Purely Reasonable: Functionally Enhancing Your UI Workflows
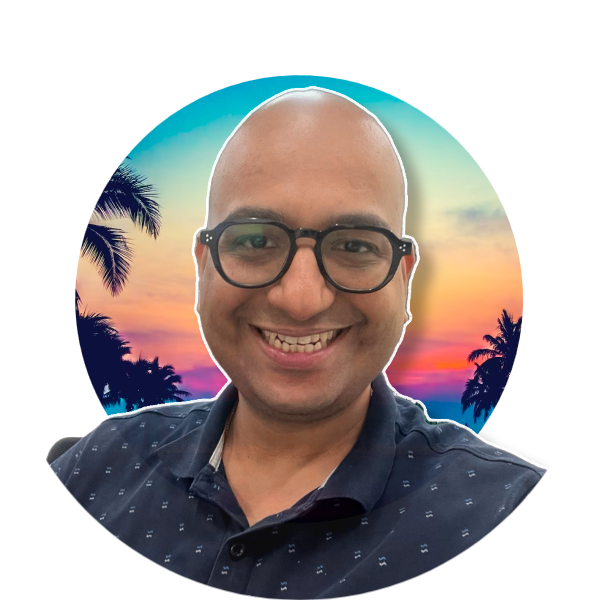
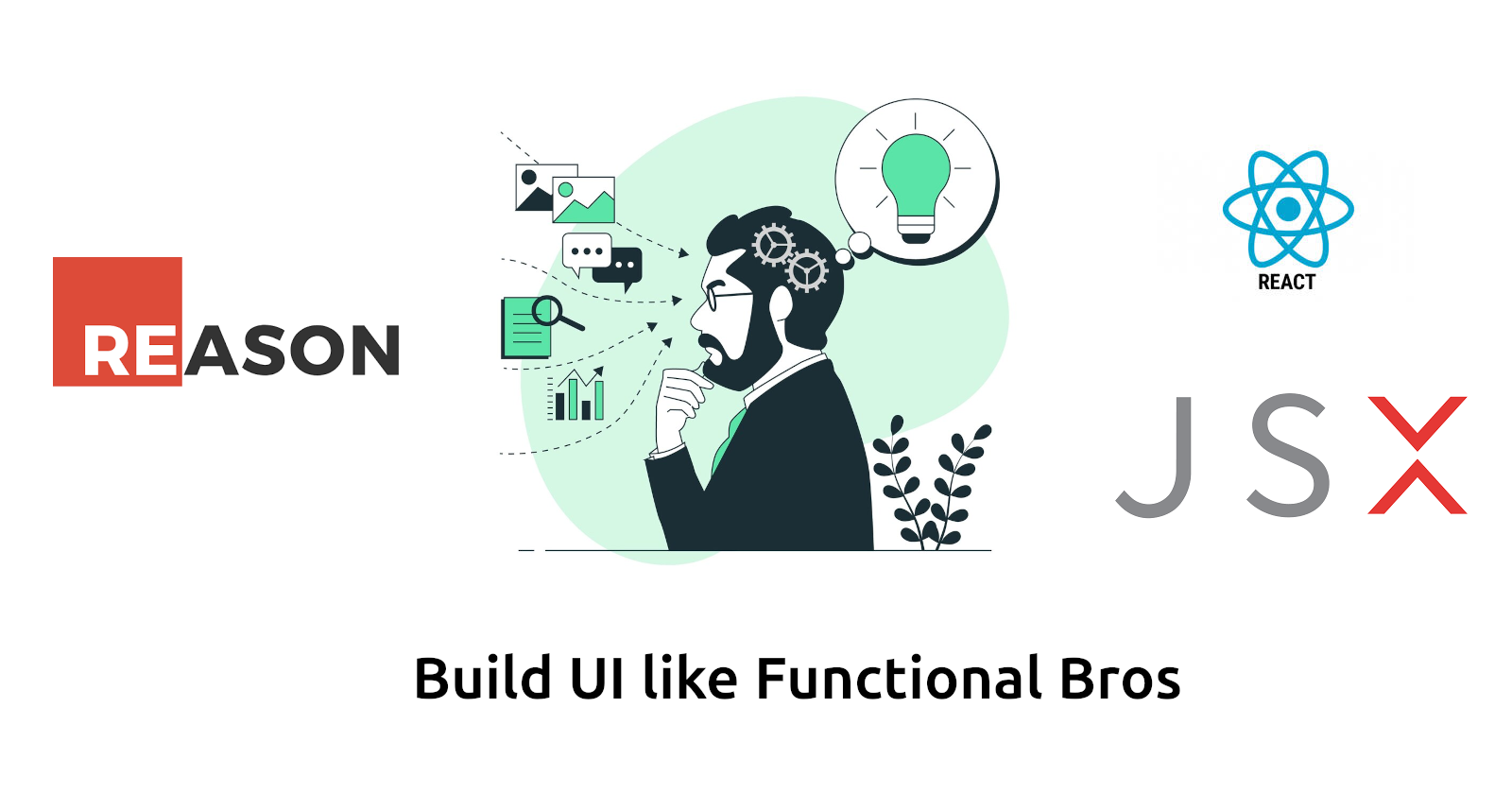
Intro
ReasonML is a powerful programming language that combines functional programming with a familiar C-like syntax, making it both approachable and robust. Built on top of OCaml’s robust type system, ReasonML is an excellent tool for developers familiar with JavaScript, as it offers seamless integration with the JavaScript ecosystem through projects like Melange.
The language is versatile, capable of compiling to bytecode, native code, and JavaScript. This multipurpose capability makes it an attractive choice for developers who are looking to efficiently scale their projects across various platforms. ReasonML's first-class JSX support and fast compilation times further enhance its suitability for modern web development and complex software engineering projects.
What is ReasonML?
Imagine you have a magic toolbox where your tools automatically adjust to the task you're doing, ensuring you hardly make mistakes. That's like ReasonML for coding. It's a smart programming language that helps prevent errors before your code runs, making the process of building apps safer and more efficient.
Examples
1. Let Bindings
let x = 10;
let y = 20;
let z = x + y;
Immutable Variables: This code snippet declares immutable variables using
let
.Basic Arithmetic: Shows how ReasonML handles simple arithmetic operations.
2. Function Definition
let add = (x, y) => x + y;
Function Syntax: Defines a function
add
that takes two parameters and returns their sum.Arrow Functions: Utilizes arrow function syntax similar to JavaScript.
3. Recursive Functions
let rec factorial = (n) =>
n <= 0 ? 1 : n * factorial(n - 1);
Recursion: Illustrates defining recursive functions in ReasonML for calculating factorial.
Conditional Expressions: Uses a ternary operator for conditional logic.
4. Pattern Matching
let getUserType = (user) =>
switch (user.type) {
| Admin => "Admin"
| Guest => "Guest"
| Member => "Member"
};
Pattern Matching: Demonstrates how to handle multiple conditions in a clean and readable way.
Custom Types: Uses custom type variants for different user types.
5. Handling Options
let greet = (name) =>
switch (name) {
| Some(n) => "Hello, " ++ n
| None => "Hello, guest"
};
Options: Shows how to handle optional values which might be
None
orSome(value)
.String Concatenation: Simple string concatenation example.
Use Cases
Web Development
JSX Support: ReasonML supports JSX out of the box, making it ideal for React developers.
Type Safety: Ensures type safety in web apps, reducing runtime errors.
Cross-Platform Desktop Apps
Native Compilation: Compile to native code for performance-critical applications.
Consistent Logic Across Platforms: Shared business logic for desktop platforms.
Complex Frontend State Management
Immutable Data: Leverages immutable data structures to simplify state management.
Robust Type System: Avoids common bugs associated with state changes.
Backend Applications
Performance: Fast execution as backend services compiled to native code.
Concurrent Programming: Efficient and safe concurrent programming capabilities.
Command-Line Tools
Rapid Development: Quick iteration and compilation cycles.
Portable Code: Code can be run across different operating systems without changes.
Conclusion
ReasonML presents a blend of functional programming principles with a syntax that is familiar to JavaScript developers, resulting in a robust, efficient, and enjoyable coding experience. Its compatibility with existing JavaScript and OCaml ecosystems allows developers to harness the power of both worlds. With its strong type system and versatile compilation options, ReasonML is a compelling choice for a wide range of programming tasks.
References
Building React Apps with ReasonML
%[https://www.youtube.com/watch?v=j9Vn6PC2u8k&t=667s&pp=ygUIcmVhc29ubWw%3D]
%[https://www.youtube.com/watch?v=sjWsAYJF8BA]
Subscribe to my newsletter
Read articles from Nikhil Akki directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
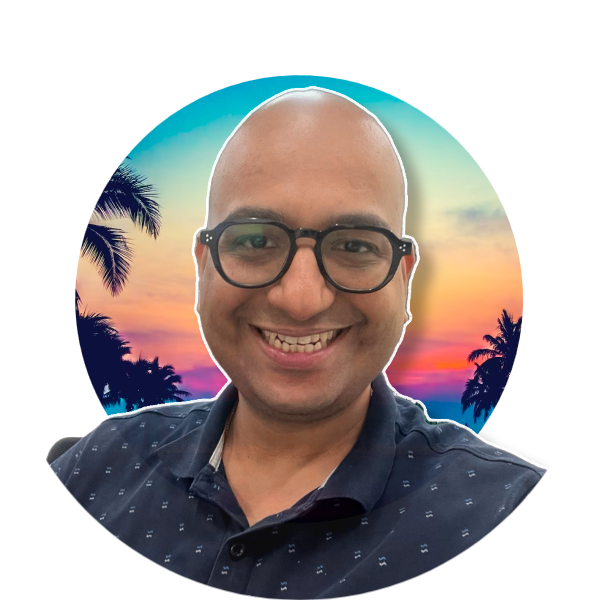
Nikhil Akki
Nikhil Akki
I am a Full Stack Solution Architect at Deloitte LLP. I help build production grade web applications on major public clouds - AWS, GCP and Azure.