)
Slider:
- Allows the user to select a value from a range.
Slider(
value: sliderValue,
min: 0,
max: 100,
onChanged: (newValue) {
setState(() {
sliderValue = newValue;
});
},
)
GestureDetector:
- Detects various gestures on its child widget.
GestureDetector(
onTap: () {
// Handle tap
},
onLongPress: () {
// Handle long press
},
child: Container(
width: 100,
height: 100,
color: Colors.blue,
child: Center(child: Text('Tap me')),
),
)
AppBar:
- A material design app bar typically containing a title, actions, and optional leading and trailing widgets.
dartCopy codeAppBar(
title: Text('My App'),
actions: [
IconButton(icon: Icon(Icons.search), onPressed: () {}),
IconButton(icon: Icon(Icons.settings), onPressed: () {}),
],
)
Scaffold:
- Implements the basic material design visual layout structure, including an app bar, body, and bottom navigation.
Scaffold(
appBar: AppBar(title: Text('My App')),
body: Center(child: Text('Hello, Flutter!')),
)
SnackBar:
- Displays a brief message at the bottom of the screen.
ElevatedButton(
onPressed: () {
ScaffoldMessenger.of(context).showSnackBar(SnackBar(content: Text('This is a SnackBar')));
},
child: Text('Show SnackBar'),
)
BottomNavigationBar:
- A material design bottom navigation bar with tabs for switching between different views or routes.
BottomNavigationBar(
currentIndex: _currentIndex,
onTap: (index) {
setState(() {
_currentIndex = index;
});
},
items: [
BottomNavigationBarItem(icon: Icon(Icons.home), label: 'Home'),
BottomNavigationBarItem(icon: Icon(Icons.search), label: 'Search'),
BottomNavigationBarItem(icon: Icon(Icons.person), label: 'Profile'),
],
)
CupertinoNavigationBar:
- A navigation bar with iOS-style transition animations.
CupertinoNavigationBar(
middle: Text('Title'),
trailing: CupertinoButton(
child: Icon(Icons.add),
onPressed: () {},
),
)
CupertinoButton:
- A button with an iOS-style appearance.
CupertinoButton(
child: Text('Press me'),
onPressed: () {},
color: CupertinoColors.activeBlue,
)
CupertinoTextField:
- A text field with an iOS-style appearance.
CupertinoTextField(
placeholder: 'Enter your name',
onChanged: (value) {
print('Input changed: $value');
},
)
AnimatedContainer:
- Animates changes to its properties such as size, color, and alignment.
AnimatedContainer(
duration: Duration(seconds: 1),
width: _isExpanded ? 200 : 100,
height: _isExpanded ? 200 : 100,
color: _isExpanded ? Colors.blue : Colors.red,
child: Text('Animated Container'),
)
AnimatedOpacity:
- Animates changes to the opacity of its child widget.
AnimatedOpacity(
opacity: _isVisible ? 1.0 : 0.0,
duration: Duration(milliseconds: 500),
child: Container(
width: 200,
height: 200,
color: Colors.blue,
),
)
Hero:
- Enables a widget to animate between different routes or screens.
Hero(
tag: 'imageHero',
child: Image.network('https://example.com/image.jpg'),
)
ClipRRect:
- Clips its child widget to a rounded rectangle.
ClipRRect(
borderRadius: BorderRadius.circular(10),
child: Image.network('https://example.com/image.jpg'),
)
Opacity:
- Changes the opacity of its child widget.
Opacity(
opacity: 0.5,
child: Container(
width: 100,
height: 100,
color: Colors.blue,
),
)
Placeholder:
- A widget that draws a box of a specified size is typically used as a placeholder for future content.
Placeholder(
color: Colors.grey,
strokeWidth: 2.0,
fallbackHeight: 100,
fallbackWidth: 100,
)
Divider:
- A horizontal line is used to separate content.
Divider(
color: Colors.grey,
thickness: 2.0,
height: 20,
)
ContainerTransform:
- An animated container transformation widget that transitions between two shapes.
ContainerTransform(
transform: _selected ? Matrix4.rotationZ(pi) : Matrix4.identity(),
child: Container(
width: 100,
height: 100,
color: Colors.blue,
),
)
Alright devs, This is the conclusion of our blog. I trust that this content has provided you with a clear understanding of Widgets In Flutter. Moving forward, our next topic will dive into Canvas in Flutter. Stay tuned for more insightful discussions and engaging content on Flutter development. Thank you for reading!
Connect with Me:
Hey there! If you enjoyed reading this blog and found it informative, why not connect with me on LinkedIn? ๐ You can also follow my Instagram page for more mobile development-related content. ๐ฒ๐จโ๐ป Letโs stay connected, share knowledge and have some fun in the exciting world of app development! ๐
Subscribe to my newsletter
Read articles from Mayursinh Parmar directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by

Mayursinh Parmar
Mayursinh Parmar
๐ฑMobile App Developer | Android & Flutter ๐๐ก Passionate about creating intuitive and engaging apps ๐ญโจ Letโs shape the future of mobile technology!