Day 63 : Terraform Variables

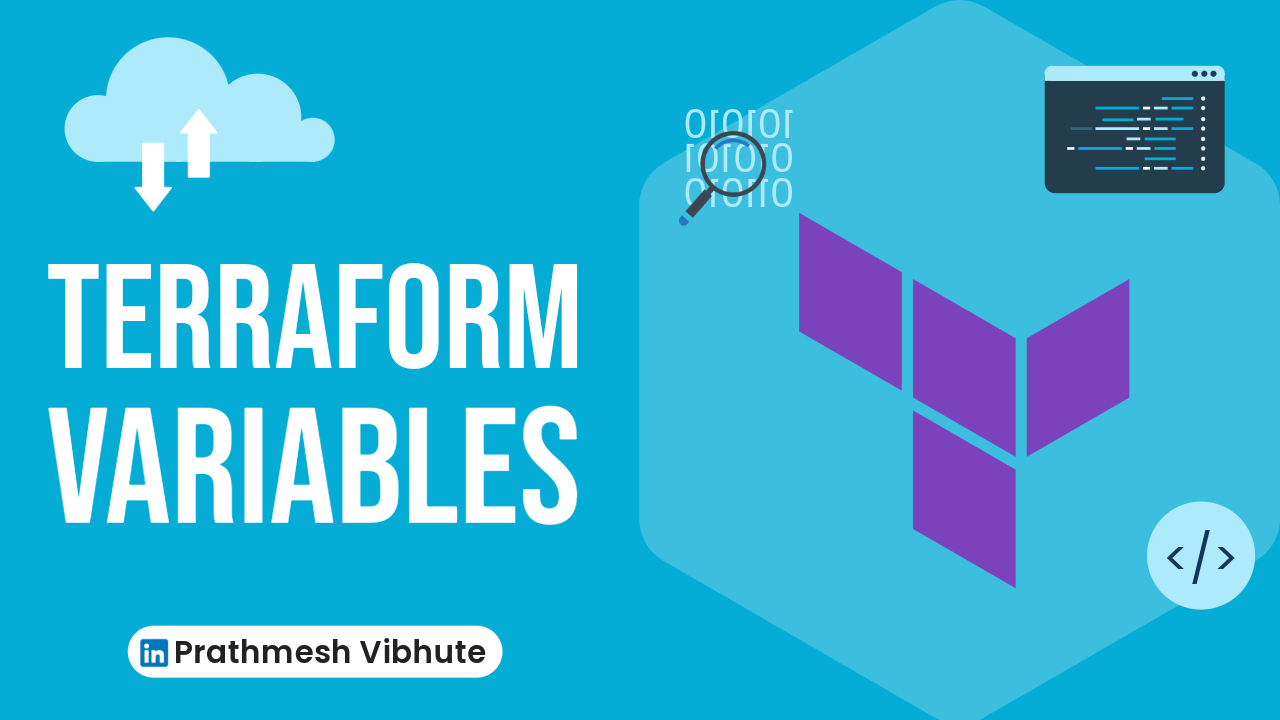
Variables in Terraform serve as the glue that holds together the different parts of your infrastructure deployment. Whether you're managing instances, configurations, or any other resource, understanding and utilizing Terraform variables effectively is key to a smooth and scalable infrastructure as code (IaC) workflow.
Defining Variables
The first step in harnessing Terraform variables is to create a variables.tf
file where you'll declare all your variables. Let's take a look at a simple example:
variable "filename" {
default = "/home/ubuntu/terrform/terraform-variables/demo-var.txt"
}
variable "content" {
default = "This is coming from a variable which was updated"
}
In this snippet, we define two variables: filename
and content
. The filename
variable holds the path to a file, while content
contains some text that we'll use later on.
Task-01
Create a local file using Terraform.
create main.tf file
resource "local_file" "devops" {
filename = var.filename
content = var.content
}
Terraform code block creates a local_file resource named "DevOps" that writes a file to disk with the filename and content specified by the filename and content variables.
Initializes a new or existing terraform working directory
terraform will take to reach the desired state specified in the configuration file
Run Terraform apply, Terraform will create a file called demo-var.txt in the local directory with the content specified in the content variable.
Check file is created in a specified folder using ls command.
Data Types in Terraform
Map
variable "file_contents" {
type = map
default = {
"statement1" = "this is cool"
"statement2" = "this is cooler"
}
}
Task-02
Use terraform to demonstrate usage of List, Set and Object datatypes
In the example, a map variable named content_map is defined with a default value of two key-value pairs. The keys are strings and the values are also strings.
This variable can be used in your Terraform configuration to reference the values associated with each key defined in the map. For example, you might use it to configure a resource that requires content,
resource "local_file" "devops" {
filename = "/home/ubuntu/terraform/terraform-variable/type=map/demo.txt"
content = var.content["statement1"]
}
terraform init
terraform plan
terraform apply
Check demo.txt file content
List:
A list is an ordered collection of values of the same type. To define a list variable, use the list type and specify the type of elements in the list. Here's an example:
variable "file_list"{
type = list
default = ["/home/ubuntu/terraform/terraform-variable/type-map/file1.txt","/home/ubuntu/terraform/terraform-variable/type-map/file2.txt"]
}
In this example, we define a variable named "file_list" as a list of strings with a default value that contains a file path.
This variable can be used in your Terraform configuration to reference the file paths defined in the list.
resource "local_file" "devops" {
filename = var.file_list[0]
content = var.content_map["content1"]
}
In this example, we use the file_list variable to set the filename parameter of an local_file resource. We reference the first element of the list using the square bracket notation and var.file_list[0] syntax.
terraform plan
terraform apply
Object:
An object is a structural type that can contain different types of values, unlike map, list. It is a collection of named attributes that each have their own type.
In the example, an object variable named aws_ec2_object is defined with a default value of a set of properties for an EC2 instance.
This variable can be used in your Terraform configuration to reference the individual properties of the EC2 instance defined in the object.
COPY
COPY
variable "aws_ec2_object" {
type = object({
name = string
instances = number
keys = list(string)
ami = string
})
default = {
name = "test-ec2-instance"
instances = 4
keys = ["key1.pem","key2.pem"]
ami = "ubuntu-bfde24"
}
}
main. tf
This output can be used to display the value of the ami property in the Terraform output after applying the configuration.
terraform plan
terraform apply
you can see the output of aws-ec2-instance value.
Set:
A set is an unordered collection of unique values of the same type. To define a set variable, use the set type and specify the type of elements in the set. Here's an example:
COPY
COPY
variable "security_groups" {
type = set
default = ["sg-12345678", "sg-87654321"]
}
In this example, we define a variable named "security_groups" as a set of strings with a default value.
Use terraform refresh
To refresh the state of your configuration file, reload the variables
terraform refresh is a Terraform command that retrieves the current state of the resources defined in your configuration and updates the Terraform state file to match that state.
By running Terraform refresh, you can update the state file to reflect the current state of your resources, so that Terraform has an accurate view of what needs to be changed. This command does not make any changes to your resources but simply updates the state file to match the current state of your resources.
Conclusion :
Terraform variables are a fundamental aspect of infrastructure as code, enabling dynamic and reusable configurations. By mastering variables and understanding data types, you empower yourself to create robust and scalable infrastructure deployments with Terraform.
I'm confident that this article will prove to be valuable, helping you discover new insights and learn something enriching .
thank you : )
Subscribe to my newsletter
Read articles from Prathmesh Vibhute directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
