Day 66 - Terraform Hands-on Project - Build Your Own AWS Infrastructure with Ease using Infrastructure as Code (IaC)

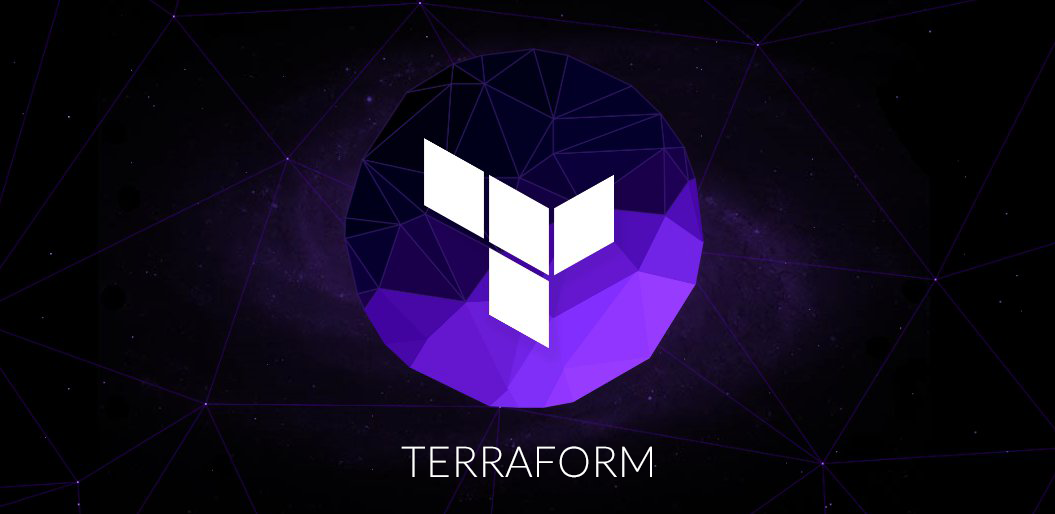
Task:
1.Create a VPC (Virtual Private Cloud) with CIDR block 10.0.0.0/16
resource "aws_vpc" "main" {
cidr_block = "10.0.0.0/16"
tags = {
Name = "main"
}
}
This will create a new VPC in your AWS account with the specified CIDR block and a name tag of "main".
The terraform block defines the version of Terraform that is required to execute this configuration. In this case, it specifies that the Terraform version must be >= 1.2.0. also Add the region where you want your instances to be.
Run the terraform init command to initialize the working directory and download the required providers. After that, run terraform apply to create the VPC in your AWS account.
Go to VPC console and check new VPC with name 'main' is successfully created.
2. Create a public subnet with CIDR block 10.0.1.0/24 in the above VPC.
To create a public subnet with CIDR block 10.0.1.0/24 in the VPC that you created in the previous step, you can use the following Terraform code:
resource "aws_subnet" "public_subnet" {
vpc_id = aws_vpc.main.id
cidr_block = "10.0.1.0/24"
tags = {
Name = "Public Subnet"
}
}
Run terraform apply to create public subnet in your AWS account.
Go to VPC console then go to Subnets.
Check "Public Subnet" is created successfully.
3. Create a private subnet with CIDR block 10.0.2.0/24 in the above VPC.
This Terraform code creates a private subnet with CIDR block 10.0.2.0/24 in the VPC with ID aws_vpc.main.id, and tags it with the name "Private Subnet".
resource "aws_subnet" "private_subnet" {
vpc_id = aws_vpc.main.id
cidr_block = "10.0.2.0/24"
tags = {
Name = "Private Subnet"
}
}
Terraform apply-
'Private Subnet' is successfully created using terraform
4. Create an Internet Gateway (IGW) and attach it to the VPC.
resource "aws_internet_gateway" "gw" {
vpc_id = aws_vpc.main.id
tags = {
Name = "igw"
}
}
This Terraform code creates a internet gateway in the VPC with ID aws_vpc.main.id, and tags it with the name "igw".
terraform apply-
Go to VPC then go to Internet gateways
Check new internet gateway is created with name 'igw'.
VPC is attached to the internet gateway.
5. Create a route table for the public subnet and associate it with the public subnet. This route table should have a route to the Internet Gateway.
resource "aws_route_table" "public" {
vpc_id = aws_vpc.main.id
route {
cidr_block = "0.0.0.0/0"
gateway_id = aws_internet_gateway.gw.id
}
tags = {
Name = "route-table"
}
}
resource "aws_route_table_association" "public" {
subnet_id = aws_subnet.public_subnet.id
route_table_id = aws_route_table.public.id
}
First create a route table for public subnet.
aws_route_table block creates a new route table in the VPC specified by vpc_id attribute. It also defines a route that sends all traffic with destination CIDR 0.0.0.0/0 to the internet gateway specified by gateway_id attribute. The tags attribute sets a name for the route table for easy identification.
Then associate route table with public subnet.
aws_route_table_association block associates the newly created route table with a public subnet specified by the subnet_id attribute. The route_table_id attribute refers to the ID of the route table created in the previous block.
In Route tables, new route table is successfully created.
Route table routes with internet gateway.
Route table is associate with public subnet using terraform.
6. Launch an EC2 instance in the public subnet with the following details:
AMI
Instance type: t2.micro
aws_instance block creates a new EC2 instance in the public subnet specified by subnet_id attribute. It uses the Amazon Machine Image (AMI) ID ami-0ddda618e961f2270, which is a Ubuntu 18.04 LTS image. The key_name attribute specifies the name of the key pair used to SSH into the instance. The vpc_security_group_ids attribute specifies a list of security groups to attach to the instance, in this case, it refers to the ID of the security group created in the next block.
resource "aws_instance" "web_server" {
ami = "ami-0ddda618e961f2270"
instance_type = "t2.micro"
key_name = "batch-6-key"
subnet_id = aws_subnet.public_subnet.id
vpc_security_group_ids = [
aws_security_group.ssh_access.id
]
Security group: Allow SSH access from anywhere
aws_security_group block creates a new security group that allows inbound traffic on ports 22 (SSH) and 80 (HTTP) from any source (0.0.0.0/0). The name_prefix attribute sets a name prefix for the security group, and the vpc_id attribute specifies the ID of the VPC where the security group will be created.
resource "aws_security_group" "ssh_access" {
name_prefix = "ssh_access"
vpc_id = aws_vpc.main.id
ingress {
from_port = 22
to_port = 22
protocol = "tcp"
cidr_blocks = ["0.0.0.0/0"]
}
ingress {
from_port = 80
to_port = 80
protocol = "tcp"
cidr_blocks = ["0.0.0.0/0"]
}
}
Security group is created with SSH(Port 22) and HTTP(Port 80) access.
User data: Use a shell script to install Apache and host a simple website
The user_data attribute specifies the script to run when the instance is launched. This script updates the package manager, installs Apache web server, creates a basic HTML file, and restarts Apache.
user_data = <<-EOF
#!/bin/bash
sudo apt-get update -y
sudo apt-get install apache2 -y
sudo systemctl start apache2
sudo systemctl enable apache2
echo "<html><body><h1>Welcome to my website!</h1></body></html>" > /var/www/html/index.html
sudo systemctl restart apache2
EOF
Create an Elastic IP and associate it with the EC2 instance.
aws_eip block creates a new Elastic IP address and associates it with the instance created in the first block by specifying the instance ID in the instance attribute. The tags attribute sets a name for the Elastic IP for easy identification.
resource "aws_eip" "eip" {
instance = aws_instance.web_server.id
tags = {
Name = "test-eip"
}
}
Elastic IP is successfully created.
Terraform main.tf file for creating EC2 instance in the public subnet.
main.tf file
Run terraform apply to create the EC2 instance in your AWS account.
New instance is created (terraform-instance) using terraform.
7. Open the website URL in a browser to verify that the website is hosted successfully.
Thank you for reading!
Subscribe to my newsletter
Read articles from Nikhil Yadav directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by

Nikhil Yadav
Nikhil Yadav
I am a highly motivated and enthusiastic individual completed B.Tech from Savitribai Phule University, Pune . With a strong interest in DevOps and Cloud technologies, I am eager to kick-start my career in this domain. Although I do not have much professional experience, I possess a willingness to learn, excellent problem-solving skills, and a passion for technology. I am committed to contributing my best to any team I work with.