Find Palindrome With Fixed Length
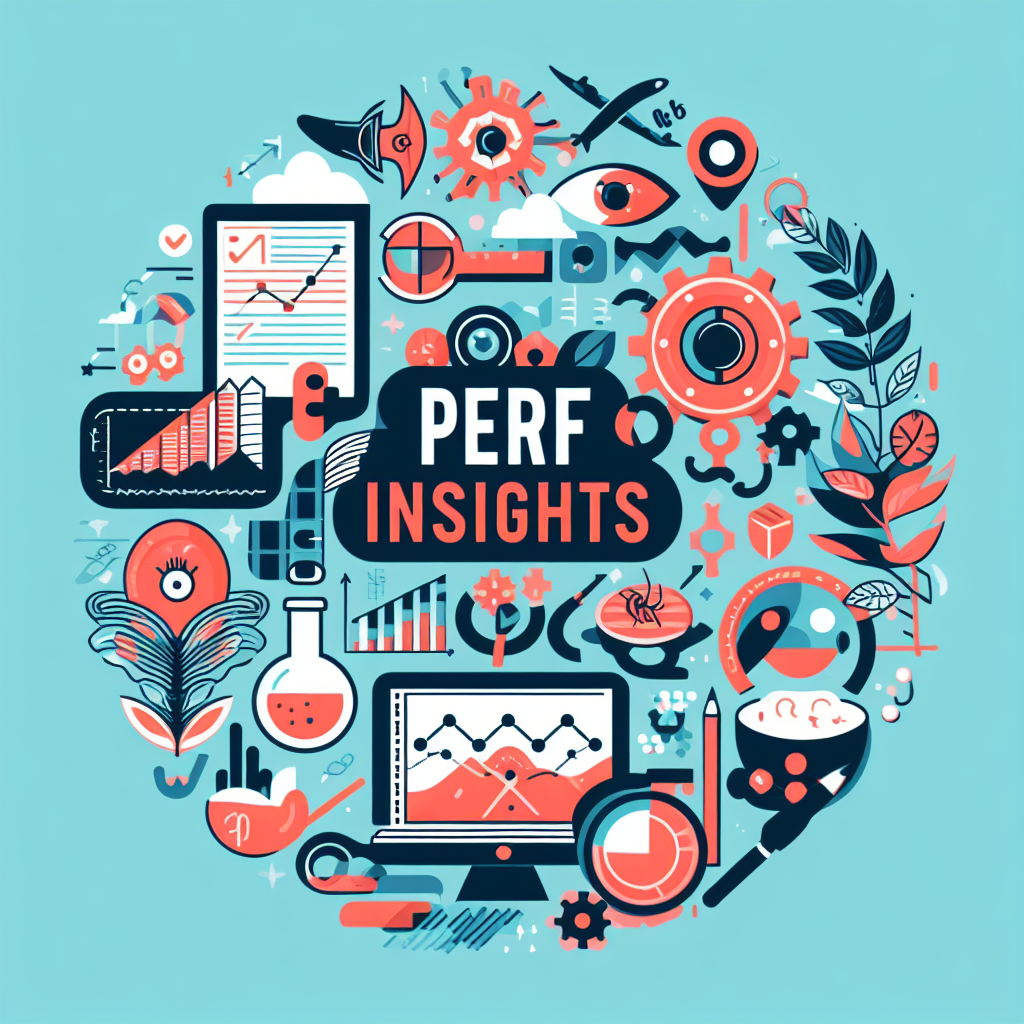
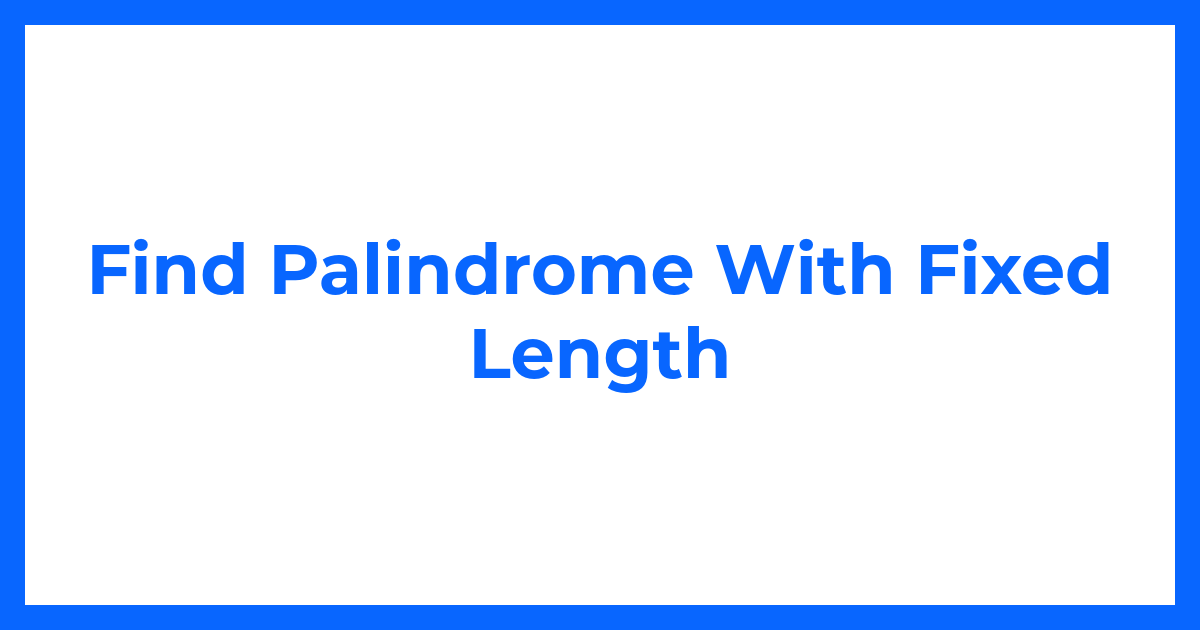
Given an integer array queries
and a positive integer intLength
, return an array answer
where answer[i]
is either the queries[i]<sup>th</sup>
smallest positive palindrome of length intLength
or -1
if no such palindrome exists.
A palindrome is a number that reads the same backwards and forwards. Palindromes cannot have leading zeros.
LeetCode Problem - 2217
class Solution {
// Method to find k-th palindrome numbers for given queries and integer length
public long[] kthPalindrome(int[] queries, int intLength) {
// Array to store results
long[] ans = new long[queries.length];
// Calculate half length and whether it's even or odd
int half = (intLength + 1) / 2;
boolean even = intLength % 2 == 0;
// Calculate the start and end range for palindromes
long start = (long) Math.pow(10, half - 1);
long end = (long) Math.pow(10, half) - 1;
// Iterate through queries
for (int i = 0; i < queries.length; i++) {
// Check if query is within valid range
if (queries[i] <= end - start + 1) {
// Generate the k-th palindrome and store in result array
ans[i] = generatePalindrome(queries[i] + start - 1, even);
} else {
// If query exceeds range, store -1
ans[i] = -1;
}
}
// Return the result array
return ans;
}
// Method to generate palindrome from given number and whether it's even or odd
long generatePalindrome(long n, boolean even) {
// Initialize result with given number
long res = n;
// If it's an odd palindrome, remove the last digit
if (!even)
n = n / 10;
// Append the reverse of the remaining digits to generate the palindrome
while (n > 0) {
res = (res * 10) + (n % 10);
n = n / 10;
}
// Return the palindrome
return res;
}
}
Subscribe to my newsletter
Read articles from Gulshan Kumar directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
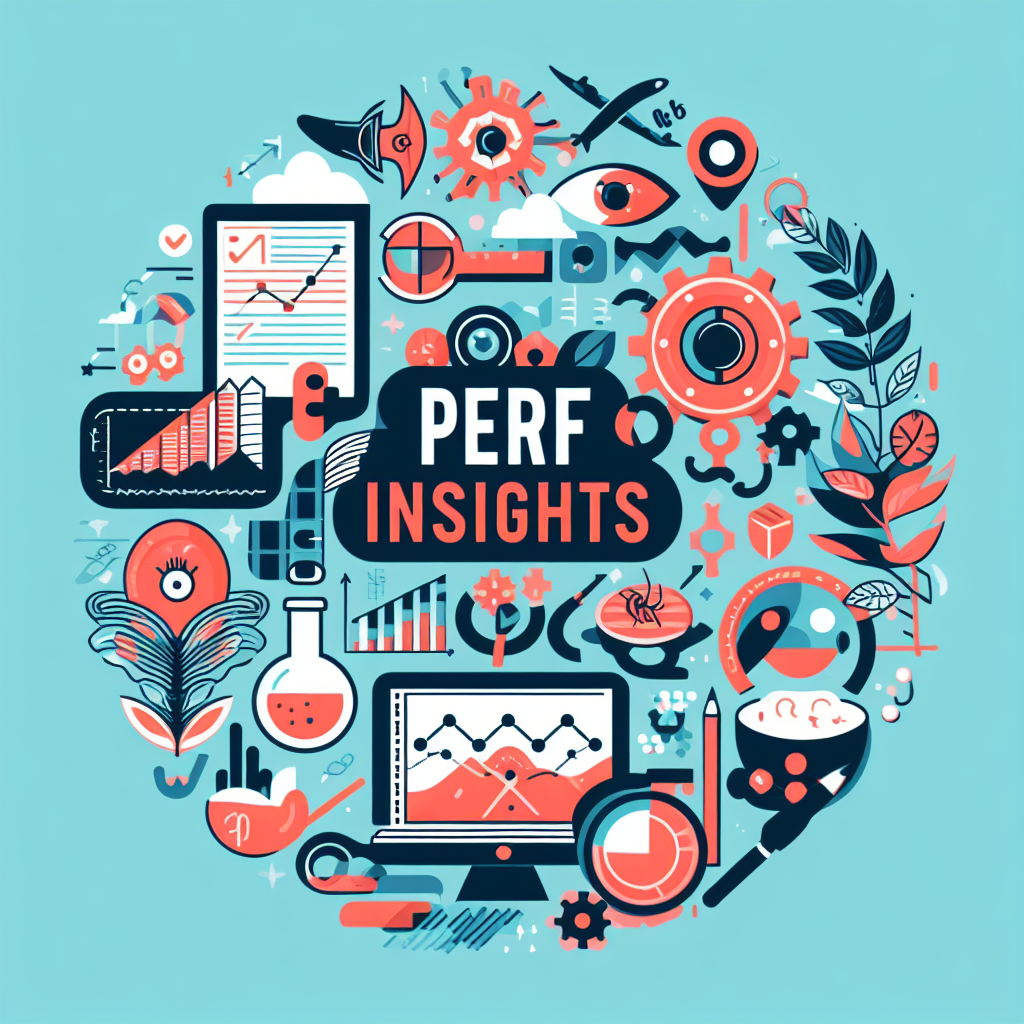
Gulshan Kumar
Gulshan Kumar
As a Systems Engineer at Tata Consultancy Services, I deliver exceptional software products for mobile and web platforms, using agile methodologies and robust quality maintenance. I am experienced in performance testing, automation testing, API testing, and manual testing, with various tools and technologies such as Jmeter, Azure LoadTest, Selenium, Java, OOPS, Maven, TestNG, and Postman. I have successfully developed and executed detailed test plans, test cases, and scripts for Android and web applications, ensuring high-quality standards and user satisfaction. I have also demonstrated my proficiency in manual REST API testing with Postman, as well as in end-to-end performance and automation testing using Jmeter and selenium with Java, TestNG and Maven. Additionally, I have utilized Azure DevOps for bug tracking and issue management.