Swift Code Explained: Implementing Safe Array Subscription
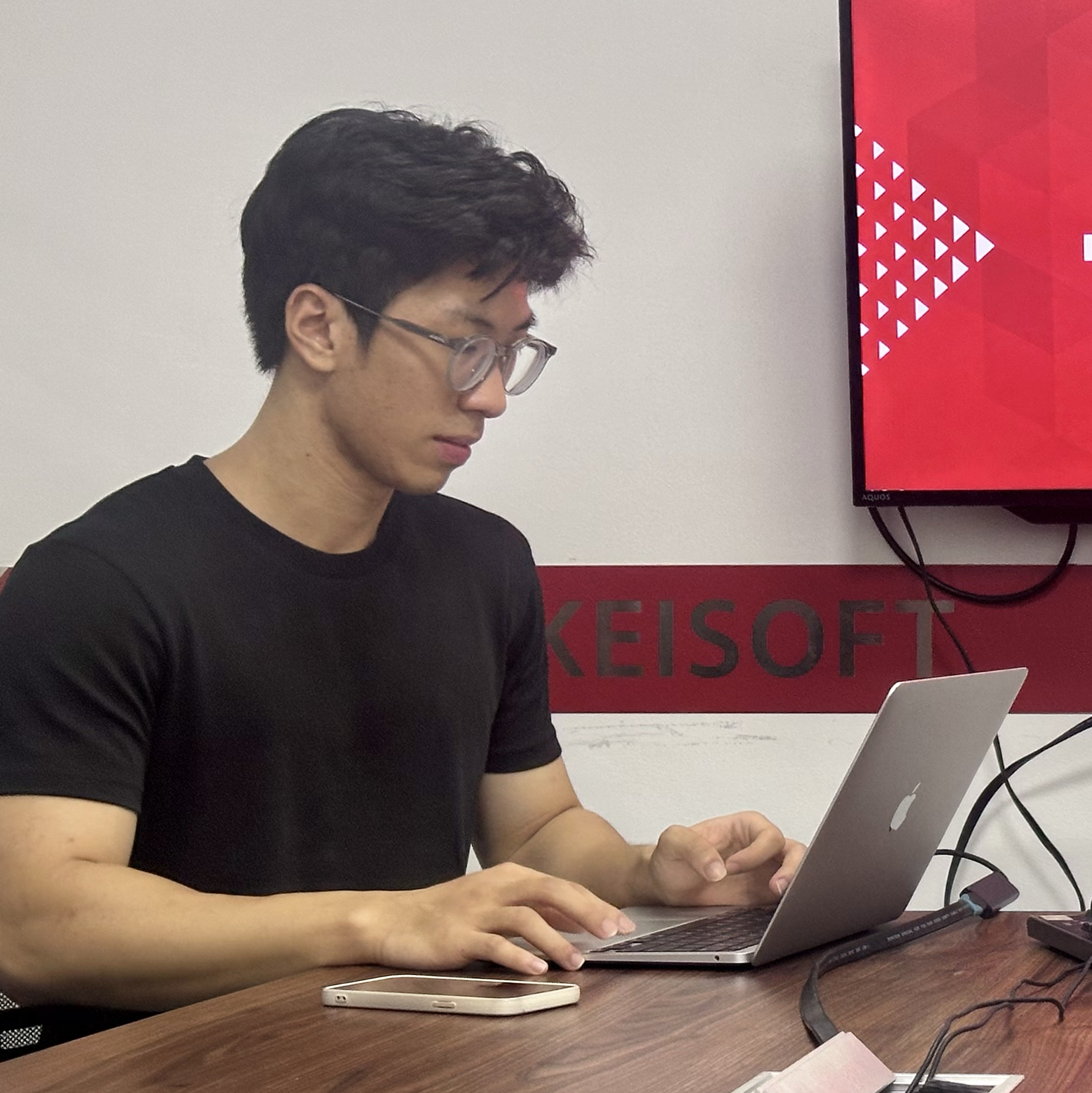
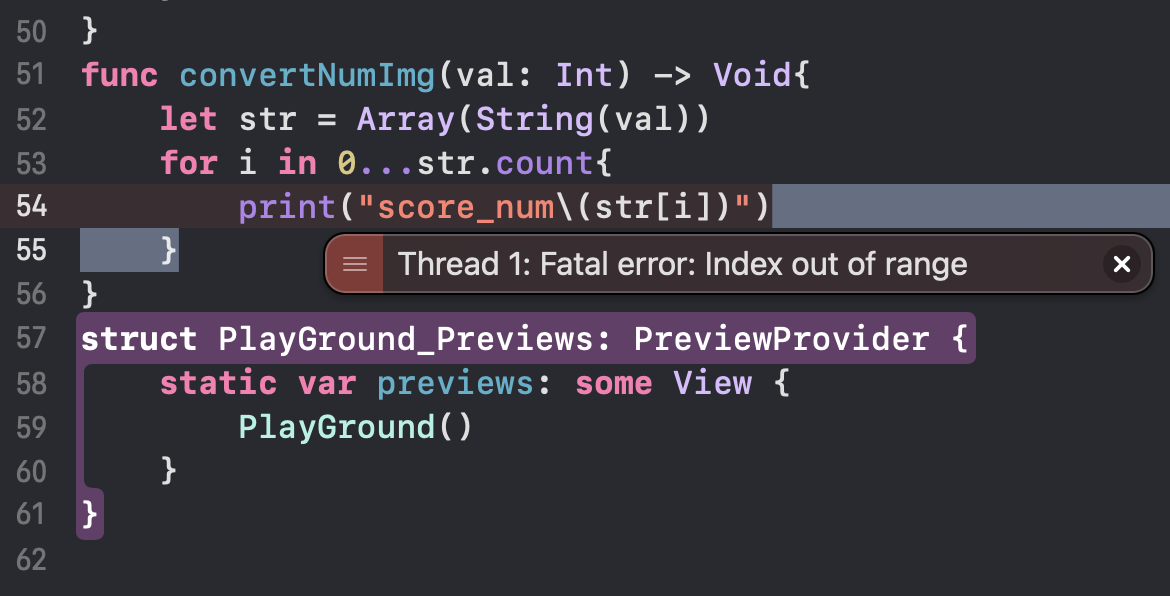
When working with arrays in Swift, you might have come across a common error called “Fatal error: Index out of range”. This error typically occurs when you unintentionally try to access an index that is not present in the array. Actually, accessing elements from an array is not safe by default. Therefore, today we’re going to explore how to code more securely when accessing elements in an array. 😊
Let’s look at an example:
let shoppingList = ["apples", "raspberries"]
let item = shoppingList[2]
Accessing an element this way will crash the app if the element isn’t there.
We’ll define an extension on the Collection type that adds a “safe” subscript. This subscript returns an optional value, which will be nil if the index is out of bounds.
extension Collection {
/// Returns the element at the specified index if it exists, otherwise nil.
subscript (safe index: Index) -> Element? {
return indices.contains(index) ? self[index] : nil
}
}
By using “if let” statement to unwrap the optional value, we can safely access the array item without worrying about out-of-bounds errors
if let item = shoppingList[safe: 2] {
// use item
}
Thanks for Reading! ✌️
If you have any questions or corrections, please leave a comment below or contact me via my LinkedIn account Pham Trung Huy.
Happy coding 🍻
Subscribe to my newsletter
Read articles from Phạm Trung Huy directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
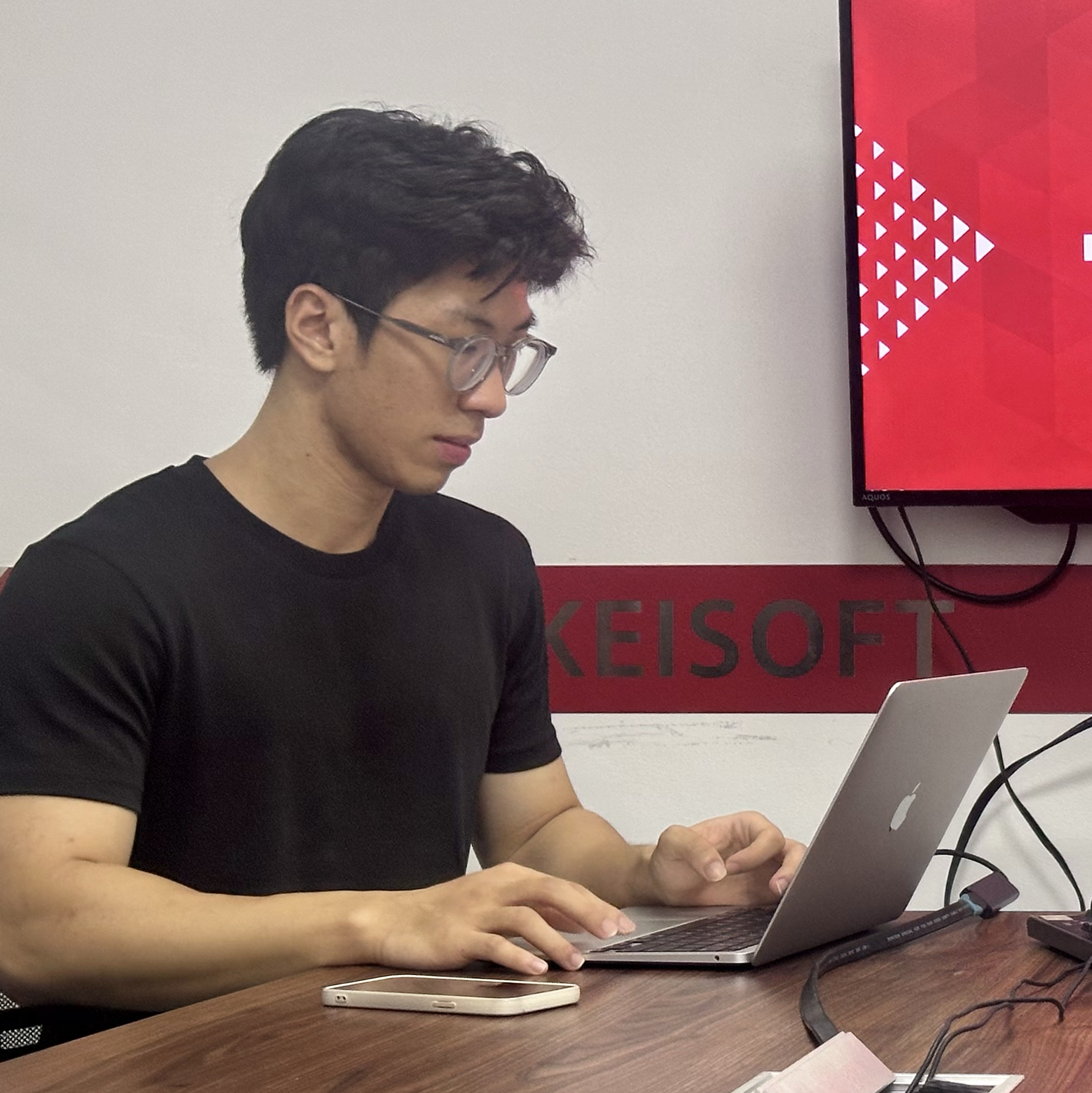
Phạm Trung Huy
Phạm Trung Huy
👋 I am a Mobile Developer based in Vietnam. My passion lies in continuously pushing the boundaries of my skills in this dynamic field.