Level Up Your App's Real-Time Magic with Laravel Broadcasting
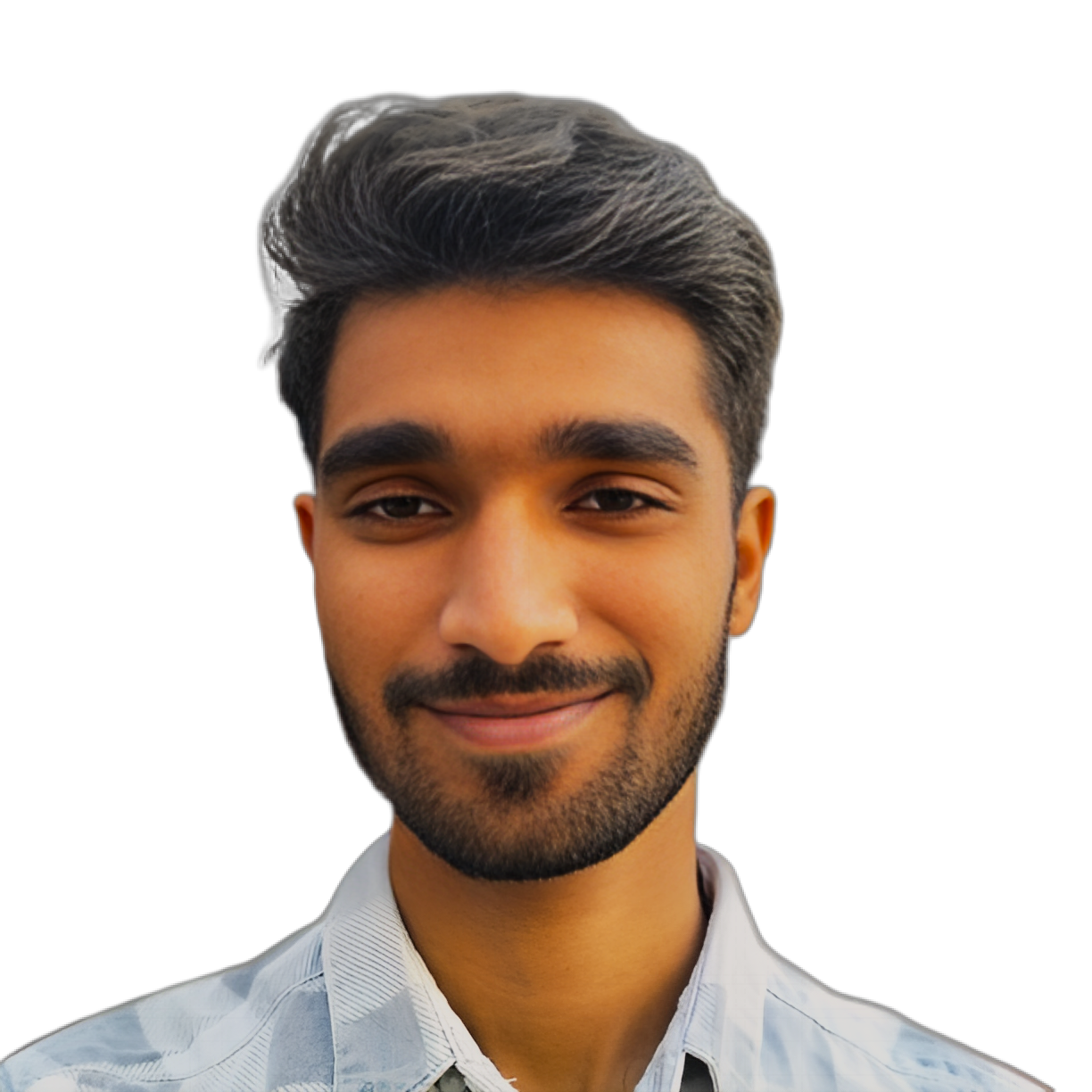
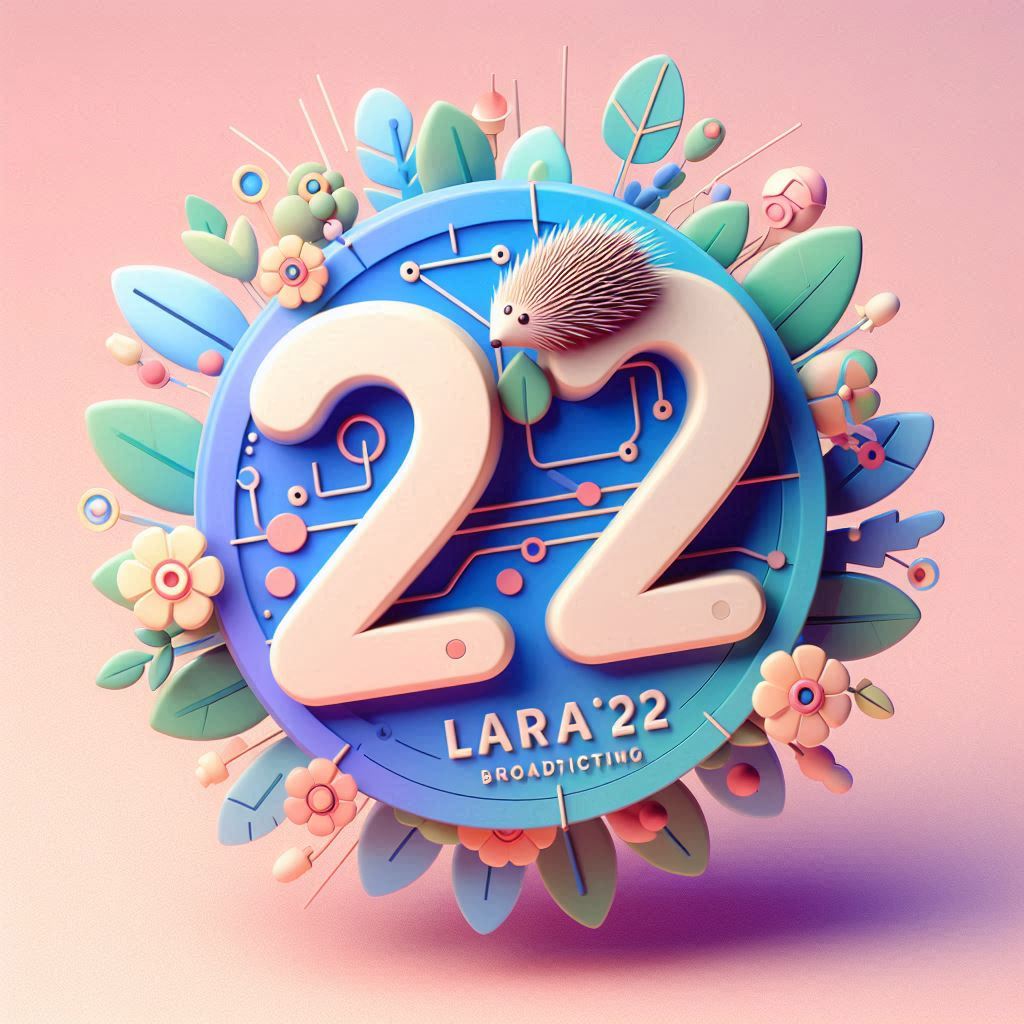
In today's fast-paced world, users expect web applications to be dynamic and responsive. Constant page refreshes can be a frustrating experience. Laravel Broadcasting provides a powerful solution for real-time updates in your Laravel application, enhancing user engagement and interactivity.
Real-World Examples of Broadcasting:
Live chat applications (e.g., Facebook Messenger)
Stock tickers displaying real-time market data
Collaborative editing tools (e.g., Google Docs)
Social media feeds with instant updates
Under the Hood: How Broadcasting Works
Laravel Broadcasting leverages WebSockets, a technology that enables persistent, two-way communication between the browser and server. Events are broadcasted over designated channels, allowing subscribed clients to receive the data in real-time. Choose your preferred driver (Pusher, Redis, etc.) to manage the real-time communication infrastructure.
Step-by-Step Tutorial:
Configure Broadcasting:
Open your
.env
file and configure the broadcasting driver you'll use. For example, to use Redis:BROADCAST_DRIVER=redis CACHE_DRIVER=redis QUEUE_CONNECTION=redis
If using a driver like Pusher, obtain your credentials from their website and set the corresponding environment variables (
PUSHER_APP_ID
,PUSHER_APP_KEY
,PUSHER_APP_SECRET
, andPUSHER_APP_CLUSTER
).
Create an Event:
Use the
php artisan make:event
command to generate a new event class. For example, to create an event for sending new messages:Bash
php artisan make:event NewMessageEvent
Implement the
Illuminate\Contracts\Broadcasting\ShouldBroadcast
interface in your event class. This enables broadcasting for the event.
Define Broadcast Channel:
In your event class, specify the channel(s) over which the event should be broadcasted. Use the
broadcastOn
method and return the appropriate channel object(s). For example, to broadcast theNewMessageEvent
to a channel specific to a room:PHP
class NewMessageEvent implements ShouldBroadcast { use Dispatchable, InteractsWithQueue, SerializesModels; public $message; public function __construct($message) { $this->message = $message; } public function broadcastOn(): array { return new PresenceChannel('chat-room.' . $roomId); // Replace with your actual room ID } }
Dispatching the Event:
In your code where you want to broadcast an event, use the
Broadcast::channel
facade to dispatch it on the appropriate channel(s). For example, when a new message is created:PHP
Broadcast::channel('chat-room.' . $roomId, new NewMessageEvent($message));
Client-Side Setup (Using Laravel Echo):
Install the
laravel/echo
package using Composer:Bash
composer require laravel/echo
Include the Echo JavaScript library in your blade template:
HTML
<script src="{{ asset('js/app.js') }}"></script> // Assuming your compiled assets are in the 'js' directory
In your JavaScript code, create an Echo instance using your broadcasting driver's configuration (e.g., Pusher credentials):
JavaScript
import Echo from 'laravel-echo'; window.Echo = new Echo({ broadcaster: 'pusher', // Replace with your actual driver key: process.env.MIX_PUSHER_APP_KEY, cluster: process.env.MIX_PUSHER_APP_CLUSTER, forceTLS: true });
Listen for the broadcasted event on the corresponding channel and handle the received data:
JavaScript
Echo.channel('chat-room.' . roomId) .listen('NewMessageEvent', (e) => { // Update your UI with the received message data (e.g., display the new message) });
Additional Considerations:
Choose the appropriate broadcasting driver based on your application's requirements and scalability needs.
For private channels that require authentication, implement authorization logic in your event class using the
authorizes
Subscribe to my newsletter
Read articles from Asis Sharma directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
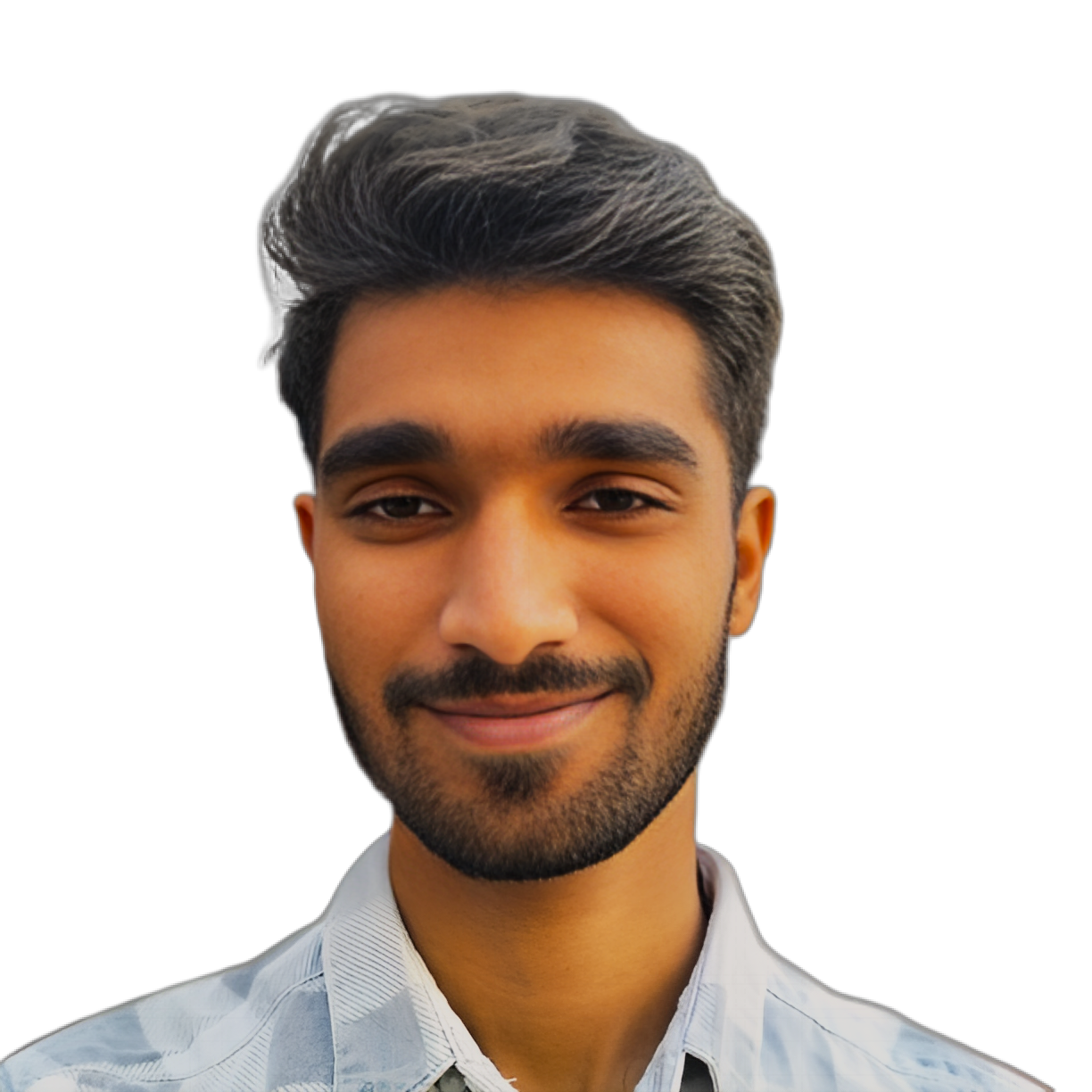
Asis Sharma
Asis Sharma
I'm an ambitious and motivated software developer, eager to apply my knowledge and skills to contribute to the development of innovative and cutting-edge software solutions. I'm well-versed in a variety of programming languages, including Javascript, C++, and Node.js, and have a understanding of object-oriented programming and algorithms. I'm passionate about developing and I am eager to learn and collaborate with others.