Producing Invoice Reports with the Blazor Server Application
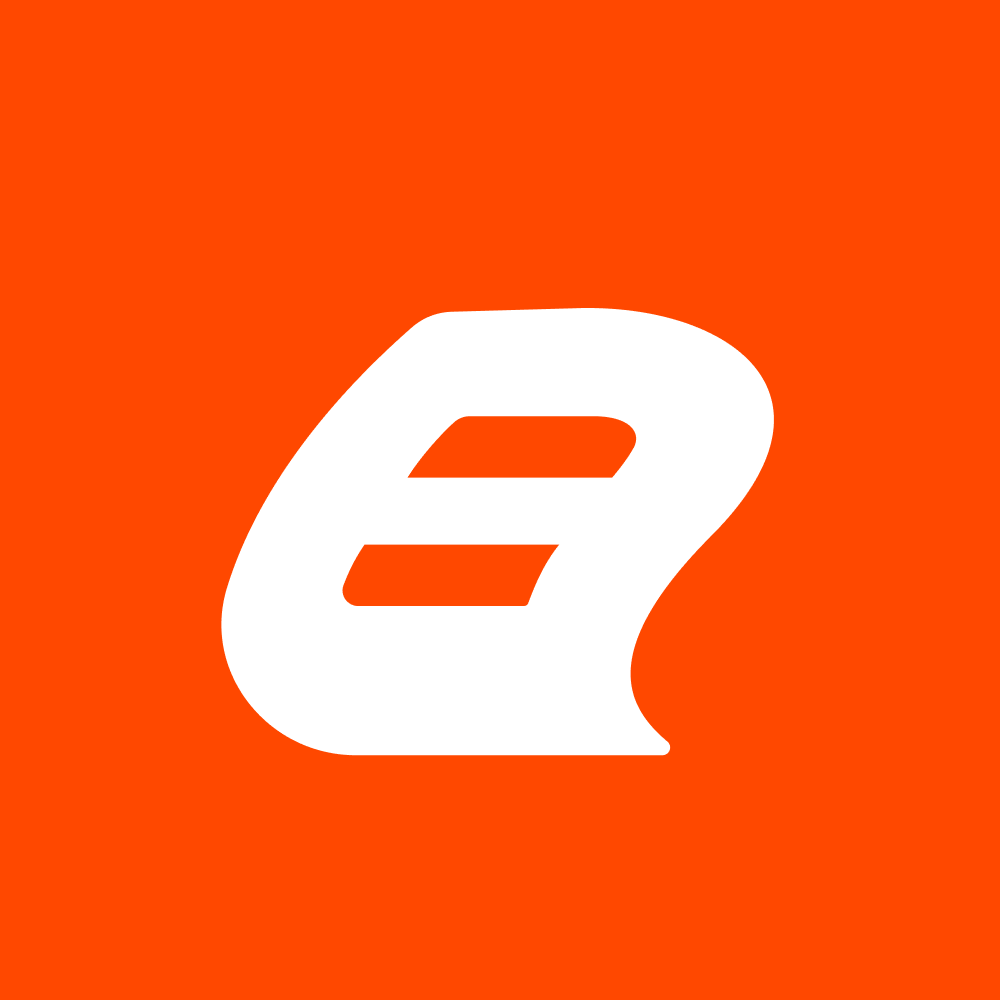
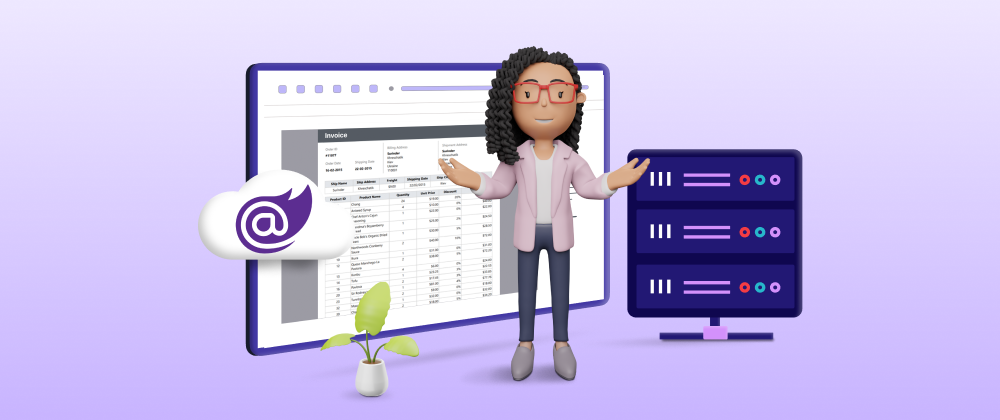
Generating invoice reports is an important task in accounting and finance departments, yet it often involves navigating complex coding processes. In this blog post, we’ll explore a streamlined method for generating invoice reports as PDFs without the need to view them. We’ll use the report’s invoice ID and the Bold Reports Report Writer within a Blazor Server application.
With the Report Writer, you can easily generate reports in PDF, Word, Excel, and CSV formats without viewing them. Before we dive into the specifics, it’s helpful to check out our previous blog post, where we discussed creating invoice reports with the Bold Report Designer, to get a better understanding of Bold Reports in invoice reporting.
Create a New Blazor Server Application
I will be using Visual Studio Code to generate an invoice report as a PDF.
Prerequisites
Before getting started, ensure your development environment includes the following:
.NET SDK installed on your machine.
Visual Studio Code to add the code.
Create a Blazor Server application
Run the following command prompt to create a Blazor Server application by the name of BlazorInvoiceGenerator.
dotnet new blazorserver --name BlazorInvoiceGenerator
The new Blazor Server application will be created.
Install NuGet packages
In this section, we will install NuGet packages in the application to add the necessary dependencies. To install the NuGet packages:
Change the current working directory to the application root folder.
cd BlazorInvoiceGenerator
Add the BoldReports.Net.Core packages to the application by running the following command.
dotnet add package BoldReports.Net.Core
The NuGet packages contain all the dependency packages to add the Report Writer to the application.
Setting up resources for RDL reports
- Create a folder named Resources in the wwwroot folder in this application. This is where the RDL reports will be kept.
Adding the invoice-report.rdl
2. In this setup, add the invoice-report.rdl file to the Resources folder.
Add an API method to generate the expected document in the controller
Create a new file named BoldReportWriterController.cs in the Data folder of your Blazor Server project.
Inside this file, add the required directives for ASP.NET Core MVC and input-output. Make sure to add the Bold Reports Writer namespace to make it directly accessible in the Controller file.
using Microsoft.AspNetCore.Mvc; using System.IO; using BoldReports.Writer;
Add the namespace and class to the controller file for structuring functionality and organizing the code to avoid naming conflicts.
Next, set the Route attribute for the BoldReportWriterController. This attribute helps in setting up URLs that match the pattern needed to link requests with specific controller actions.
namespace BlazorInvoiceGenerator.Data { [Route("api/{controller}/{action}/{id?}")] public class BoldReportWriterController: ControllerBase { } }
To read the report file from the application, use the IHostingEnvironment interface by importing the AspNetCore.Hosting namespace and injecting IHostingEnvironment through dependency injection.
private Microsoft.AspNetCore.Hosting.IWebHostEnvironment _hostingEnvironment; public BoldReportWriterController(Microsoft.AspNetCore.Hosting.IWebHostEnvironment hostingEnvironment) { _hostingEnvironment = hostingEnvironment; }
Next, add the Export method with the HttpGet action to export the invoice associated using its invoice ID.
[HttpGet] public IActionResult Export(string invoiceID) { }
In the Export method, we are going to read the report file as a stream and use it with the Report Writer:
1. Initialize the report path with the RDL report location.
2. Read the report template in the application using FileStream and the report path.
3. Create a memory stream to copy the report template content and use it with the Report Writer.
string reportPath = Path.Combine(_hostingEnvironment.WebRootPath, "Resources/invoice-report.rdl");
FileStream fileStream = new FileStream(reportPath, FileMode.Open, FileAccess.Read);
MemoryStream reportStream = new MemoryStream();
fileStream.CopyTo(reportStream);
reportStream.Position = 0;
fileStream.Close();
Note This copy process will be helpful to avoid access problems in the server while running the application on it.
4. Create a new instance of the Bold Reports Report Writer.
ReportWriter writer = new ReportWriter();
5. Load the report in the Report Writer.
writer.LoadReport(reportStream);
6. We need to generate the report based on the invoice ID number. So, create a parameter list for the report and assign the InvoiceID parameter value to the report using the set parameters.
List<BoldReports.Web.ReportParameter> userParameter = new List<BoldReports.Web.ReportParameter>();
//Add the desired parameters
userParameter.Add(new BoldReports.Web.ReportParameter()
{
Name = "InvoiceID",
Values = new List<string>() { invoiceID }
});
writer.SetParameters(userParameter);
Note: The Name value should be the same as the parameter name in the report.
7. Generate the PDF using the Save option by specifying the PDF format.
MemoryStream memoryStream = new MemoryStream();
writer.Save(memoryStream, WriterFormat.PDF);
//Download the generated export document to the client side.
memoryStream.Position = 0;
Note: The generated PDF report will be in the stream, which will be provided for the FileResultStream to download by the client. After downloading, the FileResultStream will read the stream again for the process, so the stream position is set back to ‘0’ after the Report Writer’s save action.
Next, we need to return the generated file with FileStreamResult. For that, create the FileStreamResult with the exported report’s memory stream value to get the exported document on the client.
FileStreamResult fileStreamResult = new FileStreamResult(memoryStream, "application/pdf");
fileStreamResult.FileDownloadName = "invoice-report.pdf";
return fileStreamResult;
Register a valid license key
By default, the report generated using the Report Writer will show a license message. To remove this message, we need to register either an online license token or an offline license key.
Click here to learn how to register an online license token. Click here to learn how to register an offline license key.
Enabling controller routing
Invoke the app.MapController function to enable routing to controller action methods, allowing incoming HTTP requests to be mapped to the appropriate actions based on convention or attributes.
app.MapControllers();
Implementation of API on the client side
On the client side, add an option to enter the invoice ID and a Generate button to retrieve documents based on the invoice ID.
Open the Host.cshtml file from the Pages folder and add the necessary scripts.
<script src="https://cdn.boldreports.com/external/jquery-1.10.2.min.js" type="text/javascript"></script> <script> </script>
Add the downloadFile method to get the format and set the browser’s location to the API endpoint API/BoldReportWriter/Export with a query parameter specifying the invoice.
function downloadFile() { var invoiceID = ""; invoiceID = $('#invoiceID').val(); location.href = 'api/BoldReportWriter/Export?invoiceID=' + invoiceID; }
Note This action triggers the browser to download the PDF file with the specific invoice ID from the Export API added in the BoldReportWriterController file.
Open the Index.razor file from the Pages folder.
Add the code to inject JSRuntime and invoke this JavaScript interop in the Index.razor file.
@page "/" @using Microsoft.JSInterop @using Microsoft.AspNetCore.Components @inject IJSRuntime JSRuntime
Next, add the following code snippet in the application. This code will create an interface that allows users to enter the invoice ID and a Generate button that will download the PDF file when it’s clicked.
<div class="Common"> <div class="tablediv"> <div class="rowdiv"> <label id="design"> Provide an Invoice ID to export the report with the specific Invoice ID. <br /> <br /> </label> </div> <div class="rowdiv"> <div class="celldiv" style="padding:10px"> <label> <strong> Invoice ID :</strong> </label> <input id="invoiceID" type="text" name="invoiceID" style="margin-left: 15px" /> <br /> <br /> <input class="buttonStyle" type="submit" name="button" value="Generate" style="width:150px;" @onclick="PDFReport"/> </div> </div> </div> </div>
Next, add the PDFReport This snippet of code will asynchronously trigger the client-side JavaScript function downloadFile, enabling users to start downloading the file.
@code { public async void PDFReport() { await JSRuntime.InvokeVoidAsync("downloadFile"); } }
Build and run the application.
Now, everything is ready to load the Report Writer. Build and run the application. You will see the text box to enter the invoice ID and the Generate button. When you provide the invoice ID and click the Generate button, the PDF file will be generated.
Report Exported in PDF Format
When opening the downloaded file, you will see the exported invoice for the provided invoice ID.
Generated PDF Report Using Invoice ID
Export in different formats
If you want the report in Word format, you can use with the followig code in the IActionResult export method. You will see the report is exported.
...
writer.Save(memoryStream, WriterFormat.Word);
...
FileStreamResult fileStreamResult = new FileStreamResult(memoryStream, "application/docx");
fileStreamResult.FileDownloadName = "invoice-report.docx";
...
When opening the downloaded file, you will see the exported invoice.
Generated Word Report Using Invoice ID
Conclusion
I hope this blog provided sufficient guidance for producing invoice reports in a Blazor Server application. Bold Reports offers a comprehensive guide for exporting RDL files and linked reports in your Blazor Server application. To learn more about the Blazor reporting tools in the Bold Reports Report Designer, look through our documentation. To experience the features live, please check out our demo samples and solutions.
Bold Reports offers a 15-day free trial with no credit card information required. We welcome you to start a free trial and experience Bold Reports. Try it, and let us know what you think!
Subscribe to my newsletter
Read articles from Bold Reports Team directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
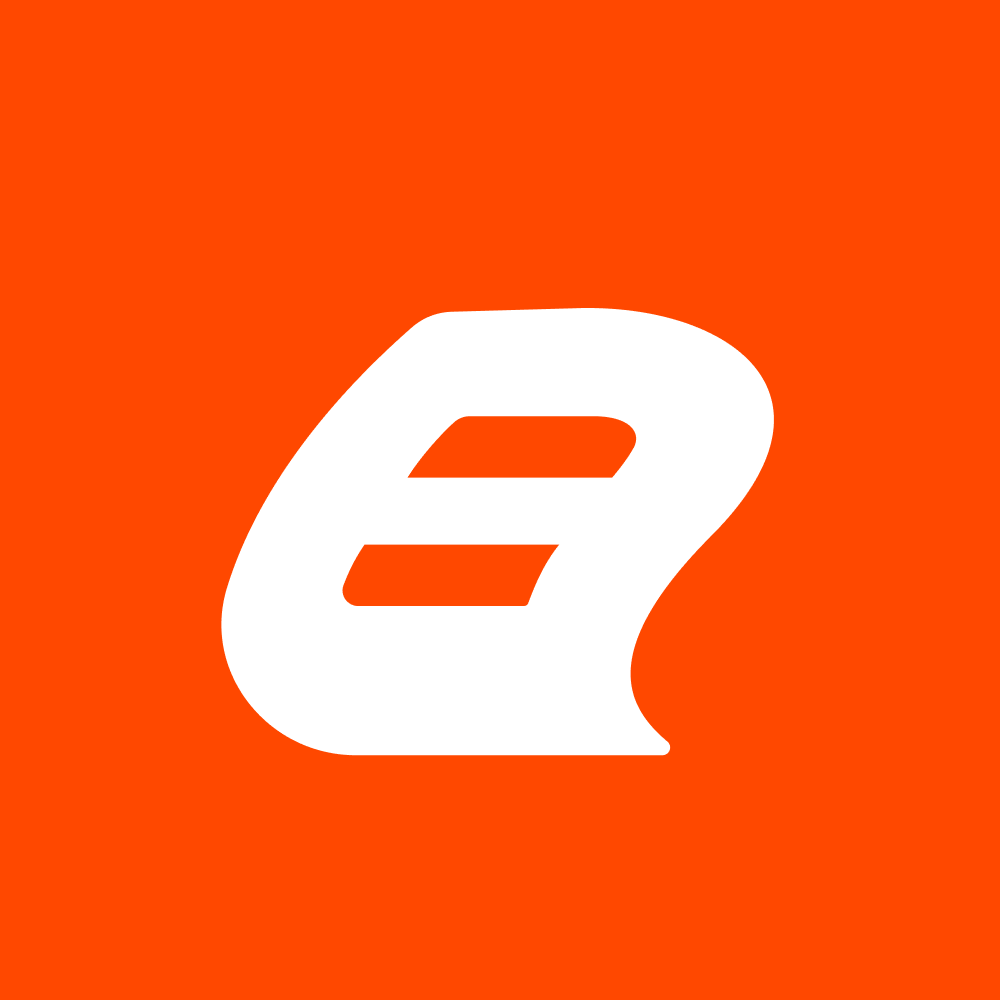