Taming the File Beast: Mastering File Storage in Laravel
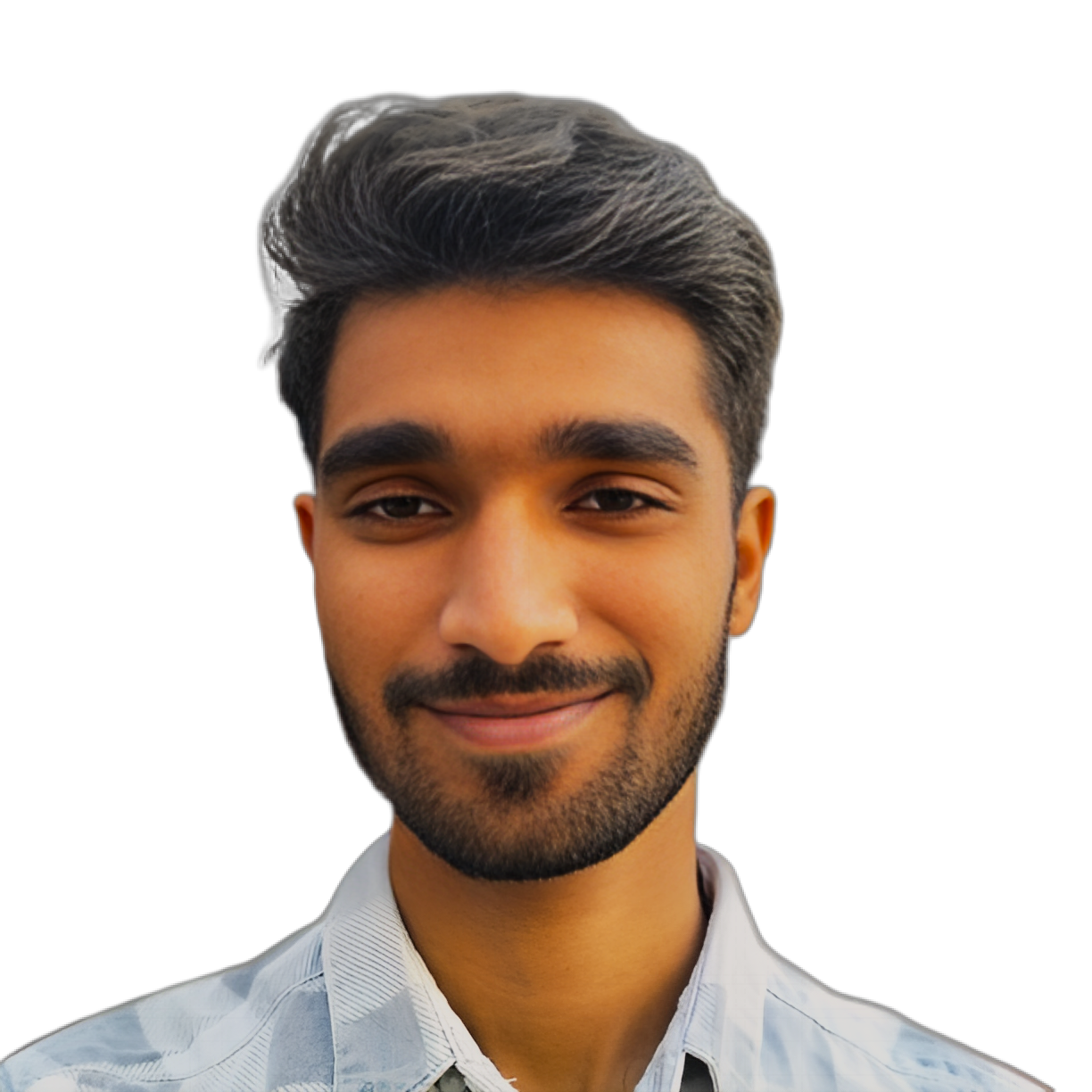
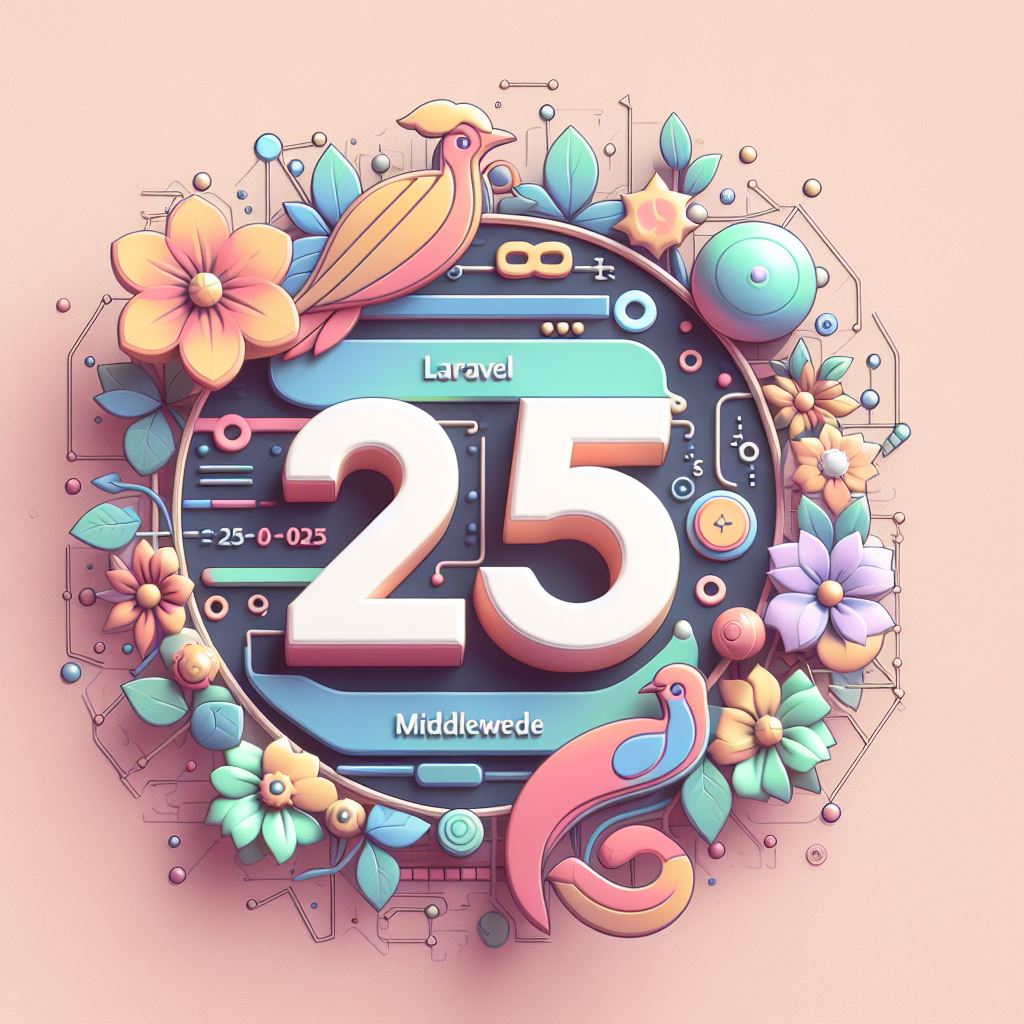
For developers working with Laravel, managing uploaded files can feel like wrangling a wild beast. But fear not! Laravel boasts a robust file storage system that takes the bite out of this crucial task. In this blog post, we'll unveil the secrets of Laravel's file storage, guiding you through storing, managing, and retrieving files with ease. So, whether you're dealing with user-uploaded photos, documents, or any other file type, Laravel has you covered.
Understanding Local Storage: Your App's Digital Filing Cabinet
By default, Laravel utilizes local storage. This means your uploaded files reside within a designated folder on the server where your application is located. Think of it as a digital filing cabinet for your application's files. This approach is perfectly suited for development environments and smaller projects where you have complete control over the server's resources.
Here's a breakdown of how local storage works in Laravel:
Uploading a File: When a user uploads a file through your application, Laravel retrieves it from the request object.
Generating a Unique Filename: To avoid conflicts, Laravel typically generates a unique filename for the uploaded file. This often involves combining the current timestamp with the original file extension.
Storing the File: Using the
Storage
facade, Laravel places the uploaded file within the designated local storage folder on the server.
Going Beyond Local: Cloud Storage for Scalability
While local storage offers a convenient solution, it might not always be enough for your application's needs. As your project grows, you may require:
Increased Storage: Local storage capacity can become limited, especially as your user base and uploaded files grow.
Enhanced Redundancy: Local storage doesn't offer built-in redundancy. If something happens to the server, your uploaded files could be lost.
Improved Accessibility: For geographically distributed users, accessing files stored locally might introduce latency issues.
This is where cloud storage comes to the rescue. Laravel seamlessly integrates with popular cloud storage providers like Amazon S3. By leveraging cloud storage, you can:
Scale Storage Effortlessly: Cloud storage offers virtually limitless capacity, allowing you to scale your application's storage needs effortlessly.
Boost Redundancy: Cloud storage providers typically offer robust redundancy features, ensuring your files remain safe even in case of server failure.
Enhance Accessibility: Cloud storage geographically distributes your files, making them readily accessible to users from all over the world.
Uploading Files with Laravel: A Code Example
Let's delve into a practical example showcasing how to upload a file using Laravel's file storage system. Here's a code snippet demonstrating the process:
PHP
use Illuminate\Http\Request;
use Illuminate\Support\Facades\Storage;
public function store(Request $request)
{
$file = $request->file('image');
$fileName = time().'.'.$file->getClientOriginalExtension();
Storage::disk('local')->put('uploads/'.$fileName, file_get_contents($file));
// Further processing or saving the filename to database...
}
Explanation:
We retrieve the uploaded file from the request object using
$request->file('image')
.We generate a unique filename by combining the current timestamp with the original file extension using
time().'.'.$file->getClientOriginalExtension()
.We leverage the
Storage
facade to store the uploaded file. We specify the local disk ('local'
) and the desired path within the storage folder ('uploads/'.$fileName'
).The
file_get_contents($file)
method reads the file's content.The
put
method stores the file content within the specified location.
Remember: This is a basic example. You can further process the uploaded file (e.g., resize images) or save its details (filename, path) in your database for future reference.
Exploring Laravel's File Storage Features
Laravel's file storage system offers a rich set of functionalities beyond basic uploads. Here are some additional features to explore:
Disk Configuration: Laravel allows you to configure various disks for different storage needs (local, cloud storage).
File Manipulation: You can use Laravel's helper functions to manipulate uploaded files (resize, rename, etc.).
File Visibility: Control how uploaded files are accessed publicly or privately.
URL Generation: Generate URLs for uploaded files for easy access within your application.
By delving deeper into these functionalities, you can truly harness the power of Laravel's file storage system and manage your application's files with efficiency and ease.
Ready to Tame the File Beast?
With this newfound knowledge, you're well-equipped to tackle file storage challenges in your Laravel projects
Subscribe to my newsletter
Read articles from Asis Sharma directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
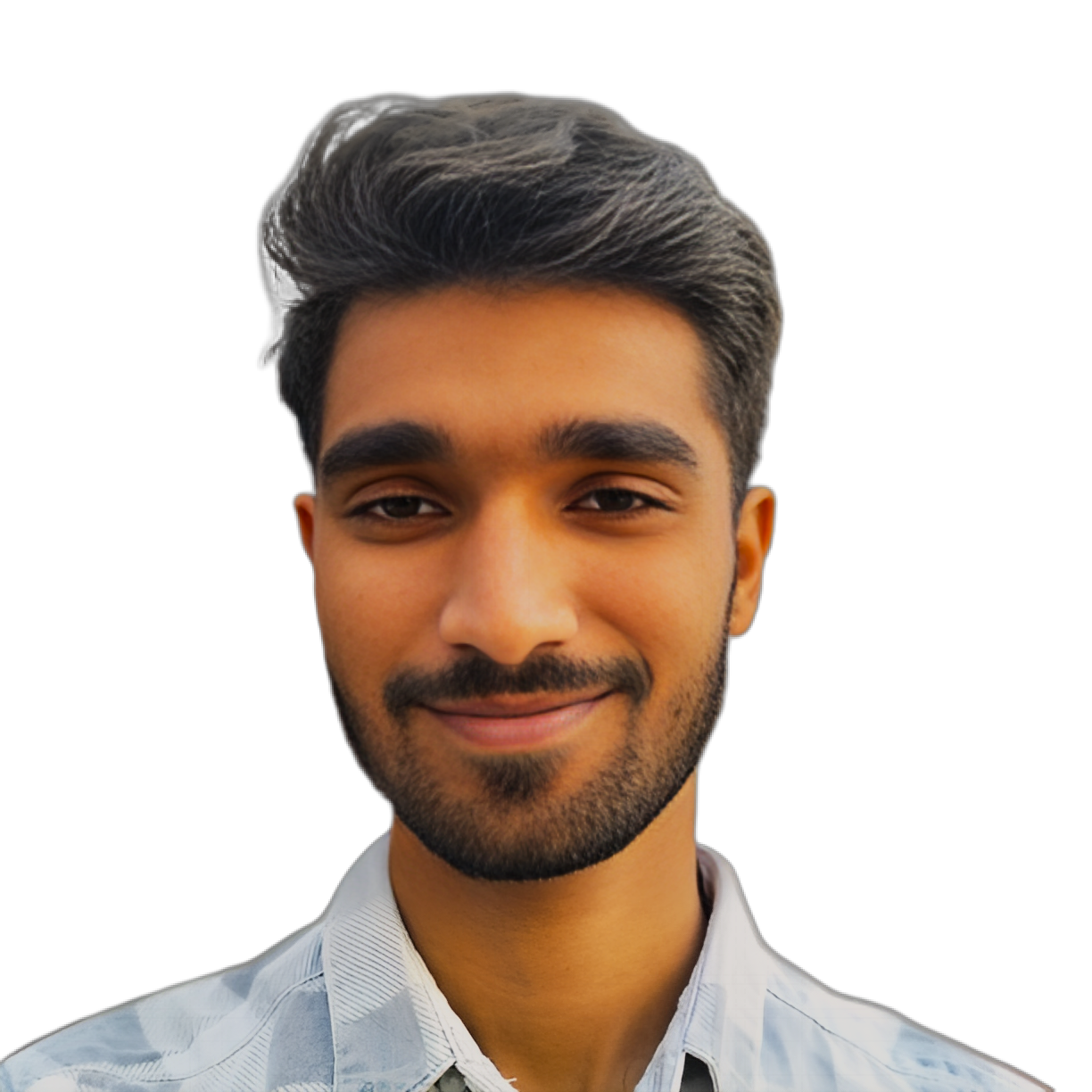
Asis Sharma
Asis Sharma
I'm an ambitious and motivated software developer, eager to apply my knowledge and skills to contribute to the development of innovative and cutting-edge software solutions. I'm well-versed in a variety of programming languages, including Javascript, C++, and Node.js, and have a understanding of object-oriented programming and algorithms. I'm passionate about developing and I am eager to learn and collaborate with others.