Introduction to JSX In React.
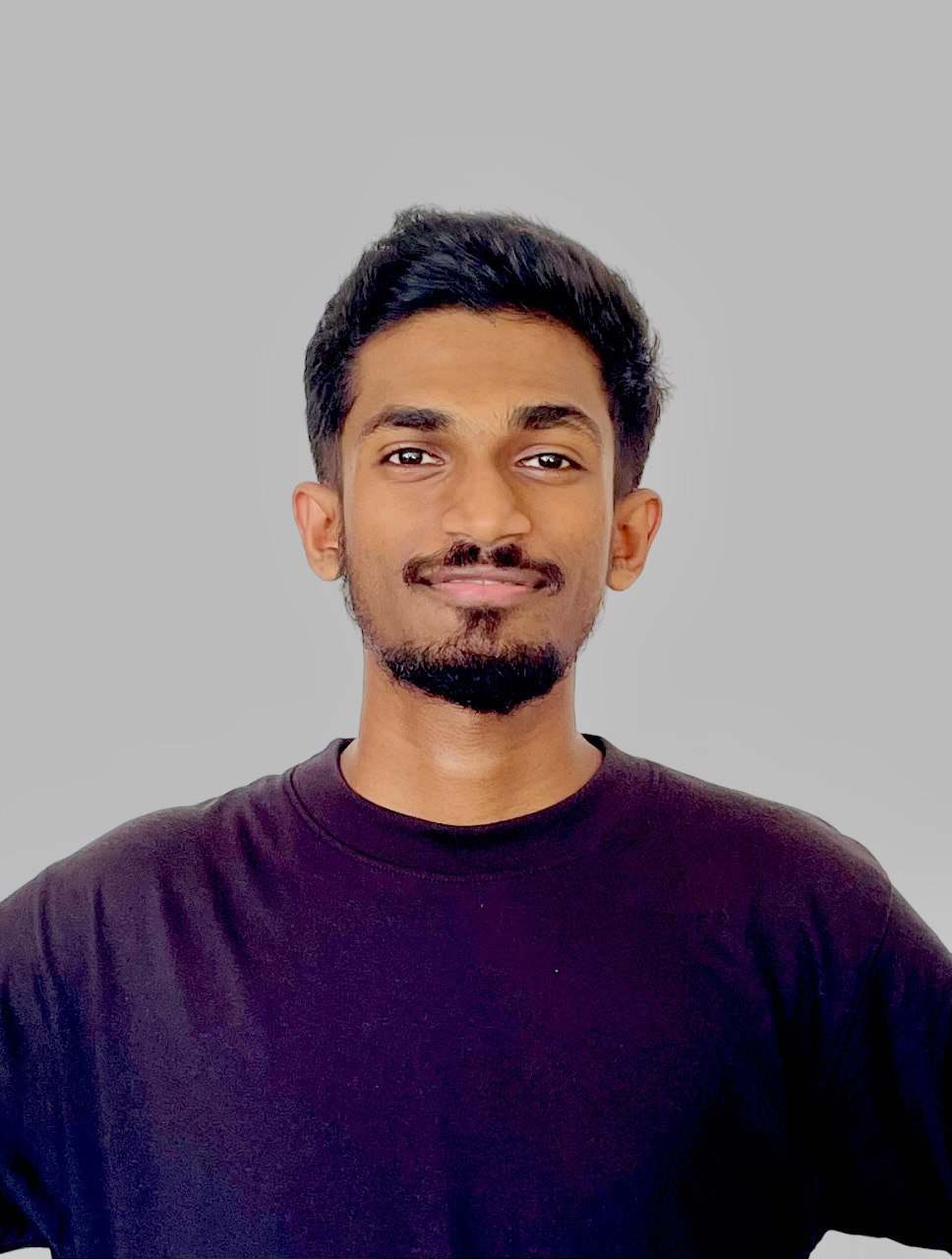
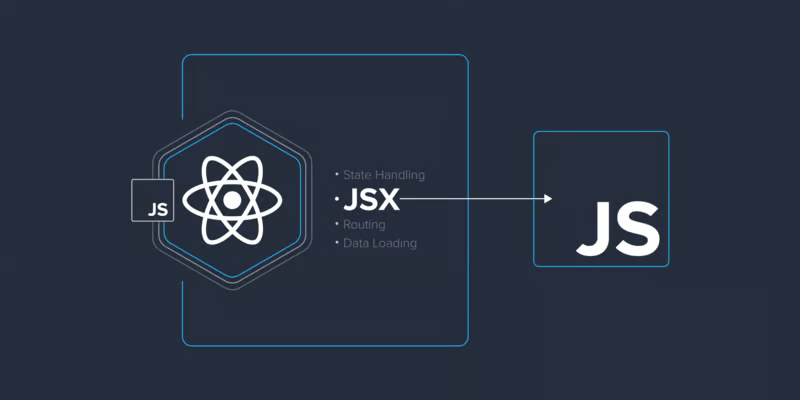
Presently, I'm engrossed in delving into React JS, and today's focus has been on grasping the concept of JSX within React, which stands for JavaScript XML. Consequently, I've been inspired to draft a blog post dedicated to exploring JSX in greater detail. Below, I've crafted an introduction delineating JSX's essence and its pragmatic applications.
JSX, or JavaScript XML, is an extension syntax for JavaScript often used with React, a popular library for building user interfaces. JSX allows developers to write HTML-like code within JavaScript, which is then transformed into regular JavaScript code during compilation. This syntax simplifies the process of creating and manipulating the structure of user interfaces, as developers can seamlessly integrate HTML-like markup with JavaScript logic. JSX also enables the use of familiar HTML tags and attributes, making it easier for developers to reason about and maintain their code. Despite its name, JSX is not limited to XML-like syntax; it supports embedding JavaScript expressions within curly braces, enabling dynamic content generation. This powerful combination of JavaScript and markup has contributed to the widespread adoption of React for building modern web applications.
const example=<h1>Hellow JSX</h1>;
Why We Use JSX in React?
· JavaScript XML (JSX): JSX is an extension syntax for JavaScript.
· Used with React: It's primarily utilized with React, a popular library for building user interfaces.
· HTML-like Syntax: Allows developers to write HTML-like code within JavaScript.
· Transformed into JavaScript: JSX code is transformed into regular JavaScript during compilation.
· Simplifies UI Development: Makes it easier to create and manipulate the structure of user interfaces.
· Integration with JavaScript Logic: Seamlessly integrates HTML-like markup with JavaScript logic.
· Supports Familiar HTML Tags and Attributes: Enables the use of familiar HTML tags and attributes.
· Dynamic Content Generation: Supports embedding JavaScript expressions within curly braces for dynamic content generation.
· Contributes to React's Popularity: The combination of JavaScript and markup has contributed to React's widespread adoption for modern web applications.
Let's talk about How to JSX Use in React
1. Import React: Begin by importing the React library at the top of your file. This step is necessary because JSX gets transpiled into React.createElement() calls.
2.Define React JSX components: Components are the building blocks of React applications. You can create functional components or class components. To define a functional component, declare a function that returns JSX code. JSX elements are similar to HTML tags and allow you to describe the structure and content of your components. You can use these elements within your component’s return statement. Commonly developers use functional components in react.
import React from 'react';
function Welcome() {
return (
<div>
<h1>Hello welcome </h1>
</div>
);
}
export default Welcome;
3. Render JSX: Finally, render your JSX code by including your component within the ReactDOM.render() function, passing in the component and the target DOM element where you want to render it.
import React from "react";
import Welcome from "./Welcom";
function App() {
return (
<>
<Welcome />
</>
);
}
export default App;
Then, use the ReactDOM.render() method to render your top-level component into that root element.
import React from 'react'
import ReactDOM from 'react-dom/client'
import App from './App.jsx'
import './index.css'
ReactDOM.createRoot(document.getElementById('root')).render(
<React.StrictMode>
<App />
</React.StrictMode>,
)
<!doctype html>
<html lang="en">
<head>
<meta charset="UTF-8" />
<link rel="icon" type="image/svg+xml" href="/vite.svg" />
<meta name="viewport" content="width=device-width, initial-scale=1.0" />
<title>Vite + React</title>
</head>
<body>
<div id="root"></div>
<script type="module" src="/src/main.jsx"></script>
</body>
</html>
By following these steps, you can start using JSX to build interactive web applications with React.
What are the Benefits of Using JSX With React.js?
Component-Based Development: React.js promotes a component-based development approach, where the user interface is divided into reusable and independent components. JSX facilitates the creation and composition of these components by allowing developers to define them as functions or classes directly in the code.
Efficient Development Workflow: With JSX, developers can write both the markup and the logic of a component in a single file, eliminating the need to switch between multiple files. This improves the development workflow and reduces the complexity of managing separate HTML templates and JavaScript files.
Static Type Checking: JSX can be used with TypeScript, a popular statically-typed superset of JavaScript. When combined with TypeScript, JSX provides static type-checking capabilities, allowing developers to catch type-related errors during compile time rather than runtime. This leads to improved code quality and robustness.
Performance Optimization: React.js uses a virtual DOM to efficiently update and render components. JSX plays a crucial role in this optimization process by allowing React to perform efficient diffing (comparing and updating only the necessary parts) between the virtual DOM and the actual DOM. This results in improved rendering performance.
Ecosystem and Tooling Support: JSX has gained widespread adoption and has a thriving ecosystem around it. Numerous tools, libraries, and extensions have been developed to enhance the development experience with JSX and React.js. This includes powerful code editors, syntax highlighting, linting tools, and more, which can help developers write clean and error-free JSX code.
JSX is like the special ingredient that makes React's user interfaces so dynamic and cool. It's a way of writing code that mixes HTML-like tags with JavaScript, making it easier and faster to create interactive parts of a website or app. With JSX, developers can do more with their code, making it easier to understand and work with. It's a big deal because it lets developers use all the cool features of JavaScript to control how things look and work on a webpage. So, basically, using JSX with React opens up a ton of exciting possibilities for building really awesome and flexible apps.
Thank you for reading and for your feedbacks!
Subscribe to my newsletter
Read articles from Uvindu Abeywardhana directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
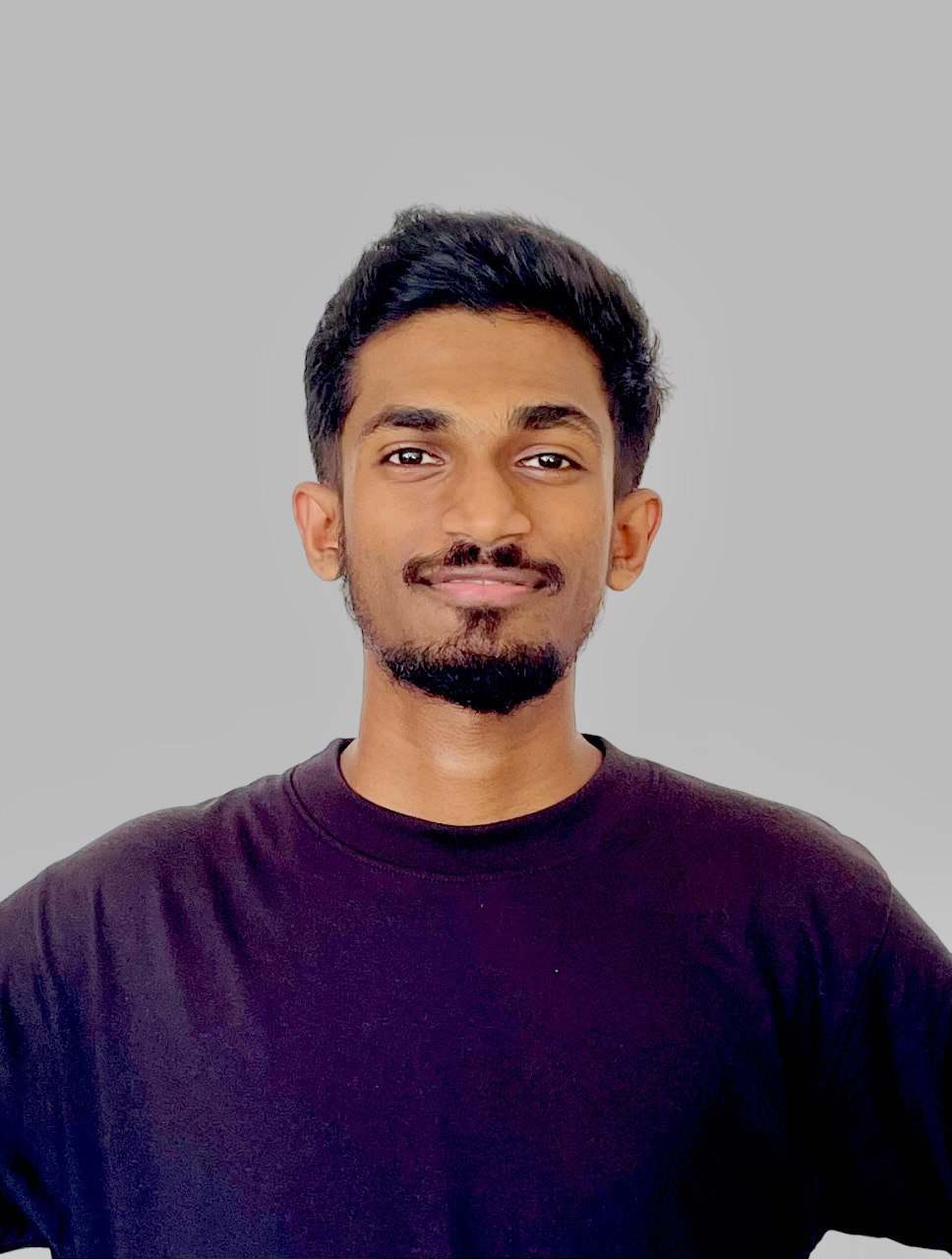
Uvindu Abeywardhana
Uvindu Abeywardhana
I am undergraduate student of the University of Sri Jayewardenepura. I have strong technical skills as well as excellent interpersonal skills that enable me to interact with a team. My greatest desire in life is to further develop my skills and share my knowledge with other people and organizations.I'm seeking position as technologist with reputable company where I can utilize my analytical, technical skills and knowledge which can further help the development of the company.