Enhance JSON String Readability with this Simple JavaScript Hack
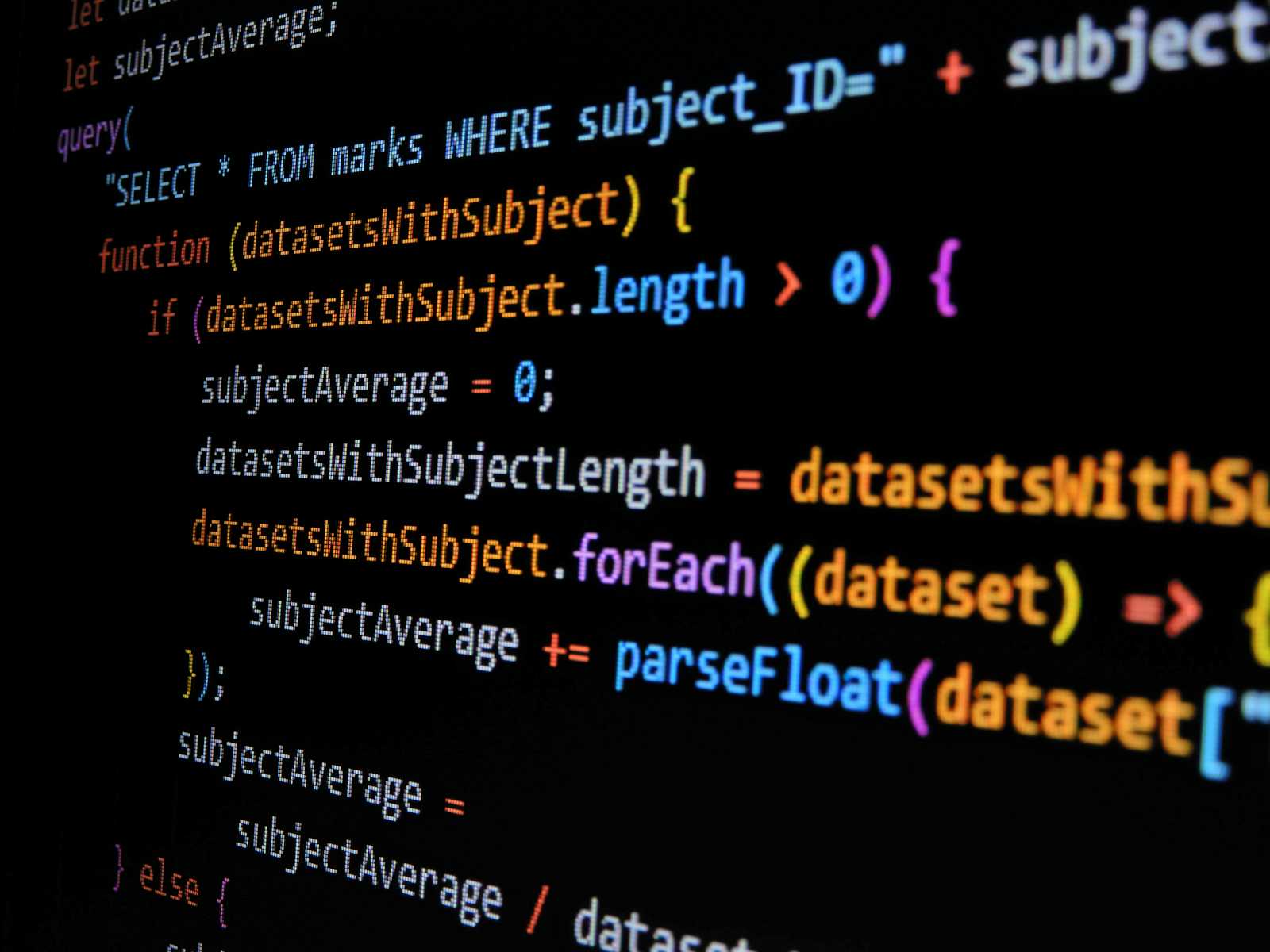
Developers often face the scenario where they deal with a substantial JavaScript object crafted on the front-end. But, before sending it to a REST endpoint, they aim to ensure its validity through validation. Typically, this is done by logging the object on the browser console and prettifying it. Let's understand this with an example
Example
Consider a case where you have a javascript object with a decent number of properties
let employee = {
firstName: "Nirav",
middleName: "Manish",
lastName: "Soni",
birthDate: new Date("January 1, 1982"),
department: "Engineering",
numYearsEmployment: 7,
isActive: true,
salary: 100000,
joiningDate: new Date("December 1, 2016"),
annualLeaves: 30,
vacationLeaves: 30,
isEligibleForESOP: true,
isRemote: true
}
So, to convert it to JSON, the easiest way is to use the JSON.stringify method
Let's say when we execute this method against this employee object that we've defined at the top, we see this output
// Convert to JSON string
console.log(JSON.stringify(employee));
Output
{"firstName":"Nirav","middleName":"Manish","lastName":"Soni","birthDate":"1981-12-31T18:30:00.000Z","department":"Engineering","numYearsEmployment":7,"isActive":true,"salary":100000,"joiningDate":"2016-11-30T18:30:00.000Z","annualLeaves":30,"vacationLeaves":30,"isEligibleForESOP":true,"isRemote":true}
This gets more and more ugly and un-readable as the number of properties within the object increases
Is there a better way to view this JSON? - YES!
There are multiple overloads of the stringify method - See below
JSON.stringify(value)
JSON.stringify(value, replacer)
JSON.stringify(value, replacer, space)
The one we can use to serve our purpose is JSON.stringify(value, replacer, space)
method
Testing the overloaded method
console.log(JSON.stringify(employee, null, 2));
Output
{
"firstName": "Nirav",
"middleName": "Manish",
"lastName": "Soni",
"birthDate": "1981-12-31T18:30:00.000Z",
"department": "Engineering",
"numYearsEmployment": 7,
"isActive": true,
"salary": 100000,
"joiningDate": "2016-11-30T18:30:00.000Z",
"annualLeaves": 30,
"vacationLeaves": 30,
"isEligibleForESOP": true,
"isRemote": true
}
And now, we have a neat pretty looking formatted JSON string which is much easier to read and interpret!
Subscribe to my newsletter
Read articles from Nirav Soni directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
Nirav Soni
Nirav Soni
Constantly evolving is what makes me tick! I am a life long learner, self-motivated individual with proven leadership qualities in 8 years of experience in designing and developing software for different domains primarily using .NET stack. Throughout my career, I've had privilege to work with clients and companies of diverse nature be it by size, culture and the domain they operate in. I have a solid experience in requirement gathering, analysis, architecting and developing cloud based applications. I am a quick learner with exceptional communication skills committed to deliver timely and quality software coupled with positive attitude and team spirit. I constantly keep on educating, refining and driving myself to constantly be better at what I do I am constantly learning because I never settle I stay calm in adverse situations I focus on high-quality decisions I have worked on software with variety of domains such as People Mobility Management Healthcare BFSI EdTech I enjoy meeting and getting to know people and hearing about perspective. Reach out to me if you want to talk to me about emerging trends of software development or tech in general. Professional Strengths : Software Development | Requirement Gathering | Software Architecture | Agile Development | Product Grooming | SaaS My ToolKit : C# | .NET Core | WebAPI | SQL | Docker | Kubernetes | Serverless | Cloud (Azure, AWS) | DevOps | Terraform