Project Trouble Shooting 2 : Postman URL Errors (https://, http://) and 'Http method names must be tokens' from console
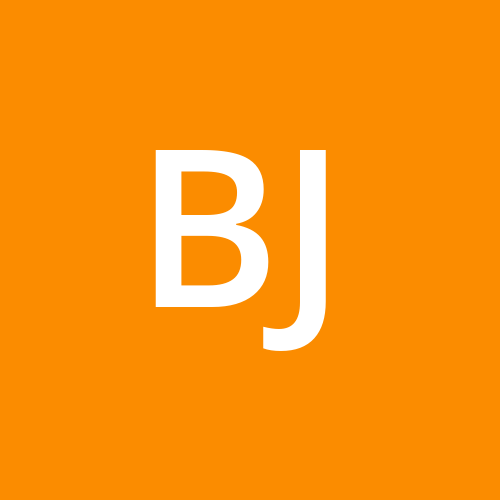
#Foreword
Now I am participating in a team project, although I haven't yet properly started. As I mentioned before, we are only two members. I am preparing for a proper launch by starting to keep a record of my progress, especially when encountering errors or difficulties. Even if it's not a long document, it will be succint and helpful for future reference.
Run Application (SignUp - User)
:
Sending Request using postman
Error Messages on Console
Http method names must be tokens?
: This issue is typically related to HTTP request handling and may be occurring due to incorrect configuration or misuse of annotations.
SignUp Source Code
@RestController
@RequestMapping("/user")
@RequiredArgsConstructor
public class UserController {
private final UserService userService;
@PostMapping
public ResponseEntity<ResponseDTO<SignUpResponse>> createSignup(
@RequestBody @Valid SignUpRequest signupRequest
) {
SignUpResponse signUpResponse = userService.signUp(signupRequest);
return ResponseEntity.ok(ResponseDTO.okWithData(signUpResponse));
}
}
--------------------
public record SignUpRequest(
@Email(message = "email의 형식이 잘못됐습니다.")
@NotNull(message = "email은 필수값입니다.")
String email,
@Size(min = 6, message = "password는 6자 이상이어야 합니다.")
@NotNull(message = "password는 필수값입니다.")
String password
) {
public User toEntity(String encryptedPassword) {
return User.builder()
.email(email)
.encryptedPassword(encryptedPassword)
.build();
}
}
--------------------
public record SignUpResponse(
Long id,
String email
) {
public static SignUpResponse from(User user) {
return new SignUpResponse(
user.getId(),
user.getEmail()
);
}
}
--------------------
@Service
@RequiredArgsConstructor
@Transactional(readOnly = true)
public class UserService {
private final UserRepository userRepository;
@Transactional
public SignUpResponse signUp(SignUpRequest signUpRequest) {
if (userRepository.existsByEmail(signUpRequest.email())) {
throw new UserAlreadyRegisteredException();
}
User user = signUpRequest.toEntity(signUpRequest.password());
User savedUser = userRepository.save(user);
return SignUpResponse.from(savedUser);
}
}
-------------------------
@Repository
public interface UserRepository extends JpaRepository<User, Long> {
boolean existsByEmail(String email);
}
----------------------------
@Entity
@Table(name = "users")
@NoArgsConstructor
@Getter
public class User extends BaseTimeEntity {
@Id
@GeneratedValue(strategy = GenerationType.IDENTITY)
private Long id;
@Column(unique = true)
private String email;
private String encryptedPassword;
@Builder
public User(String email, String encryptedPassword) {
this.email = email;
this.encryptedPassword = encryptedPassword;
}
}
Why did these errors occur?
: Before sending a request on postman, the Tomcat server ran smoothly. I initially suspected an error in my code and spent some time to rebuild it, particularly focusing on the HTTP request handling. However, Surprisingly, the issue turned out to be related to URL : Https:// , Http://
Furthermore, I attempted to adjust Postman settings, as shown in the image below, but it didn't work.
SSL certificate verification was off, so I checked it to make it 'on', but it wasn't a proper solution.
After I changed https:// -> http://
it works well, as shown in the image below
In conclusion,
This type of issue comes when you use 'https://' instead of 'http://' Change the URL to use Http
Example : https://localhost:8080/user
Change it to (solution) : http://localhost:8080/user
Subscribe to my newsletter
Read articles from Byung Joo Jeong directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
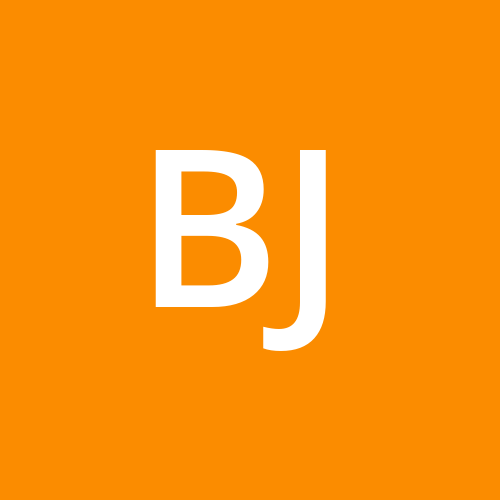