Day 85/100 100 Days of Code
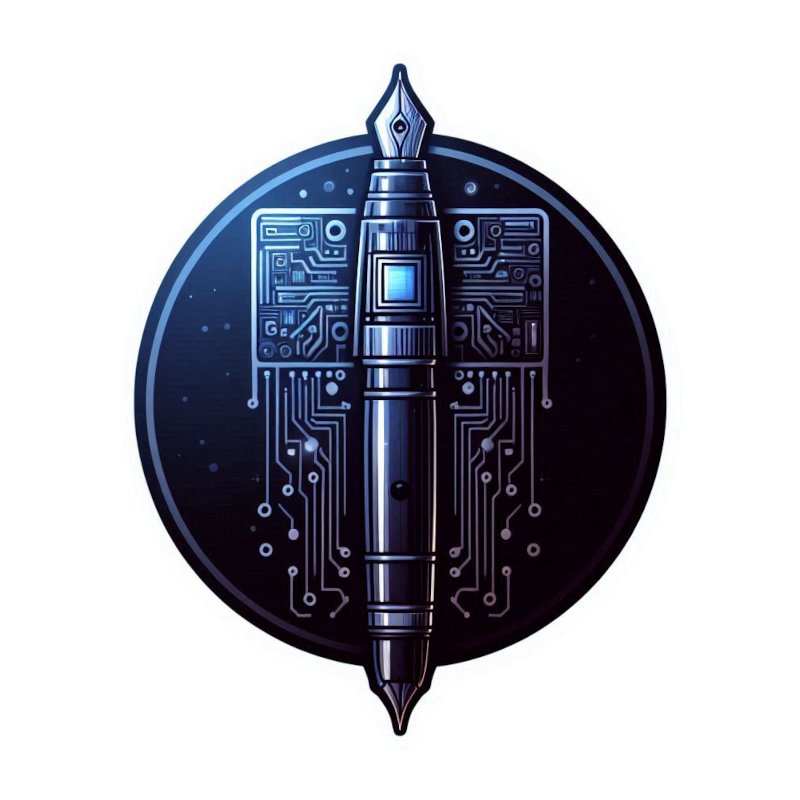
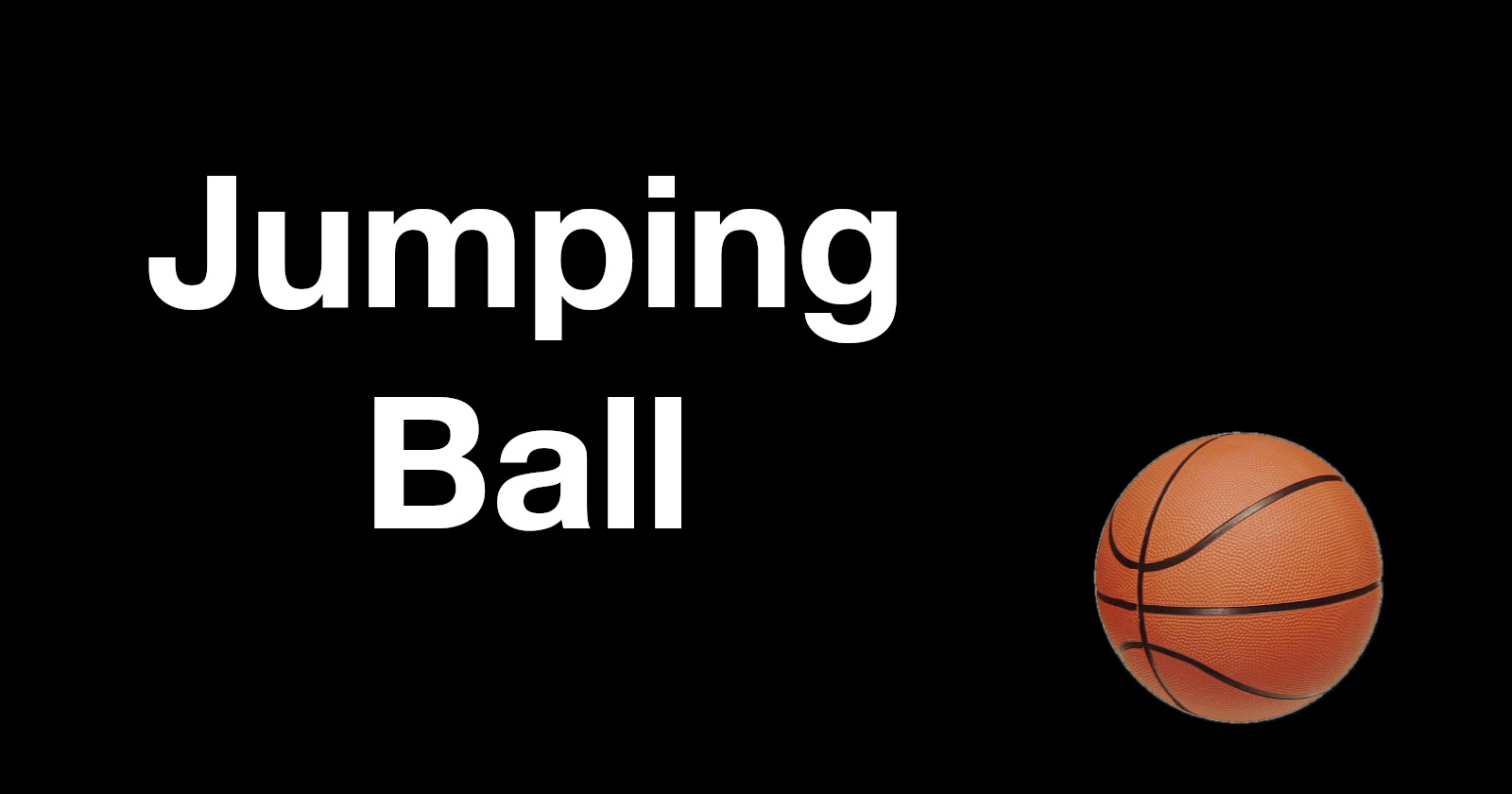
I kept working on the text box. When clicked, it lets the user enter only digits; letters are not accepted, and the value cannot exceed three digits. After exiting edit mode, the cursor is disabled, and the value is displayed. The only thing left is to fix the size of the characters. It might be a good idea to find a way to make the cursor blink.
SDL uses the SDL_StartTextInput()
function to begin reading keyboard input. The SDL_StopTextInput()
function disables this feature. Each keystroke triggers the SDL_EVENT_TEXT_INPUT
event, and event.text.text
can be used to retrieve the character.
I added the SDL_StartTextInput()
and SDL_StopTextInput()
in the same parts of the code where the text box is enabled or disabled.
case SDL_EVENT_MOUSE_BUTTON_UP:
if (mouseState == LEFT_MOUSE_BUTTON)
{
if (IsHoveringExteriorBox(mouseX, mouseY, exteriorTextBox))
{
if(!interiorTextBox.isEnabled)
{
interiorTextBox.isEnabled = true;
SDL_StartTextInput();
}
break;
}
interiorTextBox.isEnabled = false;
SDL_StopTextInput();
}
break;
case SDL_EVENT_KEY_UP:
switch(events.key.keysym.sym)
{
case SDLK_ESCAPE:
interiorTextBox.isEnabled = false;
SDL_StopTextInput();
break;
}
To manage text input and deletion, I integrated several functions and variables to handle the content efficiently and use the right amount of memory. The calloc function has been particularly helpful. Also, I had to ensure that only digits are inserted and that there are no more than three of them.
case SDL_EVENT_TEXT_INPUT:
if (currentStringSize >= 3)
{
break;
}
int getVal = (int)*events.text.text;
if (getVal - 48 < 0 || getVal - 48 > 9)
{
break;
}
temp = calloc(currentStringSize + 1, sizeof(char));
strncpy(temp, interiorTextBox.content, currentStringSize);
strncat(temp, events.text.text, 1);
free(interiorTextBox.content);
interiorTextBox.content = calloc(strlen(temp), sizeof(char));
strncpy(interiorTextBox.content, temp, strlen(temp));
free(temp);
break;
case SDL_EVENT_KEY_UP:
switch(events.key.keysym.sym)
{
case SDLK_ESCAPE:
interiorTextBox.isEnabled = false;
SDL_StopTextInput();
break;
case SDLK_BACKSPACE:
if (currentStringSize <= 0)
{
break;
}
temp = calloc(currentStringSize - 1, sizeof(char));
strncpy(temp, interiorTextBox.content, currentStringSize - 1);
free(interiorTextBox.content);
interiorTextBox.content = calloc(strlen(temp), sizeof(char));
strncpy(interiorTextBox.content, temp, strlen(temp));
free(temp);
break;
}
break;
Demonstration
Subscribe to my newsletter
Read articles from Chris Douris directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
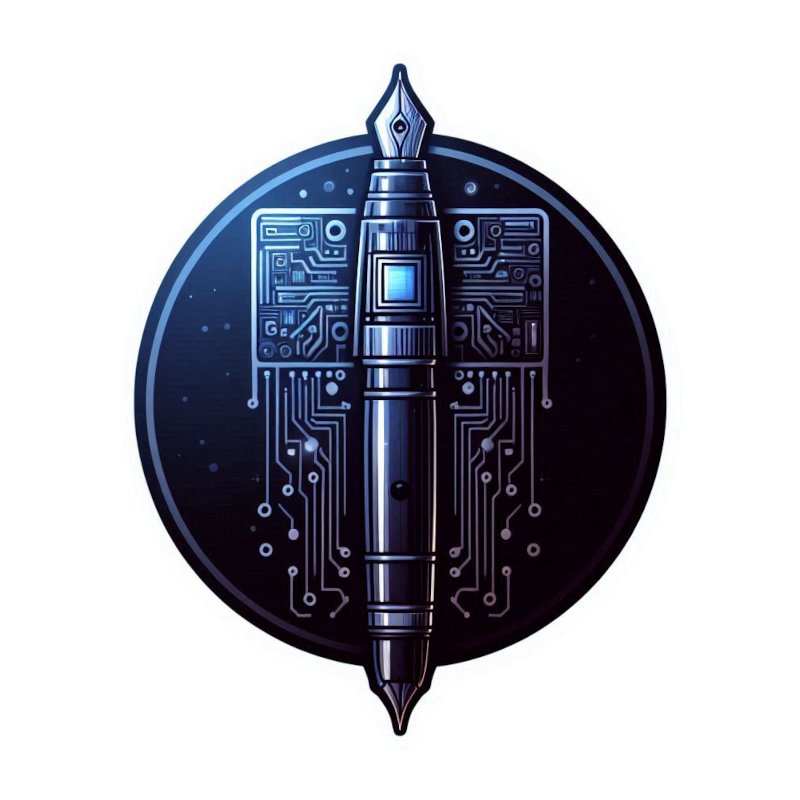
Chris Douris
Chris Douris
AKA Chris, is a software developer from Athens, Greece. He started programming with basic when he was very young. He lost interest in programming during school years but after an unsuccessful career in audio, he decided focus on what he really loves which is technology. He loves working with older languages like C and wants to start programming electronics and microcontrollers because he wants to get into embedded systems programming.