Taming Form Chaos: Mastering Laravel Form Requests
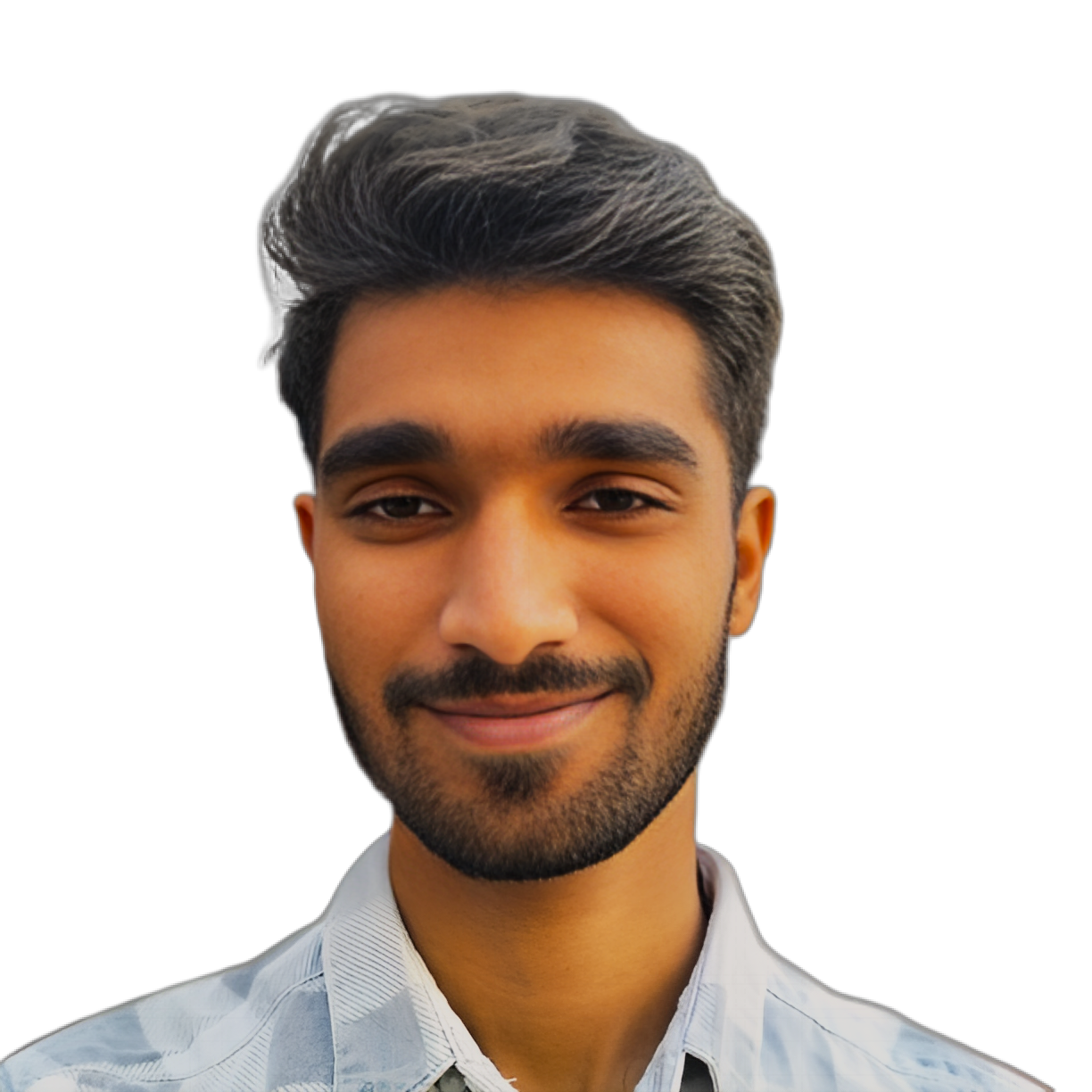
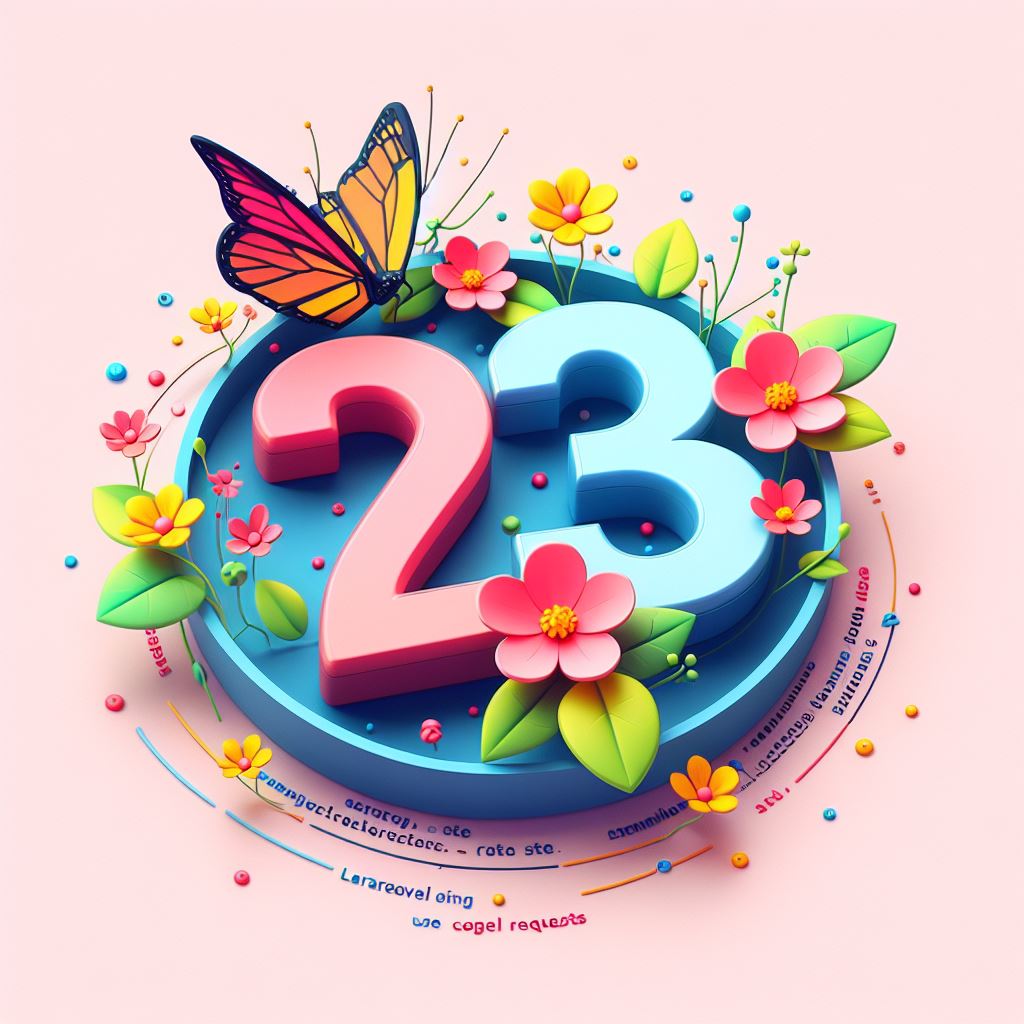
Ever felt overwhelmed by messy form validation code scattered across your Laravel controllers? Do you crave a cleaner, more organized approach to handling form data? Look no further than Laravel Form Requests, a powerful feature that streamlines the validation process and keeps your codebase sparkling. In this blog post, we'll not only explain what Form Requests are, but also provide a step-by-step guide to using them effectively.
What are Laravel Form Requests?
Imagine a world where validation logic doesn't pollute your controllers. A world where validation rules reside in a centralized location, promoting code organization and separation of concerns. This utopian vision becomes reality with Laravel Form Requests. These are dedicated classes specifically designed to validate form data. By defining validation rules within these classes, you decouple validation logic from your controllers, keeping your code clean and maintainable.
Benefits of Using Form Requests
Improved Code Organization: Separate validation logic from controllers, leading to a more focused and readable codebase.
Reduced Boilerplate Code: Eliminate repetitive validation code and streamline your development process.
Enhanced Maintainability: Easily modify validation rules in a central location, improving code maintainability.
Automatic Data Retrieval: Access validated data directly after successful validation for a smoother development experience.
Customizable Error Messages: Craft informative error messages to provide clear feedback to users.
Authorization Integration: Implement authorization checks to restrict form access based on user roles or permissions.
Reusable Validation Logic: Share validation logic across different controllers, promoting code reusability.
A Step-by-Step Tutorial: Creating Your First Form Request
Let's create a basic Form Request for validating a new blog post submission. Here's a breakdown of the process:
- Generate the Form Request Class:
Use the Laravel Artisan command to generate a new form request class:
Bash
php artisan make:request StorePostRequest
This command creates a class named StorePostRequest
within the App\Http\Requests
directory.
- Define Validation Rules:
Open the newly created StorePostRequest
class and define the validation rules within the rules
method:
PHP
<?php
namespace App\Http\Requests;
use Illuminate\Foundation\Http\FormRequest;
class StorePostRequest extends FormRequest
{
/**
* Determine if the user is authorized to make this request.
*
* @return bool
*/
public function authorize()
{
return true; // Change this to implement authorization logic if needed
}
/**
* Get the validation rules that apply to the request.
*
* @return array
*/
public function rules()
{
return [
'title' => 'required|string|max:255',
'body' => 'required|string',
];
}
}
In this example, the rules
method defines the validation rules for the title
and body
fields. The title
field is required, must be a string, and has a maximum length of 255 characters. Similarly, the body
field is also required to be a string.
- Utilize the Form Request in Your Controller:
Within your controller method handling the form submission, use the validate
method to leverage the form request:
PHP
public function store(StorePostRequest $request)
{
$validatedData = $request->validated();
// Process the validated form data (e.g., create a new post)
return redirect()->route('posts.index')->with('success', 'Post created successfully!');
}
The validate
method automatically validates the incoming form data based on the rules defined in the StorePostRequest
class. Additionally, you can access the validated data directly using the validated
method after successful validation.
Unleash the Power of Form Requests
Laravel Form Requests offer a powerful and elegant approach to form validation, promoting code organization, maintainability, and a better user experience. By embracing Form Requests, you'll write cleaner code, reduce boilerplate, and simplify your development workflow. So, the next time you encounter a form in your Laravel project, remember the power of Form Requests – they're here to tame the chaos and keep your codebase singing!
Subscribe to my newsletter
Read articles from Asis Sharma directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
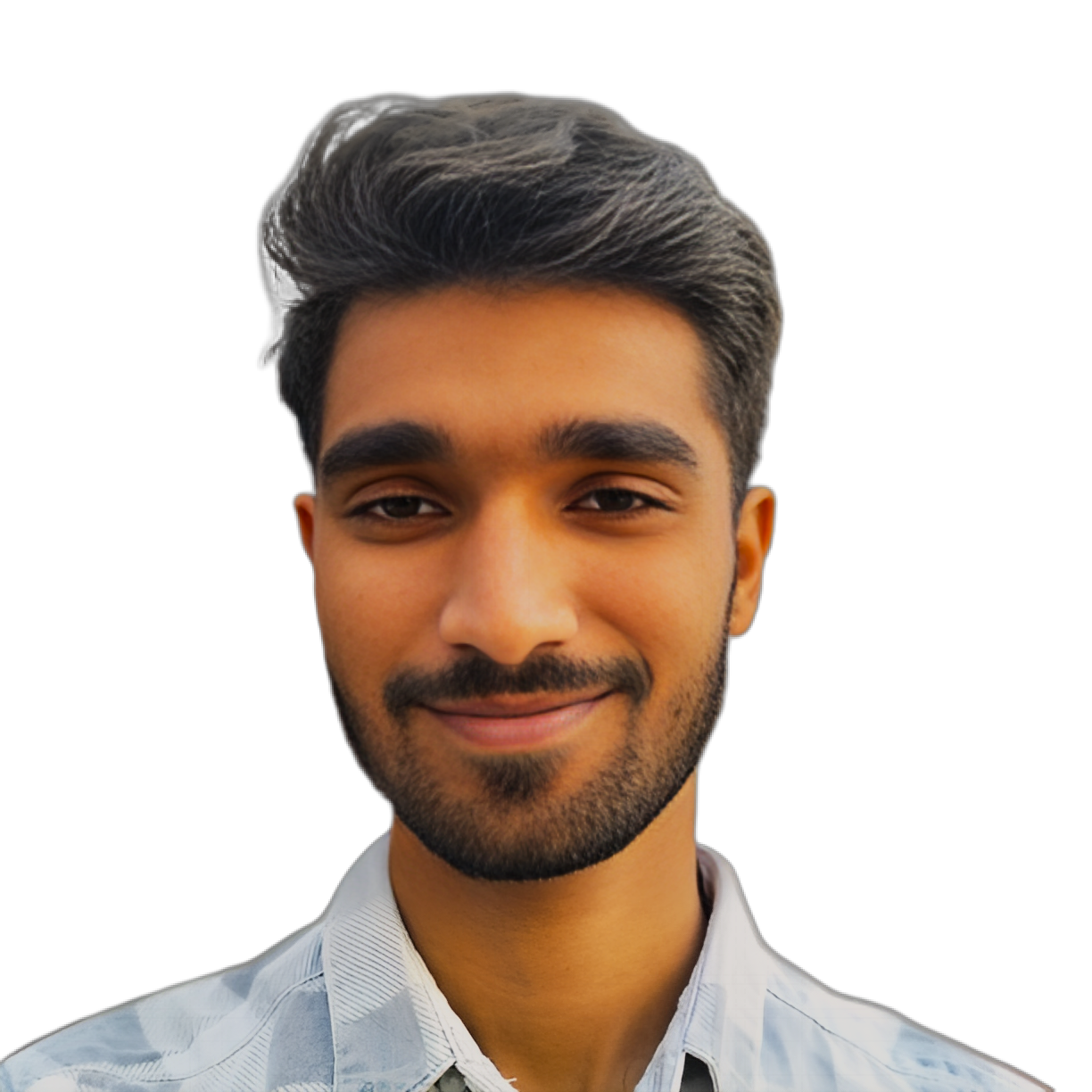
Asis Sharma
Asis Sharma
I'm an ambitious and motivated software developer, eager to apply my knowledge and skills to contribute to the development of innovative and cutting-edge software solutions. I'm well-versed in a variety of programming languages, including Javascript, C++, and Node.js, and have a understanding of object-oriented programming and algorithms. I'm passionate about developing and I am eager to learn and collaborate with others.