Linked List creation In "Python"

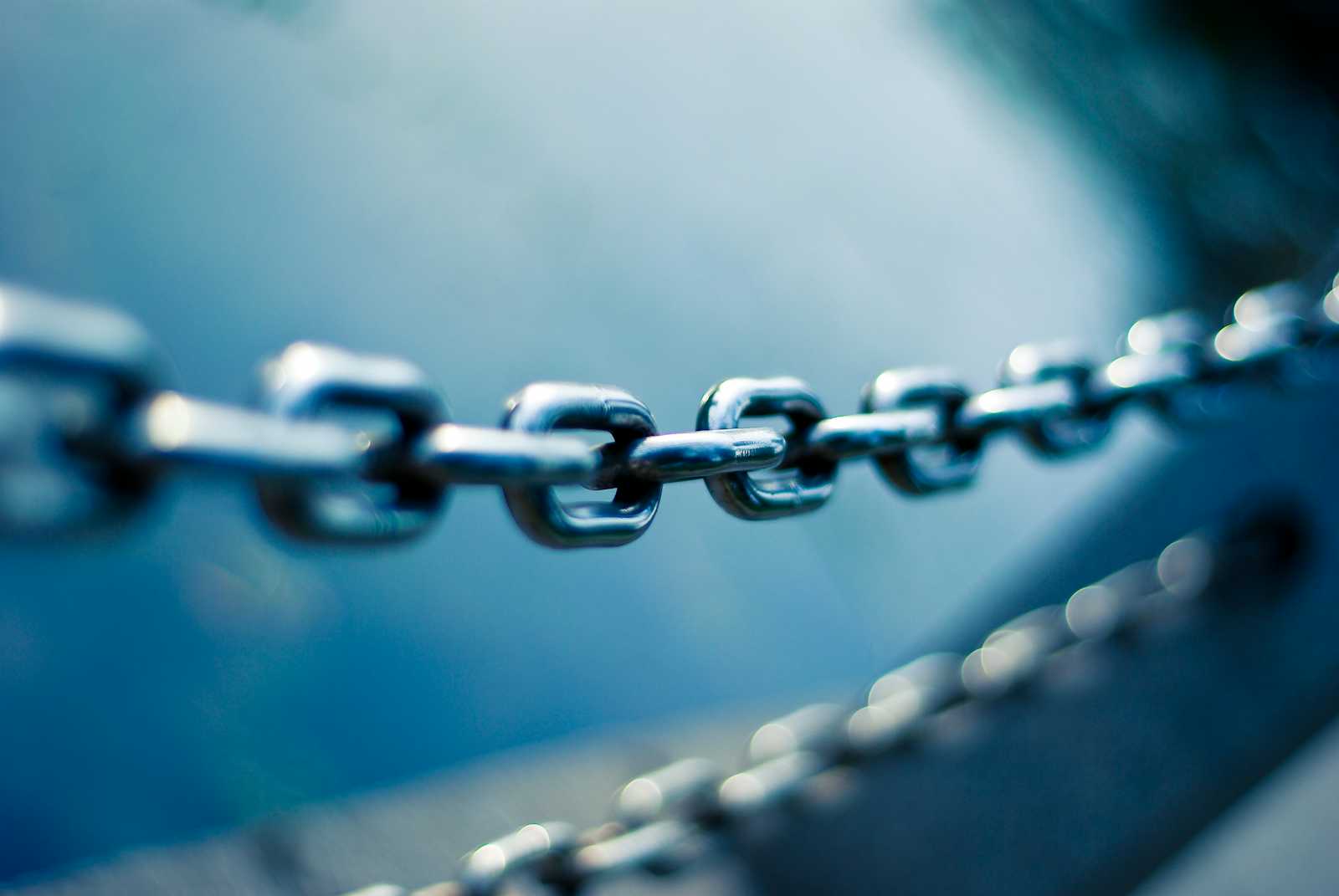
We can create linked list easily in 2 possible ways
Using direct object linking
Using insert method
Direct object linking
Linkedlist node is the combination of value as well as next node address
class LinkedList:
def __init__(self, value):
self.value = value
self.next = None
node1 = LinkedList(1) #1->None
node2 = LinkedList(2) #2->None
node3 = LinkedList(3) #3->None
node1.next = node2 #1->2->None
node2.next = node3 #1->2->3->None
current_node = node1 #pointing the current node with this variable
while current_node:
print(current_node.value,'->', end="")
if current_node.next is None:
print('None')
breaak
current_node = current_node.next
Output:
1->2->3->None
The above example explains
First we created each node individually
To make connection between the nodes we attached each node next attribute to the next node.
In the previous step only we created the linked list
To print the linkedlist in the console we have to traverse through the linkedlist
First assigned the first node to the current_node variable
After printing the current_node value we assigned the this variable to the next node
Using insert method
class LinkedList:
def __init__(self, value):
self.value = value
self.next = None
class LL:
def __init__(self, head):
self.head = None
def insert(self, value):
node = LinkedList(value)
if self.head is None:
self.head = node
return
current = self.head
while current:
print(current.value, '->', end="")
if current.next is None:
curren.next = node
break
current = current.next
def printLL(self):
current = self.head
while current:
print(current.value, '->', end="")
current = current.next
print('None')
LL_obj = LL()
LL_obj.insert(1)
LL_obj.print()
Output:
1->None
Here the creation a bit tricky
After immediately calling the class we are assigning out head value to the None
With the help of insert method weather we create out first linkedlist node or insert the value to the existing linkedlist.
If it is our first value we just create out first node will return from there
if we are trying to add some values to the existing linkedlist then first we will assign our head value to the currrent_node.
After we have to traverse through the entire linkedlist until we meet the condition where out current.next is None because this is where we have to add our node.
here I defined another method called printLL in order to print the linkedlist.
Subscribe to my newsletter
Read articles from Malavi Pande directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by

Malavi Pande
Malavi Pande
A young woman utterly captivated by the pursuit of knowledge.