#C4 Agent Selection Screen
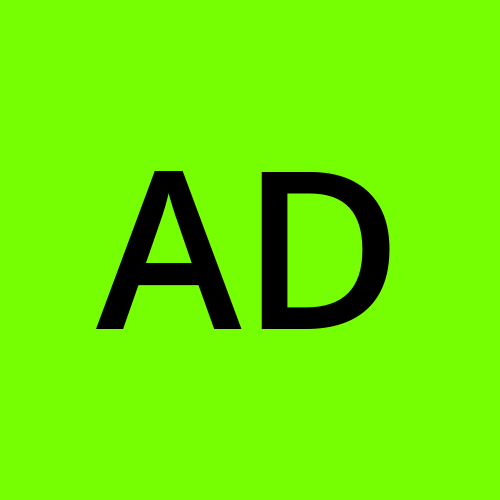
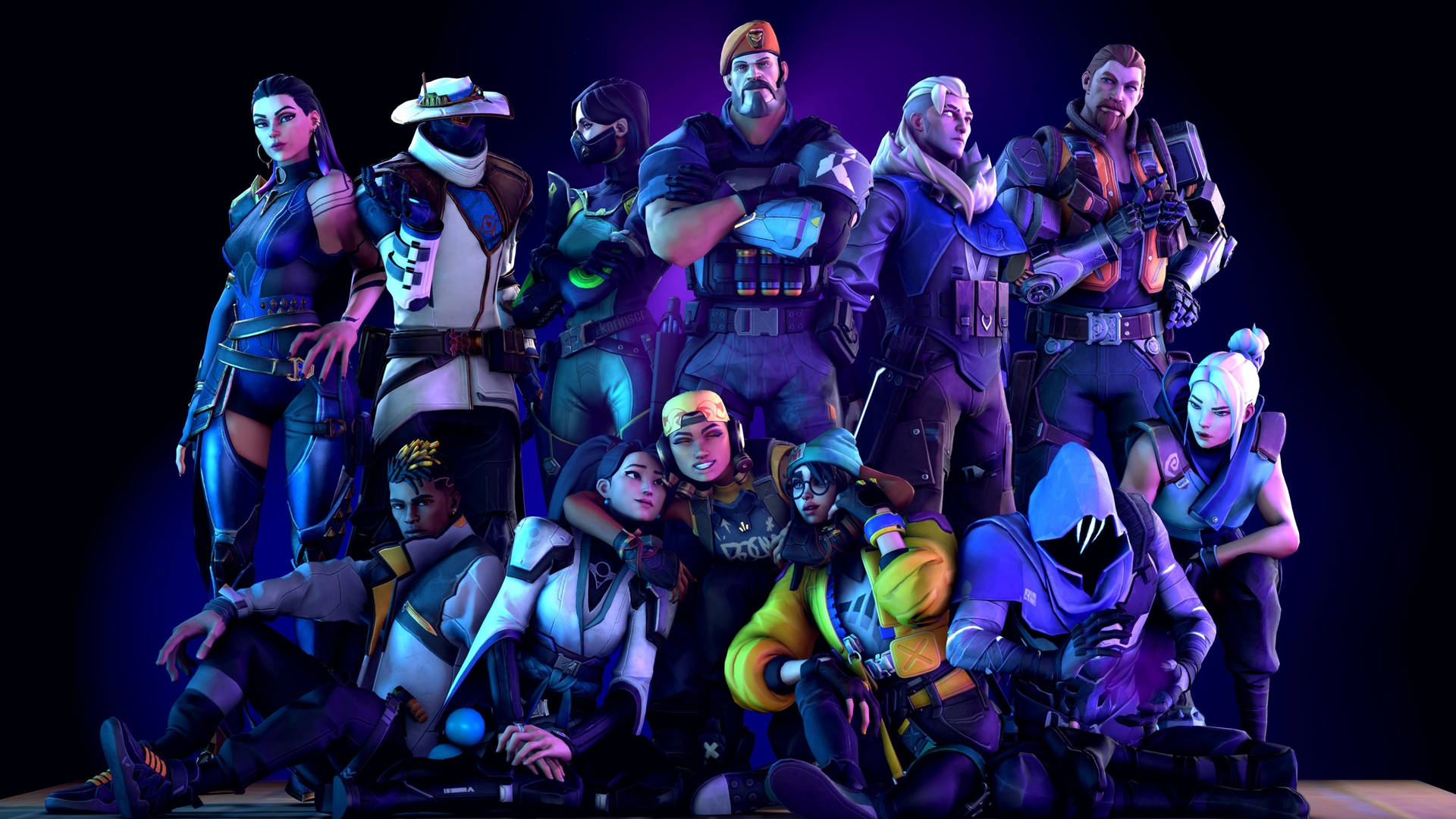
Introduction:
Welcome back to our development journey! In this update, we'll dive into the design and implementation of the agent selection screen, a pivotal step in our game's progression. The agent selection screen serves as the gateway for players to choose their characters, each with unique abilities and playstyles. Join us as we explore the intricacies of bringing this crucial feature to life.
Creating the Agent Selection Screen:
With the foundation of our lobby system in place, we shifted our focus to designing the agent selection screen. Leveraging Unity's UI tools and Photon Unity Networking (PUN), we crafted an immersive interface that allows players to browse and select from a roster of available agents. Each agent offers distinct abilities and traits, providing players with diverse options to suit their playstyles and strategies.
Agent Selection Buttons:
To facilitate agent selection, we implemented interactive buttons for each available agent. Players can choose from a variety of characters, including Connor, Chloe, and Ryan, each with their own unique abilities and roles. These buttons serve as intuitive tools for players to navigate the selection process and make informed decisions about their chosen characters.
public void SelectAgent(int agentIndex)
{
if (!agentsLocked)
{
// Get the selected agent name based on the index
string selectedAgent = "";
switch (agentIndex)
{
case 0:
selectedAgent = "Connor";
break;
case 1:
selectedAgent = "Chloe";
break;
case 2:
selectedAgent = "Ryan";
break;
}
// Update selected agent for the local player
selectedAgents[PhotonNetwork.LocalPlayer.ActorNumber] = selectedAgent;
// Update UI for the local player
UpdatePlayerUI(PhotonNetwork.LocalPlayer.ActorNumber, selectedAgent);
// Sync selected agent across all players
photonView.RPC("SyncSelectedAgent", RpcTarget.All, PhotonNetwork.LocalPlayer.ActorNumber, selectedAgent);
//Enabling the lock button after selecting a character
lockButton.SetActive(true);
}
}
Locking in Agent Selections:
Once players have chosen their desired agents, they have the option to lock in their selections using the lock button. This action signals that the player is ready to proceed and confirms their chosen agent for the upcoming match. Once all players have locked in their selections, the game advances to the next phase, preparing for the action-packed gameplay to come.
public void LockAgents()
{
agentsLocked = true;
lockButton.SetActive(false); // Hide the lock button after locking
// Update selected agent in the player's custom properties
string selectedAgent = selectedAgents[PhotonNetwork.LocalPlayer.ActorNumber];
Hashtable hash = new Hashtable();
hash.Add("SelectedAgent", selectedAgent);
PhotonNetwork.LocalPlayer.SetCustomProperties(hash);
//Hiding the Char buttons
ConnorButton.SetActive(false);
RyanButton.SetActive(false);
ChloeButton.SetActive(false);
//Local Player's Team
string currentPlayerTeam = (string)PhotonNetwork.LocalPlayer.CustomProperties["Team"];
// Locally done the locking, so syncing to other players
photonView.RPC("SyncLockStatus", RpcTarget.Others, PhotonNetwork.LocalPlayer.ActorNumber, agentsLocked, selectedAgent, currentPlayerTeam);
}
Syncing Agent Selections Across Players:
To ensure consistency and fairness, we implemented mechanisms to sync agent selections across all players in the room. Using Photon's networking capabilities, we transmit each player's chosen agent to all other players, providing everyone with real-time updates on the composition of their team and the opposing team. This synchronization fosters cohesion and coordination among teammates, setting the stage for thrilling multiplayer battles.
[PunRPC]
void SyncSelectedAgent(int actorNumber, string selectedAgent)
{
selectedAgents[actorNumber] = selectedAgent;
UpdatePlayerUI(actorNumber, selectedAgent);
}
[PunRPC]
void SyncLockStatus(int actorNumber, bool locked, string selectedAgent, string team)
{
string currentPlayerTeam = (string)PhotonNetwork.LocalPlayer.CustomProperties["Team"];
if (team == currentPlayerTeam)
{
if (selectedAgent == "Connor")
{
ConnorButton.SetActive(false);
}
else if (selectedAgent == "Chloe")
{
ChloeButton.SetActive(false);
}
else if (selectedAgent == "Ryan")
{
RyanButton.SetActive(false);
}
}
}
}
Conclusion:
The development of the agent selection screen represents a significant milestone in our game's evolution. With its intuitive design and seamless functionality, the agent selection screen empowers players to customize their gameplay experiences and express their individuality on the battlefield. In the next update, we'll explore the main game level, where players will put their chosen agents to the test in intense, action-packed matches. Stay tuned for more exciting developments as we continue to push the boundaries of multiplayer gaming!
Subscribe to my newsletter
Read articles from Adhisivan directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
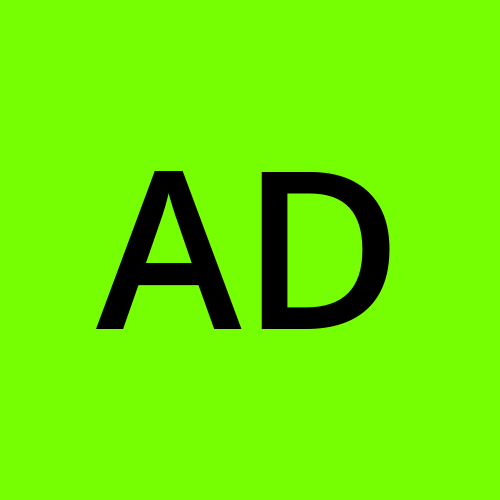