Leetcode 118. Pascal's Triangle

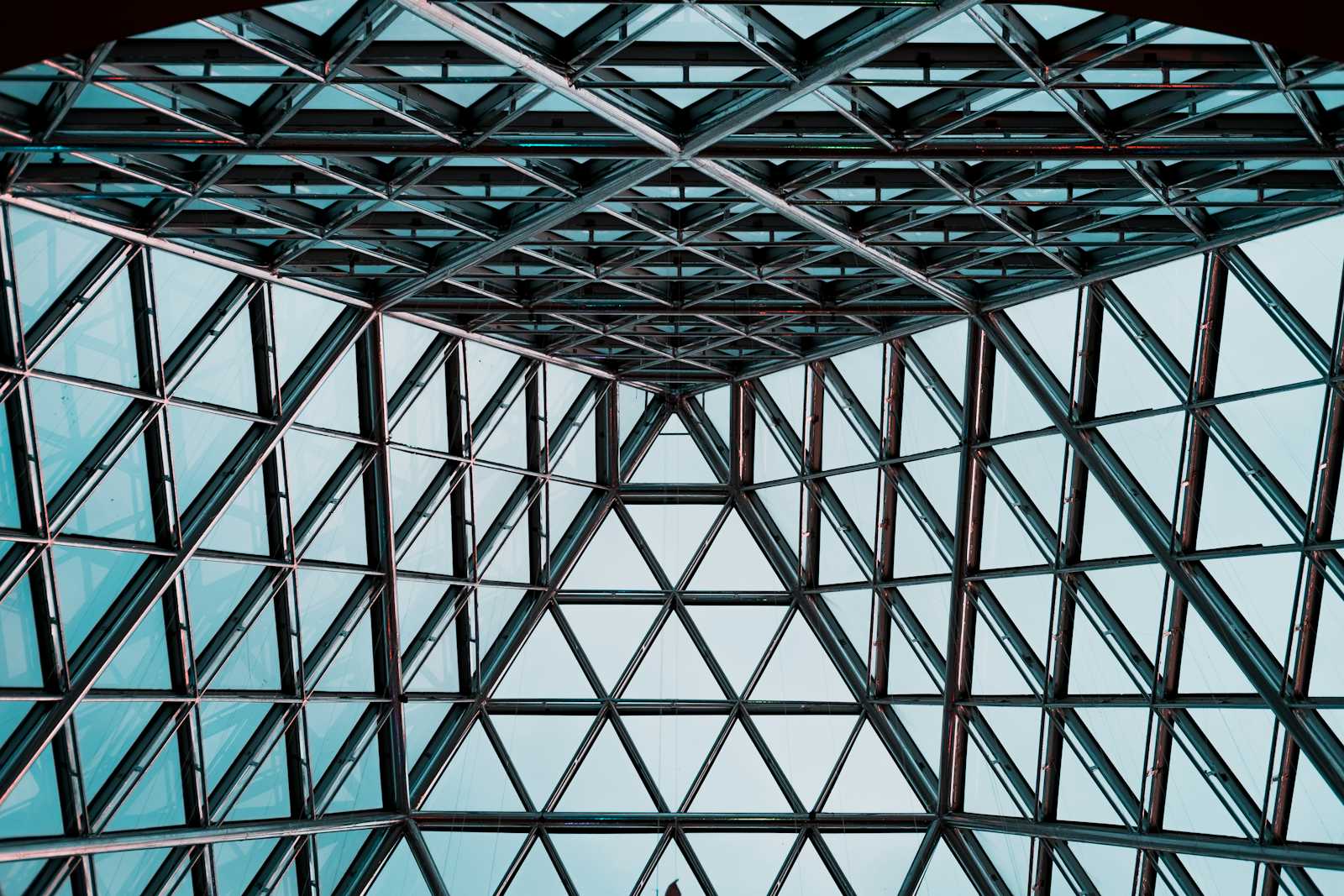
Description:
Pascal's Triangle is a mathematical concept that is represented as a triangular array of binomial coefficients. Each number in the triangle is the sum of the two directly above it. The first and last numbers in each row are always 1.
Variation 1: Given row number r and column number c. Print the element at position (r, c) in Pascal’s triangle.
Variation 2: Given the row number n. Print the n-th row of Pascal’s triangle.
Variation 3: Given the number of rows n. Print the first n rows of Pascal’s triangle.
Example:
Variation 1: Input: row = 5, col = 3
Output: 10
Variation 2: Input: row = 10
Output: [1, 9, 36, 84, 126, 126, 84, 36, 9, 1]
Variation 3: Input: row = 6
Output: [[1, 0, 0, 0, 0], [1, 1, 0, 0, 0], [1, 2, 1, 0, 0], [1, 3, 3, 1, 0], [1, 4, 6, 4, 1]]
Constraints:
1 <= row <= 30
Solution:
Variation 1: N choose R
Given a row number n
and a column number r
, the task is to find the value of the element at row n
and column r
in Pascal's Triangle.
Intuition: The value of an element in Pascal's Triangle at row n
and column r
can be calculated using the formula C(n-1, r-1)
, where C(n, r)
is the binomial coefficient n choose r
.
Algorithm:
Subtract 1 from both
n
andr
.Initialize a result variable to 1.
Iterate from 0 to
r-1
and calculate the result using the formula(n - i) * res / (i + 1)
.Return the result.
Pseudo Code:
row = row - 1
col = col - 1
res = 1
for i = 0 to col-1:
res = res * (row - i)
res = res / (i + 1)
return res
Time Complexity:
O(c), where c = given column number.
Reason: We are running a loop for r times, where r is c-1.
Space Complexity:
O(1) as we are not using any extra space.
Variation 2: Get nth Row
Given a row number n
, the task is to print the entire nth row of Pascal's Triangle.
Intuition: Similar to Variation 1, calculate each element of the row using the formula for binomial coefficients and store them in an array.
Algorithm:
Create an array of size
n
to store the row elements.Initialize the first element of the row to 1.
Iterate from 1 to
n-1
and calculate each element using the formula(row - i) * ans / i
.Store each element in the array.
Return the array.
Pseudo Code:
opArr[0] = 1
ans = 1
for i = 1 to row-1:
ans = ans * (row - i)
ans = ans / i
opArr[i] = ans
return opArr
Time Complexity:
O(N) where N = given row number. Here we are using only a single loop.
Space Complexity:
O(1) as we are not using any extra space.
Variation 3: Get Pascal's Triangle
Given a number n
, the task is to generate the first n
rows of Pascal's Triangle.
Intuition: Generate each row of Pascal's Triangle by calculating the elements using Variation 1 and Variation 2.
Algorithm:
Initialize an empty list to store the rows of Pascal's Triangle.
Iterate from 1 to
n
and generate each row using Variation 2.Add each row to the list.
Return the list.
Pseudo Code:
ans = empty list
for row = 1 to n-1:
tempLst = empty list
for col = 1 to n-1:
tempLst.add(NcR(row, col))
ans.add(tempLst)
return ans
Time Complexity:
O(n2), where n = number of rows(given).
Reason: We are generating a row for each single row. The number of rows is n. And generating an entire row takes O(n) time complexity.
Space Complexity:
In this case, we are only using space to store the answer. That is why space complexity can still be considered as O(1).
Conclusion:
Pascal's Triangle is a fascinating mathematical concept that has various applications in combinatorics and probability. In this blog post, we explored different variations of the Pascal's Triangle problem and how to solve them using pseudo code.
If you enjoy detailed problem-solving approaches like this, consider following my page for more content. You can also connect with me on LinkedIn, Twitter, and GitHub for updates and more coding insights.
Subscribe to my newsletter
Read articles from Koustav Hazra directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by

Koustav Hazra
Koustav Hazra
Spreading some of my knowledge — whatever I am learning on my journey to be a backend engineer.