Exploring Binary Trees and Traversals in Python: Depth First Search approach

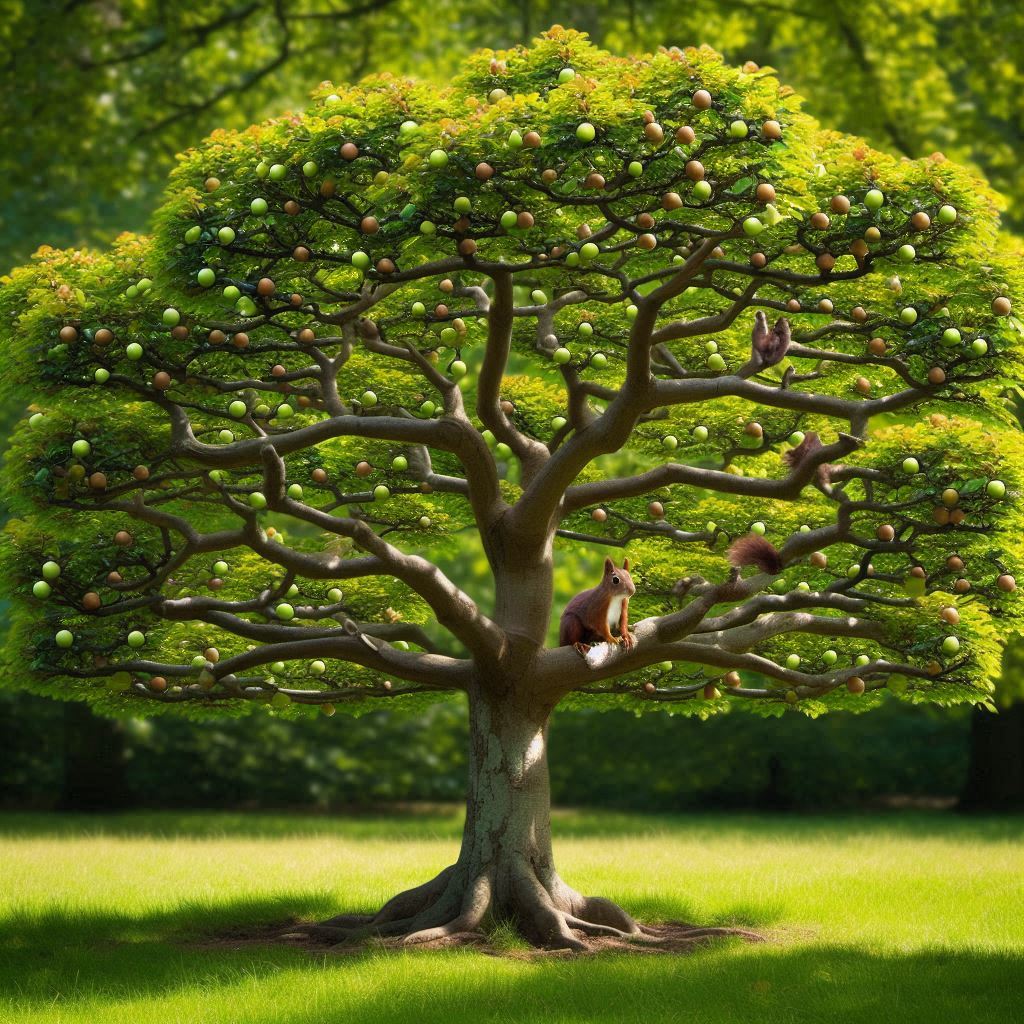
Binary trees are fundamental data structures in computer science and are widely used in various applications such as search algorithms, expression parsing, and more. A binary tree is a hierarchical data structure composed of nodes, where each node has at most two children, referred to as the left child and the right child. Understanding binary trees and their traversal algorithms is crucial for any programmer.
Depth-First Search (DFS) is a fundamental algorithm for traversing or searching tree or graph data structures. It starts at the root node and explores as far as possible along each branch before backtracking. In the context of binary trees, DFS involves systematically visiting nodes in a depthward motion, going deeper into the tree before exploring siblings. This traversal strategy is particularly useful for exploring solutions in problem-solving scenarios or for systematically examining elements in a hierarchical structure.
In this blog post, we'll delve into the basics of binary trees and explore three common depth-first search traversal methods: inorder, preorder, and postorder. We'll discuss how each traversal algorithm works and provide Python implementations for each. By the end of this post, you'll have a solid understanding of binary trees and depth-first search traversal techniques, empowering you to apply them in various programming challenges and real-world scenarios.
Let's dive in!
Let's start by defining a binary tree node and a binary tree class in Python:
For each algorithm, we will provide an example and the result of applying the alogorithm on the following binary tree:
Depth First Search Algorithms
Inorder Traversal: In inorder traversal, we visit the left subtree, then the root, and finally the right subtree recursively. This traversal method results in nodes being visited in ascending order in a binary search tree.
Applying an Inorder Traversal algorithm would generate the result below for the above binary tree:
Preorder Traversal: Preorder traversal visits the root node first, then the left subtree, and finally the right subtree recursively.
Applying an Preorder Traversal algorithm would generate the result below for the above binary tree:
Postorder Traversal: In postorder traversal, we visit the left subtree, then the right subtree, and finally the root recursively.
Applying an Postorder Traversal algorithm would generate the result below for the above binary tree:
To recap, we explored binary trees and three fundamental depth-first search traversal algorithms: inorder, preorder, and postorder. We began by defining binary trees and their nodes in Python, highlighting their hierarchical structure. Depth-first search (DFS) was introduced as a key algorithm for systematically traversing binary trees, starting from the root and exploring each branch in depth before backtracking. Each traversal method— inorder, preorder, and postorder— was explained with Python implementations and visualized on a simple binary tree. Through this exploration, we gained a solid understanding of binary tree traversal techniques, empowering us to apply these concepts in problem-solving scenarios and real-world programming challenges.
Subscribe to my newsletter
Read articles from Emeron Marcelle directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by

Emeron Marcelle
Emeron Marcelle
As a doctoral scholar in Information Technology, I am deeply immersed in the world of artificial intelligence, with a specific focus on advancing the field. Fueled by a strong passion for Machine Learning and Artificial Intelligence, I am dedicated to acquiring the skills necessary to drive growth and innovation in this dynamic field. With a commitment to continuous learning and a desire to contribute innovative ideas, I am on a path to make meaningful contributions to the ever-evolving landscape of Machine Learning.