Is Closure in JS really that complicated
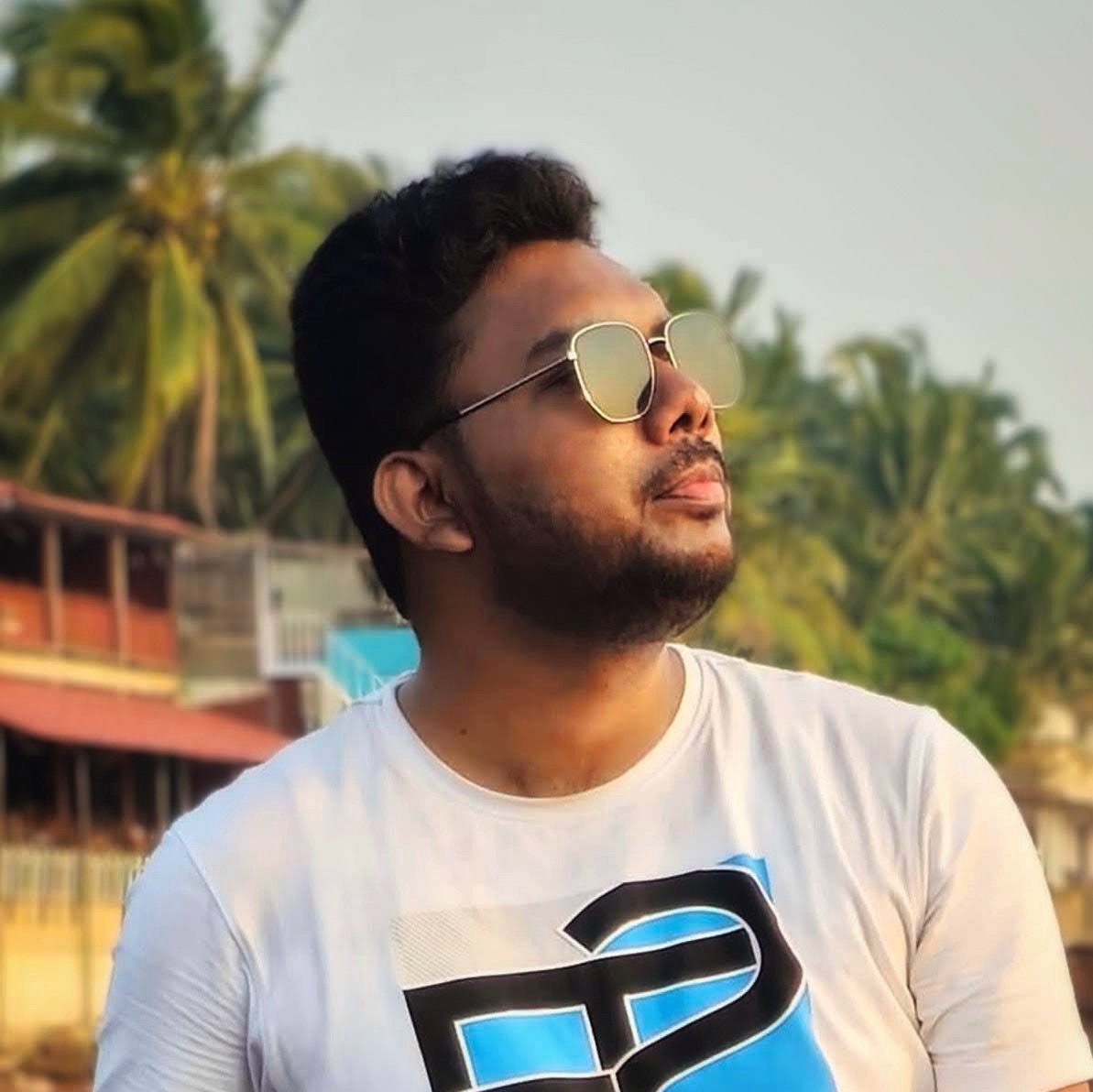
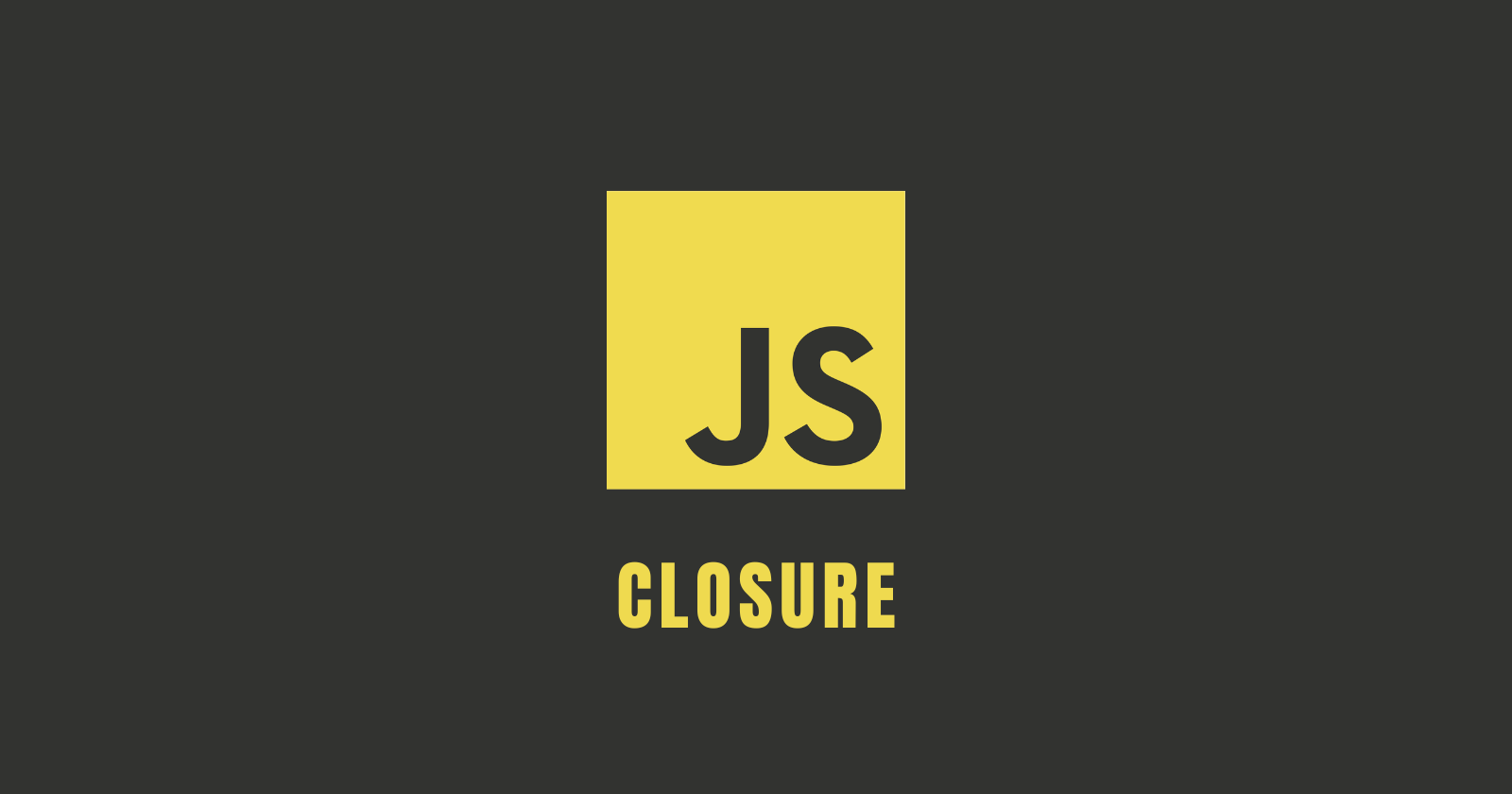
The definition
Closure is bundling of two or more functions, where inner function has access to the properties and methods of the outer functions.
function calculate() {
var num1 = 10;
function add(num2) {
return num1 + num2; // returning the sum of num1 and num2
}
return add; // returning the add function
}
var addition = calculate();
console.log(addition(20)); // printing the "addition" function output
// output: 30
Explanation: If you carefully observe, calculate functions's inner function "add" is able to access "num1" value, even if it's not declared inside the function. It is possible because of the "Closure" feature.
In conclusion, closures in JavaScript are a powerful feature that allows an inner function to retain access to the variables and parameters of its outer function even after the outer function has finished executing. This capability enables the creation of modular, encapsulated code and facilitates patterns like data hiding, module creation, and function factories. By understanding closures and their behaviour, developers can write more flexible and maintainable code in JavaScript.
Subscribe to my newsletter
Read articles from Rohit Dasu directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
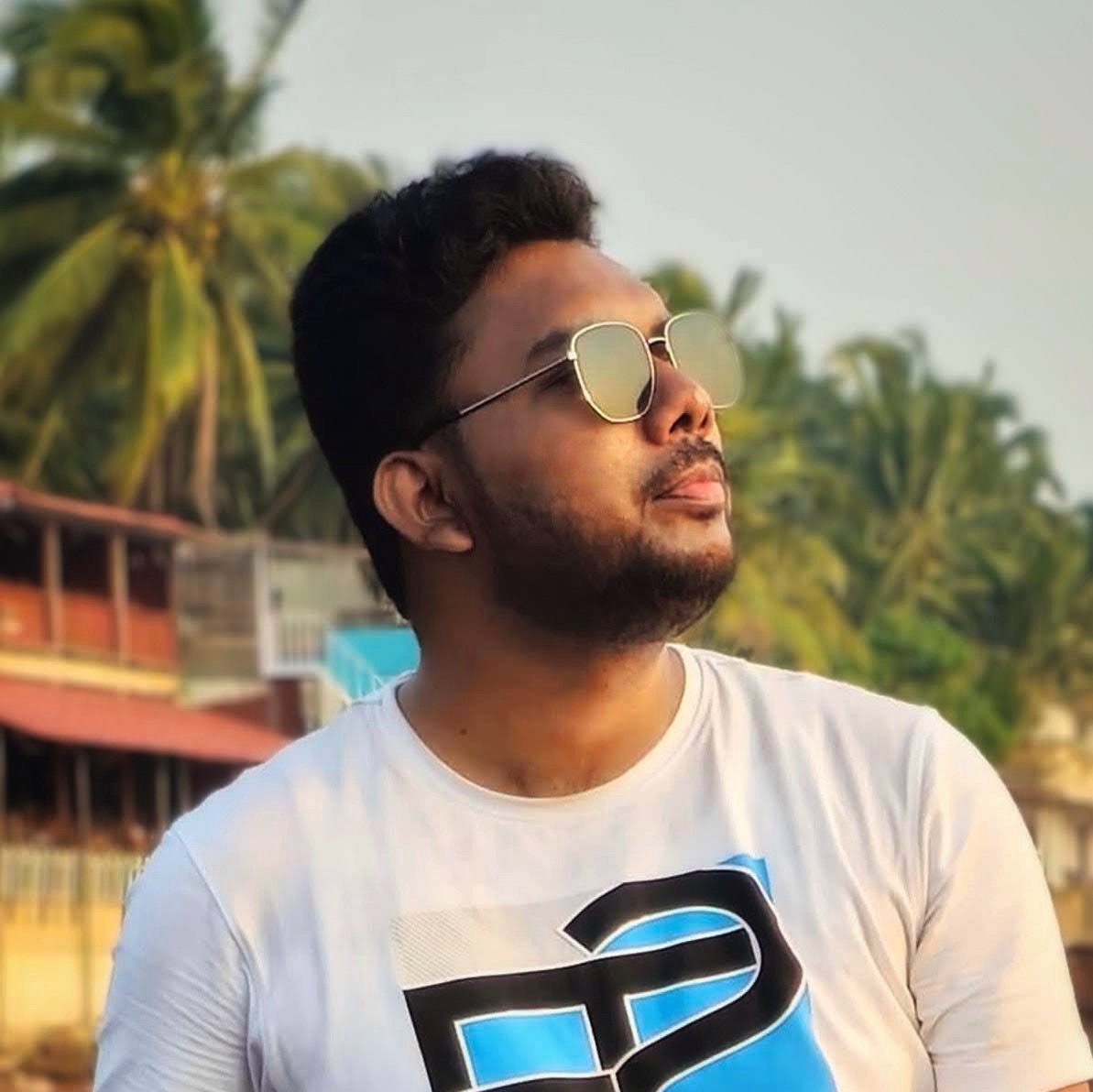
Rohit Dasu
Rohit Dasu
A Developer's Journey of Thought and Discovery