Must-Have Resources for Building a Game Boy Emulator

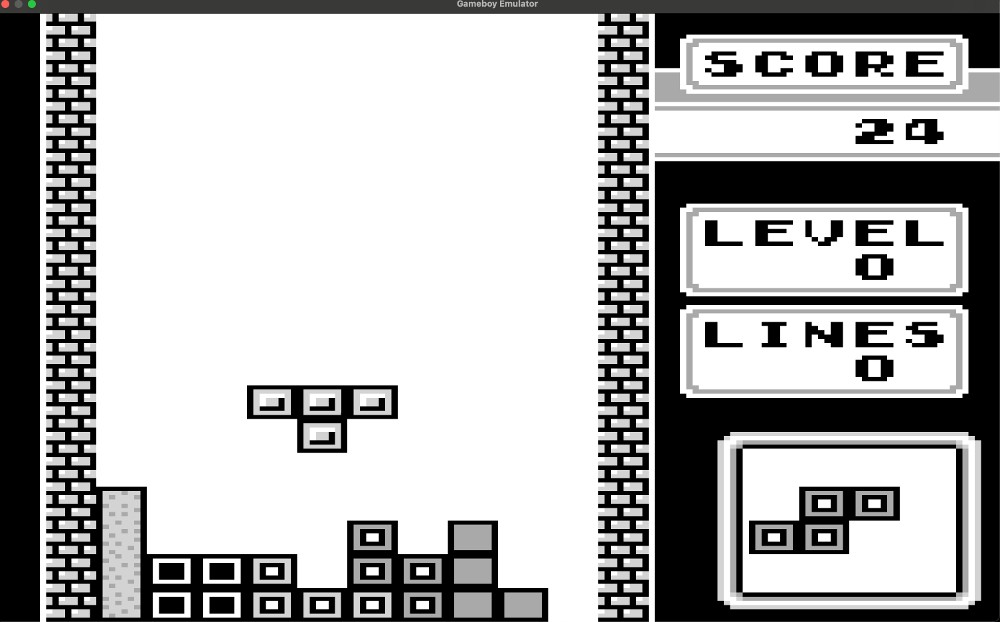
I have vivid memories of playing games on my Game Boy Color as a child, the most memorable being Pokemon Red. Eventually, I played around with Gameshark codes that would allow me to hack certain aspects of games so I could cheat, or cause weird visual effects and glitches. Later, I downloaded Visual Boy Advanced on my computer and started playing Game Boy games on it. I also messed around with software that would allow me to create my own ROM hacks.
I was always fascinated by how the Game Boy worked internally; it felt like magic to me back then. After becoming a professional software engineer, I pursued this curiosity and delved into the realm of game system emulation: creating software that mimics the internal mechanisms of various game systems.
When I first decided to start building my own Game Boy Emulator (after making a CHIP-8 Emulator as a beginner project: https://github.com/smparsons/lazy-chip8), I found many resources that helped me create a functional CPU and GPU. In this blog post, I wanted to cover some of these resources to benefit other developers on a similar journey.
Game Boy CPU Manual
I want to preface this first suggestion by saying that this manual is well known for having a few mistakes.
That being said, it's documentation on CPU instructions was still quite helpful, and guided me towards making a working CPU fairly quickly. When I was building my CPU, I would consult this manual and work through each opcode one by one, doing little by little with the small amount of free time I had. If you decide to use this manual, my suggestion would be to couple this with the opcode table provided by Pan Docs, which provides more accurate information on instruction clock cycles and affected registers for any given instruction.
Pan Docs
Pan Docs is a large collection of comprehensive documentation on the Game Boy. If you need to learn anything about how the Game Boy works, this is the first place you should look.
I was especially impressed with its detailed information on the GPU. It covers proper GPU timings, rendering background/window/sprites, the functionality of each LCDC register flag, scrolling behavior, palettes, and even compares the GPU behavior of the original monochrome Game Boy and the Game Boy Color. When I built my GPU, for the most part I only used this documentation as a guide to push me in the right direction.
Test ROMs
Link to Blargg's Test ROM Collection
Test ROMs can be helpful for verifying that your emulator is functioning correctly. One of the most notable test ROM collections is Blargg's test ROMs, which require at the very least a minimally functioning CPU and GPU to run them. Another notable collection of test ROMs is the Mooneye Test Suite, which includes GPU tests. I have not tried using any of the tools provided by the Mooneye Test Suite for my emulator yet, although I plan to eventually.
Blargg's test ROMs were a pivotal tool in allowing me to finally get my emulator to run Tetris without crashing. Without it, I would have had to review each of my opcode implementations one by one and make sure they are functioning as expected, which would have been slow and tedious. With it, I could work much quicker and immediately find out which opcodes are failing.
Load up the main CPU instructions test ROM, and the display will show you which tests are passing and which are failing. The ones that are failing will show an error code. For example:
Then, it's a matter of running the individual test ROM that has failing tests to see which opcodes are malfunctioning. For example, in the above summary, test 11 is returning an error code, so I loaded the individual test ROM for 11 and saw this output:
Which told me that opcodes 8E, 9E, and CB 36 are not functioning correctly.
While these tests do point to which opcodes are failing, they do not tell you why they are failing. However there's one more additional tool that can provide that missing information which can speed debugging along even further, and that's Game Boy Doctor.
Game Boy Doctor
Link to Game Boy Doctor GitHub Repo
Game Boy Doctor is a simple Python script that compares the state of your CPU with the state of a CPU for an emulator that passes all of Blargg's CPU instruction tests.
The setup process is simple, and documented in the repo's README file. It's just a matter of setting the state of your CPU registers to the state they would be after executing the Game Boy BIOS. Then, you need to output the state of your CPU registers to a log file in the format described in the README, and run one of Blargg's test ROMs.
If you pass the generated log file to Game Boy Doctor, it will tell you where the state of your CPU diverges with the state of a CPU that passes all opcode tests.
This will clearly pinpoint the issue, and save you hours of debugging time.
Imran Nazar's Series on Game Boy Emulator Development
This series was a fantastic overview of all of the different components that make up a Game Boy Emulator. His tutorial covers how to write a Game Boy Emulator using Javascript, however you don't have to be writing a Javascript emulator to benefit from the tutorial.
Each part of the series dives in depth into a particular topic, using easy to follow code examples, diagrams, and clear explanations of different components. After reading his series, you will understand how the Game Boy works at a much deeper level.
His series covers the main core components of a Game Boy Emulator, including the CPU, MMU, GPU, interrupts, timers, and accepting keyboard input. Unfortunately, his series does not have a section on audio.
Other Emulators
This wouldn't be my first choice as it can suck the fun out of trying to figure out how to do it yourself, but if you're really stuck, I would also suggest looking at the source code of other working emulators. Sometimes it can be enlightening to see how others approach solving the same problems. If you're looking at other emulators to help you with your CPU, make sure the emulator passes all of Blargg's CPU instruction tests. Typically the developer will mention that on the README.
I would also encourage you to not only look at the code, but also understand how it works. Try to understand why the developer made certain decisions so you can learn from it.
One Final Suggestion
I also wanted to mention that you will hugely benefit by developing your emulator with a Test Driven Development (TDD) approach. What do I mean by that?
I am not going to go into the nitty gritty details of TDD in this blog post, as there are tons of resources online where you can learn about that. But at its simplest, TDD just means writing a failing unit test first that will exercise a small unit of functionality. After you watch the test fail, you write code that will cause the test to pass. Rinse and repeat.
For the CPU, this is a must-have approach to avoid stupid mistakes when implementing opcodes, and also helps you design your CPU in such a way that its loosely coupled and easily testable. When I worked on my CPU, I wrote one unit test per opcode for the most part (some groups of opcodes have the exact same logic but operate on different registers). I would refer to the Game Boy CPU Manual and other resources to get a basic idea of how an opcode should work, write a unit test for it, then implement the opcode so the unit test passes.
It is also helpful for writing your GPU, as you can setup your test by setting certain LCDC register flags, setting test data in OAM and VRAM memory, then calling your function to write a scanline to the frame buffer and checking that the scanline contains the correct bytes.
Conclusion
I hope this article helped push you in the right direction. Enjoy building your Game Boy Emulator. It will teach you a lot about low level programming, and being able to play games on it in the end is super satisfying.
Subscribe to my newsletter
Read articles from Samuel Parsons directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
