Enhancing Gameplay with Visual Effects

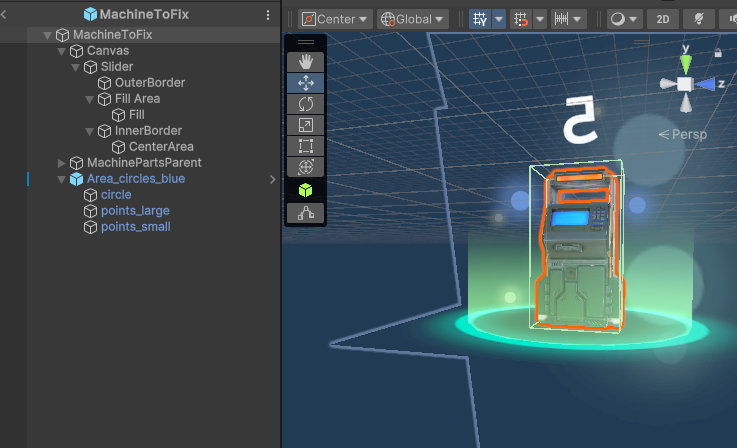
Recognizing the need to improve our game's visuals, we've introduced several new visual effects to enhance gameplay clarity and make the game more visually engaging. Considering the feedback from our final presentation, we have following implementations:
Machine Highlighting: To draw player attention more effectively, we've added a VFX particle effect around the Machine. This not only makes the Machine stand out but also guides players smoothly towards their objective.
Machine Parts Locator: Similarly, VFX particles now encircle Machine Parts, making them easier for players to locate. This enhancement simplifies interaction within the game environment.
Switch Indication: VFX particles have been implemented around character prefabs to indicate the switch mechanism. This activates when players initiate the realm switch mechanism by pressing 'V'.
To maintain game balance, we've introduced a cooldown period of five seconds for this feature. The VFX assets were added to a specially created public GameObject dedicated to these effects.
This mechanism was implemented in the 'MoveOnKeyPress' class which is linked to realm switch mechanism. A Public GameObject is created to reference the VFX prefab in unity and Public Text Mesh pro is created to reference the cool down text. Further in the Update method PlayRealmSwitchVFX is called. After the VFX is played a DestroyVFXObject function is called to destroy the VFX particle. Here is the Script:
using System.Collections; using UnityEngine; using Photon.Pun; using TMPro; public class MoveOnKeyPress : MonoBehaviourPun { private float lrOffset = 317f; private float drOffset = 317f; public TextMeshProUGUI cooldownText; public GameObject realmSwitchVFXPrefab; // Reference to the VFX Prefab private bool canSwitchRealm = true; void Start() { cooldownText.enabled = false; // Disable the cooldown message initially } void Update() { PhotonNetwork.LocalPlayer.CustomProperties.TryGetValue("CurrentRealm", out object _currentRealm); bool isLightRealm = ((string)_currentRealm == "Light"); // If we are in the second realm, we can't go back to the first realm if (photonView.IsMine) { if (Input.GetKeyDown(KeyCode.V) && canSwitchRealm) { AudioSource mainCameraAudioSource = gameObject.GetComponent<AudioSource>(); mainCameraAudioSource.clip = realmSwitch; mainCameraAudioSource.loop = false; mainCameraAudioSource.Play(); if (isLightRealm) { PhotonNetwork.LocalPlayer.SetCustomProperties(new ExitGames.Client.Photon.Hashtable { { "CurrentRealm", "Dark" } }); photonView.RPC("MoveToDR", RpcTarget.AllBuffered, lrOffset); CameraLayersController.switchToDR(); PlayRealmSwitchVFX(transform.position); // Play the VFX when switching to Dark realm } else { PhotonNetwork.LocalPlayer.SetCustomProperties(new ExitGames.Client.Photon.Hashtable { { "CurrentRealm", "Light" } }); photonView.RPC("MoveToLR", RpcTarget.AllBuffered, drOffset); CameraLayersController.switchToLR(); PlayRealmSwitchVFX(transform.position); // Play the VFX when switching to Dark realm } //Start the cooldown co routine StartCoroutine(StartCooldown()); } else if (Input.GetKeyDown(KeyCode.V) && !canSwitchRealm) { Debug.Log("Can't switch realms yet"); cooldownText.enabled = true; //Show the cooldown text cooldownText.text = "Cannot switch realms yet. Cooldown is active."; StartCoroutine(HideCooldownMessage()); } } if (Input.GetKeyDown(KeyCode.M)) { NetworkManager.SpawnMachineParts(); } } [PunRPC] IEnumerator StartCooldown() { canSwitchRealm = false; yield return new WaitForSeconds(5); canSwitchRealm = true; } [PunRPC] IEnumerator HideCooldownMessage() { yield return new WaitForSeconds(2f); //Hide the cooldown message after 2 seconds cooldownText.enabled = false; } [PunRPC] void MoveToDR(float yOffset) { Debug.Log("Moving to DR, offset: " + lrOffset); Vector3 currentPos = transform.position; currentPos.y += lrOffset; transform.position = currentPos; } [PunRPC] void MoveToLR(float dOffset) { Debug.Log("Moving to LR, offset: " + drOffset); Vector3 currentPos = transform.position; currentPos.y -= drOffset; transform.position = currentPos; } [PunRPC] void PlayRealmSwitchVFX(Vector3 position) { // Add a downward offset (adjust the value as needed) position -= Vector3.up * 1.1f; //Todo - this could be done in a nicer way taking the location of the ground and player into account // Instantiate the VFX Prefab at the specified position and play it GameObject vfxObject = PhotonNetwork.Instantiate(realmSwitchVFXPrefab.name, position, Quaternion.identity); if (vfxObject == null) { Debug.LogError("Failed to instantiate VFX prefab!"); return; } // Instantiate the VFX Prefab at the specified position and play it vfxObject.GetComponent<ParticleSystem>().Play(); // Get the duration of the particle system ParticleSystem particleSystem = vfxObject.GetComponent<ParticleSystem>(); float duration = particleSystem.main.duration + particleSystem.main.startLifetime.constantMax; // Destroy the VFX object after the duration for all the clients in game StartCoroutine(DestroyVFXObject(vfxObject, duration)); } IEnumerator DestroyVFXObject(GameObject vfxObject, float duration) { yield return new WaitForSeconds(duration); PhotonNetwork.Destroy(vfxObject); } }
Respawn Notification: Addressing feedback regarding player awareness upon respawning, we've integrated a distinct VFX that triggers within the respawn function in the NetworkManager class. This helps clarify the respawn event visually and re-engages players quickly after being killed.
Timer Implementation: We have implemented a timer to ensure that rounds end when the time expires. The current game round is set at 60 seconds, but this duration is adjustable based on gameplay needs.
To know more about this please clickhereSilver Coin Calculator and Display: A new feature, the silver coin calculator, has been introduced. It rewards players for achieving game objectives and for actions like scoring hits, kills, or assists. The UI elements for Silver and Gold coins have been added next to the respective coin texts.
To know more about this please clickhere
As deadlines approach, our focus will shift towards refining the UI for the Game Over scene, setting us the stats and ironing out any remaining bugs through testing.
Acknowledgements
A special thanks to Molly for her excellent work on setting up the machine mechanics, timer and silver coin calculator.
References
The VFX used was downloaded from Unity Asset Store
https://assetstore.unity.com/packages/vfx/particles/hyper-casual-fx-200333
Thank you for your interest in our project’s development. Stay tuned for more updates.
Shashank Kumar Singh
Subscribe to my newsletter
Read articles from Shashank Kumar Sukumar Singh directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
