The Power of Clean Code: Strategies for Writing Maintainable and Scalable JavaScript Applications
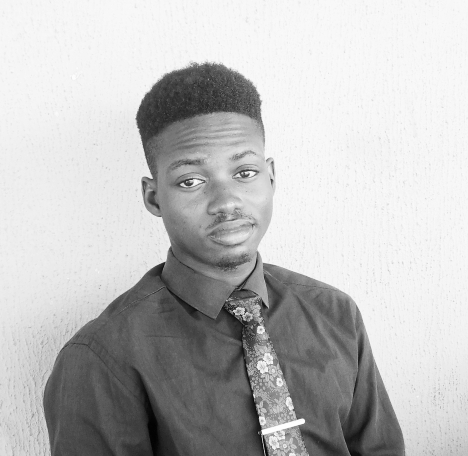
Table of contents
- Introduction
- The Importance of Clean Code in Enhancing Code Maintainability and Scalability
- Key Principles and Best Practices for Writing Clean and Readable JavaScript Code
- Strategies for Refactoring and Optimizing Existing Codebases to Improve Clarity and Efficiency
- Tools and Techniques for Automated Code Quality Checks and Continuous Integration
- The Impact of Clean Code on Collaboration, Debugging, and Long-Term Project Success
- Summary
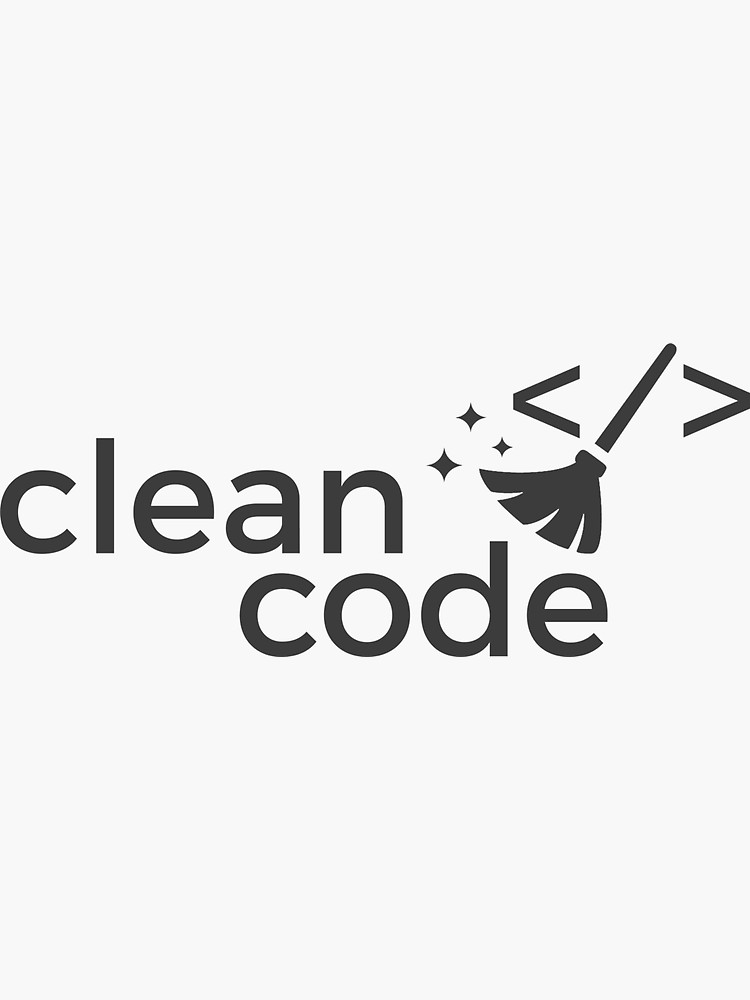
Introduction
In the dynamic landscape of software development, the importance of clean code cannot be overstated. Clean code not only enhances code maintainability and scalability but also fosters collaboration, simplifies debugging, and contributes to long-term project success. In this article, we'll explore the significance of clean code and delve into practical strategies for writing and maintaining clean and readable JavaScript applications.
What You'll Learn
By the end of this article, you'll gain insights into:
The importance of clean code in enhancing code maintainability and scalability.
Key principles and best practices for writing clean and readable JavaScript code.
Strategies for refactoring and optimizing existing codebases to improve clarity and efficiency.
Tools and techniques for automated code quality checks and continuous integration.
The impact of clean code on collaboration, debugging, and long-term project success.
Key Terms to Understand
Before we dive into the strategies for writing clean code, let's clarify some key terms:
Clean Code: Code that is easy to read, understand, and maintain. It follows established coding conventions and best practices, making it accessible to other developers.
Refactoring: The process of restructuring existing code without changing its external behavior to improve readability, maintainability, or performance.
Code Quality Checks: Automated processes that analyze code for adherence to coding standards, identifying potential issues or areas for improvement.
Continuous Integration: A development practice where code changes are automatically tested and integrated into the main codebase, ensuring that the application remains functional and stable.
The Importance of Clean Code in Enhancing Code Maintainability and Scalability
Clean code serves as the foundation upon which successful software projects are built. Its significance extends far beyond mere aesthetics; it profoundly impacts the maintainability and scalability of a codebase. Let's delve into the extensive implications of clean code in these critical areas:
1. Maintainability
Maintainability refers to the ease with which a software system can be modified, extended, or debugged over its lifecycle. Clean code plays a pivotal role in enhancing maintainability through several key mechanisms:
Readability: Clean code is inherently readable, with clear and descriptive names for variables, functions, and classes. This readability reduces the cognitive load on developers, making it easier to understand the codebase and navigate through it. When developers can grasp the intent of the code at a glance, they can quickly identify areas that need modification or improvement.
Modularity: Clean code promotes modularity by adhering to the Single Responsibility Principle (SRP) and other SOLID principles. By breaking down complex systems into smaller, self-contained modules, clean code reduces dependencies and isolates changes. This modularity enables developers to make targeted modifications without risking unintended side effects elsewhere in the codebase.
Consistency: Clean code follows consistent coding conventions and style guidelines, ensuring uniformity across the codebase. Consistency reduces confusion and ambiguity, allowing developers to predictably locate and modify code segments. When every developer follows the same conventions, collaboration becomes smoother, and the risk of introducing errors due to misunderstanding or miscommunication decreases.
Documentation: While clean code prioritizes self-documentation through clear naming and expressive syntax, it also encourages the inclusion of supplemental documentation where necessary. Documentation helps developers understand the rationale behind design decisions, the expected behavior of functions and modules, and any potential caveats or constraints. Well-documented code accelerates onboarding for new team members and facilitates knowledge transfer within the team.
In essence, clean code fosters maintainability by minimizing cognitive overhead, promoting modularity and consistency, and facilitating effective communication through self-documentation.
2. Scalability:
Scalability refers to a system's ability to accommodate increasing workload or demand while maintaining performance and reliability. Clean code lays a robust foundation for scalability by addressing key scalability concerns:
Flexibility: Clean code is inherently flexible and adaptable to changing requirements and evolving business needs. Its modular structure allows developers to add or modify functionality with minimal disruption to existing code. As new features are introduced or existing ones are enhanced, clean code can accommodate these changes gracefully, without introducing excessive technical debt or complexity.
Performance Optimization: Clean code emphasizes efficiency and performance optimization from the outset. By writing code that is clear, concise, and focused on the task at hand, developers can identify opportunities for optimization and implement them judiciously. Optimized code reduces resource consumption, enhances responsiveness, and ensures that the system can scale gracefully to handle increased demand.
Testing and Validation: Clean code promotes testability by decoupling components and minimizing dependencies. This testability enables developers to create comprehensive test suites that validate the behavior of the system under various conditions. By thoroughly testing each module in isolation and as part of the integrated system, developers can identify scalability bottlenecks early in the development process and address them proactively.
Adaptability: Clean code embraces principles such as Dependency Inversion and Open/Closed Principle, which encourage loose coupling and high cohesion. These principles enable developers to introduce new functionality or replace existing components without requiring extensive modifications to the existing codebase. As the system evolves and grows, clean code remains adaptable, allowing it to scale seamlessly without becoming brittle or unwieldy.
Essentially, clean code facilitates scalability by fostering flexibility, performance optimization, testability, and adaptability. By adhering to clean coding practices, developers can build software systems that are not only maintainable but also capable of scaling to meet the demands of an ever-changing technological landscape.
Key Principles and Best Practices for Writing Clean and Readable JavaScript Code
Writing clean and readable JavaScript code is essential for fostering maintainability, collaboration, and overall code quality in software projects. Let's explore the key principles and best practices that contribute to clean and readable JavaScript code in extensive detail:
1. Single Responsibility Principle (SRP)
The Single Responsibility Principle dictates that a module, function, or class should have only one reason to change. This principle encourages developers to decompose complex functionalities into smaller, more focused units, each responsible for a single task. By adhering to SRP, code becomes more modular, reusable, and easier to understand and maintain.
2. Descriptive Naming
Meaningful and descriptive names for variables, functions, classes, and methods are crucial for enhancing code readability. Names should accurately reflect the purpose and functionality of the entity they represent. Avoid cryptic abbreviations or overly generic names, opting instead for clarity and expressiveness. Well-named identifiers serve as self-documenting code, reducing the need for additional comments or documentation.
3. Consistent Coding Style
Consistency in coding style and formatting improves code readability and promotes maintainability. Adopting a consistent coding style across the codebase ensures that developers can quickly understand and navigate unfamiliar sections of code. Consistent indentation, spacing, naming conventions, and code organization contribute to a cohesive and harmonious codebase, facilitating collaboration and reducing cognitive overhead.
4. Modularization and Encapsulation
Breaking down complex systems into smaller, modular components promotes code reusability, maintainability, and scalability. Modules should encapsulate related functionality and expose a clear and concise interface for interaction with other parts of the system. Modularization reduces dependencies and isolates changes, making it easier to reason about and modify individual components without impacting the entire codebase.
5. Documentation
While clean code prioritizes self-documentation through clear naming and expressive syntax, supplemental documentation is essential for providing context, rationale, and usage guidelines. Comments should explain the intent, behavior, and constraints of functions, classes, and modules. Documentation also includes high-level overviews, API references, and examples to guide developers in using and extending the codebase effectively.
6. Error Handling
Robust error handling is crucial for ensuring the reliability and stability of JavaScript applications. Properly handled errors prevent unexpected failures and provide informative feedback to users and developers. Error messages should be clear, informative, and actionable, guiding users toward resolution steps or recovery options. Additionally, error handling code should be separated from the main logic, promoting code readability and maintainability.
7. Testing and Quality Assurance
Comprehensive testing, including unit tests, integration tests, and end-to-end tests, validates the correctness and functionality of JavaScript code. Test-driven development (TDD) encourages writing tests before writing code, ensuring that code meets specified requirements and behaves as expected. Automated testing frameworks such as Jest, Mocha, and Jasmine facilitate test creation and execution, enabling developers to catch bugs early and maintain code quality over time.
8. Continuous Integration and Deployment (CI/CD)
Continuous integration and deployment pipelines automate the process of testing, building, and deploying JavaScript applications, reducing manual effort and minimizing the risk of human error. CI/CD pipelines enforce coding standards, run automated tests, and deploy code changes to production environments in a systematic and controlled manner. By integrating CI/CD into the development workflow, teams can accelerate the delivery of high-quality software while maintaining code readability and stability.
In summary, adhering to key principles and best practices such as SRP, descriptive naming, consistent coding style, modularization, documentation, error handling, testing, and CI/CD promotes clean and readable JavaScript code. By prioritizing clarity, simplicity, and maintainability, developers can create codebases that are easier to understand, collaborate on, and extend, ultimately leading to higher-quality software products.
Strategies for Refactoring and Optimizing Existing Codebases to Improve Clarity and Efficiency
Refactoring and optimizing existing codebases are essential practices in software development for maintaining code quality, improving performance, and enhancing developer productivity. Let's explore extensive strategies for refactoring and optimizing existing codebases to achieve clarity and efficiency:
1. Identify Code Smells
The first step in refactoring is to identify code smells—indicators of potential design flaws or areas for improvement in the codebase. Common code smells include duplicated code, long methods or functions, excessive nesting, and tight coupling between components. By conducting code reviews, static code analysis, and automated linting, developers can pinpoint areas of the codebase that require attention and refinement.
2. Break Down Complex Functions
Complex functions or methods are often difficult to understand, maintain, and test. Refactoring complex functions into smaller, more focused units improves code clarity and promotes reusability. By breaking down functionality into cohesive and modular components, developers can isolate concerns, reduce dependencies, and make code more understandable and maintainable.
3. Extract Reusable Components
Identify common patterns or functionality that are repeated across the codebase and extract them into reusable components or modules. Reusable components promote code reuse, reduce duplication, and simplify maintenance. By encapsulating shared functionality in standalone modules, developers can streamline development efforts, improve consistency, and ensure that changes are propagated consistently throughout the codebase.
4. Improve Naming and Documentation
Clear and descriptive naming is crucial for enhancing code readability and understanding. Refactor ambiguous or misleading names to accurately reflect the purpose and functionality of variables, functions, classes, and modules. Additionally, supplement code with comprehensive documentation, including comments, docstrings, and inline explanations, to provide context, usage guidelines, and rationale for design decisions.
5. Optimize Performance Bottlenecks
Identify performance bottlenecks or areas of inefficiency in the codebase and optimize them to improve overall performance and responsiveness. Common performance optimization techniques include reducing unnecessary computations, optimizing data structures and algorithms, caching frequently accessed data, and minimizing I/O operations. By profiling the codebase and identifying hotspots, developers can prioritize optimizations to achieve maximum impact.
6. Refactor for Testability
Design code with testability in mind, making it easier to write, maintain, and execute automated tests. Refactor tightly coupled components to decouple dependencies and improve isolation. Use dependency injection, mocking, and stubbing techniques to facilitate unit testing and minimize external dependencies. By designing code that is modular, loosely coupled, and easily testable, developers can ensure code quality and reliability through comprehensive test coverage.
7. Leverage Modern Language Features and Libraries:=
Take advantage of modern language features, syntactic sugar, and libraries to simplify code and improve readability. Use features such as destructuring, arrow functions, template literals, and object shorthand notation to write concise and expressive code. Additionally, leverage utility libraries and frameworks, such as lodash, Ramda, or Underscore.js, to streamline common operations and reduce boilerplate code.
8. Adopt Design Patterns and Architectural Principles
Apply design patterns and architectural principles, such as MVC (Model-View-Controller), MVVM (Model-View-ViewModel), or SOLID principles, to guide the refactoring process and improve code structure and organization. Design patterns provide proven solutions to common design problems, while architectural principles promote modularity, flexibility, and maintainability. By adhering to established patterns and principles, developers can ensure that the codebase remains cohesive, scalable, and adaptable to changing requirements.
In summary, refactoring and optimizing existing codebases require a systematic approach that involves identifying code smells, breaking down complexity, extracting reusable components, improving naming and documentation, optimizing performance, designing for testability, leveraging modern language features and libraries, and adopting design patterns and architectural principles. By continuously refining and improving the codebase, developers can enhance clarity, efficiency, and maintainability, ultimately delivering high-quality software products that meet the evolving needs of users and stakeholders.
Tools and Techniques for Automated Code Quality Checks and Continuous Integration
Automated code quality checks and continuous integration (CI) are integral components of modern software development workflows, enabling teams to maintain code quality, detect issues early, and streamline the development process. Let's explore extensively the tools and techniques used for automated code quality checks and continuous integration:
1. Linting Tools
Linting tools analyze source code for potential errors, stylistic inconsistencies, and adherence to coding standards and best practices. Popular JavaScript linting tools include ESLint, JSHint, and TSLint (for TypeScript). These tools provide configurable rule sets that can be tailored to enforce specific coding conventions, such as indentation, variable naming, whitespace usage, and more. Linting tools can be integrated into code editors, version control systems, and CI pipelines to ensure consistent code quality across the entire codebase.
2. Code Formatting Tools
Code formatting tools automate the process of formatting source code according to predefined style guidelines. Prettier is a widely used code formatting tool that supports multiple programming languages, including JavaScript. Prettier automatically formats code based on configurable rules, eliminating debates over coding style and ensuring consistent formatting throughout the codebase. Integrating code formatting tools into the development workflow ensures that code is consistently formatted and adheres to established coding standards.
3. Unit Testing Frameworks
Unit testing frameworks enable developers to write and execute automated tests to validate individual units of code (e.g., functions, methods, or classes) in isolation. Popular JavaScript unit testing frameworks include Jest, Mocha, Jasmine, and Ava. These frameworks provide utilities for defining test suites, writing test cases, and asserting expected behavior. By automating unit tests, developers can verify code correctness, detect regressions, and ensure that changes do not introduce unintended side effects.
4. Static Code Analysis Tools
Static code analysis tools analyze source code for potential defects, security vulnerabilities, and performance issues without executing the code. SonarQube, CodeClimate, and ESLint (with specific plugins) are examples of static code analysis tools commonly used in JavaScript projects. These tools perform comprehensive code scans and provide actionable insights into code quality, maintainability, security, and compliance. Integrating static code analysis into CI pipelines enables early detection of code issues and facilitates continuous improvement of code quality.
5. Continuous Integration (CI) Services
Continuous integration services automate the process of integrating code changes into a shared repository and running automated tests and code quality checks on every commit. Popular CI services for JavaScript projects include Jenkins, Travis CI, CircleCI, and GitHub Actions. These services support configuring pipelines that define the sequence of steps to be executed, such as code linting, unit testing, static code analysis, code coverage analysis, and deployment to staging environments. Continuous integration ensures that code changes are validated and integrated frequently, reducing the risk of integration conflicts and ensuring the stability and reliability of the codebase.
6. Continuous Deployment (CD) Pipelines
Continuous deployment pipelines automate the process of deploying code changes to production environments after passing all automated tests and quality checks. CD pipelines typically include stages for building artifacts, running additional integration or end-to-end tests, and deploying changes to production servers or cloud platforms. Tools like AWS CodeDeploy, Google Cloud Build, and Azure DevOps provide capabilities for orchestrating continuous deployment pipelines and managing application deployments at scale.
7. Code Review Tools
Code review tools facilitate collaborative code reviews by providing features for commenting on code changes, suggesting improvements, and tracking review status. GitHub, GitLab, and Bitbucket offer built-in code review features as part of their version control platforms. Additionally, tools like Reviewable, Gerrit, and Crucible provide advanced code review capabilities, including customizable workflows, automated review assignments, and integration with CI pipelines. Code reviews help identify code quality issues, share knowledge among team members, and ensure that changes meet quality standards before being merged into the main codebase.
In summary, automated code quality checks and continuous integration are essential practices in modern software development, enabling teams to maintain code quality, detect issues early, and accelerate the delivery of high-quality software products. By leveraging a combination of linting tools, code formatting tools, unit testing frameworks, static code analysis tools, CI services, CD pipelines, and code review tools, teams can establish robust development workflows that promote collaboration, code quality, and continuous improvement.
The Impact of Clean Code on Collaboration, Debugging, and Long-Term Project Success
Clean code is not just about aesthetics or adherence to coding conventions—it has a profound impact on various aspects of software development, including collaboration, debugging, and long-term project success. Let's explore extensively how clean code influences these critical areas:
1. Collaboration
Clean code fosters collaboration among team members by promoting clarity, readability, and maintainability. Here's how clean code facilitates collaboration:
Clarity and Readability: Clean code is easy to understand at a glance, with clear and descriptive names for variables, functions, and classes. When code is readable, team members can quickly grasp its intent and functionality, reducing the time and effort required to understand and modify it. This clarity accelerates collaboration by minimizing misunderstandings and enabling effective communication among team members.
Consistency: Clean code adheres to consistent coding conventions and style guidelines across the codebase. Consistency reduces cognitive overhead by providing a familiar and predictable coding environment for developers. When everyone follows the same conventions, collaboration becomes smoother, and the risk of miscommunication or conflicting coding styles diminishes. Consistent codebases are easier to navigate and contribute to, fostering a cohesive and unified development team.
Modularity and Reusability: Clean code promotes modularity by breaking down complex systems into smaller, self-contained modules. Modular codebases enable parallel development, allowing team members to work on different parts of the system independently without stepping on each other's toes. Additionally, reusable components simplify collaboration by providing common building blocks that can be shared across projects, teams, and organizations. By leveraging existing components, developers can focus on solving unique problems rather than reinventing the wheel.
Ease of Onboarding: Clean code accelerates the onboarding process for new team members by providing a clear and structured codebase to navigate. New developers can quickly orient themselves, understand the codebase's architecture and design patterns, and start contributing effectively from day one. Well-documented clean codebases further facilitate onboarding by providing supplemental explanations, usage guidelines, and examples to guide newcomers through the codebase.
In essence, clean code promotes collaboration by fostering clarity, consistency, modularity, and ease of onboarding, enabling development teams to work together more effectively and efficiently.
2. Debugging
Clean code simplifies the debugging process by reducing complexity, isolating issues, and providing clear diagnostic information. Here's how clean code facilitates debugging:
Reduced Cognitive Load: Clean code minimizes cognitive load by eliminating unnecessary complexity, redundant logic, and obscure constructs. When code is clean and well-structured, developers can focus their attention on understanding the problem at hand rather than deciphering convoluted code. This clarity accelerates the debugging process by enabling developers to identify and isolate issues more quickly.
Clear Intent and Documentation: Clean code communicates its intent and purpose clearly through meaningful variable names, descriptive comments, and expressive syntax. When code is self-documenting, developers can understand its behavior and assumptions without resorting to external documentation or additional context. This clarity aids in debugging by providing insights into the developer's intentions and expectations, facilitating the identification of potential sources of errors.
Isolation of Concerns: Clean code follows principles such as the Single Responsibility Principle (SRP), which encourages breaking down complex functionalities into smaller, focused units. By isolating concerns and minimizing dependencies, clean code makes it easier to localize and troubleshoot issues. When each component has a clear and well-defined purpose, developers can narrow down the scope of their investigation and pinpoint the root cause of problems more effectively.
Comprehensive Testing: Clean code is accompanied by comprehensive test suites that validate its behavior under various conditions. Automated unit tests, integration tests, and end-to-end tests ensure that code changes do not introduce regressions or unintended side effects. By running tests regularly as part of the development workflow, developers can catch bugs early, validate assumptions, and maintain code quality over time.
In summary, clean code streamlines the debugging process by reducing cognitive load, providing clear intent and documentation, isolating concerns, and facilitating comprehensive testing. By adhering to clean coding practices, developers can diagnose and resolve issues more efficiently, ensuring the stability and reliability of their software projects.
3. Long-Term Project Success
Clean code lays the foundation for long-term project success by promoting maintainability, scalability, and adaptability. Here's how clean code contributes to the success of software projects over the long term:
Maintainability: Clean code is easier to maintain and extend over time, reducing the cost and effort associated with ongoing development and maintenance activities. When code is well-structured, modular, and documented, developers can make changes confidently without introducing unintended side effects or breaking existing functionality. This maintainability ensures that software projects remain responsive to evolving requirements and market demands.
Scalability: Clean code scales gracefully as projects grow in size and complexity, enabling developers to add new features, refactor existing code, and optimize performance without compromising stability or readability. By following principles such as modularity, encapsulation, and separation of concerns, clean codebases remain flexible and adaptable to changing business needs. This scalability allows software projects to evolve iteratively, accommodating new features, technologies, and user demands over time.
Adaptability: Clean code promotes adaptability by facilitating continuous improvement, experimentation, and innovation. When code is clean and well-structured, developers can refactor, optimize, and experiment with confidence, knowing that the codebase can absorb changes without becoming brittle or unmaintainable. This adaptability enables software projects to evolve in response to market feedback, emerging technologies, and competitive pressures, ensuring their relevance and longevity in the ever-changing landscape of software development.
In essence, clean code is a cornerstone of long-term project success, providing a solid foundation for maintainability, scalability, and adaptability. By prioritizing clean coding practices, development teams can build software projects that stand the test of time, delivering value to users and stakeholders for years to come.
To wrap it up, a clean code has a profound impact on collaboration, debugging, and long-term project success in software development. By promoting clarity, readability, maintainability, and scalability, clean code enables development teams to collaborate effectively, debug efficiently, and achieve sustainable success over the life of their projects. Prioritizing clean coding practices is essential for building high-quality software that meets the needs of users and stakeholders, both now and in the future.
Summary
Clean code is the cornerstone of successful software development, impacting code maintainability, scalability, and developer productivity. By adhering to principles such as the SOLID principles and following coding conventions, developers can design modular and maintainable codebases. Strategies for refactoring and optimizing code, including identifying code smells and breaking down complex functions, further enhance code quality and reusability.
In addition to manual practices, automated tools like ESLint and testing suites such as Jest play a crucial role in maintaining code quality and preventing regressions. Clean code not only fosters collaboration among team members but also simplifies debugging processes, reducing the time and effort required to identify and fix issues.
In conclusion, clean code is more than just a coding style—it's a mindset that leads to excellence in software development. By embracing the principles and best practices of clean coding, developers can build JavaScript applications that are robust, scalable, and a pleasure to work with. Let's unite in embracing the power of clean code to elevate the quality of our JavaScript applications together!
Frequently Asked Questions (FAQ)
1. What is clean code, and why is it important in software development?
Clean code refers to well-structured, readable, and maintainable code that follows established coding conventions and best practices. It is important in software development because it enhances code maintainability, scalability, and developer productivity. Clean code reduces technical debt, fosters collaboration among team members, and simplifies debugging and troubleshooting processes.
2. How does clean code impact collaboration among development teams?
Clean code promotes collaboration among development teams by making code reviews and pair programming more effective and efficient. Clear and readable code reduces the cognitive overhead for team members, enabling them to understand, review, and contribute to the codebase more easily. Consistent coding conventions and style guidelines foster a unified development environment, reducing conflicts and misunderstandings among team members.
3. What are some common code smells and anti-patterns that indicate the need for refactoring?
Common code smells and anti-patterns include duplicated code, long methods or functions, tight coupling between components, and excessive nesting or complexity. These indicators suggest areas of the codebase that could benefit from refactoring to improve code quality, readability, and maintainability. Refactoring aims to address these issues by breaking down complex code into smaller, more manageable units, extracting reusable components, and reducing dependencies.
4. How can developers ensure adherence to clean coding principles and best practices?
Developers can ensure adherence to clean coding principles and best practices by following established guidelines, such as the SOLID principles and coding conventions like the Airbnb JavaScript Style Guide or Google JavaScript Style Guide. Additionally, integrating linting tools like ESLint or JSHint into the development workflow helps enforce coding standards and detect potential errors or inconsistencies automatically. Writing self-documenting code with meaningful variable names, descriptive comments, and clear function names further enhances code readability and maintainability.
5. What role do automated code quality checks and continuous integration play in maintaining clean code?
Automated code quality checks and continuous integration (CI) are essential components of maintaining clean code. Linting tools, testing suites like Jest or Mocha, and static code analysis tools help identify and prevent common coding errors, code smells, and anti-patterns. CI pipelines automate the process of running these checks on every code change, ensuring that code quality standards are upheld consistently across the codebase. By integrating automated code quality checks into the development workflow, developers can catch issues early, reduce technical debt, and maintain a high level of code quality over time.
Subscribe to my newsletter
Read articles from Adeoye David directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
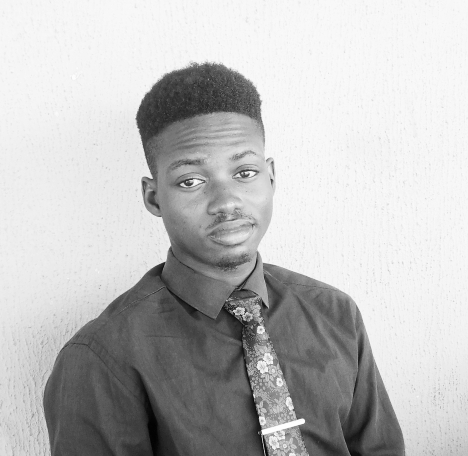
Adeoye David
Adeoye David
I'm David, a passionate backend developer and avid technical writer dedicated to crafting clean, efficient, and scalable software solutions. With a deep-rooted love for code and a knack for communicating complex technical concepts in a clear and concise manner, I thrive in the intersection of technology and writing. As a backend developer, I specialize in building robust and reliable server-side applications using technologies like Node.js, Express.js, and MongoDB. Whether it's architecting scalable APIs, optimizing database performance, or implementing secure authentication mechanisms, I'm always up for the challenge of tackling backend complexities. Beyond my coding endeavors, I find immense joy in the art of technical writing. I believe that effective documentation is just as important as writing code—it empowers users, fosters collaboration, and ensures the long-term success of software projects. From comprehensive API guides to in-depth tutorials, I strive to create informative and accessible content that educates and inspires fellow developers. On this platform, you'll find a blend of my technical insights, coding adventures, and musings on software engineering best practices. Whether you're a seasoned developer seeking to deepen your knowledge or a newcomer navigating the world of backend development, I invite you to join me on this journey of exploration and discovery.