Exploring the Power of Node.js REPL: A Developer's Guide

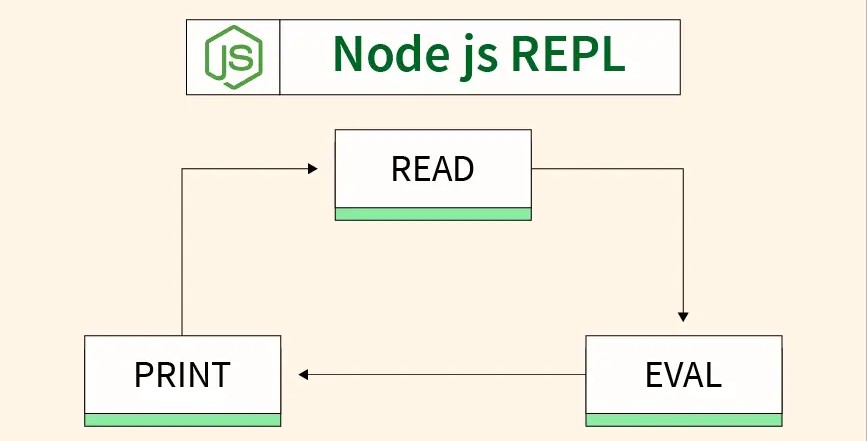
Introduction
Node.js, with its efficient runtime environment and vast ecosystem, has become a go-to choice for developers worldwide. At the core of Node.js lies its Read, Evaluate, Print, Loop (REPL) cycle, a powerful tool that facilitates rapid prototyping, debugging, and learning. In this article, we'll delve into the workings of Node.js REPL, understand its components, and explore how it powers interactive JavaScript development.
Understanding Node.js REPL (Read , Evaluate , Print , Loop)
Read: The first step in the REPL cycle involves reading input from the user or a script. This input typically consists of JavaScript expressions, statements, or functions.
Evaluate: Once input is received, Node.js evaluates the entered code, executing it and producing results in real-time. This immediate feedback loop allows developers to experiment with code snippets and quickly observe outcomes.
Print: After evaluation, Node.js prints the result of the executed code to the console. This output could be anything from simple values or objects to the result of complex operations or functions.
Loop: With the result displayed, the REPL cycle continues, awaiting further input from the user. This iterative process enables developers to refine their code incrementally, testing different approaches and troubleshooting errors.
Let's calculate the factorial of a number using Node.js REPL:
function factorial(n) {
if (n === 0 || n === 1) {
return 1;
} else {
return n * factorial(n - 1);
}
}
factorial(5);
Upon evaluation, the REPL would display the factorial of 5, which is 120, providing instant feedback on our code.
Read -> Evaluate -> Print -> Loop
^ | | |
|_________|____________|__________|
How Node.js Works
Node.js operates on a single-threaded, event-driven architecture, making it highly efficient for handling I/O operations asynchronously. Here's a rough overview of how Node.js works:
Event Loop: Node.js utilizes an event loop to handle asynchronous operations. When an asynchronous task completes, it triggers a callback function, allowing Node.js to continue executing other tasks in the meantime.
Libuv: Node.js leverages the Libuv library to manage asynchronous I/O operations efficiently. Libuv provides a platform-independent abstraction layer for file system, networking, and other system-related tasks.
V8 Engine: Node.js uses the V8 JavaScript engine, developed by Google, to execute JavaScript code. V8 compiles JavaScript into machine code, optimizing performance and enabling fast execution.
Modules: Node.js follows a modular approach, allowing developers to organize code into reusable modules. These modules can be imported using the
require()
function, facilitating code organization and reusability.
How does the command line interface (CMD) on desktop systems support Node.js REPL?
The CMD provides a command-line interface for executing Node.js scripts and accessing the Node.js REPL directly. By navigating to the directory containing Node.js files and typing
node
followed by the Enter key, users can enter the Node.js REPL environment and interactively execute JavaScript code. Additionally, users can run Node.js scripts directly from the CMD by specifying the script filename after thenode
command, allowing for seamless development and debugging.
Subscribe to my newsletter
Read articles from Vishwajit Vm directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by

Vishwajit Vm
Vishwajit Vm
Hi there, I'm Vishwajit vm, a skilled Laravel and PHP developer. With years of experience in the field, I have a proven track record of delivering high-quality projects on time and within budget. I am passionate about using my expertise in Laravel and PHP to create innovative and efficient web applications that meet the unique needs of each project. I am always open to collaboration and am eager to work with others on any project related to Laravel and PHP. My goal is to deliver the best possible results for each project, using my deep understanding of the framework and its capabilities to create custom solutions that meet the specific needs of each project. In my free time, I enjoy pursuing my hobbies of photography, cycling, and playing video games. I also have a YouTube channel where I share my knowledge and insights on Laravel and PHP development, offering valuable tips and advice to others in the field. If you're looking for a skilled Laravel and PHP developer who is passionate about their work and dedicated to delivering the best possible results, feel free to reach out to me at vishwajitmall50@gmail.com or call me at 91-8920352115. I'm always happy to discuss potential projects and collaborations.