Introduction to Solidity
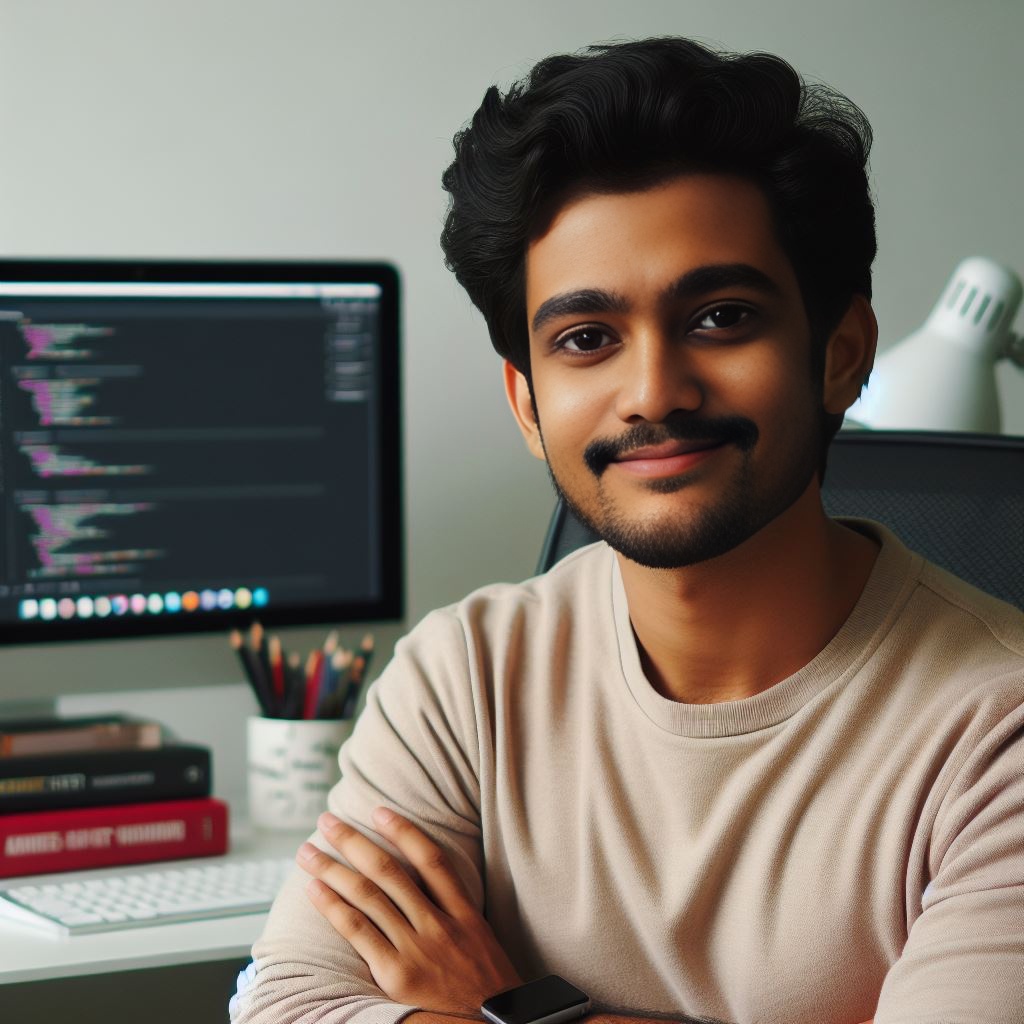
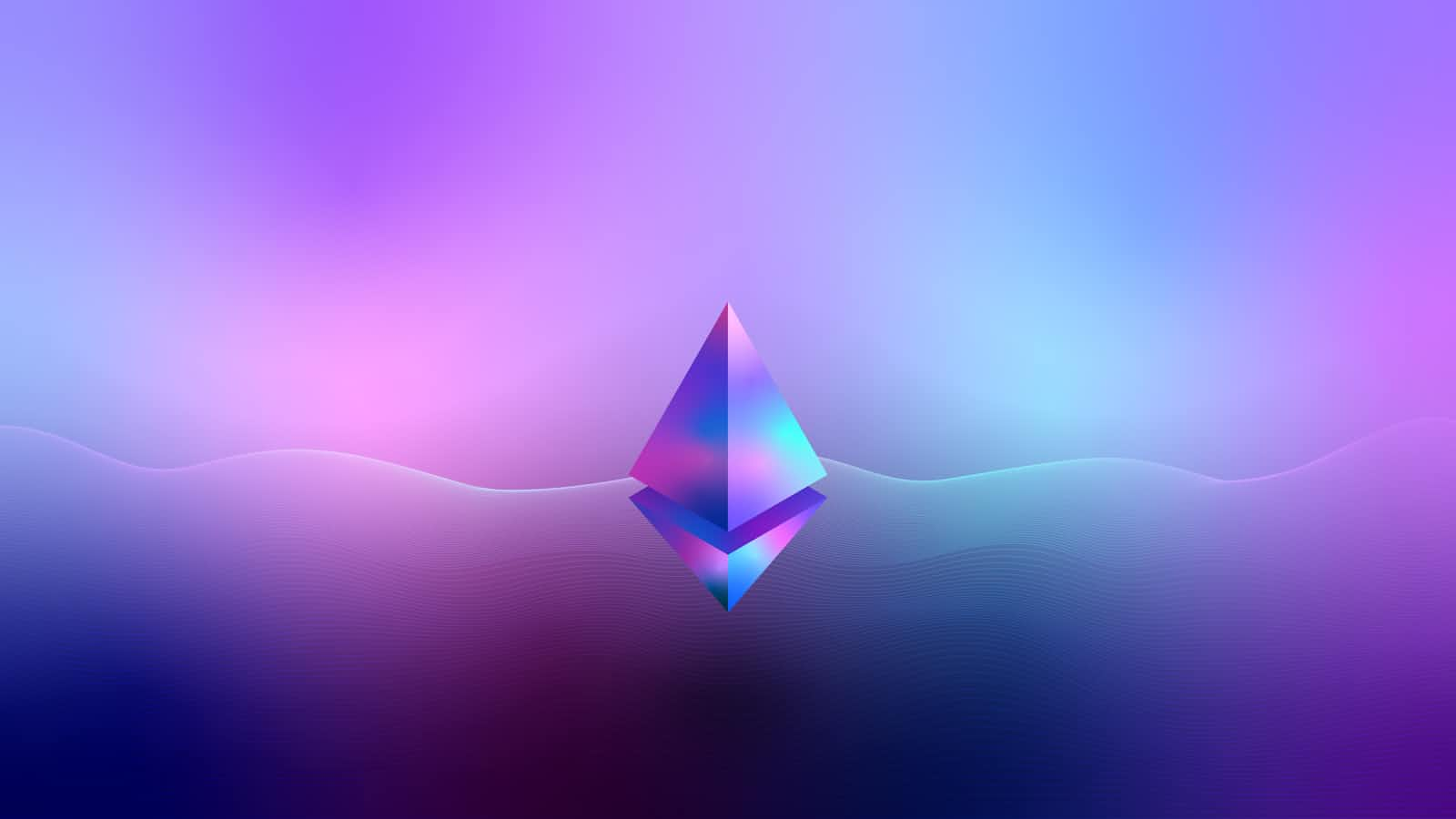
Why was Solidity language created?
This is a very common question that might come to your mind, so we are going to address it before diving into the syntax of Solidity. There are already many general-purpose languages like JavaScript, C#, Java, C++, etc., which are Turing complete and can do almost everything. However, they often require many steps to solve even small problems, making simple solutions quite complex. That's why we came up with a new language specifically designed for blockchain, with features tailored for building decentralized applications (dApps).
How Solidity executes?
well well as of our current knowledge is limited and it maybe difficult to understand to follow this thing if you havent ever coded before so i will try to keep it very very simple!
Writing the Code: People write special programs called smart contracts using a language called Solidity. These contracts are like rules that run on the Ethereum system.
Turning into Bytecode: The Solidity code is transformed into a kind of computer language called bytecode. This bytecode is what the Ethereum system understands.
Putting it on Ethereum: The bytecode is uploaded or deployed onto the Ethereum network. It becomes a smart contract that lives on the Ethereum blockchain.
code -> compile -> bytecode(platform independent) -> execution in EVM
Doing Actions: When someone wants to use the smart contract (like sending money or triggering a function), they send a request or transaction.
Processing the Transaction: Miners on the Ethereum network receive and process these transactions. They follow the instructions in the bytecode of the smart contract.
Changing State: Depending on the transaction, the Ethereum system updates its records (like account balances or contract variables) according to what the smart contract specifies.
Paying Fees: Every action on Ethereum costs some fees called gas. The person sending the transaction pays these fees to the miners.
Getting Results: Once the transaction is processed and confirmed by the network, the results are stored on the blockchain. The transaction is complete!
Your first solidity smart contract : Hello world
okay okay i know have given you enough of such teoretical boring information about ethereum and soldity, now its time to write some code.
Step 1 : Go to the https://remix.ethereum.org its an online integrated development environment which is very easy to write solidity smart contracts.
Step 2 : You will se bunch of files and folders in your left side if the screen as shown in the below image.
Now go ahead and delete each one of them one by one.
Step 3 : Create a HelloWorld.sol file and paste the following code.
// SPDX-License-Identifier: MIT
pragma solidity ^0.8.0;
contract HelloWorld {
string public greet = "helloWorld";
}
Now follow the below video to compile deploy and run the code
Understanding Syntax line by line
- SPDX Liscence identifier : The line
// SPDX-License-Identifier: MIT
at the top of a Solidity file is a special comment that tells us about the license that applies to the smart contract's code.
//
: In programming,//
means the rest of the line is a comment, which is just for humans to read and doesn't affect how the code runs.SPDX-License-Identifier: MIT
: This part is a code tag that identifies the type of license being used. "MIT" refers to a specific type of open-source license called the MIT License.When you see
// SPDX-License-Identifier: MIT
in a Solidity file, it means that the smart contract's code can be freely used, modified, and shared by anyone under the rules of the MIT License. This license allows for a lot of freedom in using the code while still requiring credit to be given to the original authors.
Pragma : In Solidity,
pragma
is a directive used to specify compiler settings, such as the compiler version to be used for compiling the contract. Thepragma
statement is placed at the beginning of a Solidity source file.Now, let's break down the Solidity code line by line :
contract HelloWorld {
: This line starts the definition of a new smart contract calledHelloWorld
. A smart contract is like a small program that can be deployed on the Ethereum blockchain.string public greet = "helloWorld";
: This line declares a variable namedgreet
inside theHelloWorld
contract.string
: This tells us thatgreet
is a type of data that holds text (a string of characters).public
: This means that anyone can see the value ofgreet
but cannot change it directly. It creates a special function called a getter that allows others to read the value ofgreet
.greet
: This is the name of the variable we are declaring.=
: This is the assignment operator, which sets the initial value ofgreet
."helloWorld"
: This is the initial value assigned togreet
, which is a piece of text (a string) that says "helloWorld".
So, when you put it all together, this Solidity code defines a smart contract named HelloWorld
that has a public variable called greet
containing the text "helloWorld"
. The greet
variable can be read by anyone, allowing them to see the greeting message stored in the contract.
This contract demonstrates a simple use of a state variable (greet
) in Solidity, which holds a fixed greeting message that can be accessed publicly on the blockchain.
Woohoo! Great Job Buddy!!!
If you've made it this far, be proud of yourself! Keep working hard Mate!
See you in my next blog. Let's Fucking go!
Subscribe to my newsletter
Read articles from Yash Gupta directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
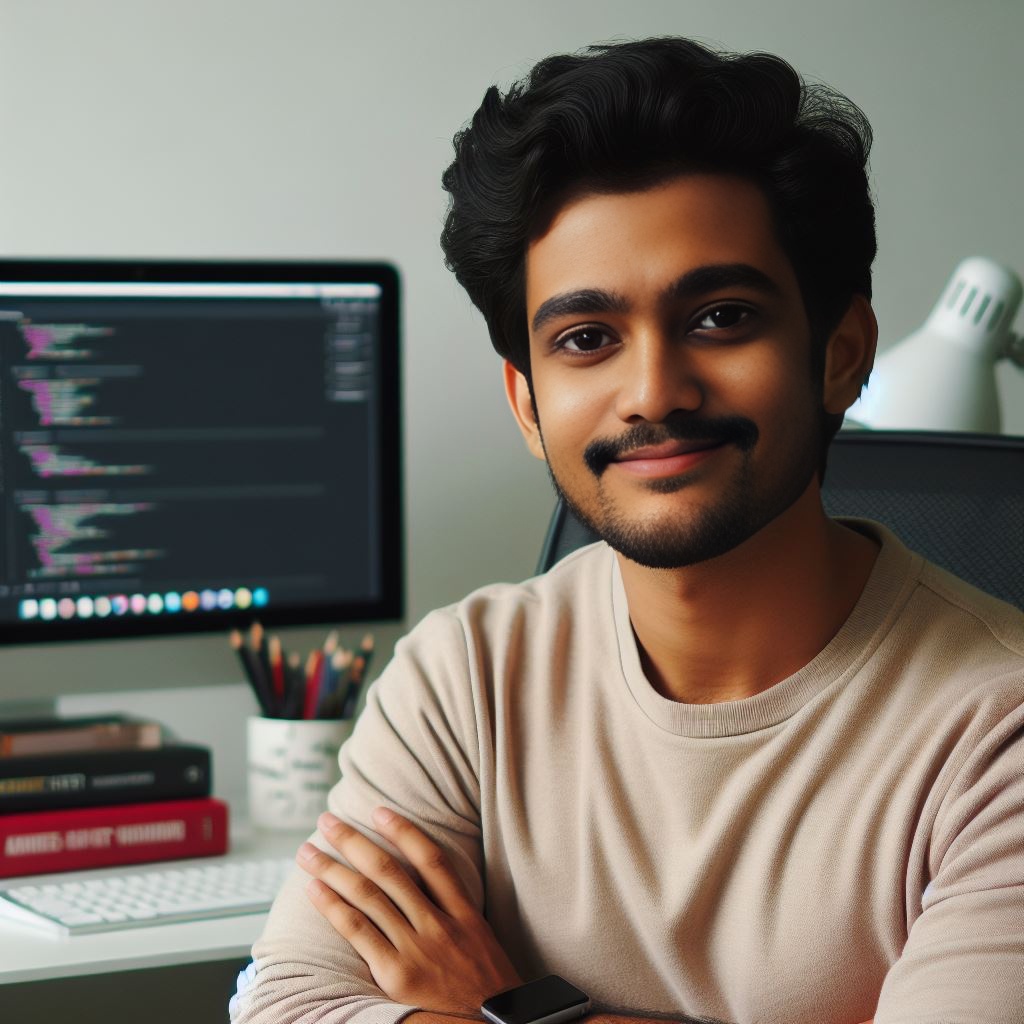
Yash Gupta
Yash Gupta
I’m an Open-Source enthusiast & a junior pursuing my Bachelors in Electronics & Communication Engineering. I am passionate about DevOps, BlockChain, Web Dev & I enjoy learning new things. My intention is to do my best and continue honing my skills while also learning new ones. My goal is to build a community so as a technical writer I am creating simple useful user documentation on various technologies and helping newbie students at DevRelDAO, and as developer, I want to build & innovate impactful projects.