3. Building Minimal APIs with Route Parameters in ASP.NET Core
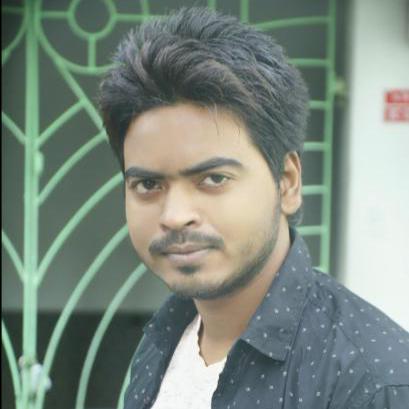
When developing web applications, effectively managing different routes is crucial. ASP.NET Core offers robust routing capabilities, including support for route parameters. Route parameters enable you to extract values from the URL and incorporate them into your application's logic.
This article delves into leveraging route parameters in ASP.NET Core Minimal APIs, with a focus on a practical demonstration.
Suppose you aim to establish an endpoint that caters to requests with URLs such as /helloworld/123
, where 123 signifies an ID. Below is a guide on accomplishing this using ASP.NET Core Minimal APIs:
using Microsoft.AspNetCore.Builder;
using Microsoft.AspNetCore.Http;
using Microsoft.AspNetCore.Routing;
var app = WebApplication.Create();
app.MapGet("/helloworld/{id:int}", (int id) =>
{
return Results.Ok($"Received ID: {id}");
});
app.Run();
Key points to note in the provided code snippet:
Utilization of MapGet to define a route that responds to GET requests.
The route pattern
/helloworld/{id:int}
specifies a route with a parameter named id of type int.Upon a request to a URL matching this pattern
(e.g., /helloworld/123)
, the id parameter is extracted from the URL.Within the request handler, we retrieve the id parameter and incorporate it into the response.
Upon accessing /helloworld/123
, the response will display "Received ID: 123", where 123 signifies the value of the id parameter extracted from the URL.
Route parameters serve as a potent tool in ASP.NET Core, enabling the creation of adaptable and dynamic routes in web applications. Whether constructing a RESTful API or a conventional web application, comprehending the utilization of route parameters is pivotal for architecting well-structured and effective APIs.
That concludes this article! I trust it has aided in elucidating the concept of route parameters in ASP.NET Core Minimal APIs. Keep an eye out for additional ASP.NET Core tutorials and insights!
Happy coding<๐>!
Subscribe to my newsletter
Read articles from Md Asif Alam directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
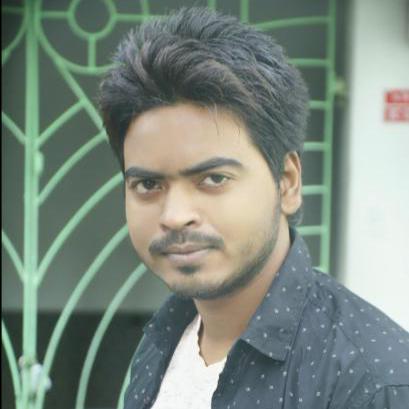
Md Asif Alam
Md Asif Alam
๐ Full Stack .NET Developer & React Enthusiast ๐จโ๐ป About Me: With 3+ years of experience, I'm passionate about crafting robust solutions and seamless user experiences through code. ๐ผ Expertise: Proficient in .NET Core API, ASP.NET MVC, React.js, and SQL. Skilled in backend architecture, RESTful APIs, and frontend development. ๐ Achievements: Led projects enhancing scalability by 50%, delivered ahead of schedule, and contributed to open-source initiatives. ๐ Future Focus: Eager to embrace new technologies and drive innovation in software development. ๐ซ Let's Connect: Open to new opportunities and collaborations. Reach me on LinkedIn or GitHub!