What is Static Typing
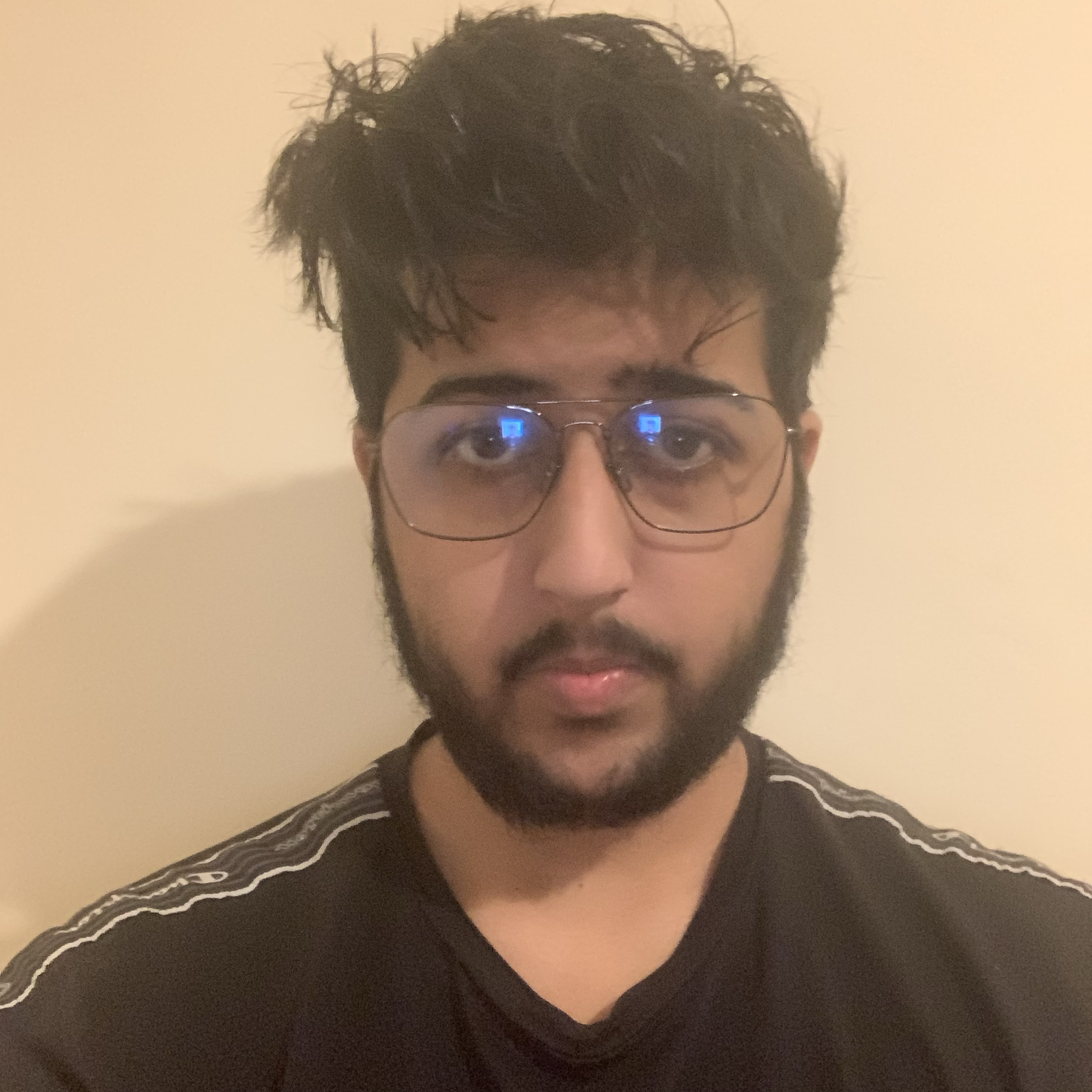
Table of contents
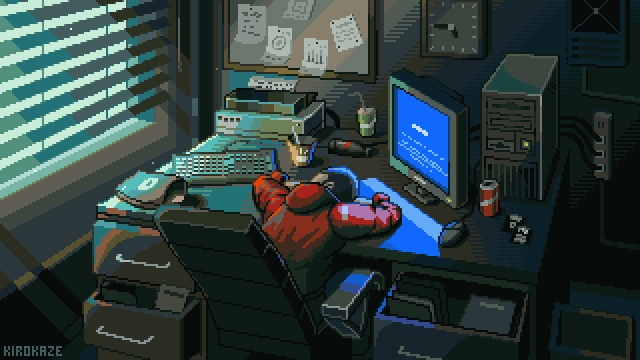
Introduction
In this article I will be talking about what is static typing and will be showing you examples of how it is used.
Static Typing
Static typing is a secure typing system where we define data types so for example if a variable is going to contain an integer value then it will be defined with the int
data type depending on what language your using. Having static typing allows you to detect errors in your code during development which you can then fix and it makes your application more secure from malicious attacks.
Type Safety Example
Here is a function in JavaScript which adds two numbers:
function add(a, b) {
return a + b;
}
console.log(add(5, 10)); // Output: 15
console.log(add("5", "10")); // Output: "510"
// (concatenation instead of addition)
In JavaScript the add function doesn't specify the types for its variables and because of this we can pass non integer values like strings to the function and it will concatenate the string values in the output.
In a statically typed language like Typescript, the same function will look like this:
function add(a: number, b: number):
number { return a + b; }
console.log(add(5, 10)); // Output: 15
console.log(add("5", "10")); // Output Error: Argument of type '"5"' is not
// assignable to parameter of type 'number'.
Here as you can see in TypeScript we can define our variables with the data type number
to specify what type of value the function can accept, in this case integers. Now if we attempt to pass strings to the function, you will see that we get an error message Argument of type '"5"' is not assignable to parameter of type 'number'
. This is happening because we have defined our variables to only accept integer values by assigning the number
data type. Hopefully now you see how having type security makes your program more robust and less prone to malicious attacks.
References
https://swalih.hashnode.dev/for-those-javascript-people-who-havent-tried-typescript
Subscribe to my newsletter
Read articles from Noor Ahmed directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
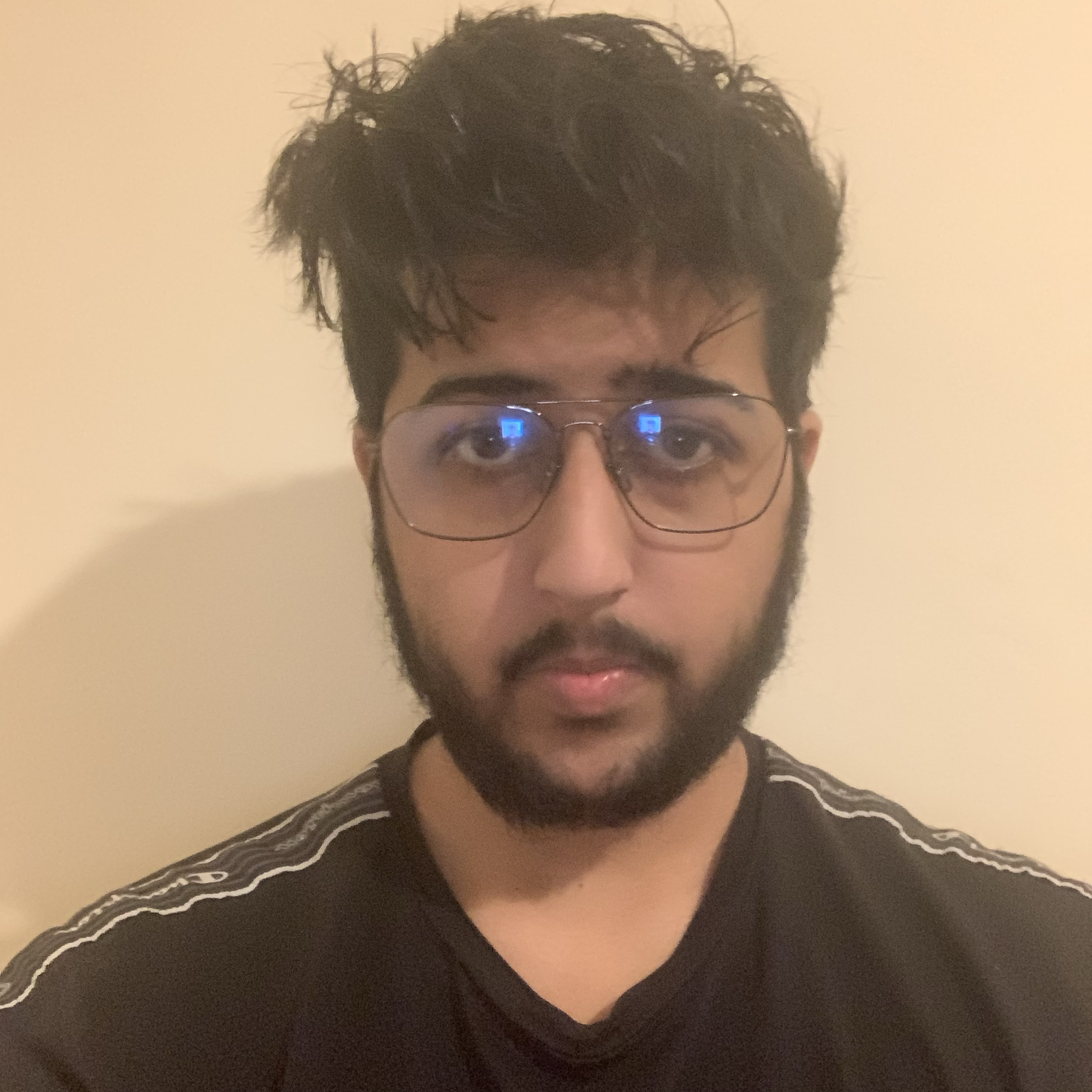
Noor Ahmed
Noor Ahmed
I am a software engineer intern from the UK and I have worked with multiple technologies like MERN stack, JavaScript, Python etc. I also like to contribute to open source on Github.