Basic Linux Shell Scripting
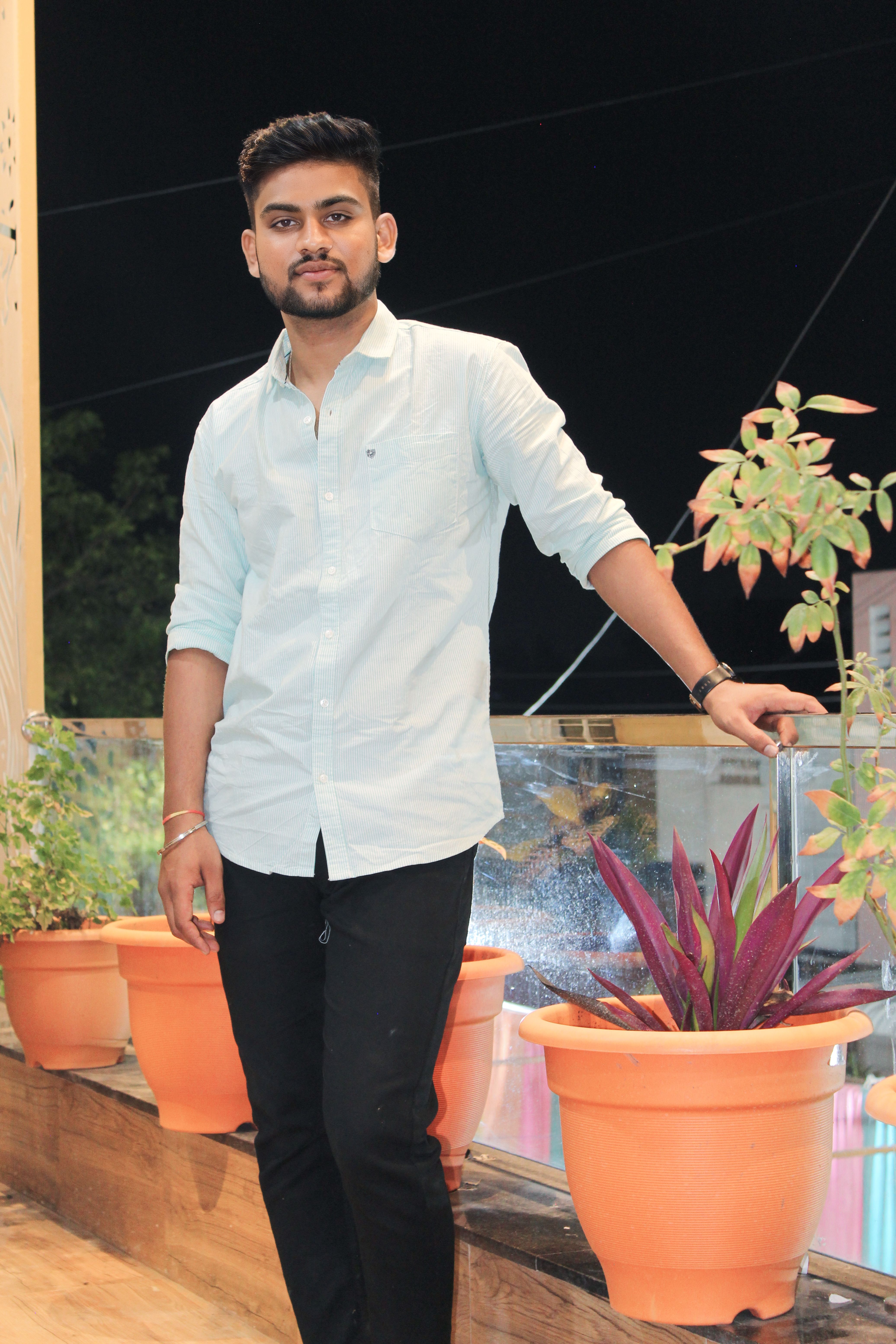
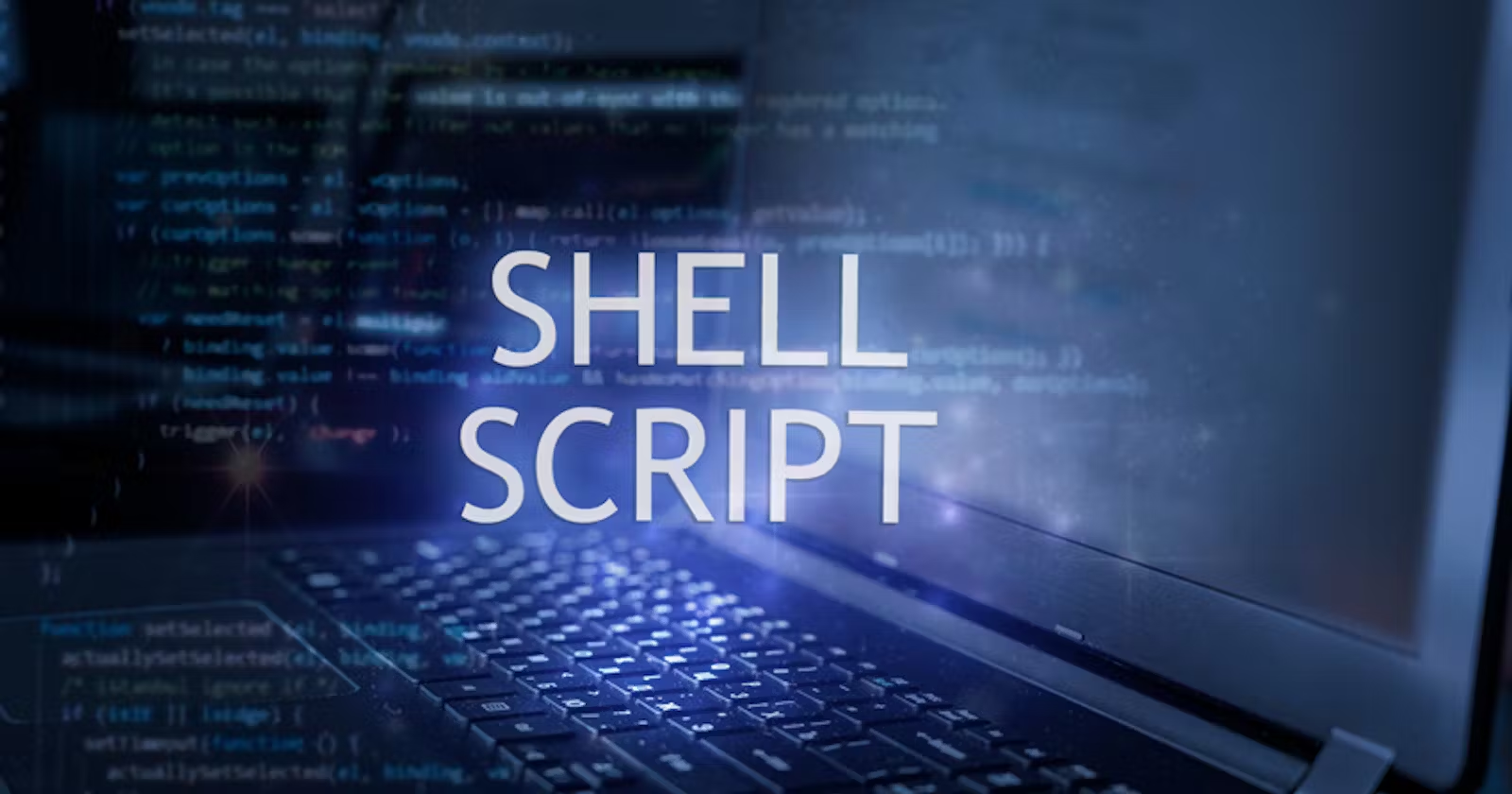
What is Kernel? π
The kernel is the core component of the Linux operating system. It manages system resources, such as memory and CPU, and facilitates communication between hardware and software.
πKey functions of the Linux kernel include:
What is Shell? π
The shell is a command-line interface that allows users to interact with the operating system. It interprets user commands and executes them by communicating with the kernel.
What is Linux Shell Scripting? π»
Linux shell scripting involves writing scripts (sequences of commands) that automate tasks and streamline workflows in the Linux environment. It enables users to perform repetitive tasks more efficiently.
What is Shell Scripting for DevOps with Examples? π οΈ
Shell scripting plays a crucial role in DevOps by automating deployment, configuration, and monitoring tasks. Examples include automating software installations, managing server configurations, and scheduling backups.
Automation of Deployment and Configuration:
Shell scripts are used to automate the deployment of applications and configuration of servers. For example, a script can be created to install dependencies, set up environment variables, and deploy the latest version of an application.
# Example: Deploying a web application #!/bin/bash echo "Updating code from version control..." git pull echo "Installing dependencies..." npm install echo "Restarting the application server..." systemctl restart my-todo-app
Continuous Integration/Continuous Deployment (CI/CD):
Shell scripts are commonly used in CI/CD pipelines to automate building, testing, and deploying applications. They integrate with CI/CD tools to execute these tasks automatically when code changes are pushed.
# Example: CI/CD pipeline script #!/bin/bash echo "Building the application..." ./build.sh echo "Running tests..." ./run_tests.sh echo "Deploying to production..." ./deploy_prod.sh
Infrastructure as Code (IaC):
Shell scripts help implement Infrastructure as Code principles, allowing DevOps teams to define and manage infrastructure using code. This includes provisioning and configuring servers, networks, and other infrastructure components.
# Example: Provisioning a virtual machine #!/bin/bash echo "Creating a new virtual machine..." virt-install --name my-vim --memory 2048 --disk size=20 --cdrom my-os.iso
Understanding Script Headers π
What is "#!/bin/bash?" β
The
#!/bin/bash
(or#!/bin/sh
) at the beginning of a script is called a shebang or hashbang. It is a special line that informs the system which interpreter should be used to execute the script. In the context of your question:#!/bin/bash
This shebang specifies that the Bash shell should be used to interpret and execute the script. Bash is a popular and feature-rich shell, and it is the default shell for many Linux distributions.
#!/bin/bash echo "Hello, this script is written in Bash."
#!/bin/sh
:This shebang indicates that the Bourne shell should be used. The Bourne shell is a simpler shell and is often used for writing more portable scripts that should work on a variety of Unix-like systems.
#!/bin/sh echo "Hello, this script is written in the Bourne shell."
β Write a Shell Script to take user input, input from arguments and print the variables.
#!/bin/bash
# Taking user input
echo "Enter your name:"
read username
# Taking input from command-line arguments
arg1=$1
arg2=$2
# Printing the variables
echo "User input: $username"
echo "Argument 1: $arg1"
echo "Argument 2: $arg2"
Save this script in a file, for example, input_
script.sh
. Give execute permissions to the script using the chmod +x input_
script.sh
command.
You can then run the script and provide command-line arguments like this:
./input_script.sh argument1 argument2
β Write an Example of If else in Shell Scripting by comparing 2 numbers.
#!/bin/bash
# Prompt the user to enter two numbers
echo "Enter the first number:"
read num1
echo "Enter the second number:"
read num2
# Compare the numbers
if [ "$num1" -eq "$num2" ]; then
echo "The numbers are equal."
else
echo "The numbers are not equal."
fi
Save this script in a file, for example, compare_
numbers.sh
, and give execute permissions using chmod +x compare_
numbers.sh
. Run the script, and it will prompt you to enter two numbers, then compare and print the results based on the if-else conditions.
Conclusion π
Congratulations! You've taken the first step towards mastering Linux shell scripting. With the knowledge gained from this guide, you'll be able to write basic scripts, automate tasks, and unleash the power of the command line. Keep practicing, exploring, and learning, and soon you'll become a shell scripting pro! Happy scripting!
πHappy Learning : )
Subscribe to my newsletter
Read articles from Paresh Parde directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
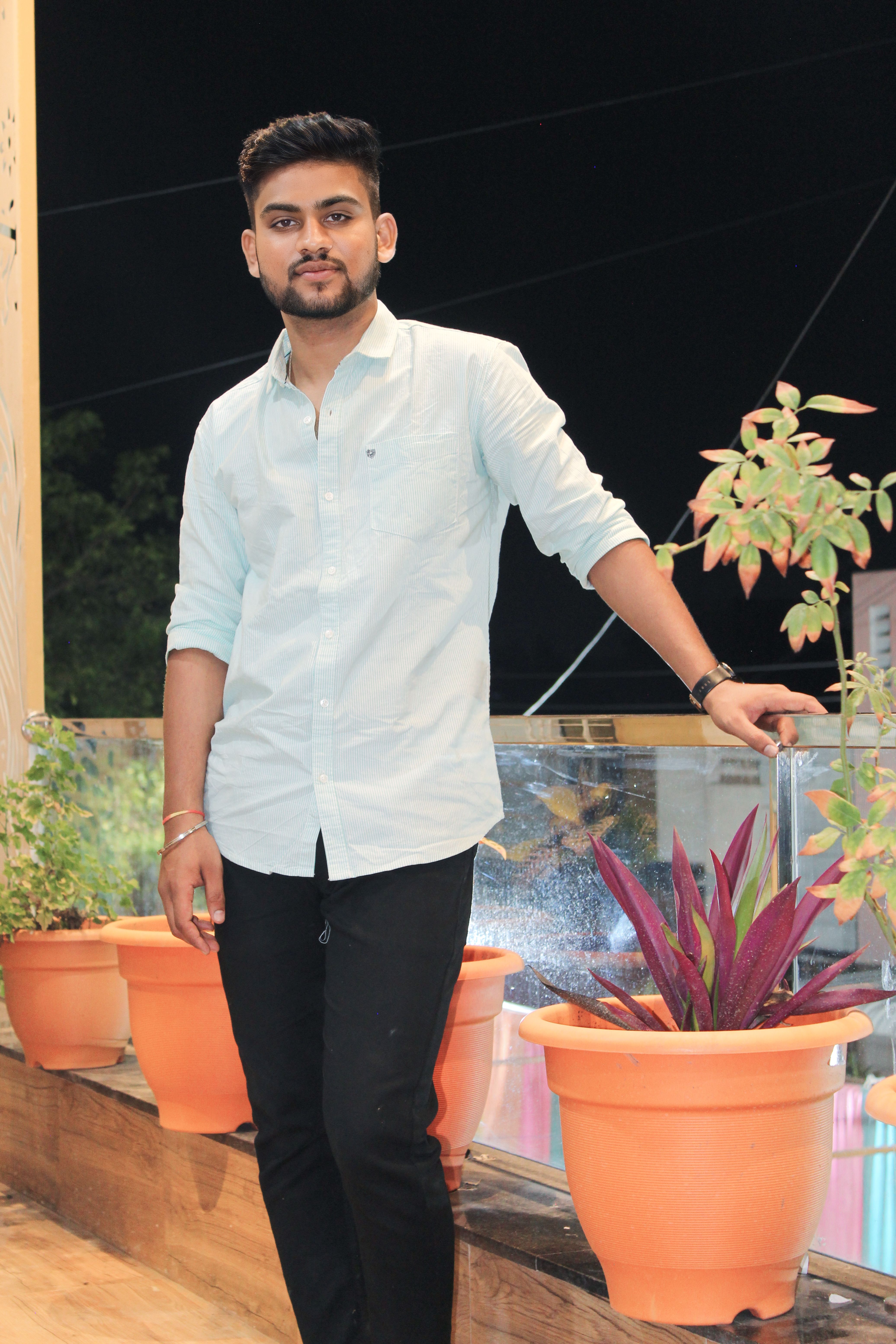
Paresh Parde
Paresh Parde
π Passionate DevOps Engineer | AWS Enthusiast | Continuous Learner π π Hello! I'm Paresh Parde, a passionate and eager DevOps enthusiast ready to embark on a journey in the world of technology. With a solid foundation in both development and operations, I bring a fresh perspective and a hunger to learn and grow within the DevOps landscape. Let's connect and explore how we can create impactful solutions together!π Follow me on LinkedIn: https://www.linkedin.com/in/paresh-parde-544493232/