Easy Guide to Creating a Web Server with Rust and Actix Web

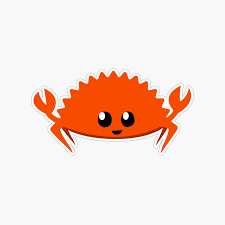
Introduction: Rust has gained popularity in recent years for its performance, safety, and expressiveness. Actix Web, a lightweight and powerful web framework, makes it easy to build web servers and web applications in Rust. In this article, we'll walk through the process of creating a simple web server using Actix Web.
Prerequisites: To follow along with this tutorial, you'll need to have Rust and Cargo installed on your system. You can install Rust and Cargo by following the instructions on the official Rust website: rust-lang.org.
Step 1: Set Up Your Project: Start by creating a new Rust project or navigating to an existing one:
cargo new my_web_server
cd my_web_server
Step 2: Add Actix Web Dependency: Open your Cargo.toml
file and add Actix Web as a dependency:
[dependencies]
actix-web = "4.0"
Step 3: Write Your Server Code: Create a new Rust file (e.g., main.rs
) and write your server code using Actix Web:
use actix_web::{ get, App, HttpServer, Responder};
// Define a handler function for the root ("/") route.
#[get("/")]
async fn hello() -> impl Responder {
format!("Hello world..!!")
}
// The main function where the server is configured and started.
#[actix_web::main]
async fn main() -> std::io::Result<()> {
let port = 8080;
println!("Staring server on port {port}");
// Start an HTTP server and bind it to the local address on port 8080.
HttpServer::new(||
// Create a new Actix Web application.
App::new().service(hello) //Register a route
)
.bind(("127.0.0.1", port))? // Bind the server to the specified address and port.
.run() // Start the server and await for it to finish running.
.await
}
Step 4: Run Your Server: Execute the following command to run your server:
cargo run
Step 5: Access Your Web Server: Open a web browser and navigate to http://localhost:8080
. You should see "Hello, World!" displayed on the page.
Conclusion: Congratulations! You've just created a simple web server in Rust using Actix Web. Actix Web provides a robust foundation for building web applications in Rust, offering features like routing, middleware, and asynchronous processing. Dive deeper into Actix Web's documentation to explore its full potential and unlock more advanced capabilities.
Happy coding! ๐๐ฆ
Subscribe to my newsletter
Read articles from Sachin Borse directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
