4. Creating a Coupon Model and Coupon Store in .Net Core Minimal API
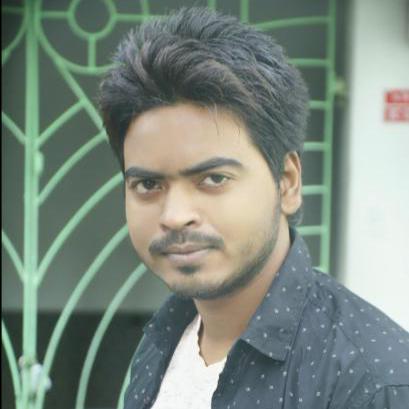
In this post, we'll explore how to create a coupon model and a coupon store in C#. Coupons are commonly used in e-commerce applications to offer discounts to customers. We'll define a Coupon class to represent individual coupons and a CouponStore class to manage a collection of coupons.
Let's start by defining the Coupon class:
public class Coupon
{
public int Id { get; set; }
public string Name { get; set; }
public int Percent { get; set; }
public bool IsActive { get; set; }
public DateTime? Created { get; set; }
public DateTime? LastUpdated { get; set; }
}
The Coupon class has properties such as Id, Name, Percent, IsActive, Created, and LastUpdated to store coupon details. The DateTime? type indicates that these properties can be nullable.
Next, let's define the CouponStore class:
public static class CouponStore
{
public static List<Coupon> couponList = new List<Coupon> {
new Coupon{Id=1,Name="10OFF",Percent=10,IsActive=true },
new Coupon{Id=2,Name="20OFF",Percent=20,IsActive=false }
};
}
The CouponStore class is a static class that contains a static list couponList
to store coupons. We initialize the list with some sample coupons for demonstration purposes.
To disable nullable reference types, you can add <Nullable>disable</Nullable>
to your project file.
That's it! You've now created a Coupon model and a CouponStore to manage coupons in your C# application.
Stay tuned for more tutorials on building e-commerce applications and managing data structures in C#!.
Subscribe to my newsletter
Read articles from Md Asif Alam directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
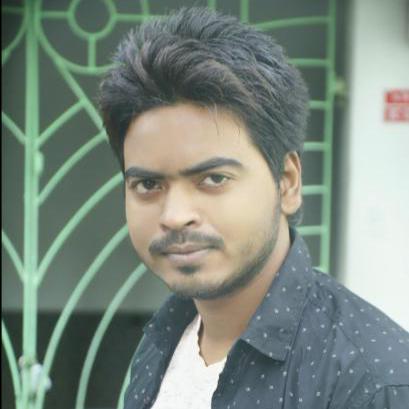
Md Asif Alam
Md Asif Alam
๐ Full Stack .NET Developer & React Enthusiast ๐จโ๐ป About Me: With 3+ years of experience, I'm passionate about crafting robust solutions and seamless user experiences through code. ๐ผ Expertise: Proficient in .NET Core API, ASP.NET MVC, React.js, and SQL. Skilled in backend architecture, RESTful APIs, and frontend development. ๐ Achievements: Led projects enhancing scalability by 50%, delivered ahead of schedule, and contributed to open-source initiatives. ๐ Future Focus: Eager to embrace new technologies and drive innovation in software development. ๐ซ Let's Connect: Open to new opportunities and collaborations. Reach me on LinkedIn or GitHub!