Pointers & Memory

Table of contents
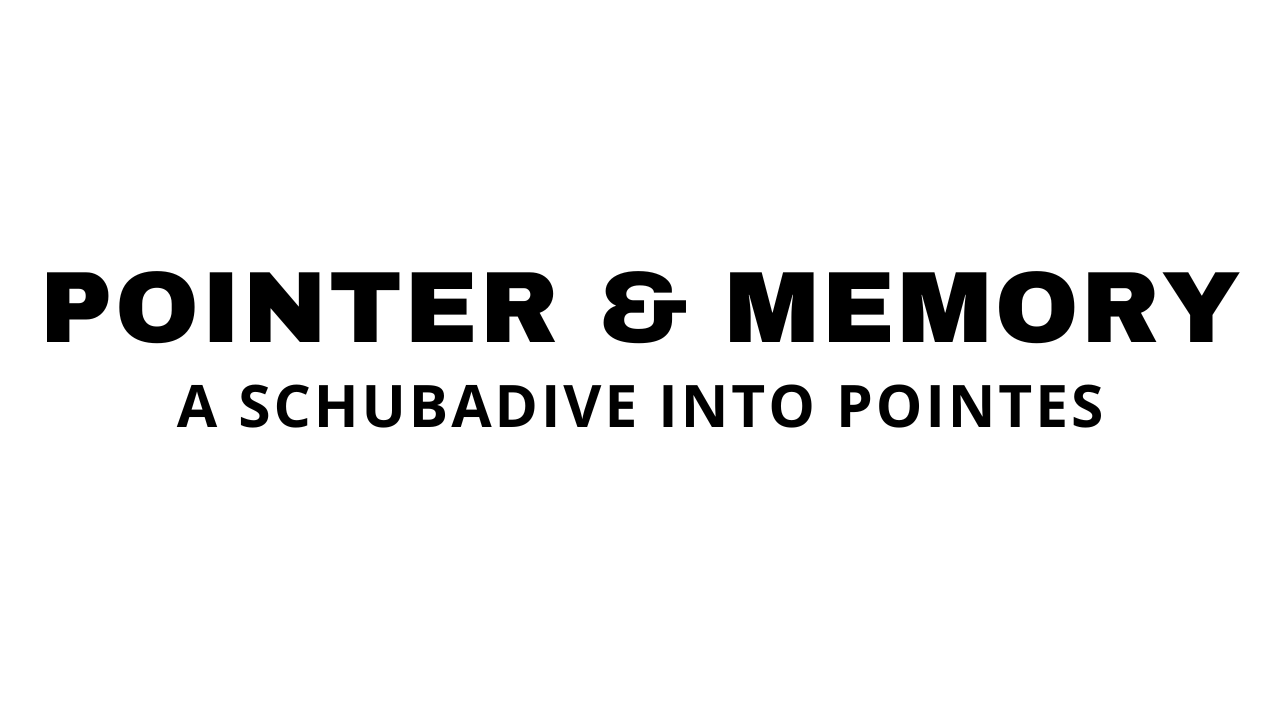
it's just an address, a way to locate where something that is stored in your computer's memory. Just like GPS helps you find your way around town, pointers help your program find its way around memory.
There are three main operators
&(ampersand) gives the memory address
*(asterisk) There are 2 cases
Help define the pointer type
Dereference a pointer to get the object
->(arrow operator) Dereference a pointer and access the methods and variable
Here are some examples!
int myVariable = 42;
int *myPointer = &myVariable;
int myVariableRef = *myPointer;
struct MyStruct {
int myVariable;
};
struct MyStruct myStruct = {.myVariable = 24};
struct MyStruct *myStructPointer = &myStruct;
int mystructVariableRef = myStructPointer->myVariable; // *myStructPointer.myVariable
Types of Pointers
null pointers
void pointers
pointers to basic data types
pointers to arrays
pointers to functions
pointer to pointers
Void Pointer
They're like shapeshifters; they can point to anything! But be vigilant, you can't directly dereference them
void *voidPointer;
Null Pointer
These bad boys don't point anywhere; they're like empty signposts waiting for a destination
int *nullPointer = NULL;
Pointer to Basic Data Type
These are your go-to pointers, pointing to integers, floats, chars, you name it!
int *intPointer;
char *charPointer;
Pointer to Function
Yep, you heard me right! Pointers can even point to functions
void (*functionPointer)(int);
int **pointerToPointer;
Pointer to Array
int arr[5] = {1, 2, 3, 4, 5};
int *arrPointer = arr;
Pointer to Pointer
int **pointerToPointer;
Some Complex examples of pointer!
#include <stdio.h>
// Define a function type that takes a pointer to a pointer and returns an int
typedef int (*FunctionPtr)(int**);
// Example function: Takes a pointer to a pointer and returns a function pointer
int add(int** ptr) {
return **ptr + 10;
}
int main() {
int x = 5;
int* ptr1 = &x; // Pointer to an integer
FunctionPtr funcPtr = &add; // Function pointer type
int result = funcPtr(&ptr1);
printf("Result: %d\n", result); // Output: Result: 15
return 0;
}
typedef int (*(*MyFunctionPtr)(void (*)(int**), void (*)(void (*)()), int***))[];
MyFunctionPtr
is the typedef name for the function pointer type.The function pointer takes three parameters:
A function pointer that takes an
int**
parameter.A function pointer that takes no parameters.
An
int***
pointer to pointer parameter.
The function pointer returns an array of pointers to functions that return integers.
Memory Allocation!
Pointer and Memory go hand-in-hand!
Stack | Heap |
It’s a temporary memory allocation scheme where the data members are accessible only if the method( ) that contained them is currently running | Heap memory is also not as threaded-safe as Stack-memory because data stored in Heap-memory are visible to all threads! Can lead to memory leak! |
It allocates or de-allocates the memory automatically as soon as the corresponding method completes its execution | This memory allocation scheme is different from the Stack-space allocation, here no automatic deallocation feature is provided |
What does memory have to do with pointer!
In the case of heap allocation there are some big problems
What if we forget to deallocate a variable?
What if our pointer gets deallocated by another thread? ( remember the property of heap )
How do you safely share pointer between different parts of the code ?
Todo: Think about more problem!
Smart pointers to the rescue?
std::unique_ptr
think of it as a pointer with a unique ownership. When thestd::unique_ptr
goes out of scope, it automatically deallocates the memory it points to.
#include <memory>
std::unique_ptr<int> uniquePtr(new int(42));
std::shared_ptr
allows multiple pointers to share ownership of the same resource. When all thestd::shared_ptr
s pointing to the resource go out of scope, the memory is automatically deallocated.
#include <memory>
std::shared_ptr<int> sharedPtr1(new int(42));
std::shared_ptr<int> sharedPtr2 = sharedPtr1;
std::weak_ptr
It allows you to observe the resource held by astd::shared_ptr
without affecting its lifetime.
#include <memory>
std::shared_ptr<int> sharedPtr(new int(42));
std::weak_ptr<int> weakPtr = sharedPtr;
Todo: comment below
more problems that occur due to pointers?
other ways to solve the pointer crisis?
Subscribe to my newsletter
Read articles from Ankan Roy directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by

Ankan Roy
Ankan Roy
Hi, Ankan here! I like to write about smart computers (AI), numbers (that's statistics!), and anything else that catches my eye. I used to wish I could share what's in my head just by writing it down. So, I'm trying to make things that I hope will make me happy and be useful to the world!