TCA vs MVVM for SwiftUI
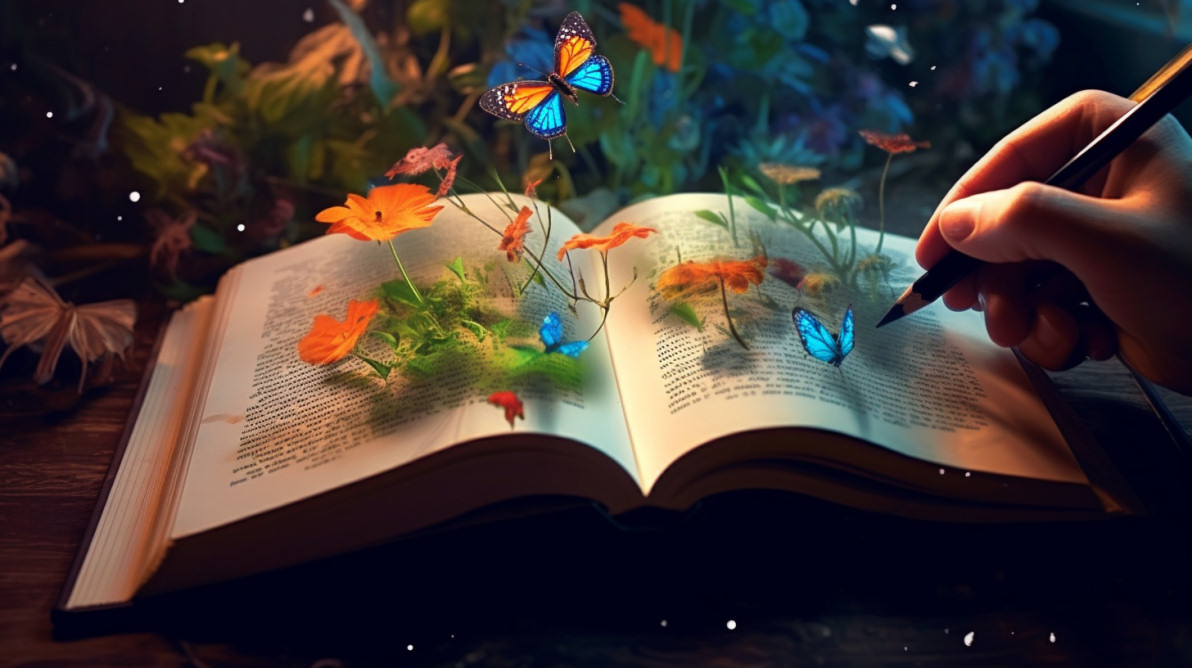
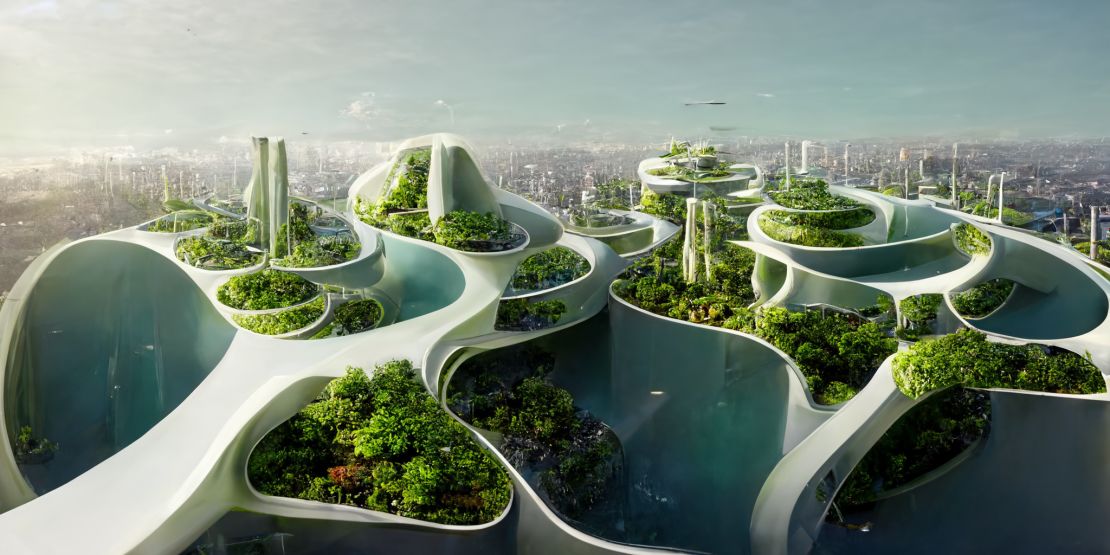
When it comes to app architecture and design patterns for SwiftUI, the two main approaches are The Composable Architecture (TCA) and the Model-View-ViewModel (MVVM) pattern. Both patterns have their own advantages and trade-offs, and the choice between them largely depends on your project’s requirements, team preferences, and the complexity of your app.
The Composable Architecture(TCA)
TCA is a unidirectional data flow architecture introduced by the Point-Free team. It’s heavily inspired by the Elm architecture and functional programming principles. The core components of TCA are:
State: Represents the entire state of your application or feature.
Action: An enum type that describes all the actions of a particular feature.
Reducers: Pure functions that handle state mutations based on specific actions.
Effects: Describe side effects (e.g., network requests, database operations) that need to be performed.
View: Responsible for rendering the UI and handling user interactions.
TCA promotes a strict unidirectional data flow, where the view can only trigger actions, and the state updates are handled by pure reducers. Effects are used to handle asynchronous operations and feed the results back into the system as new actions.
Advantages of TCA:
Enforces a strict unidirectional data flow, making it easier to reason about state changes.
Promotes functional programming principles, leading to more testable and maintainable code.
Separates concerns between state management, effects, and UI.
Drawbacks of TCA:
Potentially steeper learning curve, especially for developers new to functional programming concepts.
Can lead to boilerplate code for complex applications.
Limited tooling and community support compared to more established patterns.
Model-View-ViewModel (MVVM)
MVVM is a widely adopted architectural pattern that separates the application logic from the UI layer. In SwiftUI, the core components of MVVM are:
Model: Represents the data and business logic of the app.
View: Responsible for presenting the UI and handling user interactions.
ViewModel: Acts as an abstraction layer between the view and the model, exposing data and operations that the view can consume.
In MVVM, the view is bound to the ViewModel, which manages the state and provides transformation and validation logic. The ViewModel interacts with the model to fetch and update data, while the view only displays the data provided by the ViewModel.
Advantages of MVVM:
Widely adopted and well-understood pattern, with a large community and resources.
Promotes separation of concerns between UI, presentation logic, and data/business logic.
Facilitates testability by allowing unit testing of ViewModels independently.
Drawbacks of MVVM:
Can lead to tight coupling between the view and the ViewModel, making it harder to reuse components.
Potential for code duplication and boilerplate if not implemented carefully.
State management can become complex in larger applications.
In summary, both TCA and MVVM are viable options for SwiftUI app architecture. TCA is a more opinionated and functional approach, promoting unidirectional data flow and pure functions. MVVM is a more traditional and widely adopted pattern, separating concerns between UI, presentation logic, and data/business logic.
The choice between TCA and MVVM often comes down to team preferences, project complexity, and the trade-offs between enforced functional programming principles (TCA) and familiarity/tooling (MVVM). For smaller to medium-sized applications, MVVM might be a more straightforward choice, while TCA could be a better fit for larger, more complex applications where strict unidirectional data flow and functional programming benefits outweigh the learning curve.
Subscribe to my newsletter
Read articles from Nurul Islam directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
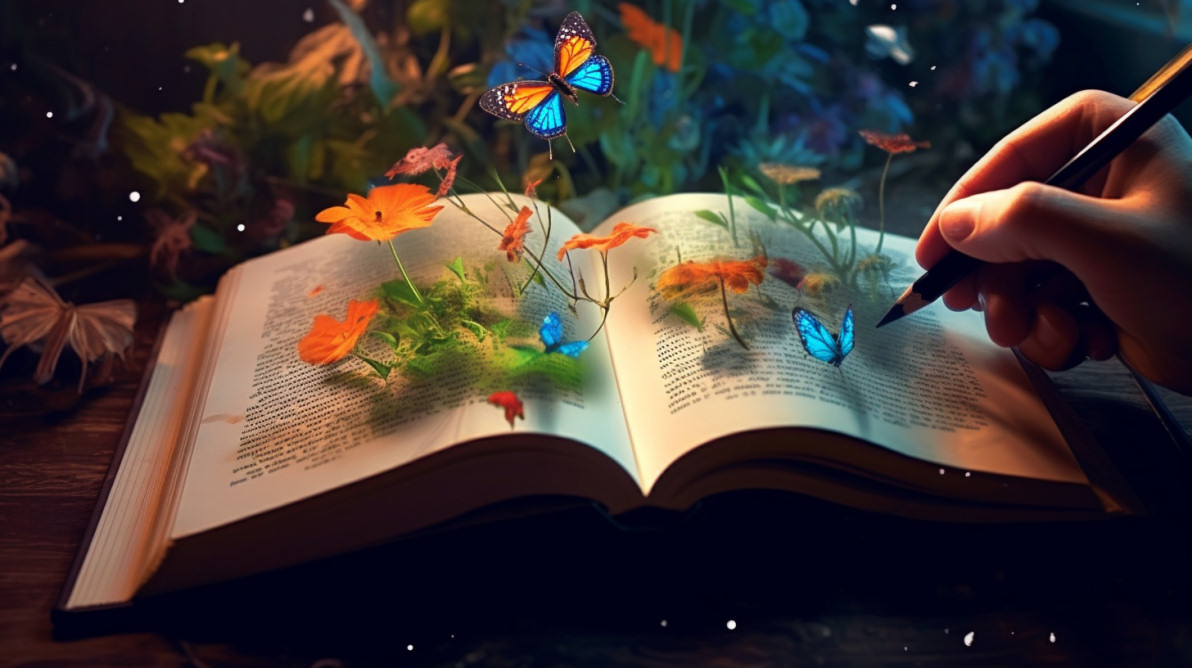
Nurul Islam
Nurul Islam
I'm an experienced mobile application developer with over 10 years of expertise in iOS development using Swift, Objective-C, and Flutter. Throughout my career, I've had the opportunity to work for multiple renowned companies where I developed and architected robust mobile applications used by millions of users worldwide. In addition to my extensive iOS experience, I also have experience working with ASP.Net Core, Entity Framework, and LINQ for backend development as well as Machine Learning and Deep Learning using Python and TensorFlow which has allowed me to collaborate closely with different teams to develop different types of products, apps or services.