Understanding Asynchronous Programming: A Beginner's Guide to Promises, Async/Await, and Callbacks
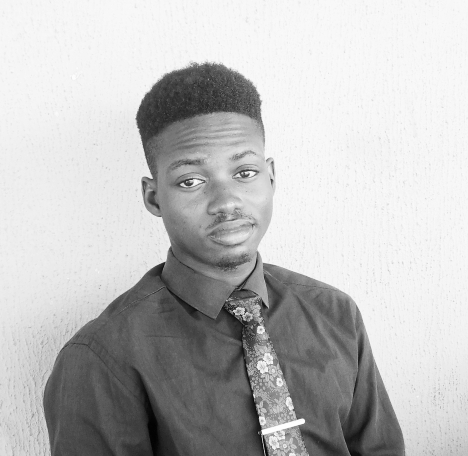
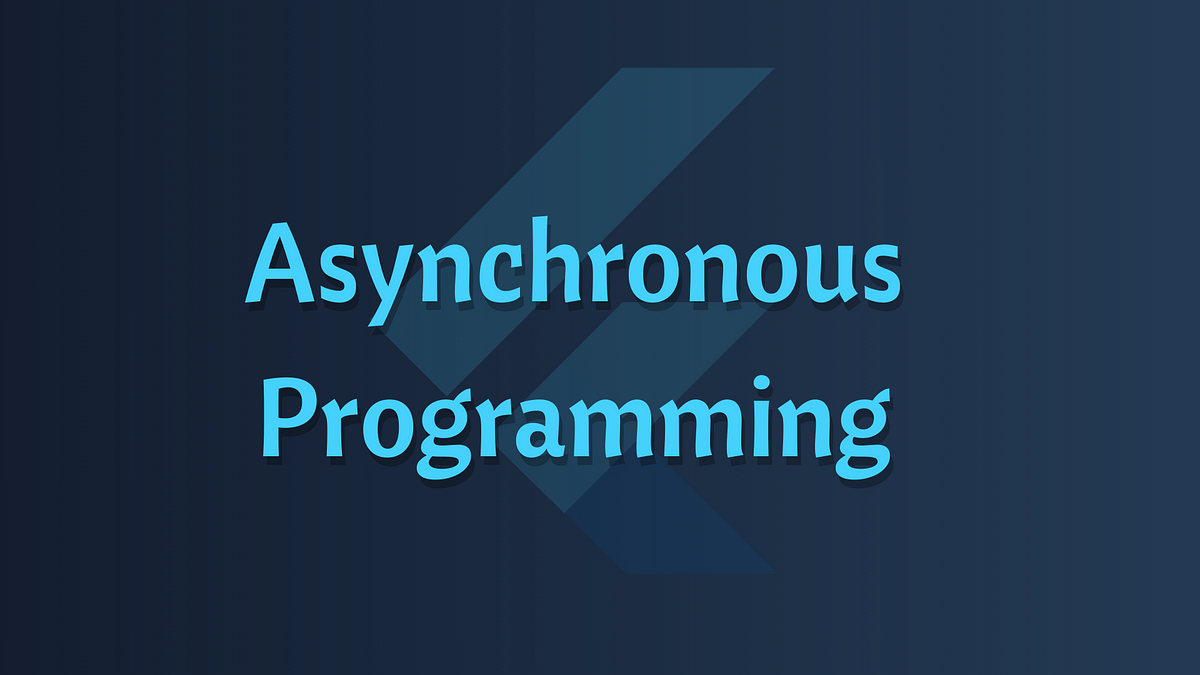
Introduction
Asynchronous programming is a cornerstone of modern development, enabling applications to perform tasks concurrently and maintain responsiveness. However, for many developers, grasping the concepts of asynchronous programming can be challenging. In this beginner's guide, we'll unravel the complexities of asynchronous programming and explore three essential techniques: promises, async/await, and callbacks.
What You'll Learn
By the end of this guide, you'll have a solid understanding of:
The fundamentals of asynchronous programming and its importance in modern development.
How promises, async/await, and callbacks enable asynchronous operations in JavaScript.
Practical examples demonstrating the implementation of each technique.
Best practices for writing clean and efficient asynchronous code.
Strategies for troubleshooting common issues encountered in asynchronous programming.
Key Terms to Understand
Before we dive in, let's clarify some key terms:
Asynchronous Programming: A programming paradigm that allows multiple tasks to execute independently without blocking the main execution thread.
Promises: Objects representing the eventual completion or failure of an asynchronous operation, providing a cleaner alternative to callbacks for handling asynchronous tasks.
Async/Await: Syntactic sugar built on top of promises, simplifying the process of writing asynchronous code by allowing developers to write asynchronous functions in a synchronous style.
Callbacks: Functions passed as arguments to other functions and executed asynchronously, commonly used in older JavaScript code to handle asynchronous operations.
Understanding Asynchronous Programming
In the realm of programming, understanding asynchronous operations is akin to mastering a multitasking juggler—it's all about managing multiple tasks simultaneously without missing a beat. Asynchronous programming is the backbone of modern web development, allowing applications to execute tasks concurrently, ensuring responsiveness, and preventing the dreaded "freeze" that can occur when one task monopolizes the system.
At the heart of asynchronous programming lies the event loop, a critical mechanism that orchestrates the flow of asynchronous tasks in JavaScript. Think of it as the conductor of a symphony, ensuring that each instrument (or task) plays its part harmoniously without hogging the spotlight. By leveraging the event loop, JavaScript can handle asynchronous operations efficiently, juggling multiple tasks without skipping a beat.
Exploring Promises
Enter promises—a powerful abstraction that simplifies asynchronous code and tames the unruly beast known as callback hell. Promises are like IOUs, representing the eventual completion (or failure) of an asynchronous operation. They provide a clean and structured way to handle asynchronous tasks, allowing developers to chain operations seamlessly and handle errors gracefully.
Creating a promise is like making a bet—you're making a promise that you'll deliver the goods, whether it's a successful outcome or a heartbreaking failure. Once the promise is made, you can await its fulfillment using the then
method, which allows you to handle the result of the asynchronous operation. And if things go awry, you can catch errors using the catch
method, ensuring that your code remains robust and resilient.
Practical Example - Promises:
function fetchData() {
return new Promise((resolve, reject) => {
setTimeout(() => {
const data = { id: 1, name: "John Doe" };
if (data) {
resolve(data); // Promise resolved with data
} else {
reject(new Error("Failed to fetch data")); // Promise rejected with error
}
}, 1000);
});
}
// Using the promise
fetchData()
.then((data) => {
console.log("Data:", data);
})
.catch((error) => {
console.error("Error:", error);
});
Harnessing Async/Await:
But wait, there's more! Enter async/await—a game-changing duo that brings the elegance of synchronous code to the asynchronous world. Async/await functions allow developers to write asynchronous code in a synchronous style, making it easier to reason about and debug. It's like having your cake and eating it too—you get the benefits of asynchronous programming without sacrificing readability or sanity.
Imagine you're baking a cake. You have a recipe (code) that tells you to mix the ingredients, put the cake in the oven, and wait for it to bake. Now, traditionally, if you're multitasking, you might start mixing the ingredients, then leave them to check your email while the cake bakes. But how do you know when it's done baking? You need to keep checking back, right?
Async/await is like having a magic button that pauses everything until the cake is ready. You can mix the ingredients, press the magic button (await), and just sit there until the cake is baked. No need to keep checking back every few minutes. Once the cake is done, you can continue with your other tasks, knowing that everything is ready.
In programming terms, async/await works similarly. It allows you to write code that looks and feels synchronous (like step-by-step instructions), even though it's actually asynchronous (running tasks in the background). So, when you use async/await, you can write code that waits for asynchronous tasks to complete without blocking the rest of your code—making it easier to understand and manage.
Declaring an async function is like planting a flag, signaling to JavaScript that you're about to embark on an asynchronous journey. Inside the async function, you can await promises using the await
keyword, pausing execution until the promise is resolved or rejected. And thanks to the magic of try-catch blocks, you can handle errors with grace and poise, ensuring that your code remains resilient in the face of adversity.
Practical Example - Async/Await:
async function fetchData() {
try {
const response = await fetch("https://api.example.com/data");
const data = await response.json();
console.log("Data:", data);
} catch (error) {
console.error("Error:", error);
}
}
// Calling the async function
fetchData();
Understanding Callbacks:
Last but not least, let's talk about callbacks—the OGs of asynchronous programming. Callbacks are like messengers, delivering news of completed tasks asynchronously. They're commonly used in older JavaScript code to handle asynchronous operations, though their unruly nature can sometimes lead to callback hell—a tangled mess of nested callbacks that's as frustrating as it is difficult to debug.
Despite their shortcomings, callbacks still have their place in the asynchronous pantheon. With careful planning and judicious use, callbacks can be wielded effectively, providing a lightweight and efficient way to handle asynchronous tasks. Just remember to keep them tidy, avoid nesting them too deeply, and handle errors with care to prevent callback-induced headaches.
SUMMARY
In the realm of modern software development, mastering asynchronous programming is not just advantageous—it's essential. Asynchronous programming serves as the cornerstone for building responsive and efficient applications, empowering developers to handle complex tasks with finesse. Throughout this article, we've explored the fundamentals of asynchronous programming, dissecting key concepts such as promises, async/await, and callbacks.
Promises, with their ability to represent the eventual completion or failure of an asynchronous operation, offer a structured approach to handling asynchronous tasks. They streamline code execution and pave the way for cleaner, more manageable codebases.
Meanwhile, async/await—a dynamic duo built on top of promises—provides developers with a synchronous-style syntax for writing asynchronous code. This powerful combination simplifies code readability and maintenance, making complex asynchronous operations more accessible and comprehensible.
Additionally, we've delved into callbacks—the OGs of asynchronous programming. Despite their reputation for leading to callback hell, callbacks still play a significant role in asynchronous JavaScript programming. By understanding their nuances and adhering to best practices, developers can leverage callbacks effectively, ensuring efficient task execution.
By embracing asynchronous programming and mastering promises, async/await, and callbacks, developers gain the ability to unlock new possibilities and navigate the complexities of modern software development with confidence. Asynchronous programming isn't just a skill—it's a paradigm shift that elevates JavaScript proficiency to new heights, enabling developers to build robust, scalable, and responsive applications that meet the demands of today's digital landscape.
FAQ
Q: Why is asynchronous programming important?
A: Asynchronous programming allows applications to perform multiple tasks concurrently, improving responsiveness and scalability.
Q: What is the difference between promises and callbacks?
A: Promises provide a cleaner and more structured approach to handling asynchronous operations compared to callbacks, reducing the likelihood of callback hell and improving code readability.
Subscribe to my newsletter
Read articles from Adeoye David directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
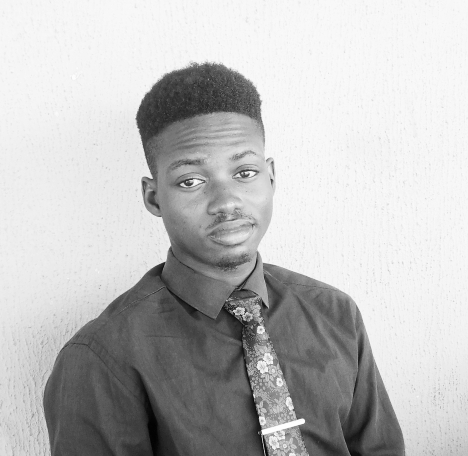
Adeoye David
Adeoye David
I'm David, a passionate backend developer and avid technical writer dedicated to crafting clean, efficient, and scalable software solutions. With a deep-rooted love for code and a knack for communicating complex technical concepts in a clear and concise manner, I thrive in the intersection of technology and writing. As a backend developer, I specialize in building robust and reliable server-side applications using technologies like Node.js, Express.js, and MongoDB. Whether it's architecting scalable APIs, optimizing database performance, or implementing secure authentication mechanisms, I'm always up for the challenge of tackling backend complexities. Beyond my coding endeavors, I find immense joy in the art of technical writing. I believe that effective documentation is just as important as writing code—it empowers users, fosters collaboration, and ensures the long-term success of software projects. From comprehensive API guides to in-depth tutorials, I strive to create informative and accessible content that educates and inspires fellow developers. On this platform, you'll find a blend of my technical insights, coding adventures, and musings on software engineering best practices. Whether you're a seasoned developer seeking to deepen your knowledge or a newcomer navigating the world of backend development, I invite you to join me on this journey of exploration and discovery.