K-th Smallest Prime Fraction
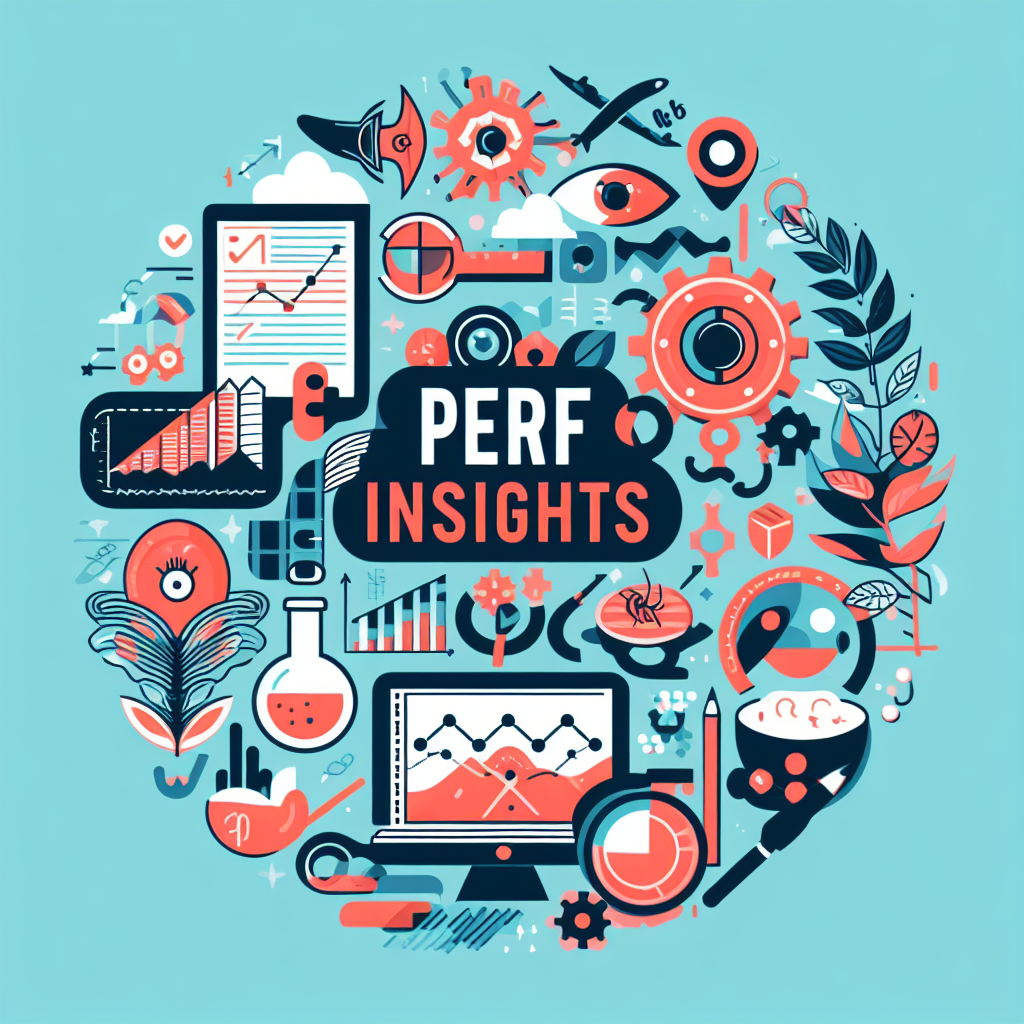
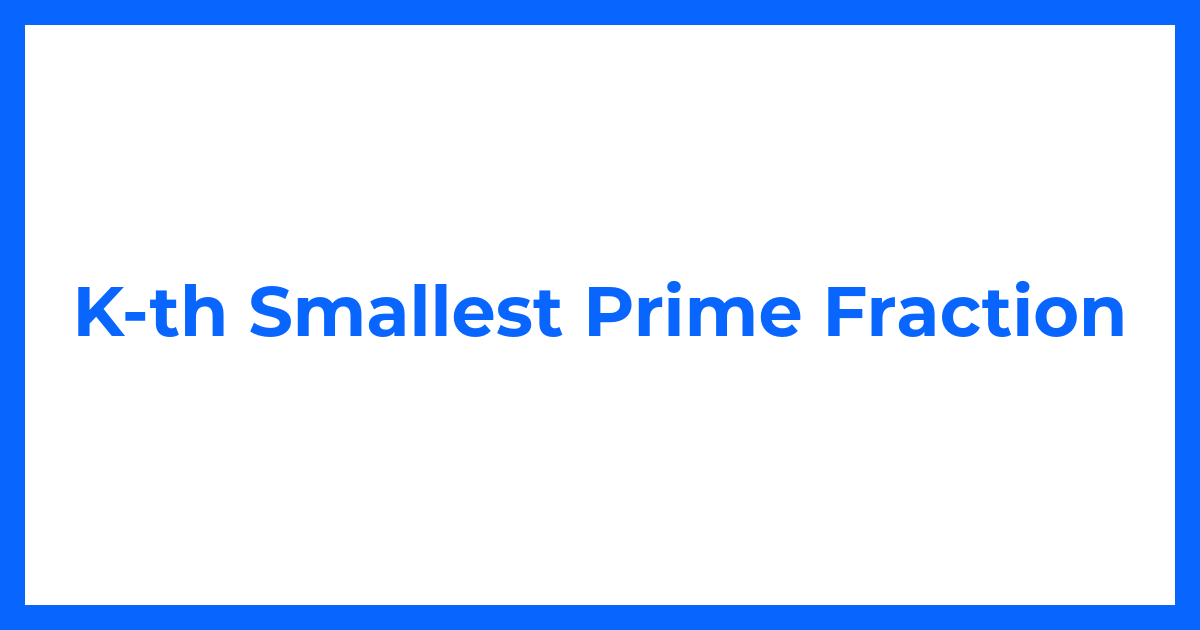
You are given a sorted integer array arr
containing 1
and prime numbers, where all the integers of arr
are unique. You are also given an integer k
.
For every i
and j
where 0 <= i < j < arr.length
, we consider the fraction arr[i] / arr[j]
.
Return the k<sup>th</sup>
smallest fraction considered. Return your answer as an array of integers of size 2
, where answer[0] == arr[i]
and answer[1] == arr[j]
.
LeetCode Problem - 786
class Solution {
// Method to find the kth smallest prime fraction
public int[] kthSmallestPrimeFraction(int[] arr, int k) {
// List to store fractions
List<Double> al = new ArrayList<>();
// Map to store fractions and their string representations
Map<Double, String> mp = new HashMap<>();
// Generate all possible fractions from the array elements
for(int i = 0; i < arr.length; i++) {
for(int j = i + 1; j < arr.length; j++) {
double x = (double) arr[i], y = (double) arr[j];
double fraction = x / y;
al.add(fraction);
String temp = arr[i] + "/" + arr[j];
mp.put(fraction, temp);
}
}
// Sort the list of fractions
Collections.sort(al);
// Get the kth smallest fraction
double answerFraction = al.get(k - 1);
// Get the string representation of the kth smallest fraction
String answerString = mp.get(answerFraction);
// Split the string to get the numerator and denominator
String[] splitAns = answerString.split("/");
// Parse the numerator and denominator to integers
int a = Integer.parseInt(splitAns[0]);
int b = Integer.parseInt(splitAns[1]);
// Return the kth smallest fraction as an array
return new int[] {a, b};
}
}
Subscribe to my newsletter
Read articles from Gulshan Kumar directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
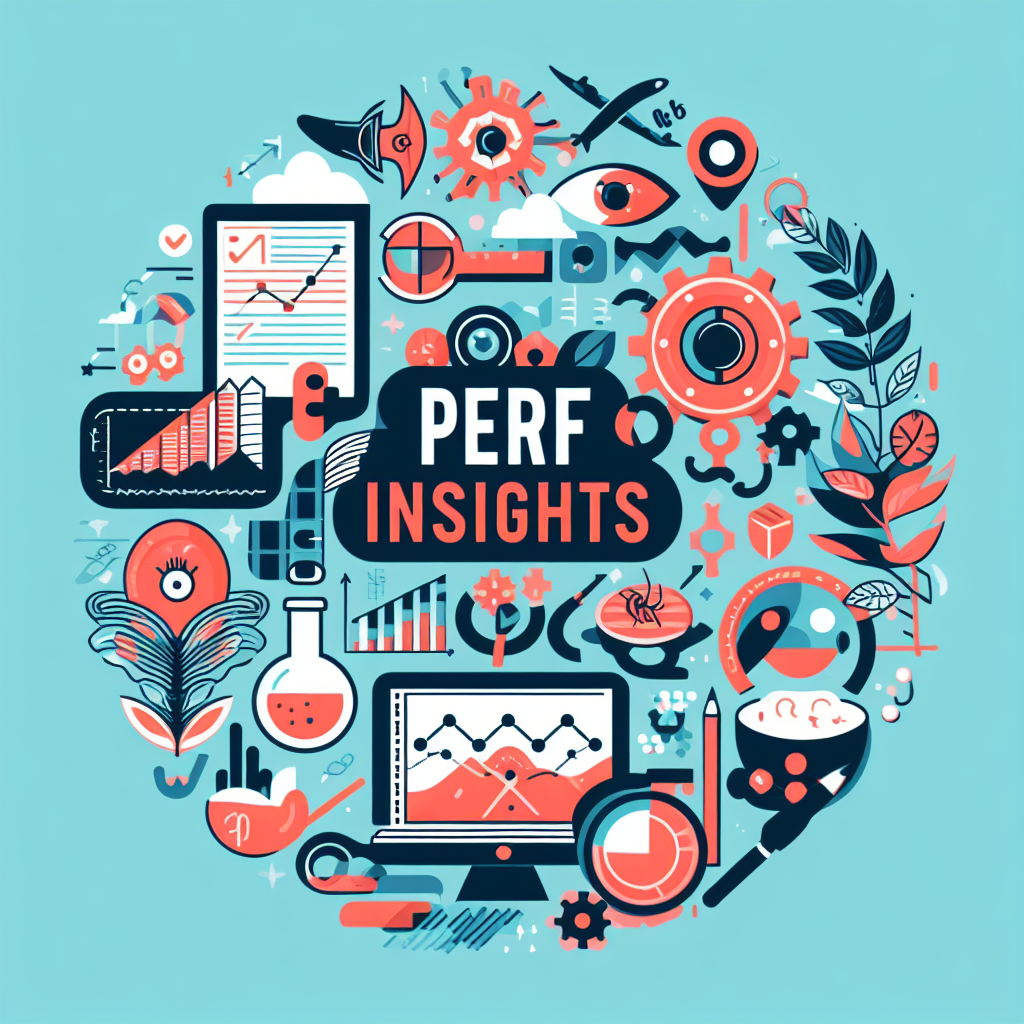
Gulshan Kumar
Gulshan Kumar
As a Systems Engineer at Tata Consultancy Services, I deliver exceptional software products for mobile and web platforms, using agile methodologies and robust quality maintenance. I am experienced in performance testing, automation testing, API testing, and manual testing, with various tools and technologies such as Jmeter, Azure LoadTest, Selenium, Java, OOPS, Maven, TestNG, and Postman. I have successfully developed and executed detailed test plans, test cases, and scripts for Android and web applications, ensuring high-quality standards and user satisfaction. I have also demonstrated my proficiency in manual REST API testing with Postman, as well as in end-to-end performance and automation testing using Jmeter and selenium with Java, TestNG and Maven. Additionally, I have utilized Azure DevOps for bug tracking and issue management.