Ensure code quality with help of static code analyzer and code coverage

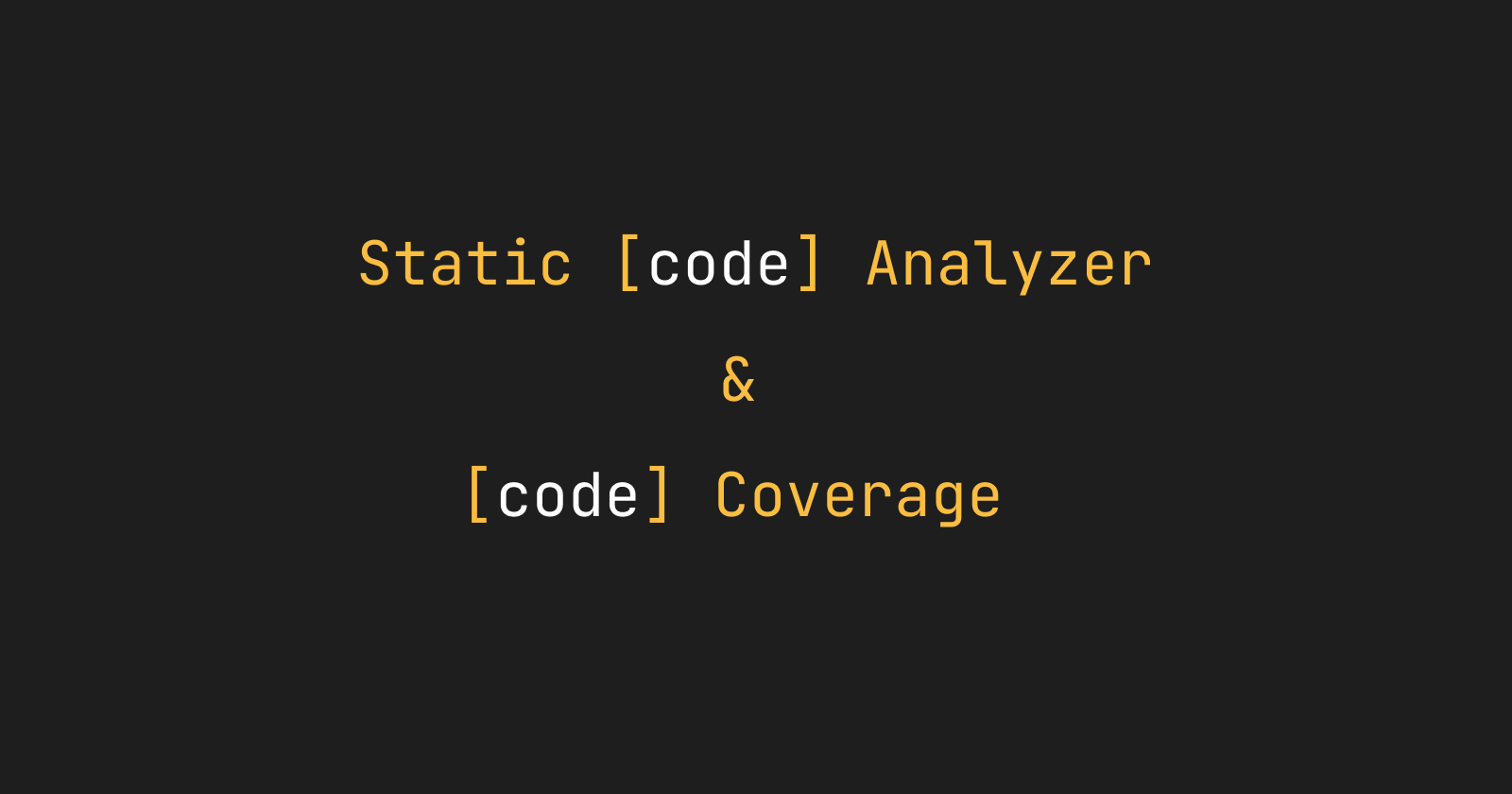
When we develop software we want to deliver best quality of code, so every line of code we write is matter, because its carries the potential of errors that can disturb business flow of our software. If you work alone on the small project maintaining code base may seems easy, because you decide your standard and things that can goes wrong relatively small.
Problems appear when your project getting bigger and team members growing, every member of the team had different style of writing code and then now you need to make sure a lot of things worked as the team expected. Now we need to make standard and design rules that all member needs follow to ensure quality and consistent code base that help us to deliver maintainable and reliable application. Design rules cover a lot of things, edge to edge, even as simple as how to using comma and spacing, for example in java:
//Person A write:
public void methodA() {
...
}
public void methodB() {
...
}
//Person B write:
public void methodA() {
...
}
public void methodB() {
...
}
Can you point out the difference between person A and person B code? Yes, its on the blank lines between methods, so which one is better? Preferences of course, but when we ask which one is following design rules of code conventions? We can agree that person B follow this code conventions. Other than this code conventions, we had a lot design rules to follow, like naming conventions, code smells, if-else statement merging, code complexity and much more. Sound a lot to ensure our code follow all the design rules? Then static code analyzer comes to help!
Static code analyzer is a tool that help us to analyze and review our code against standard and design rules, so we can detect malicious code, bad use of standard, guarantee the quality on our code before execution.
while static code analyzer guarantee the quality on our code before execution, code coverage had difference approach. Code coverage check how much of the code is tested. It shows how many parts of the code are used when tests are run. For example:
class Groceries {
public string getType(int type) {
switch(type) {
case 1:
return "vegetables";
break;
case 2:
return "fruit";
break;
case 3:
return "snack";
break;
default:
return "unknown";
break;
}
}
}
@Test
void testTypeOfGroceries() {
Groceries groceries = new Groceries();
assertEquals("fruit", groceries.getType(1));
}
Because on our test case, we only cover the test against 1 type of groceries and cannot ensure that if getType()
function on other type of groceries doesn't goes wrong. With help of code coverage developers can see which parts of the code aren't tested enough, they can decide where to focus their testing. This makes sure that important parts of the code get enough attention.
Let's Wrap Up
When developing software, following design rules and standards is crucial for maintaining code quality and consistency. Static code analyzers help ensure adherence to these rules, while code coverage tools help identify areas of code that need more testing.
That's all, Thanks for reading this far ✌️. I'm eager to hear your thoughts about Static Code Analyzer and Code Coverage.
Subscribe to my newsletter
Read articles from Kadek Dhiva Tiradika directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
