09 Java - Classes (Part III)
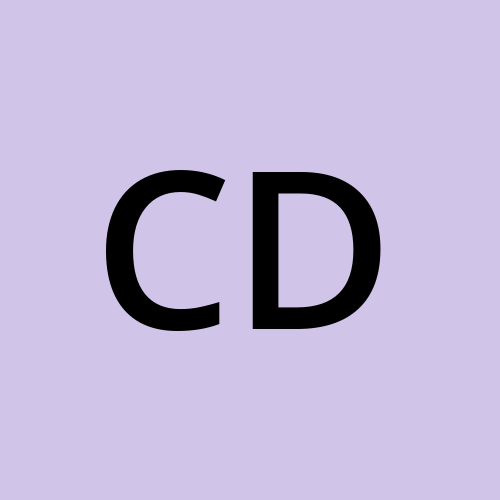
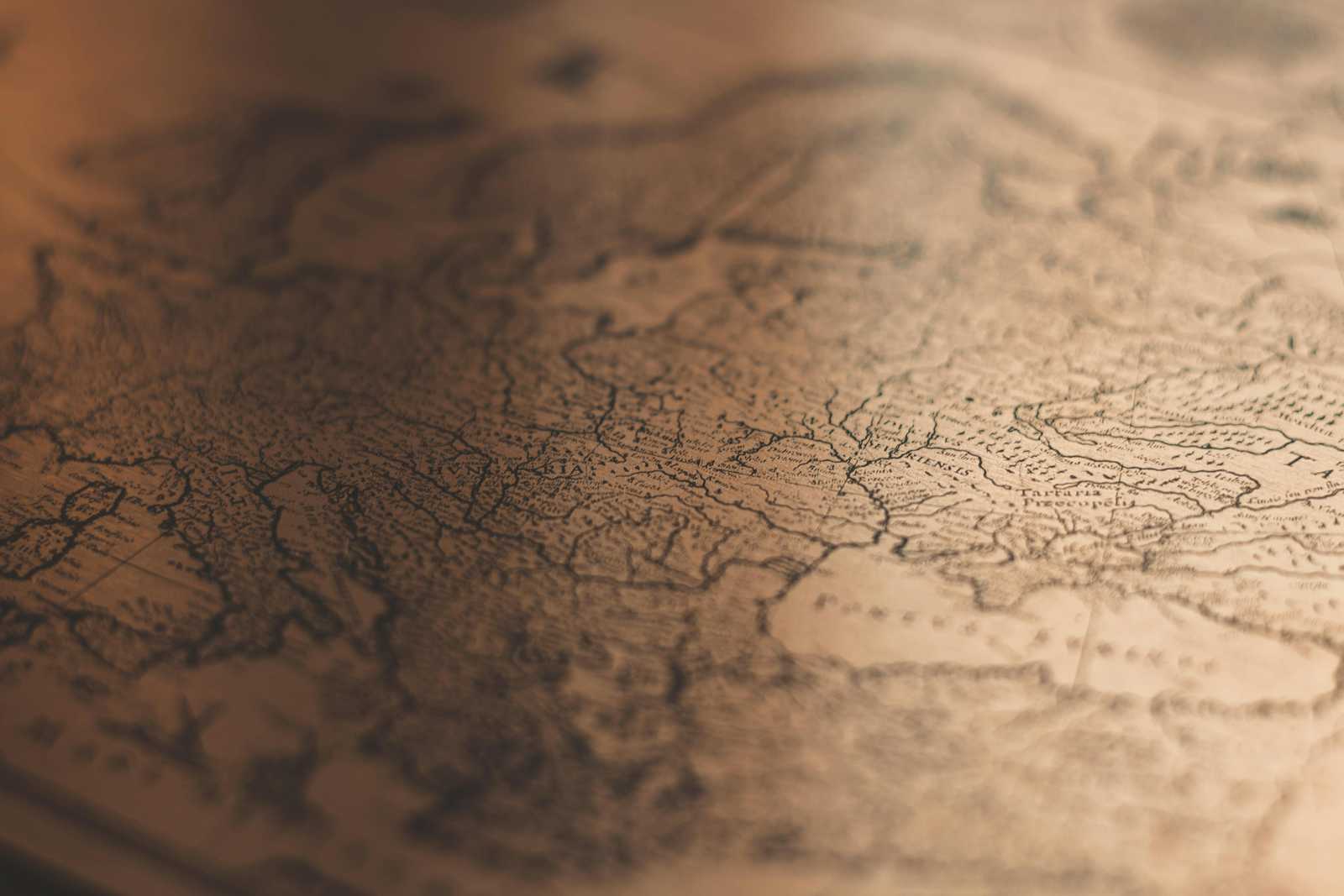
Pojo Class
Stands for "Plani Old Java Object".
Contains variables and its getter and setter methods.
Class should be public.
Public default constructor.
No annotations should be used like @ Table, @ Entity, @ Id etc..
It hould not extend any class or implement any interface.
package learn.poj;
public class Student {
int name;
private int rollNumber;
protected String address;
public int getName() {
return name;
}
public void setName(int name) {
this.name = name;
}
public int getRollNumber() {
return rollNumber;
}
public void setRollNumber(int rollNumber) {
this.rollNumber = rollNumber;
}
public String getAddress() {
return address;
}
public void setAddress(String address) {
this.address = address;
}
}
Enum Class
It has a collection of CONSTANTS (variables which values can not be changed)
Its CONSTANTS are static and final implicity (we do not have to write it).
It can not extend any class, as it internally extends java.lang.Enum class
It can implement interfaces.
It can have variables, constructor, methods.
It can not be initiated (as its constructor will be private only, even you give default, in bytecode it make it private)
Not other class can extend Enum class
It can have abstract method, and all the constant should implement that abstract method.
Normal Enum class
public enum EnumSample {
//Default values of constants
MONDAY, //0
TUESDAY, //1
WEDNESDAY, //2
THURSDAY, //3
FRIDAY, //4
SATURDAY, //5
SUNDAY; //6
}
public class Main {
public static void main(String[] args) {
/*
Common Functions which is used
- values()
- ordinal()
- valueOf()
- name()
*/
//1. Usage of values() and ordinal()
// EnumSample.values return array EnumSample[]
for (EnumSample sample: EnumSample.values()){
System.out.println(sample.ordinal());
}
//2. Usage of valueOf and name()
EnumSample enumVariable = EnumSample.valueOf("FRIDAY");
System.out.println(enumVariable.name());
}
}
output
0
1
2
3
4
5
6
FRIDAY
Enum with Custom Values
public enum EnumSample {
MONDAY(101,"1st day of the week"),
TUESDAY(102,"2nd day of the week"),
WEDNESDAY(103,"3rd day of the week"),
THURSDAY(104,"4th day of the week"),
FRIDAY(105,"5th day of the week"),
SATURDAY(106,"1st day of the week end"),
SUNDAY(107,"2nd day of the week end");
private int val;
private String comment;
EnumSample(int val, String comment) {
this.val = val;
this.comment = comment;
}
public int getVal() {
return val;
}
public String getComment() {
return comment;
}
public static EnumSample getEnumFromValue(int value){
for (EnumSample sample: EnumSample.values()){
if (sample.val == value){
return sample;
}
}
return null;
}
}
public class Main {
public static void main(String[] args) {
EnumSample sampleVar = EnumSample.getEnumFromValue(107);
System.out.println(sampleVar.getComment());
}
}
/* Output
2nd day of the week end
*/
Method Override by Constant
public enum EnumSample {
MONDAY{
@Override
public void dummyMethod() {
System.out.println("Monday dummy Method");
}
},
TUESDAY,
WEDNESDAY,
THURSDAY,
FRIDAY,
SATURDAY,
SUNDAY;
public void dummyMethod(){
System.out.println("default dummy Method");
}
}
public class Main {
public static void main(String[] args) {
EnumSample fridayEnumSample = EnumSample.FRIDAY;
fridayEnumSample.dummyMethod();
EnumSample mondayEnumSample = EnumSample.MONDAY;
mondayEnumSample.dummyMethod();
}
}
/*
default dummy Method
Monday dummy Method
*/
Enum with Abstract Method
package learn.poj;
public enum EnumSample {
MONDAY{
@Override
public void dummyMethod() {
System.out.println("In MONDAY");
}
},
TUESDAY {
@Override
public void dummyMethod() {
System.out.println("In TUESDAY");
}
},
WEDNESDAY {
@Override
public void dummyMethod() {
System.out.println("In WEDNESDAY");
}
},
THURSDAY {
@Override
public void dummyMethod() {
System.out.println("In THURSDAY");
}
},
FRIDAY {
@Override
public void dummyMethod() {
System.out.println("In FRIDAY");
}
},
SATURDAY {
@Override
public void dummyMethod() {
System.out.println("In SATURDAY");
}
},
SUNDAY {
@Override
public void dummyMethod() {
System.out.println("In SUNDAY");
}
};
public abstract void dummyMethod();
}
public class Main {
public static void main(String[] args) {
EnumSample fridayEnumSample = EnumSample.FRIDAY;
fridayEnumSample.dummyMethod();
}
}
Enum implement Interface
public interface MyInterface {
public String toLowerCase();
}
public enum EnumSample implements MyInterface {
MONDAY,
TUESDAY,
WEDNESDAY,
THURSDAY,
FRIDAY,
SATURDAY,
SUNDAY;
@Override
public String toLowerCase() {
return this.name().toLowerCase();
}
}
public class Main {
public static void main(String[] args) {
EnumSample fridayEnumSample = EnumSample.FRIDAY;
System.out.println(fridayEnumSample.toLowerCase());
}
}
Enum vs static final Keyword
What's the benefit of ENUM class when we can create constant through "static" and "final" keyword?
Readability of the code is good with enum
Enum also restricts the object to the constants present in that particular class. (Enum has the controll over the method)
public class WeekConstants {
public static final int MONDAY = 0;
public static final int TUESDAY = 1;
public static final int WEDNESDAY = 2;
public static final int THURSDAY = 3;
public static final int FRIDAY = 4;
public static final int SATURDAY = 5;
public static final int SUNDAY = 6;
}
package learn.poj;
public enum EnumSample {
MONDAY,
TUESDAY,
WEDNESDAY,
THURSDAY,
FRIDAY,
SATURDAY,
SUNDAY;
}
public class Main {
public static void main(String[] args) {
isWeekend(2); //wednesday, so it will return false
isWeekend(6); // sunday, so it will return true
isWeekend(100); // This value is not expected, but still we are able to send this in parameter
//better readability and full control on what value we can pass in parameter
isWeekend(EnumSample.WEDNESDAY); // return false
isWeekend(EnumSample.SUNDAY); // return true
}
public static boolean isWeekend(int day){
if (WeekConstants.SATURDAY == day || WeekConstants.SUNDAY == day){
return true;
}
return false;
}
public static boolean isWeekend(EnumSample day){
if (EnumSample.SATURDAY == day || EnumSample.SUNDAY == day){
return true;
}
return false;
}
}
Final Class
- Final class can not be inherited
public final class TestClass {
}
Immutable Class
We can not change the value of an object once it is created.
Declare class as 'final' so that it can not be extended.
All class members should be private. So that direct access can be avoided.
And class members are initialized only once using constructor.
There should not be any setter methods, which is generally use to change the value.
Just getter methods. And returns Copy of the member variable.
Example: String, Wrapper Classess etc.
public class MyImmutableClass {
private final String name;
private final List<Object> petNameList;
MyImmutableClass(String name, List<Object> petNameList){
this.name = name;
this.petNameList = petNameList;
}
public String getName() {
return name;
}
public List<Object> getPetNameList() {
/*
this is required, because making list final,
means you can not now point it to new list, but still can add,
delete values in it
so that's why we send the copy of it.
*/
return new ArrayList<>(petNameList);
}
}
Illustration
public class Main {
public static void main(String[] args) {
List<Object> petNames = new ArrayList<>();
petNames.add("sj");
petNames.add("pj");
MyImmutableClass obj = new MyImmutableClass("myName", petNames);
obj.getPetNameList().add("hello");
System.out.println(obj.getPetNameList());
}
}
/* Output
[sj, pj]
*/
Singleton Class
Refer - Link
Subscribe to my newsletter
Read articles from Chetan Datta directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
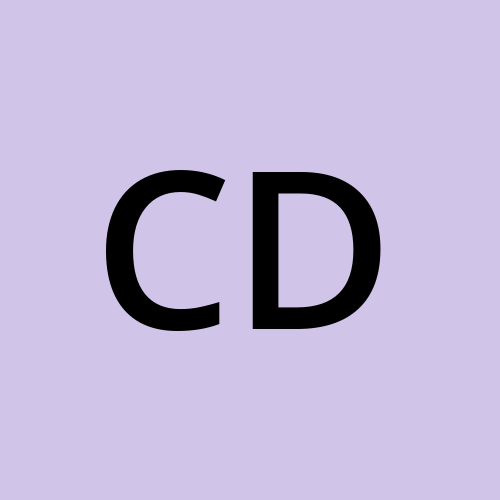
Chetan Datta
Chetan Datta
I'm someone deeply engrossed in the world of software developement, and I find joy in sharing my thoughts and insights on various topics. You can explore my exclusive content here, where I meticulously document all things tech-related that spark my curiosity. Stay connected for my latest discoveries and observations.