Common Errors in Apex (Salesforce) and What They Mean
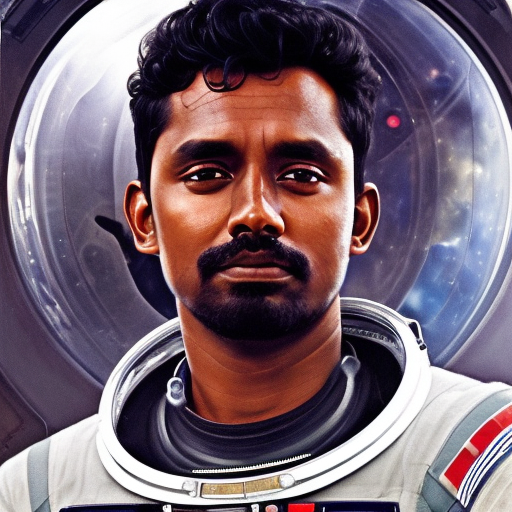
Table of contents
- 1. System.QueryException: List has no rows for assignment to SObject
- 2. System.DmlException: Insert failed. First exception on row 0; first error: REQUIRED_FIELD_MISSING
- 3. System.LimitException: Too many SOQL queries: 101
- 4. System.NullPointerException: Attempt to de-reference a null object
- 5. System.AsyncException: Future method cannot be called from a future or batch method
- 6. System.CalloutException: You have uncommitted work pending. Please commit or rollback before calling out
- 7. System.StringException: Invalid string length
- 8. System.SObjectException: DML requires SObject or SObject list type: String
- 9. System.TypeException: Invalid conversion from runtime type List to List
- 10. System.LimitException: Apex heap size too large
- Conclusion
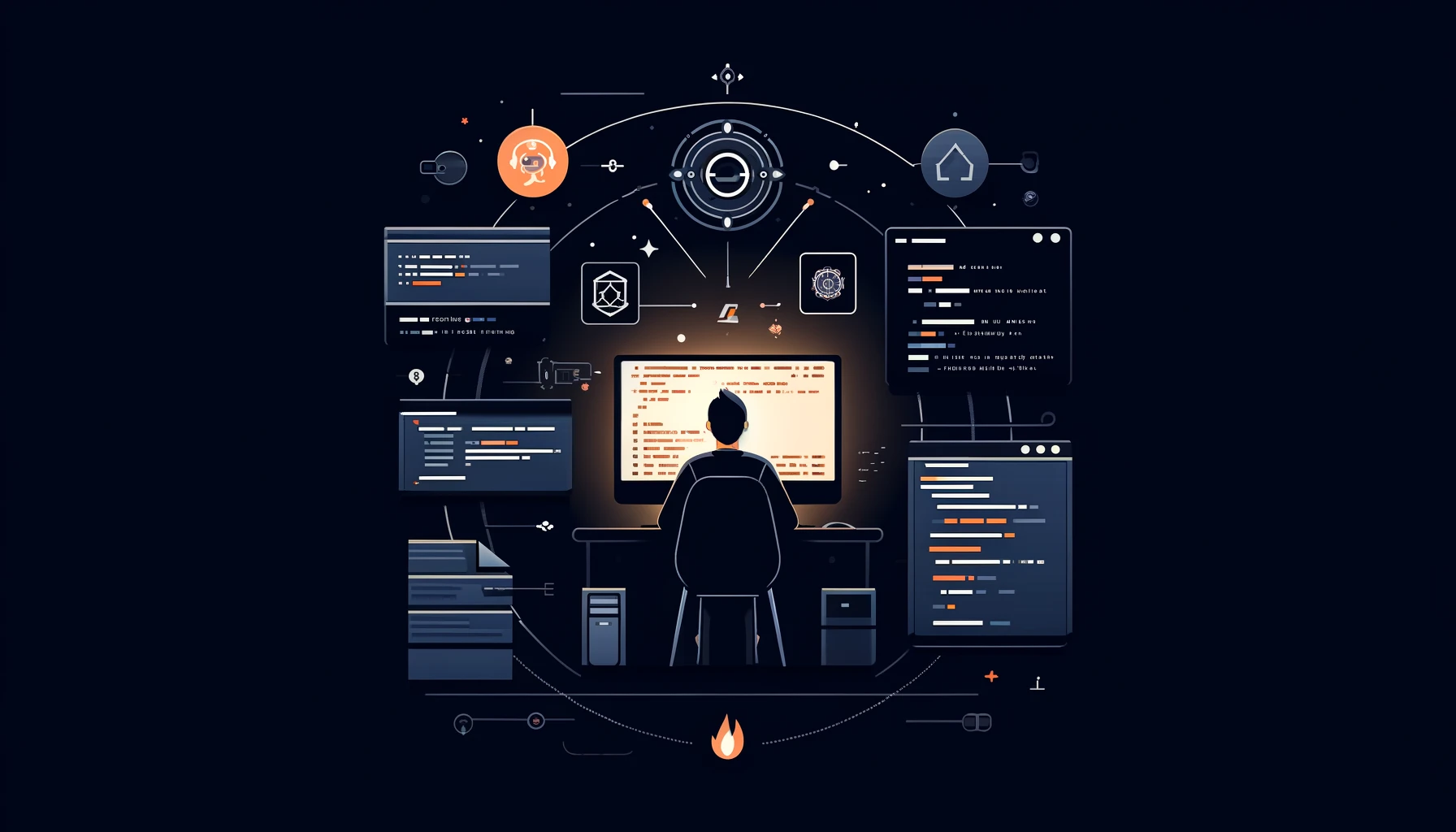
Apex, Salesforce's proprietary programming language, is powerful but can be tricky to master. Developers often encounter a variety of errors during their development journey. Understanding these errors and knowing how to fix them is crucial. Here's a rundown of some common errors in Apex and what they mean.
1. System.QueryException: List has no rows for assignment to SObject
Meaning: This error occurs when a SOQL query expecting a single result (using query()
method) returns no records. For example:
Account acc = [SELECT Id, Name FROM Account WHERE Name = 'NonExistentAccount'];
Solution: Always check if the query returns any results before assigning it to a single SObject.
List<Account> accounts = [SELECT Id, Name FROM Account WHERE Name = 'NonExistentAccount'];
if (!accounts.isEmpty()) {
Account acc = accounts[0];
}
2. System.DmlException: Insert failed. First exception on row 0; first error: REQUIRED_FIELD_MISSING
Meaning: This error indicates that a required field is missing during a DML operation (insert, update, etc.). For instance:
Account acc = new Account();
insert acc;
Solution: Ensure that all required fields are populated before performing the DML operation.
Account acc = new Account(Name = 'Test Account');
insert acc;
3. System.LimitException: Too many SOQL queries: 101
Meaning: Salesforce enforces a limit on the number of SOQL queries that can be executed within a single transaction. This error occurs when your code exceeds the limit (typically 100).
Solution: Optimize your code to reduce the number of SOQL queries. Use collections (lists, maps, sets) and bulk queries to handle multiple records at once.
List<Account> accounts = [SELECT Id, Name FROM Account WHERE Name LIKE 'Test%'];
for (Account acc : accounts) {
// Process each account
}
4. System.NullPointerException: Attempt to de-reference a null object
Meaning: This error occurs when you attempt to access or modify a property of a null object. For example:
Account acc;
System.debug(acc.Name);
Solution: Always check if an object is null before accessing its properties or methods.
Account acc = [SELECT Id, Name FROM Account LIMIT 1];
if (acc != null) {
System.debug(acc.Name);
}
5. System.AsyncException: Future method cannot be called from a future or batch method
Meaning: This error happens when you try to call a future method from another future method, batch job, or scheduled Apex.
Solution: Ensure future methods are called from synchronous context or consider using Queueable Apex for chaining asynchronous operations.
public class AsyncExample {
@future
public static void futureMethod() {
// Do something
}
}
6. System.CalloutException: You have uncommitted work pending. Please commit or rollback before calling out
Meaning: This error indicates that you're attempting an HTTP callout after performing DML operations within the same transaction.
Solution: Perform callouts before DML operations or use separate transactions for callouts and DML.
public void makeCallout() {
HttpRequest req = new HttpRequest();
req.setEndpoint('https://example.com/api');
req.setMethod('GET');
Http http = new Http();
HttpResponse res = http.send(req);
if (res.getStatusCode() == 200) {
Account acc = new Account(Name = 'Test Account');
insert acc;
}
}
7. System.StringException: Invalid string length
Meaning: This error occurs when you attempt to assign a string that exceeds the allowed length for a field.
Solution: Ensure strings are within the allowed length before assignment. Use string manipulation methods to trim or truncate strings if necessary.
String longString = 'This is a very long string...';
if (longString.length() > 255) {
longString = longString.substring(0, 255);
}
8. System.SObjectException: DML requires SObject or SObject list type: String
Meaning: This error occurs when a non-SObject type (e.g., String) is used in a DML statement.
Solution: Ensure that only SObject types are used in DML operations.
String accName = 'Test Account';
Account acc = new Account(Name = accName);
insert acc;
9. System.TypeException: Invalid conversion from runtime type List to List
Meaning: This error occurs when trying to cast a generic list to a specific type without ensuring type safety.
Solution: Use proper type casting and check the data type before casting.
List<SObject> sObjects = [SELECT Id, Name FROM Account];
List<Account> accounts = (List<Account>) sObjects;
10. System.LimitException: Apex heap size too large
Meaning: This error indicates that the heap size limit has been exceeded, which can occur when working with large collections of objects or strings.
Solution: Optimize your code to reduce memory usage. Break down large data sets into smaller chunks and process them separately.
List<Account> accounts = [SELECT Id, Name FROM Account];
for (Integer i = 0; i < accounts.size(); i += 100) {
List<Account> batch = accounts.subList(i, Math.min(i + 100, accounts.size()));
// Process batch
}
Conclusion
Errors in Apex are a natural part of the development process. By understanding common errors and their solutions, you can write more robust and efficient code. Always follow best practices, such as checking for null values, minimizing SOQL queries, and handling DML operations correctly. Happy coding!
Subscribe to my newsletter
Read articles from neelam rakesh directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
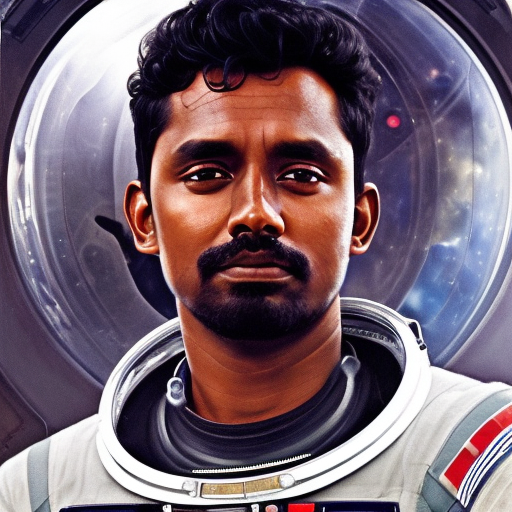